Let’s say you have just been hired as a Node.js developer, you have the task of building a module that does something when a certain event occurs. For instance, if the user needs some data and that data does not exist, some code must be triggered to properly handle the error. As a beginner, you may think of creating this functionality by building a function to handle the error and wrapping that function inside an if statement that has the event as a condition. If you do this you will be fired from your job. Here comes the Node.js Events to save your job.
Node.js Events provides a way to create custom events that can run asynchronously to perform different operations.
In more technical terms, Event is an instance of the EventEmitter class which can be used to create events to handle asynchronous operations by triggering some defined functions (listeners) on a specific occasion (event).
Let’s understand this in more depth throughout this tutorial.
Events in JavaScript vs. Node.js
You may already be familiar with events in JavaScript, let’s see if it is similar to Node.js or what are the differences.
Events in JavaScript
In JavaScript, an event is a way to detect the different types of responses from the users to perform various operations. The functionality of events in JavsScript is defined by the programmers but triggered by the users.
For example, a programmer can set what happens when a user clicks on a button using the onClick event.
Events in Node.js
The event in Node.js is quite different, they are created by programmers and are also triggered by programmers in different places to perform the operation defined during the creation of that event.
For example, a programmer defines an event that sends an email, then it is also triggered by the programmers in different places when there is a need to send an email like during the signup, login, forget the password, etc.
Node.js Event Module
For using the Events in Node.js it is required to import it.
Syntax:
const EventEmitter = require('events');
The EventEmitter Object
For calling the method, we have to create an EventEmitter object using the below statement.
Syntax:
const EventEmitter = require('events');
const emitter = new EventEmitter();
Let us now look at some of the methods of the EventEmitter class for handling events.
Methods to Add Lishners to Events
There are three methods to add listeners to an event.
- addListener
- on
- once
addListener
This method is used to add listeners to an event. We can add multiple listeners to an event. Behind the scenes, there is an array attached to a particular event that contains all the listeners for the event.
Syntax:
addListener(event, listener)
where,
- event is the string containing a name that will be used to emit the event.
- listener is a function that defines the functionality of the event.
on
This method is the same as the addListener method. It also adds a listener to the listener array for the specified event. It provides a shorter form which convenient to use.
Syntax:
on(event, listener)
once
This method is used to attach a single listener to an event that can emit only one time, then it is automatically removed.
Syntax:
once(event, listener)
Methods to Remove Listeners from Events
There are several listeners attached to a particular event and to remove them, we have two methods.
- removeListener
- removeAllListeners
removeListener
This function takes two arguments, the first is the event, and the second is the listener you want to remove from that event.
Syntax:
removeListener(event, listener)
removeAllListeners
This function takes an event as an argument and removes all listeners attached to it. Basically, it deletes all the listeners from the listener array for the specified event.
Syntax:
removeAllListeners(event)
Emit the Event
Emitting the event means triggering or calling the event. We can call events anywhere between the programs by using the below method. When an event is triggered its associated listener is executed.
emit
This method is used to emit the events. As arguments, it takes an event to emit it and multiple arguments that used as arguments for listeners attached to that event.
Syntax:
emit(event, arguments)
Complete Example of Using Node.js Events
Let’s understand the practical use of every single method we have learned by using a simple example.
const EventEmitter = require('events');
const emitter = new EventEmitter();
// create listerners
const listener1 = (name) => {
console.log("Hello " + name);
}
const listener2 = (name) => {
console.log("Goodbye " + name);
}
const listener3 = (name) => {
console.log(name + ", this listener will remove!");
};
// add listerner using addListener method
emitter.addListener("event", listener1);
// add listerner using on method
emitter.on("event", listener2);
emitter.on("event", listener3);
// emit event
emitter.emit("event", "John");
// remove listerner3 using removeListener method
emitter.removeListener("event", listener3);
console.log("------Listner3 removed------");
// emit event
emitter.emit("event", "John");
// remove all listerners using removeAllListeners method
emitter.removeAllListeners("event");
console.log("------All listeners removed!------");
// emit event
emitter.emit("event", "John");
Output:
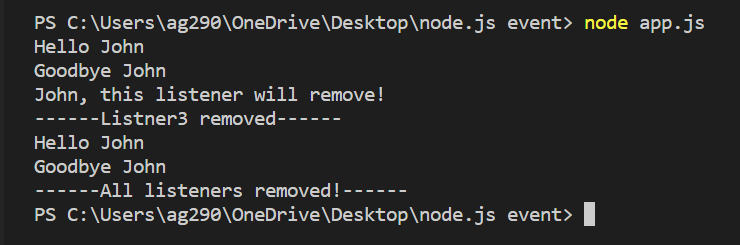
Some More Useful Methods for Node.js Events
listeners
This method takes an event as an argument and returns an array of listeners for that event.
Syntax:
listeners(event)
setMaxListeners
By default, the EventEmitters will print a warning if more than 10 listeners are attached to a particular event. But by using this method we can increase the number of the max listener.
Syntax:
setMaxListeners(n)
where n is the number of listeners.
Frequently Asked Questions (FAQs)
What are Triggers vs Events?
Triggers run automatically when some predefined actions occur, on the other hand, events can be run or emitted manually by the programmer if needed while writing the program. To emit an event, we need to run the emit() method with the name of the event as a parameter.
How Node.js is event-driven?
Node.js is event-driven as it depends heavily on events to handle a variety of operations. An event with Node.js is created using the EventEmitter class from the Node.js Events module. Node.js uses events everywhere, from asynchronous operations, I/O operations, and handling the network, to performing multiple tasks simultaneously.
Why use events in Node.js?
Events in Node.js are created using the EventEmitter object which is used to define listeners or handlers that are used to handle a variety of operations asynchronously based on the occurrence of a specific event. When an event is emitted, the listener associated with it is triggered and the specified operation is performed.
What does it mean for an event to be emitted?
Emitting an event means firing or calling an event. When an event is fired, its associated listeners execute and perform the specifically defined action. The emit() method is used to emit an event.
What is the callback in Node.js?
The callback in Node is a function passed as an argument to an asynchronous function. Once the asynchronous function completes its execution, the code inside the callback function will be executed.
Read More: Getting Data from RESTful API in Node
Summary
In Node.js custom events can be created, which can be called asynchronous without blocking the execution of other blocks of code. This event contains an array of all listeners attached to it. We can add max 10 listeners to an event, but it can be increased using the setMaxListeners function. We can remove a particular listener from an event or remove all the listeners associated with an event. Hope this article helps you to understand the concept of Events in Node.js.
Reference
https://nodejs.org/api/events.html#events