MongoDB is a NoSQL database that can store a large amount of unstructured data. Data stored in MongoDB are easy to retrieve as it can hold them in flexible formats depending upon the need such as graphs, key values, documents, etc.
Creating a MongoDB Database in Node.js
In MySQL, we have queries to perform different functions such as creating a database, and creating a collection as it supports SQL, but MongoDB is NoSQL, it does not have any query.
A Database can be created during the connection of NodeJS with MongoDB.
To make a connection between NodeJS and MongoDB the connect() method of MongoDB Client is used. This method uses a URI as an argument where data will be stored and a callback function to define the rest of the functionalities.
MongoClient.connect(URI , callback);
If you find the above code confusing, kindly read How to Connect MongoDB on Localhost 27017 in which we have explained the process of connecting NodeJS with MongoDB.
The database name is passed at the end of the URI after a slash “/” to create the database. Then inside the callback, we can get the error or the database that is just created. If the database we are trying to create already exists then it simply returns it inside the callback which can be further used for creating the collection, or we can close it using the close() method.
The code snippet for creating a MongoDB database in NodeJS is:
MongoClient.connect(URI + "/databaseName", function(err, db) {
if (err) throw err;
console.log("Database created!");
db.close();
});
where
- databaseName is the name of the database you want to create,
- URI is the connection string,
- MongoClient is MongoDB Client used to connect to it
Example of Connecting Node.js with MongoDB
Let’s see an example to cover the complete process from installing the MongoDB module to creating a database.
Project Structure: We need a folder that contains a file “app.js”, where we will write our code.
Locate the project folder in a terminal and execute the below command to initiate NPM to install the MongoDB module.
npm init -y
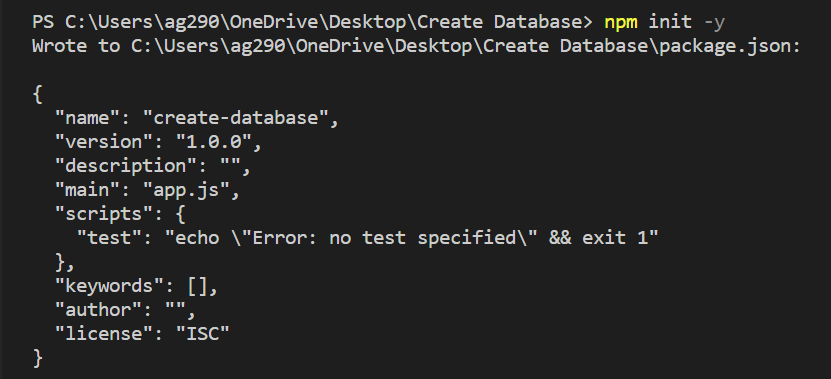
Install the MongoDB module.
npm i mongodb
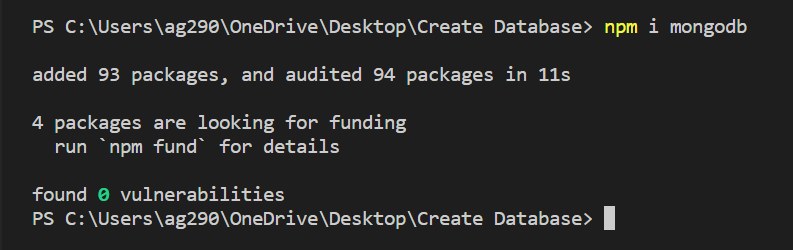
Inside the “app.js” file and import the MongoDB Client.
const MongoClient = require('mongodb').MongoClient;
Use the code snippet we explain earlier and pass the database name as “newdatabase” and URI for the local MongoDB server.
const MongoClient = require('mongodb').MongoClient;
MongoClient.connect("mongodb://localhost:27017/newdatabase", function(err, db) {
if (err) throw err;
console.log("Database created!");
db.close();
});
Run the application:
Type the below command in the terminal and press enter to execute the code.
node aap.js
If you get any error make sure that you have run the MongoDB server locally on your system. We have covered this in another tutorial Comprehensive Guide to Install and Set up MongoDB if you want to read it.
Output:

Verifying the Creation of a MongoDB Database
A database can’t be created whenever you try to create it without any data. To see the result you have to create a collection in that database and then insert some data into that collection.
After inserting the data, you can verify that the database is successfully created by using MongoDB Compass which provides a graphical way to interact with the MongoDB database.
Open the MongoDB Compass and enter the MongoDB URI and press enter.
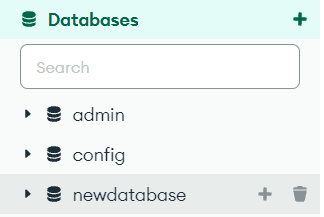
Here you can see that the database of name “newdatabse” has been created.
Summary of Creating a MongoDB Database in Node.js
A database is used to store different kinds of information in order to use it again. We can choose a SQL or NoSQL database. A SQL database store data in the form of one or more tables whereas a NoSQL database does not have any fixed structure, it can store large amounts of unorganized data and MongoDB is one of them. MongoDB does not have any special method for creating the database.
A database is created during the time of NodeJS and MongoDB connection. It passes at the end of the connection URI. Hope this tutorial helps you to understand the process of creating a MongoDB database in NodeJS.
Reference
https://stackoverflow.com/questions/35758008/create-database-node-js-with-mongodb