A class is a concept of Object Oriented Programming(OOP). Object Oriented Programming is an essential concept in programming, it helps to reduce the difficulty of scaling the application.
It provides a way to concatenate various method and property to create a blueprint from which multiple instances is created to increase the useability and reduce the redundancy in code. This instance of a class is called an object.
Javascript before the addition of classes
Class in JavaScript was introduced in EcmaScript 2015(ES6) before classes the constructor function is used to perform Object Oriented Programming(OOP) in JavaScript.
Below is the code to do OOP using the constructor function:
function Hello(name) {
this.name = name;
}
const hello = new Hello('John'); // object
Suppose it is required to add a function to the constructor to call it using its object then the code will be written as:
function Hello(name) {
this.name = name;
}
Hello.prototype.welcome = function() {
console.log(`Welcome ${this.name}`);
}
const hello = new Hello('John');
hello.welcome(); // Welcome John
The same code using the class will be written as:
class Hello {
constructor(name) {
this.name = name;
}
welcome() {
console.log(`Welcome ${this.name}`);
}
}
const hello = new Hello('John');
hello.welcome(); // Welcome John
Here you can see that the class makes the overall code simpler and more stable.
Classes in Javascript
The class in JavaScript is a function, the difference is that a function is declared with the function() keyword, whereas a class is declared using the class keyword.
Example:
const class1 = class {
constructor(name) {
this.name = name;
}
};
const fun1 = function(name) {
this.name = name;
}
console.log(class1);
console.log(fun1);
Here you can see the prototype of an object of both function and class are the function(i.e same).
Output:
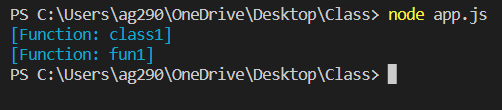
Defining a class
A class is defined by a class keyword followed by a name, then a curly bracket to define the constructor function and the rest of the class body.
A constructor function is called when an object is created from the class, it initializes the object value by letting it take arguments during its creation.
Syntax:
class classNamae {
constructor(args) {
// constructor body
}
// class body
}
Example:
Below is the code having a class ‘hello’ that has a constructor that accepts a parameter ‘name’.
class Hello {
constructor(name) {
this.name = name;
}
}
Let’s create an object ‘hello’ from the ‘Hello’ class with an argument ‘John’.
class Hello {
constructor(name) {
this.name = name;
}
}
const hello = new Hello('John');
The argument passed during the creation of the object used by the construct to initiate its value. Let’s print the object using the console.log() method.
class Hello {
constructor(name) {
this.name = name;
}
}
const hello = new Hello('John');
console.log(hello);
Output:
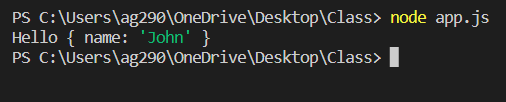
Here we see the object is having a key ‘name’ with the value passed during the object creation.
Multiple Methods in Class Definition
A class definition can contain multiple methods that can be called using its objects.
Example:
class Hello {
constructor(name) {
this.name = name;
}
welcome() {
console.log(`Welcome ${this.name}`);
}
bye() {
console.log(`Bye ${this.name}`);
}
}
const hello = new Hello('John');
hello.welcome();
hello.bye();
Output:
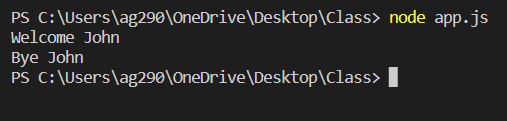
Multiple Objects of a Class
The ability to create many objects of a class is used to reduce the redundancy in code.
Example:
class Hello {
constructor(name) {
this.name = name;
}
welcome() {
console.log(`Welcome ${this.name}`);
}
bye() {
console.log(`Bye ${this.name}`);
}
}
const hello = new Hello('John');
const hello2 = new Hello('Jane');
const hello3 = new Hello('Jack');
hello.welcome();
hello.bye();
hello2.welcome();
hello2.bye();
hello3.welcome();
hello3.bye();
Output:
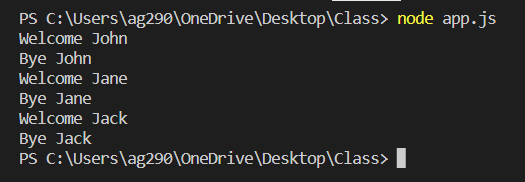
Javascript hoisting
In JavaScript, the function call can be present before the function declaration, but an object is not created from a class before its declaration.
Example:
const hello = new Hello('John');
class Hello {
constructor(name) {
this.name = name;
}
}
console.log(hello);
Output:
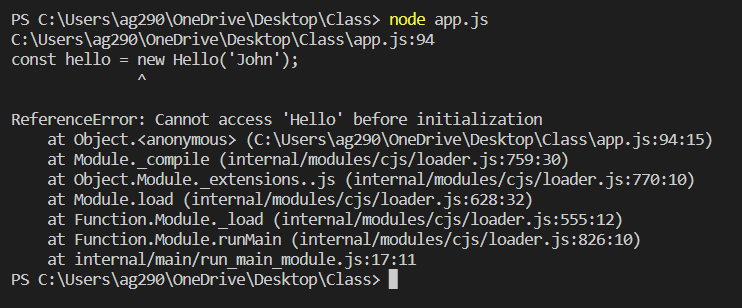
Here you have noticed that the function is called successfully before its declaration whereas JavaScript throws the reference error for the object.
Summary
Class is an essential part of Object Oriented Programming, it provides a way to combine various methods and data types that can consume by its objects, in short, it is a blueprint for its object. A class can contain multiple methods that can access by its objects. Multiple objects can be created from a class to avoid redundancy in code. Hope this tutorial helps you to learn about the concept of class in JavaScript.