Nowadays everyone has to use WhatsApp whether they are in a corporate background or just for communicating between friends and family. The app has seen numbers of users of over 2 billion in a month. In India alone, more than 340 million users are active every month. Sometimes there are some repetitive tasks that can be done with a bot, so that’s what we’ll be doing in this article.
Disclaimer: This is only a fun project to see how NodeJS can interact with browser elements. WhatsApp does not allow bots without the official API.
How to connect your Whatsapp account to the Bot?
To connect your whatsapp account to the bot we’ll be using the whatsapp-web.js module. This awesome library will allow us to connect our bot to the WhatsApp-web interface which we’ll be running under the hood. So first and foremost we’ll go ahead and install this into our node modules by running the following command:
npm i whatsapp-web.js
Install the dependencies
If you are planning to run this bot on a no-GUI-Linux server, then there are some dependencies so that the puppeteer can go ahead and emulate the chromium browser correctly, for that run the following command:
sudo apt install -y gconf-service libgbm-dev libasound2 libatk1.0-0 libc6 libcairo2 libcups2 libdbus-1-3 libexpat1 libfontconfig1 libgcc1 libgconf-2-4 libgdk-pixbuf2.0-0 libglib2.0-0 libgtk-3-0 libnspr4 libpango-1.0-0 libpangocairo-1.0-0 libstdc++6 libx11-6 libx11-xcb1 libxcb1 libxcomposite1 libxcursor1 libxdamage1 libxext6 libxfixes3 libxi6 libxrandr2 libxrender1 libxss1 libxtst6 ca-certificates fonts-liberation libappindicator1 libnss3 lsb-release xdg-utils wget
Now you are ready. Whatsapp-web uses a QR code to authenticate the client and hence we’ll also need to install another module called qrcode-terminal
npm i qrcode-terminal
Writing the first part of the bot
Now we can go ahead and write our first bit of code.
const { Client } = require('whatsapp-web.js');
const client = new Client();
client.initialize();
client.on('qr', (qr) => {
console.log('QR RECEIVED', qr);
});
client.on('ready', () => {
console.log('Client is ready!');
});
The code above is setting up a new WhatsApp Web client instance, waiting for the QR code to be received, and logging a message to the console when the client is ready. It is using the whatsapp-web.js library to set up the client and connect it to the WhatsApp platform.
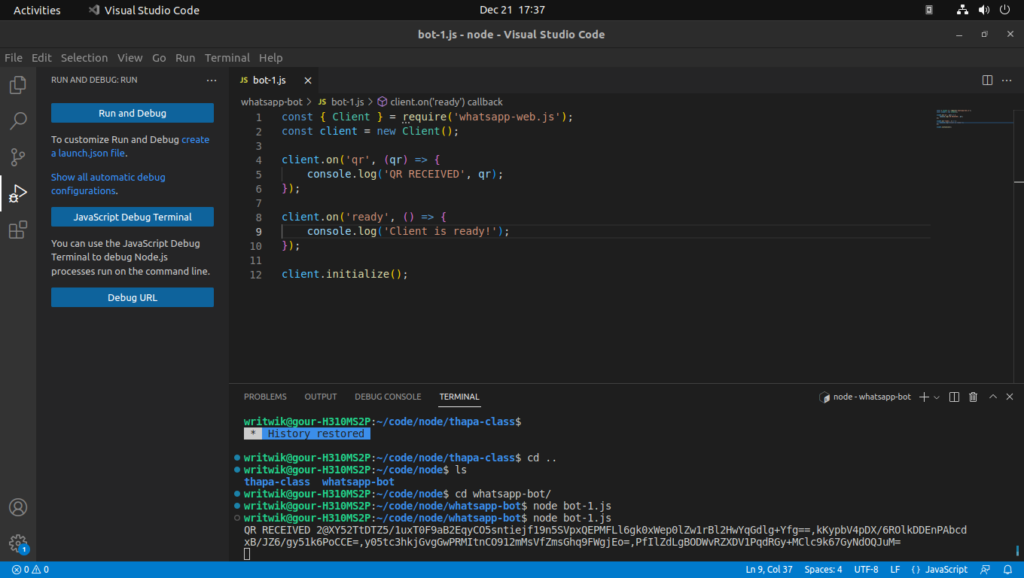
Logging into WhatsApp with the QR code
Now we simply have to integrate the qr-code library and pass in the qr code to that to see the qr-code in the terminal.
