Express.js is one of the most popular Node.js frameworks developers use to create servers and web applications. Suppose you have set up a basic Express server and you want to send an HTML file on different route URL requests to make the server act as a web app, you can do so by implementing the Express res.sendFile() method. In this article, we will explore this method and use an example to demonstrate its use.
Express res.sendFile() Method
Express provides a method in the response object of the router called sendFile() that can be used to serve static files.
You can send files directly from the Express router using this method. You can send a file of any type such as HTML, PDF, Multimedia, etc as a response to a client.
res.sendFile() method accepts absolute paths only. You can use express.static() to set the path.
Syntax:
res.sendFile(filePath[, options] [, callback])
Parameters:
- filePath: file path can be an absolute path to the file or a relative path to the correct working directory, which you want to send,
- options: options can be an optional argument containing additional conditions,
- callback: callback can be any callback function where we can handle the error if occurs
Return:
It doesn’t return any value, you can get the error inside the callback if you want.
Example of Using res.sendFile() Method
In this section, we will demonstrate the use of the res.sendFile() method for sending an HTML file by creating a very basic Express application.
Below is the step-by-step implementation for sending an HTML file in Express.js.
Step 1: Let’s start by creating a project file, locating it in a terminal and initialising a Node.js project using the below command.
npm init -y
Step 2: Then install the Express module using the below NPM command.
npm install express
Step 3: Once the installation is done, create a new folder called public folder and add a file “home.html” to this folder having the below code.
<html>
<head>
<title>Home</title>
</head>
<body>
<p>This is a test file!</p>
</body>
</html>
Step 4: Now create a JavaScript file “ app.js “ for writing the server side and write the below code to demonstrate the use of the res.sendFile() method.
const express = require('express');
const router = express.Router();
const app = express();
app.use(express.static('public'));
router.get('/', (req, res) => {
res.sendFile('home.html');
});
app.use('/', router);
app.listen(3000);
In the first line, we imported the Express module, then in the second line we created an instance of the Express router to define routes separately, then we created an instance of Express “app.js“, then defined a public directory to serve static files using the express.static() as middleware, then we have created a router for root route, where we have used sendFile() method to send HTML file as a response, finally we have used the listen() method to make the application listen on port 3000.
Step 5: You can run the above code by executing the below command.
node app.js
As you may notice, we are using express.static() as middleware to set the static path. If you want to know more about express.static() method, check out: Serving Static Files in Express Framework
Output:
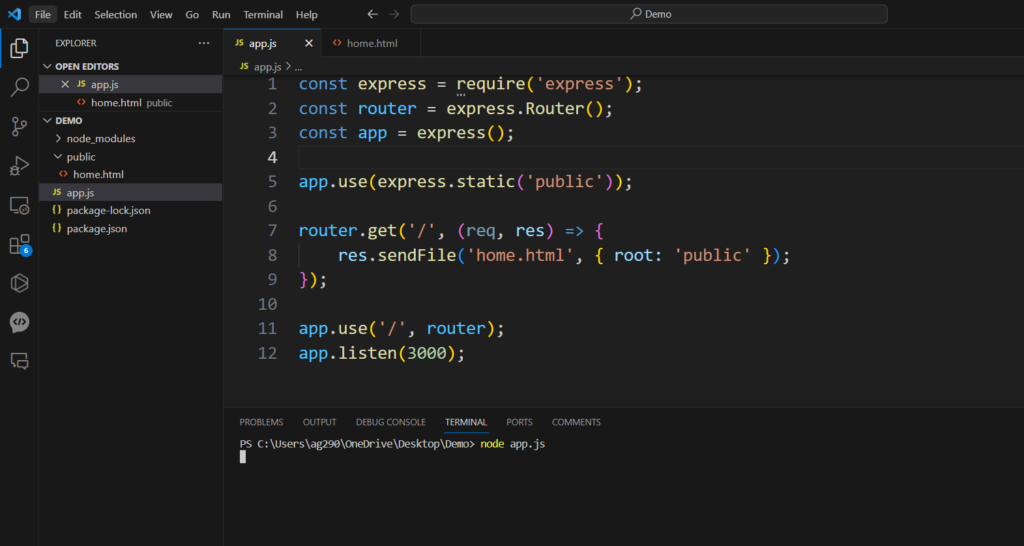
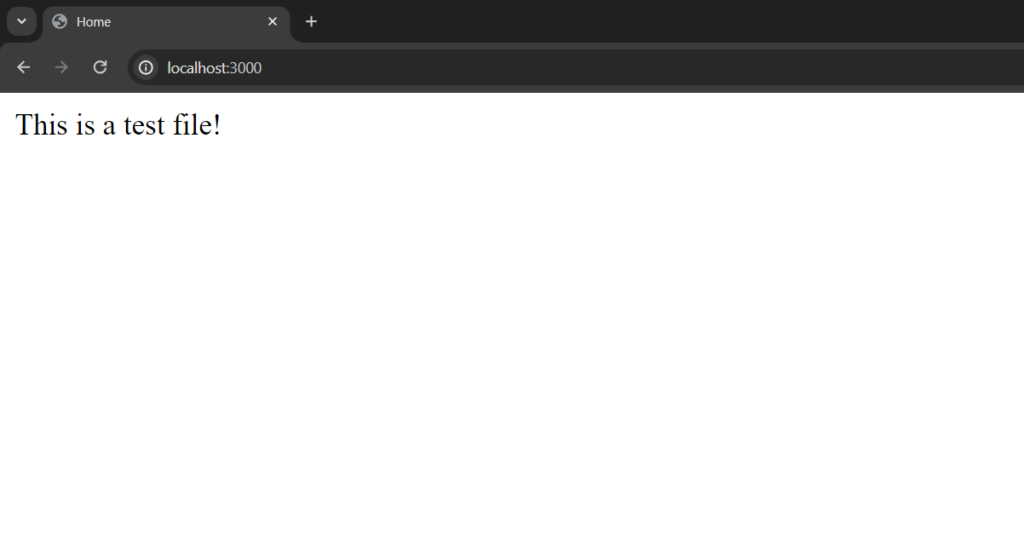
Difference Between res.send() and res.sendFile() in Express
The res.send() method is used to send an HTTP response to the client. It is used more often to handle various types of responses. It automatically detects the type of data that will be sent as a response and sets the right Content-Type header. We can use it to send various types of data like strings, HTML, JSON, Buffer objects, and arrays.
On the other hand, the res.sendFile() method is specified to be used to send files as a response. It allows us to send a file from the server filesystem to the client. You just have to pass the path of the file with the filename and you can also specify the root directory. We can use it to send static files like HTML, images, CSS, or client-side JavaScript files.
Summary
Express.js proved to be the best Node.js framework for building web applications, it comes with various features. One of them is the res.sendFile() to send static files as a response to the users. In this tutorial, we have seen the usage of the res.sendFile() method along with its syntax and properties. We have also demonstrated this using the example of sending HTML as a response using the Express route. This method is so simple and useful that you can directly implement it in your Node.js and Express.js applications where you need to send an HTML file from the server to the client making the request. Hope you have enjoyed reading the content.
Reference
https://stackoverflow.com/questions/25463423/res-sendfile-absolute-path