JavaScript is mainly used on the web. It is used more than any other programming language, approximately 97.7% of websites use it to make the page content interactive. If you are aiming to become a web developer, JavaScript is something that you just can’t avoid. In this guide about JavaScript, you will learn what it is, why people like it, and where it can be applied in the software industry.
What is JavaScript?
JavaScript is the standard and most popular language for making websites interactive. It is used abundantly because it is cross-platform, lightweight, can create animations and enables the dynamic creation of content. JavaScript is capable of being used on the client side utilizing the browser as well as can be used on the server side with Node.js.
While HTML gives structure to a webpage and CSS styles it, JavaScript makes the page interactive. Think about drop-down menus, animated carousels, or real-time content updates—all of these are powered by JavaScript.
Here is a small example of JavaScript in action:
<!DOCTYPE html>
<html lang="en">
<head>
<title>JavaScript Example</title>
</head>
<body>
<h1>Welcome to My Website</h1>
<button onclick="alert('Hello, World!')">Click Me</button>
</body>
</html>
In this example, when you click the button, it shows a message saying “Hello, World!” This shows how JavaScript can make a webpage interactive
Why JavaScript is so popular?
Here are a few reasons why it’s so popular:
- It is multi-platform in nature: It is compatible with almost any device, from personal computers to smartphones.
- It is both a front-end and back-end language: JavaScript has a very interesting property, it can be both client-side and server-side (Node.js).
- No need for complex setups: JavaScript does not need specific settings in which to run as it is a web language. It can operate directly in web browsers.
- Large network of users and regular upgrades: Being an open standard, it is also periodically revised to remain relevant and effective.
- Extensive libraries and frameworks: JavaScript has libraries like React, Angular, and Vue, which simplify complex web app development.
What is JavaScript used for?
1. Web Development
JavaScript is the backbone of modern web development. It improves usability by allowing developers to build content-rich web applications by refreshing the page instead of reloading. JavaScript can also respond to events such as clicks, mouse movements, etc.
