A MongoDB database has multiple collections. These collections can store millions of documents(data in MongoDB is called a document) and we can fetch all the data from a collection by performing a read operation using different methods like the collection.find(). These Read operations methods do not return documents instead they return a cursor.
This tutorial will help you to learn about MongoDB Cursor, its implementation, and different methods to get documents from the Cursor in NodeJS.
Also check: NodeJS MongoDB Rest API Course
What is a cursor in NodeJS?
A cursor pointed toward the documents which we tried to get. It can fetch the document in batches to reduce memory consumption and network bandwidth uses, as there can be millions of documents in a collection.
In the case of collection.find() method, if we haven’t passed any query then it returns a cursor that points toward all the documents of the respective collection.
const { MongoClient } = require('mongodb');
MongoClient.connect("mongodb://localhost:27017/", async function (err, client) {
if (err) throw err;
const database = client.db("newdatabase");
const cursor = database.collection("newcollection").find({}); // cursor
});
We have already covered the collection.find() method in another tutorial NodeJS MongoDB Select, if you want to read.
Using NodeJS Cursor to Fetch Documents
A cursor can use different methods to get the data to which the cursor points. Let’s see them one by one.
next() method
The next() method is used to get the subsequent document. In addition, we can use the hasNext() method to check whether the next document is present or not.
Example:
const { MongoClient } = require('mongodb');
MongoClient.connect("mongodb://localhost:27017/", async function (err, client) {
if (err) throw err;
const database = client.db("newdatabase");
const cursor = database.collection("newcollection").find({});
while (await cursor.hasNext()) {
console.log(await cursor.next());
}
});
Here we have created a loop that runs continuously until the cursor.hasNext() method returns false, when it returns false it means there is no next document present to fetch.
Output:
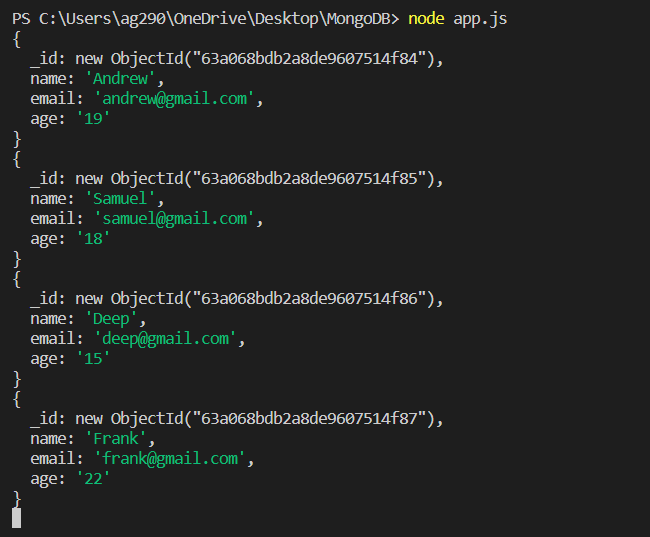
forEach() Method
This method can be used to iterate through the document to select every single document one by one.
Also Read: NodeJS Functions: A Beginner’s Introduction
Example:
const { MongoClient } = require('mongodb');
MongoClient.connect("mongodb://localhost:27017/", async function (err, client) {
if (err) throw err;
const database = client.db("newdatabase");
const cursor = database.collection("newcollection").find({});
await cursor.forEach(doc => console.log(doc));
});
Output:
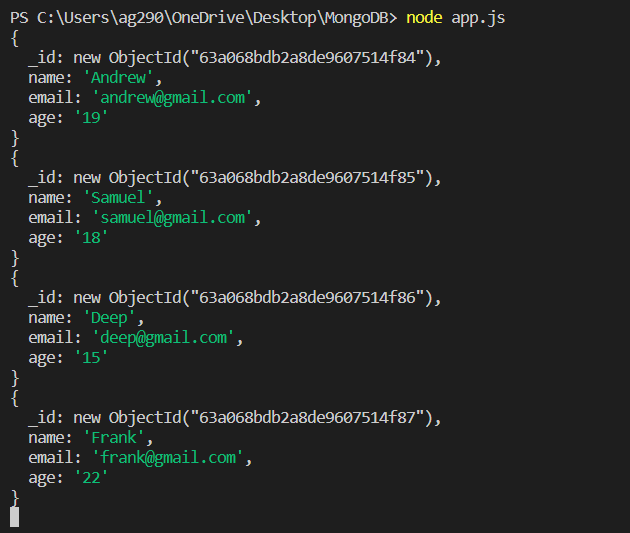
toArray() Method
This method can fetch all the documents at a time, if there is a large number of documents then this can cause operation failure or a decrease in performance.
