Node.js is well known for its use in web development, providing the runtime environment for JavaScript to create servers easily. If you are new to Node.js, I suggest you read What is Node.js? first. But the question is, what makes it so special? Why is it important to involve Node.js in web development? What is Node.js used for?
Top 10 Use Cases and Benefits of Node.js
1. Efficient Multi-Tasking with a Single-Threaded Event Loop
When we need to handle multiple tasks, Node.js facilitates us by using the single-threaded event loop mechanism. This is the one feature that makes Node.js stand out from the crowd. Let me explain what this mechanism is.
The name itself suggests that it uses a single thread to manage processes, unlike languages like Java, which use multiple threads. This proves effective as it uses fewer resources and enhances speed. This single thread runs an event loop, which continuously checks for tasks to be executed.
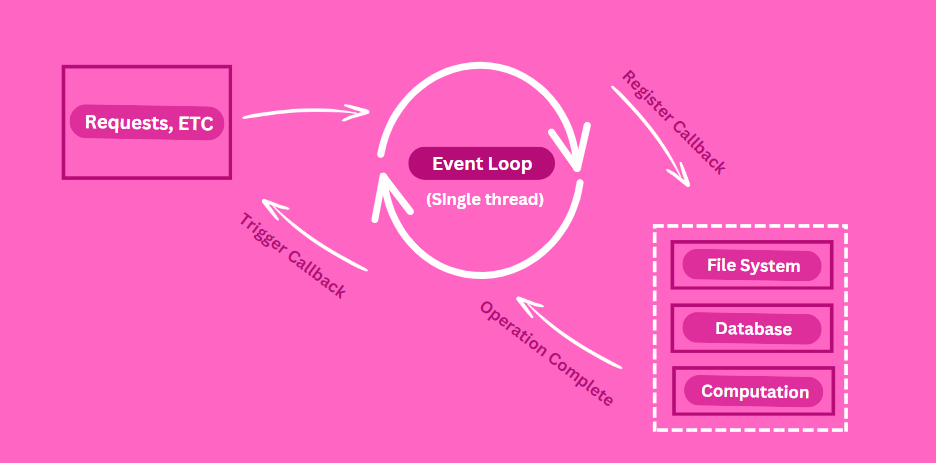
When a task like fetching data from a database or reading a file is requested, Node.js starts the task and moves on to the next one without waiting for the first task to finish. This saves time and makes things work faster. When the initial task is completed, an event is triggered. The event loop picks up this event and processes the result. This mechanism enables Node.js to handle multiple tasks in a single thread without blocking.
Here’s a simple example that shows efficient multitasking with a single-threaded event loop:
const fs = require('fs');
fs.readFile('Read1.txt', 'utf8', (err, data) => {
if (err) {
console.error(err);
return;
}
console.log('File 1 content:', data);
});
fs.readFile('Read2.txt', 'utf8', (err, data) => {
if (err) {
console.error(err);
return;
}
console.log('File 2 content:', data);
});
console.log('Started reading files without blocking');

2. Advantages of Relationship with JavaScript
We all agree with the fact that JavaScript is one of the most popular programming languages used to build websites. This is because it is easy and beginner-friendly, and a huge community of developers also uses it.
For its first 14 years, JavaScript was mainly used to make web pages interactive. Developers used it inside the <script> tag in HTML. Later, Node.js was created, allowing JavaScript to be used for server-side programming too. It uses the V8 JavaScript engine, which helps in building networking applications.
Node.js is written in C, C++, and JavaScript. It combines Google’s V8 engine, the libuv platform layer, and a core library primarily in JavaScript. And if we put all things together in an easy way, Node.js is not a programming language or a framework but it’s a runtime environment that runs JavaScript outside of a web browser.
So yes, it is very clear that JavaScript and Node.js are best friends and nicely compatible. This duo plays a major role in the market for web development. We can also say that one of the reasons Node.js is so popular is because JavaScript is famous. People working with JavaScript tend to move towards Node.js, and this provides it with a large community of developers. This is good for Node.js, as learners will find an ample amount of open resources and doubt clearance on the web. Due to its extensive use, there are always new updates and modifications made to this technology, which is good for its future and even beneficial for users.
3. Easy to Learn and Start With
JavaScript is a language familiar to every developer nowadays. Node.js uses it for server-side environments, making it easy for beginners to get started. There are many open sources available for learning and development, including its official documentation, which provides explanations for every problem. Also, our site offers Node.js articles to help simplify your learning experience.
We have got you a simple ‘Hello world!’ code in Node. js to prove our point:
const http = require('http');
const server = http.createServer((req, res) => {
res.end('Hello, World!\n');
});
server.listen(3000, () => {
console.log('Server running at http://localhost:3000/');
});
With just these few lines of code, we have created a server that listens on port 3000 and displays ‘Hello world!’.