document.getElementById("myButton").addEventListener("click", function() {
alert("Button clicked!");
});
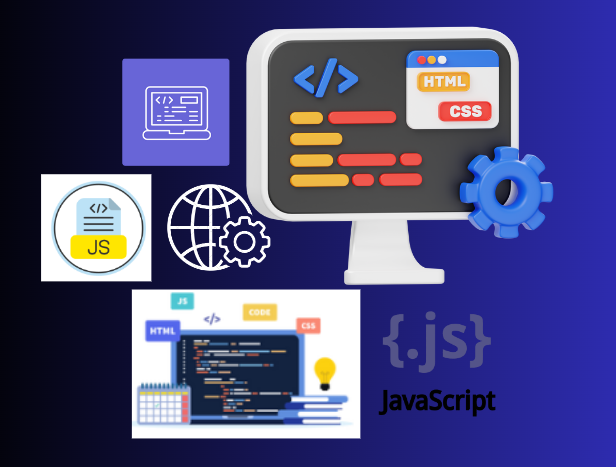
JavaScript improves the quality of the user interaction with the site by allowing the change of certain elements without the need to refresh the screen. This process is called ‘AJAX’ (Asynchronous JavaScript and XML), which enables developers to freely get the information from the server without refreshing the page and update only the necessary section of the page. Here’s a basic AJAX example with JavaScript:
<!DOCTYPE html>
<html lang="en">
<head>
<title>AJAX Example</title>
</head>
<body>
<h1>Welcome to My Website</h1>
<button onclick="alert('Hello, World!')">Click Me</button>
</body>
<script>
const xhr = new XMLHttpRequest();
xhr.open("GET", "https://pokeapi.co/api/v2/pokemon/");
xhr.onload = function() {
if (xhr.status === 200) {
const data = JSON.parse(xhr.responseText);
console.log(data);
}
};
xhr.send();
</script>
</html>
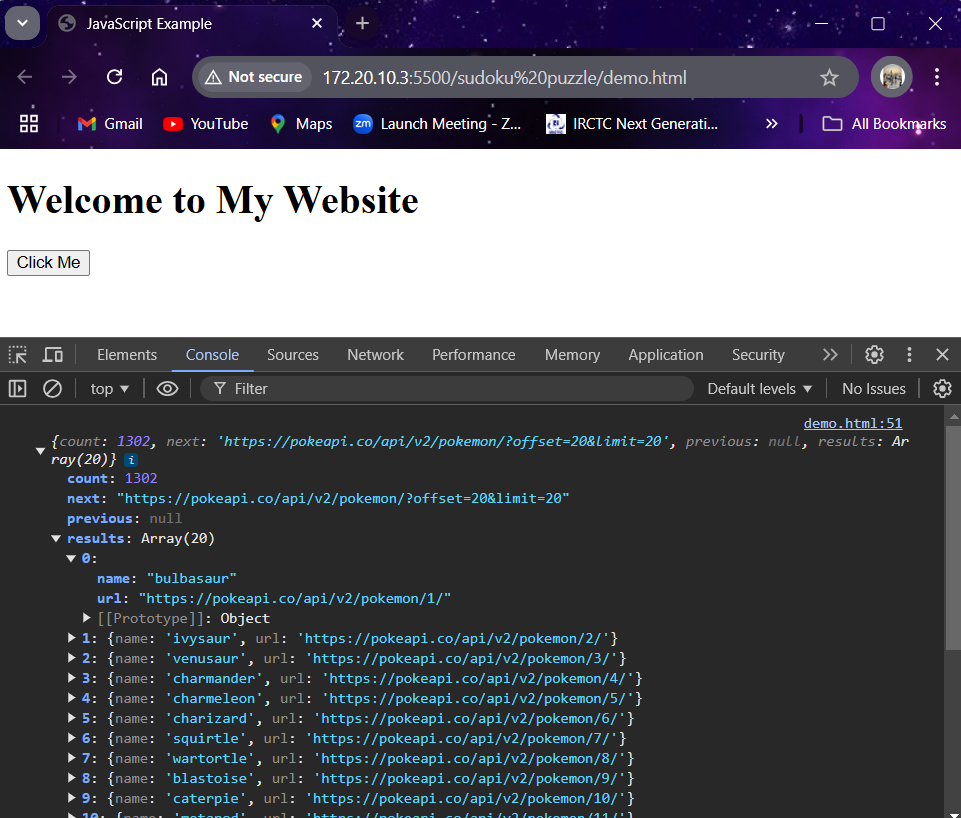
2. Web Applications
Web-based applications such as Google Maps, the user can zoom in, move the map around or load new data using JavaScript without requiring a new page to be loaded. Platforms such as React or Angular can be used for creating very reactive single-page applications (SPA) ensuring a smooth interaction of the users without latency.
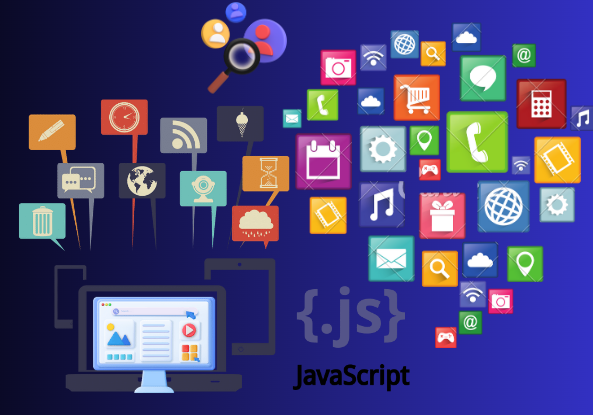
JavaScript drives single-page applications (SPAs) such as Gmail or Facebook, which update the page without reloading the entire page. Frameworks like React and Angular guarantee that components refresh without lag. For instance, React uses a “Virtual DOM” to only update specific parts of the page, improving performance and user experience:
import React, { useState } from 'react';
function Counter() {
const [count, setCount] = useState(0);
return (
<div>
<p>You clicked {count} times</p>
<button onClick={() => setCount(count + 1)}>
Click me
</button>
</div>
);
}
The click count increases without any page reload:
3. Mobile Applications
JavaScript is not about web browsers only. Through frameworks such as React Native, developers are able to build applications for both Android and iOS, from a single code base.