Example:
const { MongoClient } = require('mongodb');
MongoClient.connect("mongodb://localhost:27017/", async function (err, client) {
if (err) throw err;
const database = client.db("newdatabase");
const cursor = database.collection("newcollection").find({});
const allValues = await cursor.toArray();
console.log(allValues);
});
Output:
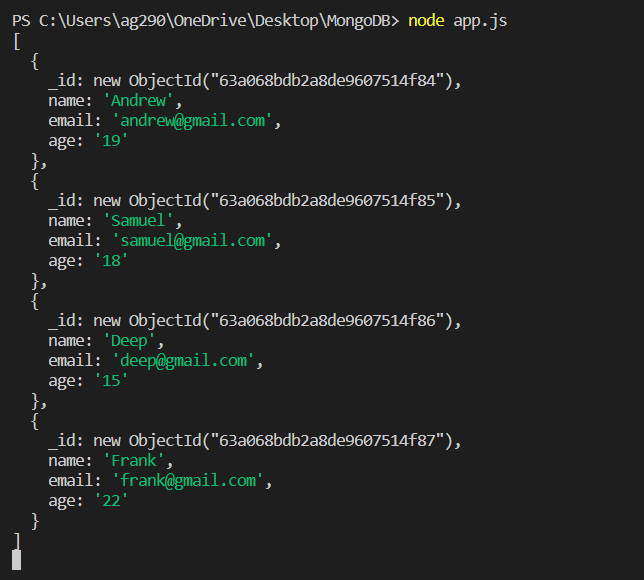
stream() Method
This method can be used to convert the cursor into Readable Streams to use Event APIs to get the documents.
We have already covered Streams in another tutorial Ultimate Guide to Understanding Streams in Nodejs and Events in Nodejs Events: What Are They and How Do They Work?, if you want to read.
Example:
const { MongoClient } = require('mongodb');
MongoClient.connect("mongodb://localhost:27017/", async function (err, client) {
if (err) throw err;
const database = client.db("newdatabase");
const cursor = database.collection("newcollection").find({});
cursor.stream().on("data", doc => console.log(doc));
});
Here we used the “data” event and a callback to print each object in the console.
Output:
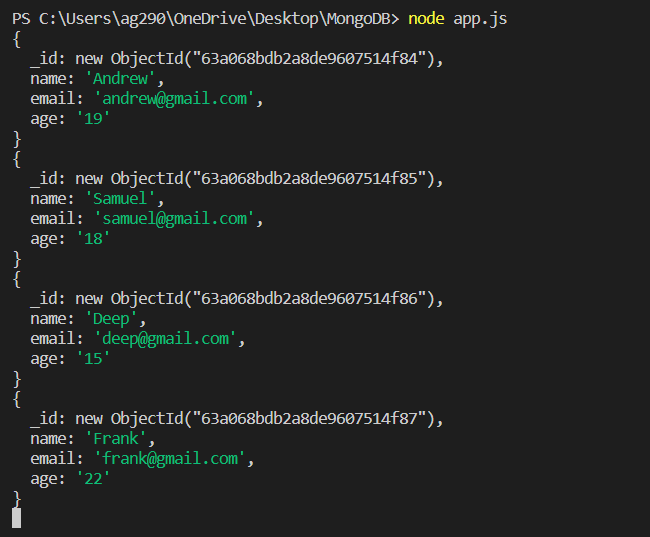
Asynchronous Iteration
NodeJS cursor supports async iteration, which means we can use the ‘for wait… of’ statement to fetch documents one by one asynchronously.
Example:
const { MongoClient } = require('mongodb');
MongoClient.connect("mongodb://localhost:27017/", async function (err, client) {
if (err) throw err;
const database = client.db("newdatabase");
const cursor = database.collection("newcollection").find({});
for await (const doc of cursor) {
console.log(doc);
}
});
Output:
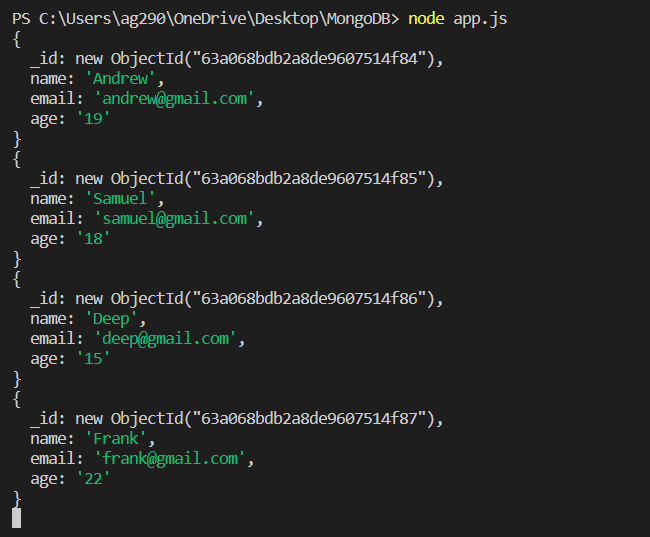
Summary
A MongoDB database uses collections to store documents and when we try to fetch documents from a MongoDB collection by performing read operations, it won’t return the documents rather it returns a cursor pointing toward the result, which fetches documents in batches to reduce memory consumption and network bandwidth uses. For getting the documents from the cursor we can use methods like next(), forEach(), toArray(), etc. Hope this tutorial helps you to learn about the MongoDB cursor in NodeJS.
Reference
https://www.mongodb.com/docs/drivers/node/current/fundamentals/crud/read-operations/cursor/