client.on('qr', qr => {
qrcode.generate(qr, {small: true});
});
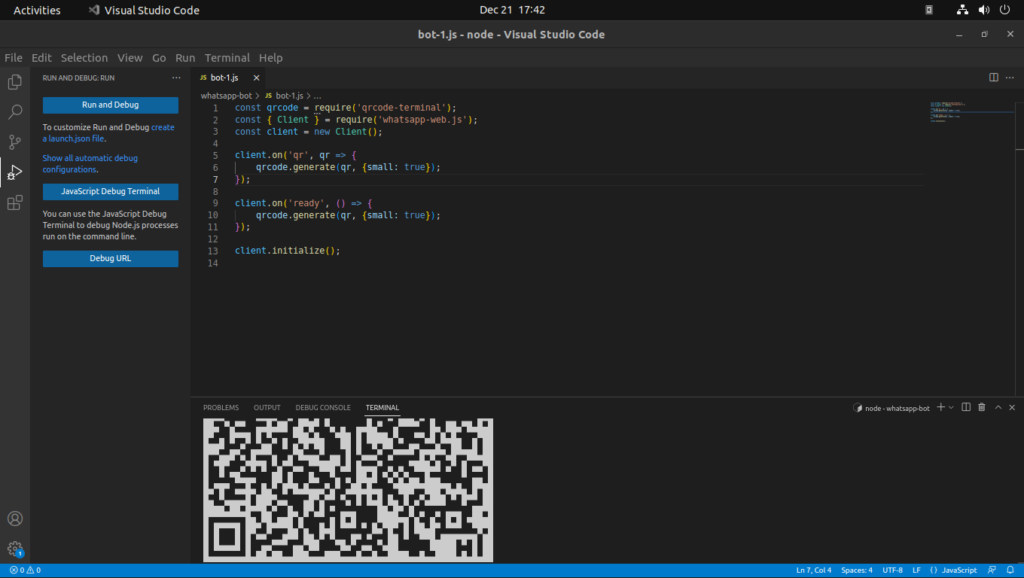
Now we can go ahead and scan the qr-code simply as we would while using the whatsapp-web interface. You can see that after we successfully scan the code there will be console output stating that “client is ready”.
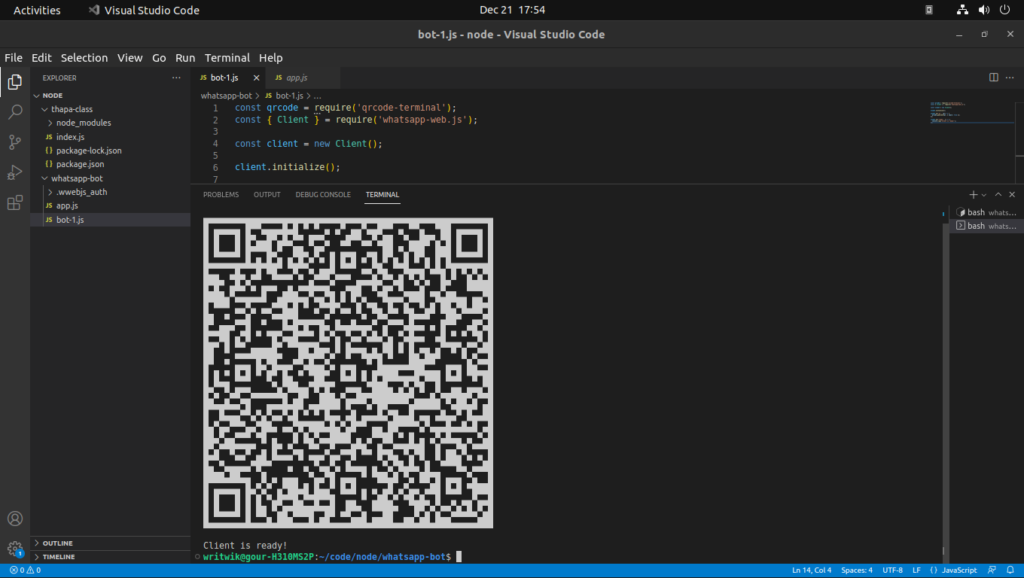
Creating a WhatsApp authentication service
To avoid scanning the qr-code every time we fire up the node-app, we can create an authentication service. This is what the code looks like after adding in the functionality:
const qrcode = require('qrcode-terminal');
const { Client, LocalAuth, MessageMedia } = require('whatsapp-web.js');
const client = new Client({
authStrategy: new LocalAuth(),
});
client.initialize();
client.on('qr', (qr) => {
qrcode.generate(qr, { small: true });
});
client.on('authenticated', () => {
console.log('Authenticated');
});
client.on('ready', () => {
console.log('Client is ready!');
});
Now in the next section we’ll see how to listen for messages and reply to them.