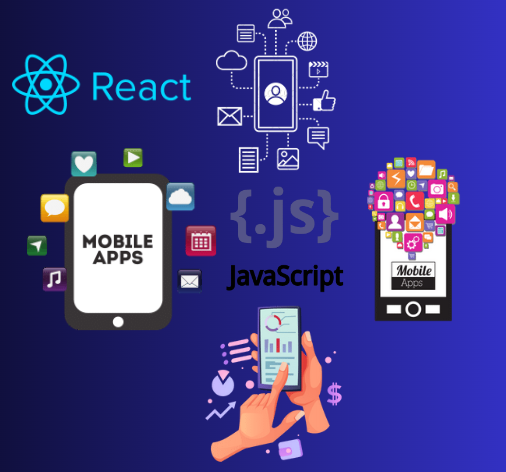
For instance, React Native converts JavaScript components into native UI components, making applications feel native on each platform:
import React from 'react';
import { Text, View, Button } from 'react-native';
const App = () => {
return (
<View>
<Text>Hello, Mobile World!</Text>
<Button title="Press me" onPress={() => alert('Button pressed!')} />
</View>
);
};
export default App;
4. Server-Side Applications
JavaScript, thanks to Node.js, has moved from the client side to the server side and continues to grow. This makes it easy for developers to create high-speed applications for computer networks. Some of the big giants such as Walmart, PayPal, and LinkedIn use Node.js for handling server requests proficiently.
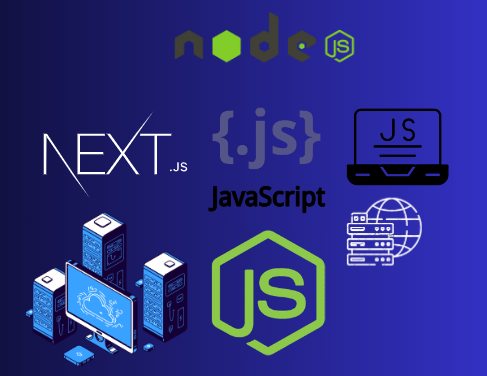
const http = require('http');
http.createServer((req, res) => {
res.writeHead(200, {'Content-Type': 'text/plain'});
res.end('Hello from the server!');
}).listen(3000);
This is a Node.js code that builds a web server that will give out “Hello from the server!” when accessed.
5. Game Development
JavaScript also enables a lot of web-based games, and here uses the HTML5 canvas tag for the rendering of 2D/3D graphics. There are so many libraries nowadays that make game development easy, including Phaser.js which gives developers an opportunity to design complex browser-based games with full interactivity.
