Lately, I created an application and put it on the server, now people can connect to my app, use it and do all types of operations. But I have a big problem: I can’t monitor the logs constantly and can’t always be connected to the server, so to check whether my application works properly, I wrote code for Node.js to log to a file instead of the console. Let’s see how I have done this.
Node.js console.log() Function
The console.log() method in Node.js can print any sort of variable as well as any message that needs to be shown in the console. By using this method developers can debug their Node.js application.
Example 1:
Anything inside console.log() can be printed to the console.
const msg = "Hello world!";
console.log(msg);
const numbers = 4;
console.log(numbers);
console.log("Hello world 2");
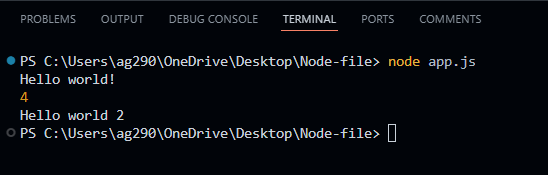
Example 2:
The ability to log anything can give us the power to log even errors or log custom messages when some operation fails.
async function processData() {
const data = await fetchData();
if (data) {
console.log('Data fetched successfully:', data);
} else {
console.log('Fetching data is not available.');
}
}
In this case, Node.js log the specified message to the console if fetching data is not available.
If you want to know more about console.log(), click here.
Implement Node.js Logging to File
Now if you have written a lot of console.log() statements in your program and you think that you can monitor everything and make relevant changes to make the app error-free, then how can you monitor the logs all the time? Instead of logging the console.log() output to the console, why not save it to a file and then check all the logs produced by the app later or on a daily basis?
Look at the below code:
const fs = require('fs');
const path = require('path');
const logFilePath = path.join(__dirname, 'app.log');
console.log = function(message) {
fs.appendFile(logFilePath, message + '\n', (err) => {
if (err) {
console.error('Error appending to log file:', err);
}
});
};
console.log('This message will be logged to file only');
Here we use ‘fs’ to interact with the file, we basically use its method fs.appendFile to insert the messages to the file, we use the ‘path’ module to get the path of the file where we want to store the logs.
The main code is very simple, we actually overwrite the console.log() function with a custom function that takes the argument passed into it and saves it to a file, simple right?
After running the script, a new file containing the logs is created:
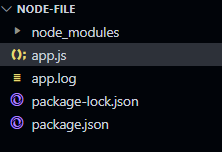
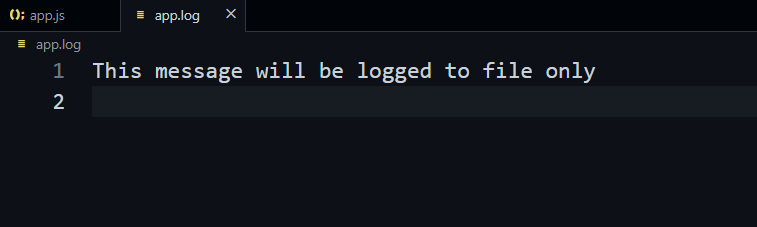
But there is no log in the actual console:
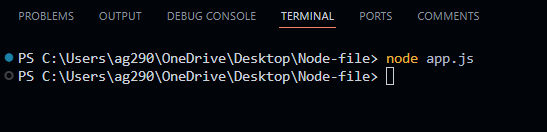
Let’s solve this issue.
Save a Reference to the Original console.log
Now after overriding the function, the logs start saving to file, but the regular logs stop printing to the console and you cannot see any more logs on the console.
To make sure the messages still print to the console as usual, you can save a reference of the original console.log.
Here is the updated code:
const fs = require('fs');
const path = require('path');
const logFilePath = path.join(__dirname, 'app.log');
console.originalLog = console.log;
console.log = function(message) {
fs.appendFile(logFilePath, message + '\n', (err) => {
if (err) {
console.error('Error appending to log file:', err);
} else {
console.originalLog(message);
}
});
};
console.log('This message will be logged to file and console');
After running the script, again a new file containing the logs is created:
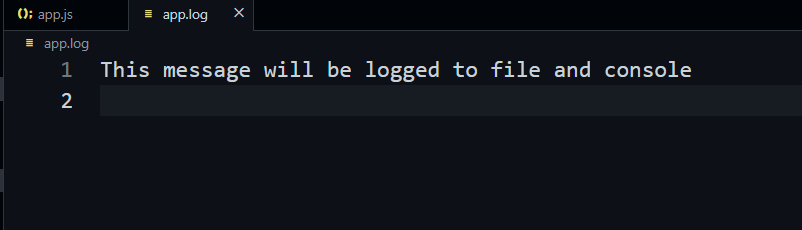
In this case, the logs are also shown in the console:
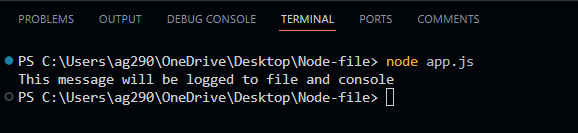
And here we go. This is the final block of code I used in my app to keep track of my logs and save them to a file.
Conclusion
In conclusion, if there are a lot of logs on your Node.js application and you are putting your app on a server then it is hard to keep an eye on them and sometimes your app may restart or lose your logs. So to keep track of them we can overwrite the console.log to get the arguments passed into it and use the ‘fs’ module to add them to a file so you can check them whenever you want and miss no single log.
Read More – Node.js Console Methods (8 Methods to Know)
Reference
https://nodejs.org/api/console.html