Listen for texts and reply to them
Now that we have successfully connected the bot to our account we can listen for messages and reply to them. See the below code:
client.on('message', message => {
console.log(message.body);
});
The above code simply prints all messages to the console, you can first connect the bot by scanning the qr code and then any incoming texts to that account will be printed onto your console. We can simply set a filter and if the body matches we can reply to it with something pre-defined. We send messages by the following method:
client.on('message', message => {
if(message.body === '!ping') {
message.reply('pong');
}
});
The above code replies to only “!ping”.
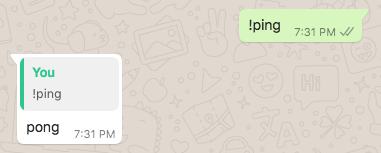
Integrating cool functionalities into the bot
Now that we have a method to listen for codewords and reply to them we can make it a little fun and add in our favorite functionalities to reply to the codewords.
For our first mini=project let’s make a quote-bot. We will first install the quotable package in our node modules and whenever a user sends us the keyword “!quote” we’ll reply with a quote. The code is as follows:
const quotable = require('quotable');
client.on('message', async (message) => {
if (message.body === '!quote'){
const aNewQuote = await quotable.getRandomQuote();
//console.log(aNewQuote.content)};
message.reply( aNewQuote.content + " " + "-" + " " + aNewQuote.author )}
});
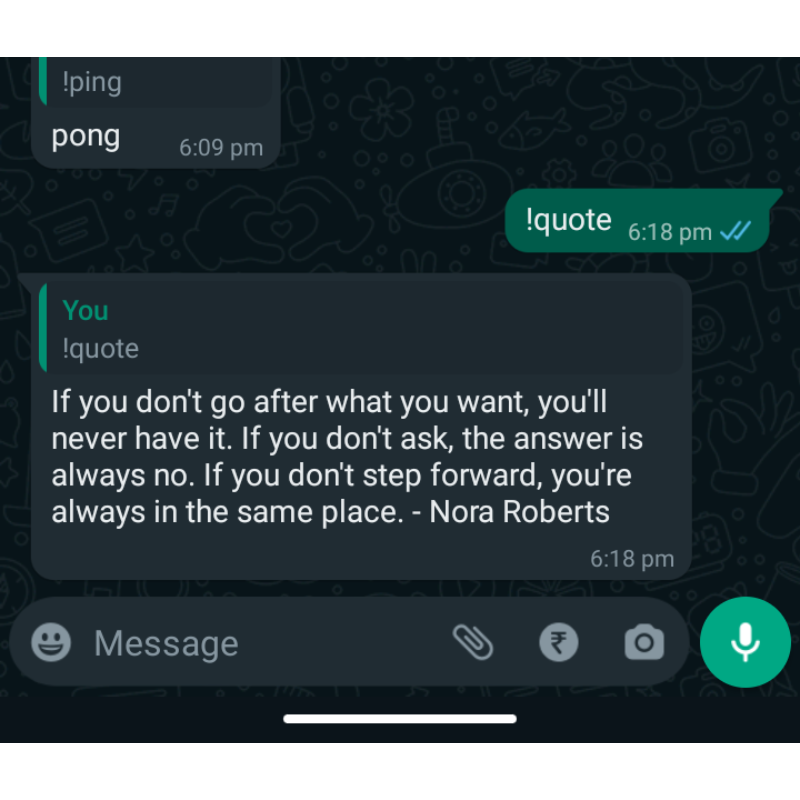
Conclusion
So there you go! Whipping up a WhatsApp bot is an easy task for you now, maybe do a prank on someone you know? or just have fun. This project is not a complete alternative to Whatsapp API, however, for some light use cases, this is a great way to go. You can also keep the bot running by hosting it in your spare laptop, it is very minimal especially if you use it in a no-GUI-Linux environment.