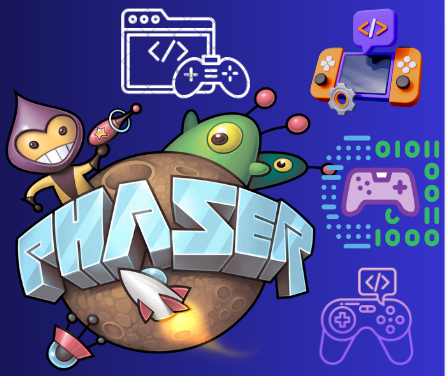
The Canvas API allows for direct manipulation of pixels, essential for rendering graphics in games:
<!DOCTYPE html>
<html lang="en">
<head>
<title>JavaScript Example</title>
</head>
<body>
<h1>Welcome to My Website</h1>
<button onclick="alert('Hello, World!')">Click Me</button>
<canvas id="gameCanvas" width="200" height="200"></canvas>
<script>
const canvas = document.getElementById('gameCanvas');
const ctx = canvas.getContext('2d');
ctx.fillStyle = 'blue';
ctx.fillRect(20, 20, 50, 50);
</script>
</body>
</html>
This draws a blue square:
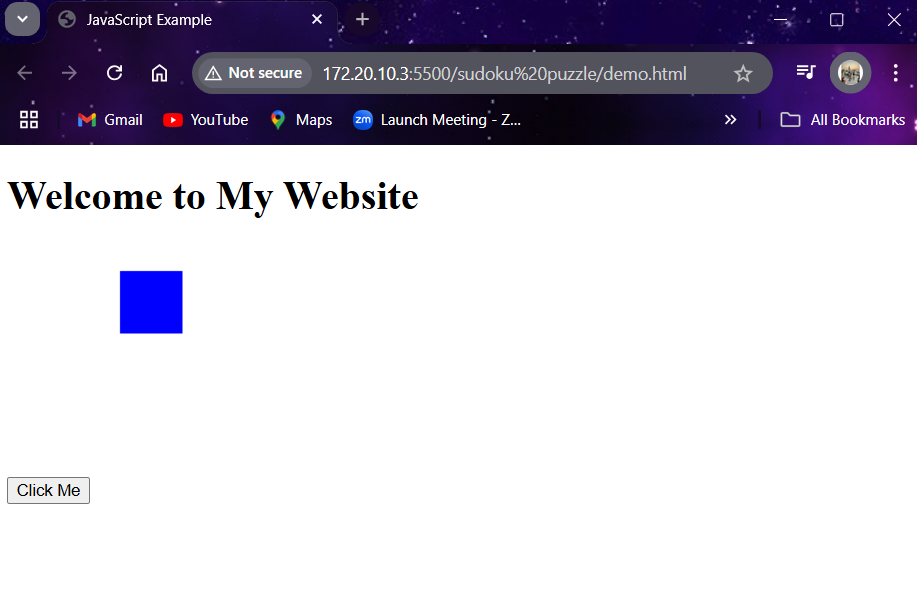
6. Presentations
JavaScript allows you to create especially business presentations as websites. Reveal is a library that you can use to design your slides with transitions and themes and even set custom animations to make your slides for web-based slideshow.
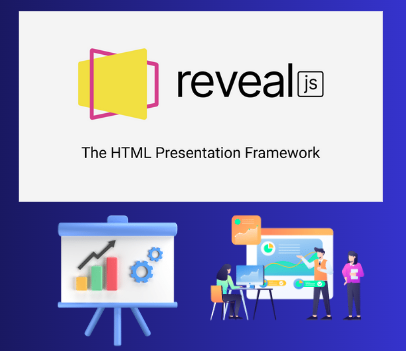
7. IoT and Robotics
JavaScript also expands to the world of hardware to allow developers to interact with and manage IoT devices and small robots. With such frameworks as Johnny-Five, JavaScript can be used to write programs into devices such as drones and smart sensors.
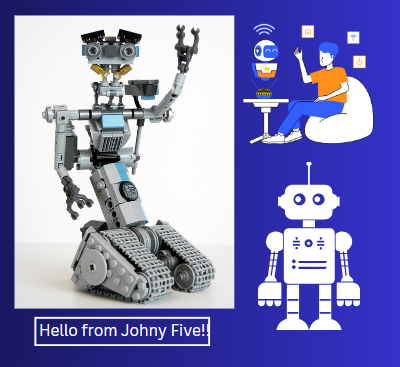
Conclusion
JavaScript is quite a universal and necessary language for almost everyone who wants to create a lively website, mobile applications and server-side programs. Whether you are a web developer, a mobile developer or even a game developer there are a number of tools and frameworks available in JavaScript. Because of its frequent release updates and extensive use, learning JavaScript is the right direction to consider when looking for a career in tech.
Start your web development journey by learning JavaScript and becoming part of a vast and distinguished community of web developers. Here are some Javascript articles to start with:
- What is a Class In JavaScript?
- How to Read a Local Text File Using JavaScript
- What does javascript:void(0) mean?
- What Is JavaScript Double Question Mark (??)
Reference
https://stackoverflow.com/questions/3497115/what-is-the-use-of-javascript