When one needs to take any number and find the multiple of which specific number it is, then the arithmetic operation that is to be put into use is division. But unlike addition and subtraction, this cannot be carried out with the fingers in our hands, one good reason why things must become a bit hasty when large chunks of data are being analysed.
With the series of articles in CodeforGeek focussing on carrying out basic arithmetic operations in Python, this article sets out to elaborate on the variety of techniques using which division can be done with aid of the divide( ) function in Python.
Also Read: Python Docstrings – Simply Explained!
Syntax of NumPy divide( ) Function
Let us get started by understanding the fundamental constituents of the divide( ) function with the help of its syntax given below.
numpy.divide(x1, x2, where=True, dtype=None)
where,
- x1 – Scalar or N-dimensional array which is the dividend
- x2 – Scalar or N-dimensional array which is the divisor
- where – Used to specify the locations within the array only for which the division is to be done, leaving the other entities unchanged
- dtype – Used to specify the type of data of the entities
The following code is used to import the numpy library before starting with the deployment of the divide() function:
import numpy as np
Examples of NumPy divide( ) Function
Let’s see examples of using the Python NumPy divide() function to divide scalars, arrays, an array by a scalar and arrays of different sizes.
Example 1: Dividing Scalars
On contrary to the arrays which contain a set of numbers, scalars are those which contain only a single number.
Given below is a code to demonstrate dividing scalars using the divide( ) function.
a = 10
b = 5
np.divide(a,b)
Output:
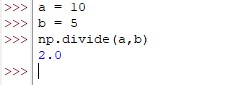
Example 2: Dividing Arrays
The logic shall be the same for the N-dimensional arrays too. But in this section, we shall have a look at dividing a pair of arrays of the same size. Yep! It is a pre-requisite that the arrays which are to be divided are of the same size for deploying the divide( ) function.
Given below is the code to demonstrate dividing two arrays which are declared by using the array( ) function from the numpy library in Python.
ar_1 = np.array([2,1,0])
ar_2 = np.array([4,3,5])
np.divide(ar_1, ar_2)
Output:
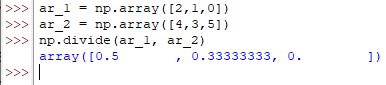
Example 3: Dividing Array & Scalar
This section will help us to understand how Python offers the agility to divide an array by a scalar entity by making the scalar entity as the denominator to divide each of the entities of the given input array.
Given below is an example to demonstrate dividing a two-dimensional array by a scalar.
ar_3 = np.array([[2,1,0],[3,4,5],[6,7,8]])
np.divide(ar_3, 10)
Output:
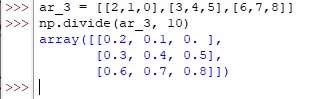
Example 4: Dividing Arrays of Different Sizes
Though in common practice, the arrays are to be of the same size for deploying the divide( ) function, there seems to be an exemption that can be exploited in Python. The same size is limited only to the number of columns in the input arrays, thereby making it possible to divide arrays with different row sizes to be divided against each other.
Let us have a look at the below example which demonstrates the division of a two-dimensional array using a one-dimensional array.
ar_2 = np.array([4,3,5])
ar_3 = np.array([[2,1,0],[3,4,5],[6,7,8]])
np.divide(ar_3, ar_2)
Output:
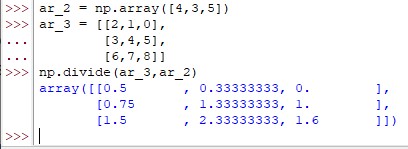
Note: It is imperative that we need to make sure that none of the elements in the divisor contain zeroes as its value, ignoring which the following error appears.

Conclusion
Now that we have reached the end of this article, hope it has elaborated on how to divide entities using the divide( ) function in Python. Here’s another article that can be your definite guide to Indentation in Python programming. There are numerous other enjoyable & equally informative articles in CodeforGeek that might be of great help to those who are looking to level up in Python. Whilst you enjoy those, adios!
Reference
https://numpy.org/doc/stable/reference/generated/numpy.divide.html