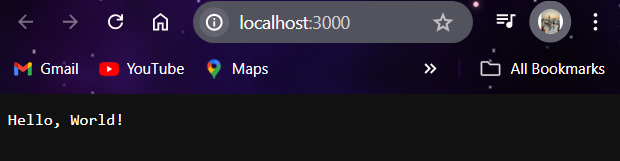
For more Click here!
4. Load Balancing
Node.js can handle a lot of requests at once because it uses cluster modules to balance the load. This means it can manage many users using an application at the same time without slowing down or crashing. It’s great for making sure your app stays fast and reliable even when lots of people are using it.
You can study how load balancing is achieved in Node.js by clicking here.
5. Fast Due to Non-Blocking Execution
A “Single Threaded Event Loop” architecture is used to handle multiple client requests, unlike Java, which uses multiple threads to do so. And if a high amount of processing is required, it assigns it to a small pool of worker threads. This is how Node.js uses fewer resources and performs faster. One more reason that it is fast is that it uses a V8 engine
Here is an example to show the non-blocking execution nature of Node.js:
console.log("Start");
setTimeout(() => {
console.log("Node.js is non-blocking");
}, 2000);
console.log("End");
When you run this code, you will notice that “Start” and “End” get printed first. After 2 seconds, “Node.js is non-blocking” is printed. This shows how Node.js can keep running other tasks while it waits for things like setTimeout to finish.

6. Rich Library of Available Packages
There are over 50,000 packages available in Node Package Manager, which allows developers to experiment with their complex ideas using fewer lines of code and provides them with ease. For example, you can easily include things like user authentication, database management, or connecting to APIs using ready-made packages. This saves time and ensures your applications are reliable, thanks to the community-supported packages.
Popular packages include Express for web development, Mongoose for working with MongoDB databases, and Axios for handling HTTP requests, making Node.js a versatile choice for building powerful applications.
Click here to see some of the famous Node.js APIs.
7. Asynchronous Nature
Node.js is great because it doesn’t waste time waiting for responses from APIs. Instead, it uses events to keep things moving smoothly. This means your applications stay responsive and can handle many tasks at once without getting stuck. This is great for apps needing real-time updates, like chats or games, and it helps manage resources efficiently, scaling easily as needed
8. Cross-Platform Support
This feature helps a lot, as the project made using Node.js will be compatible with more or less every platform as it works on Windows, UNIX, Linux, and MacOS. This saves time and money because you don’t need different versions for each platform. It helps developers reach more people with their apps, no matter what device they use. This makes Node.js great for building apps that work smoothly across different types of computers without extra hassle.
9. Allows Developers to Use JavaScript for Both the Frontend and Backend
This is one of the most important things Node.js offers us. This point is a huge topic in itself, to understand it best, click here.
10. Perfect Data Streaming
Node.js applications don’t buffer data as they process and upload files quickly. It is great for streaming data because it handles it without delays, making it perfect for real-time tasks like video streaming and uploading files. It doesn’t wait to receive all the data before working with it, so users get faster uploads and smoother experiences. This is helpful for apps like chats, streaming videos, and smart devices, where quick data processing is important. Node.js’s ability to handle streaming nicely makes it a top pick for developers who want to build fast and reliable applications.
Conclusion
Node.js is popular and used by companies like Twitter, Spotify, eBay, Reddit, LinkedIn, and GoDaddy. And if popular companies like these are using Node.js, it is pretty much clear that every good developer prefers to use it due to its non-blocking, event-driven, and asynchronous nature. Besides, it is fast, and it gets along well with JavaScript.
Reference
https://stackoverflow.blog/node-js