Data Access is an essential feature of any application. An application that can’t have access to data is nothing but a static website, these websites hardcode the information on their page, they are exhausting, hard to update, and not scalable. Even a simple blog website also accesses the data to render different information.
Examples of data accessing are blog websites that access data to show blogs to the users, Facebook access data to show your details, profile, and friend list, Google Calendar access data to show the upcoming event, etc.
We also accessing our data to show you these extraordinary tutorials, but have you wondered how we can access data in NodeJS?
This tutorial guides you toward data access in NodeJS from different types of databases.
Also read: NodeJS MySQL Create Database and NodeJS MongoDB Create Database
Data Access in Node.js From Different Types of Databases
Data Access can be easily done in NodeJS, yet it is required to know from exactly which type of database we want to access data. The database can be relational data like MySQL, or non-relational like MongoDB.
NodeJS has the ability to access data from both types of databases. Let’s see them one by one.
Accessing Data From MongoDB Using Node.js
MongoDB is best suited for NodeJs, for Data Access from the MongoDB database, NodeJS has a module ‘mongodb’ which can interact with the MongoDB database.
Syntax:
The Syntax of including the ‘mongodb’ module for data access from the MongoDB database is given below.
const MongoDB = require('mongodb');
We have some documents (data in MongoDB is called documents) inside the MongoDB Database locally on our pc, let’s access them using NodeJS.
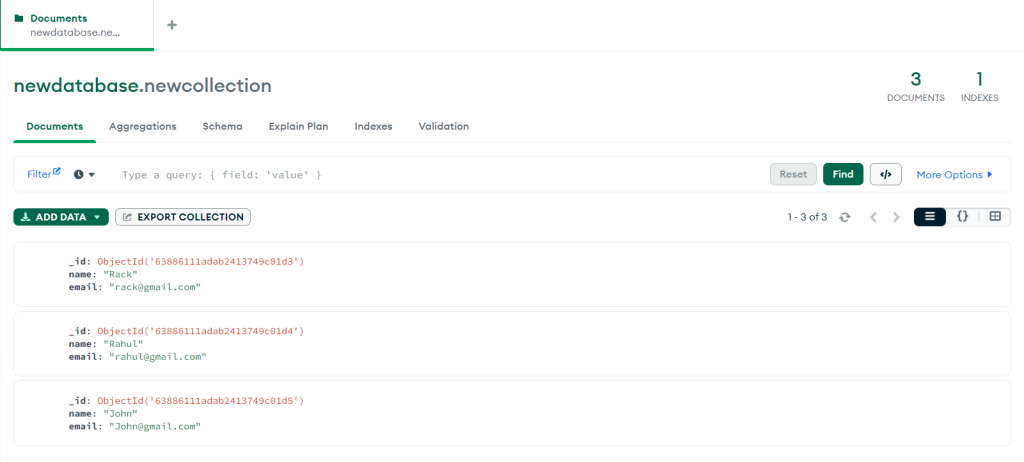
We have a separate tutorial NodeJS MongoDB Insert which covers the entire process to insert documents in the MongoDB database using NodeJS if you wanted to insert data to try out data access.
Below is the code to access data from MongoDB Database:
const { MongoClient } = require('mongodb');
MongoClient.connect("mongodb://localhost:27017/", function (err, client) {
if (err) throw err;
const database = client.db("newdatabase");
database.collection("newcollection").find({}).toArray(function(err, documentsArray) {
if (err) throw err;
console.log(documentsArray);
});
});
Here we use MongoClient from ‘mongodb’ module to make a connection to the MongoDB server, which is covered in another tutorial.
Then we use the client object to access the database, the database object to access the collection, and the find() method to access data from the collection. We have covered the entire process in detail in a separate tutorial NodeJS MongoDB Select if you want to dive deep into different methods used in the above code.
