While surfing the web, you may come across websites that redirect you to a certain page and throw up a flash message for various reasons, such as errors, warnings or successful operation. Well, we can also implement this functionality on our Node.js application using a simple module ‘connect-flash’.
For example, let’s say we are building a Node.js application for a bank, and when a user tries to make a transaction for which the amount present is not sufficient, then we can use the ‘connect-flash’ module to pop up a message ‘insufficient balance’. This can enhance the overall user experience. You may see this type of pop-up message mainly in older mobile apps.
This is just a basic explanation, using ‘connect-flash’ is not that easy, we have to apply multiple technical approaches to send flash messages using it. Let’s start the tutorial.
Node.js connect-flash Module
Express 2.x has a middleware called ‘flash’ that is used to store messages that pop up on the page that is being rendered. The stored message is removed after being shown. When Express 3.x comes, this middleware is removed. So now if anyone wants to use it, it can be done using ‘connect-flash’.
The ‘connect-flash’ module allows developers to display or render pop messages every time a user is redirected to a certain page. The ‘connect-flash’ module is not a built-in module so we have to install it manually.
Installation Syntax:
npm i connect-flash
Let’s get started with a mini-application to help you get a better understanding.
Using connect-flash to Display Flash Messages in Node.js
To get going, we will first create a Node.js project and then install and import the required packages in it.
1. Create a new folder and initiate the Node app in it:
npm init -y
Your package.json should now be created and look like this:
{
"name": "7_FLASH_NODEJS_APP",
"version": "1.0.0",
"description": "",
"main": "index.js",
"scripts": {
"test": "echo \"Error: no test specified\" && exit 1"
},
"keywords": [],
"author": "",
"license": "ISC"
}
2. Create the .js file:
touch app.js
3. Install all the required packages:
npm i express connect-flash express-session ejs
Here we will be using express as the server framework and the express-session module to create a session each time a user is redirected to a certain page and a message is flashed.
We are also using ejs as our HTML templating engine so we can serve better-looking web pages of our application. If you do not understand what ejs is, what it does, and how it works, click here to read this post on using EJS Template Engine in Node.js. If you have prior knowledge about ejs, you’re good to go!
4. Set up app.js file and import all the packages that we just installed:
const express = require('express');
const session = require('express-session');
const flash = require('connect-flash');
const path = require('path');
const app = express();
app.set('views', path.join(__dirname, 'views'));
app.set('view engine', 'ejs');
const port = 8000;
app.use(session({
secret: 'codeforgeek',
saveUninitialized: true,
resave: true
}));
app.use(flash());
app.listen(port, (err) => {
console.log(`App is running on port ${port}`);
});
In the above code, the below lines make sure that the ‘views’ folder is accessible globally:
const path = require('path');
app.set('views', path.join(__dirname, 'views'));
If you don’t get what the path module does, click here to read about it!
5. Using ‘connect-flash’ Module:
Now that we have set the basics of our application, let us move on to implementing ‘connect-flash’ and creating routes for our users.
app.get('/', (req, res) => {
req.flash('success', `You've been successfully redirected to the Message route!`)
res.redirect('/message')
})
app.get('/message', (req, res) => {
res.send(req.flash('success'))
})
In the above code snippet, we have created a home route that redirects to the message route when requested. We have also assigned a success flash message on the home route which will be displayed on the message route on successful redirection.
The req.flash() in the home route, accepts two string values as arguments. The first string defines the type of flash message you want to render, and the second string accepts the actual flash message. Although defining a type for your flash messages doesn’t change the way they look, it is good for reference for you or other developers reading your code later.
However, you could pass many types of flash messages you like but then the redirected page trying to render your flash message shouldn’t clash with similar types. This is why you should keep each of the type names unique.
The message route is where the flash message of your choice is called. We defined a success type flash message and are calling that same flash message on the message route by simply passing req.flash(‘success’).
Now, whenever a user is redirected to the message route, he/she will receive a flash message saying “You’ve been successfully redirected to the Message route!”.
Complete Code:
const express = require('express');
const session = require('express-session');
const flash = require('connect-flash');
const path = require('path');
const app = express();
app.set('views', path.join(__dirname, 'views'));
app.set('view engine', 'ejs');
const port = 8000;
app.use(session({
secret: 'codeforgeek',
saveUninitialized: true,
resave: true
}));
app.use(flash());
app.get('/', (req, res) => {
req.flash('success', `You've been successfully redirected to the Message route!`)
res.redirect('/message')
})
app.get('/message', (req, res) => {
res.send(req.flash('success'))
})
app.listen(port, (err) => {
console.log(`App is running on port ${port}`);
});
Run the Application:
The application code is written in a single file “app.js” that runs on executing the below command in the terminal.
node app.js
Open the Application:
Enter the below URL inside the search bar of a web browser to open the application.
http://localhost:8000/
Output:
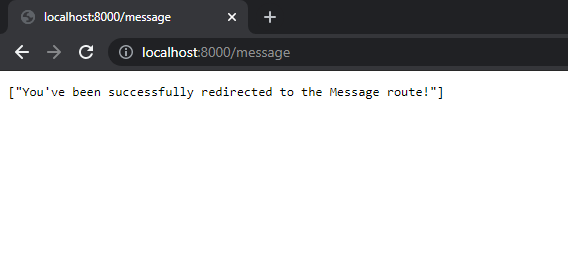
This way we can successfully render any flash messages in Node.js.
Frequently Asked Questions (FAQs)
What is a flash message in Node.js?
Flash message in Node.js is a special type of message sent to the user to give notifications about the success or failure of any operation.
What is the purpose of a flash message?
Flash messages are used to notify the user for various reasons, such as when an operation fails, whenever an error occurs, or to pop up some type of information.
What is an example of a flash message?
An example of a flash message could be when a user book a ticket online and for some reason the payment is not made successfully, the booking website sends a flash message to the user, showing that the transaction is incomplete.
What is the difference between a flash message and a normal message?
A normal message can be used for most things to show a variety of information to the users, while a flash message is used on special occasions to inform that something special has happened.
How to set flash messages in Node.js?
The best way to set up flash messages in Node.js is to use middleware like connect-flash or express-flash.
Where are flash messages stored?
Flash messages are stored in session variables that can be accessed using the request object.
Conclusion
In this article, we learned how to display flash messages using the third-party Node.js dependency connect-flash. Using the connect-flash module in Node.js we can easily set up a message and show it when the user is redirected to another page. Just make sure you have installed connect-flash manually in the project directory before using it otherwise you will end up getting an error.
Reference
https://www.npmjs.com/package/connect-flash