Run the Code:
Create a JavaScript file ‘app.js’ and write the above code, locate the file in the terminal, and type the below command to install the MongoDB module.
npm install mongodb
Then execute the below command to run the code.
node app.js
Output:
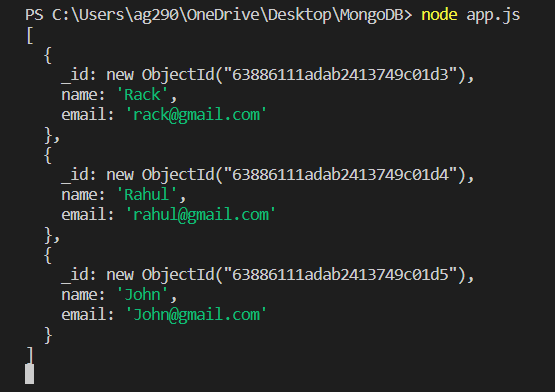
Accessing Data From MySQL Using Node.js
For Data Access from MySQL database, NodeJS has a module ‘mysql’ which can interact with MySQL database.
Syntax:
The Syntax of including the ‘mysql’ module for data access from MySQL database is given below.
const mysql = require('mysql');
We have some data inside the MySQL Database locally on our pc, let’s access them using NodeJS.
We have a separate tutorial NodeJS MySQL Insert Record which covers the entire process to insert data in the MySQL Database using NodeJS if you wanted to insert data to try out data access.
Below is the code to access data from MySQL Database:
const mysql = require('mysql');
const con = mysql.createConnection({
host: "localhost",
user: "root",
password: "",
database: "newdatabase"
});
con.connect(function (err) {
if (err) {
throw err;
}
});
con.query("SELECT * FROM temptable", function (err, result) {
if (err) throw err;
console.log(result);
});
Here we first make a connection to the MySQL server, which is covered in NodeJS MySQL Create Connection tutorial.
Then we use the connection object to access the data, which is covered in the NodeJS MySQL Select Record tutorial.
Run the Code:
Create a JavaScript file ‘app.js’ and write the above code, locate the file in the terminal, and type the below command to install the MySQL module.
npm install mysql
Then execute the below command to run the code.
node app.js
Output:
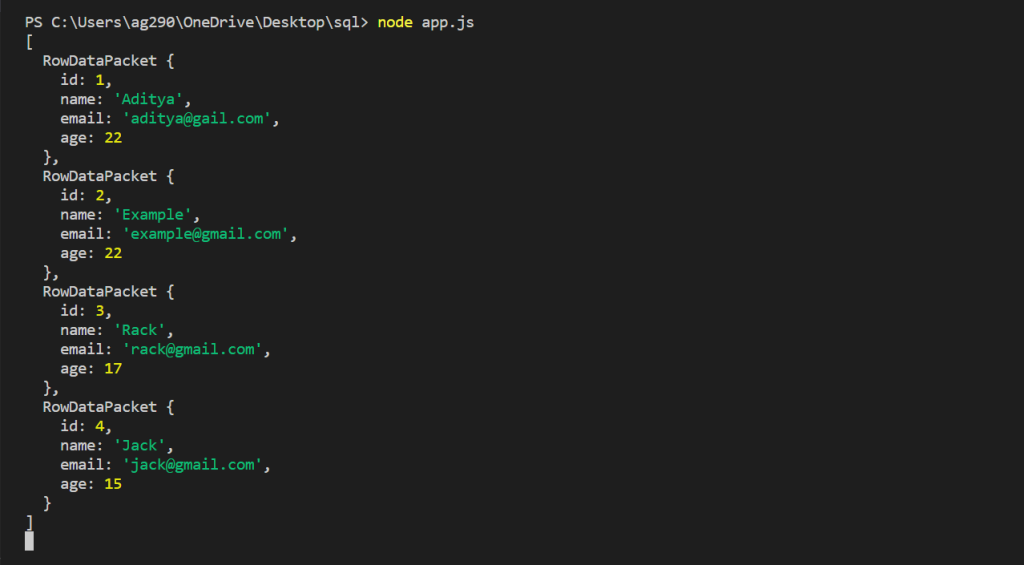
Summary
Data is everything, and the ability to data access helps in scaling the application. NodeJS can access data from different types of databases, whether relational or non-relational. For accessing data from different databases, NodeJS has respective modules that you can easily import to get started. Hope this tutorial helps you to access data in NodeJS.
Reference
https://www.npmjs.com/package/mysql
https://www.npmjs.com/package/mongodb