In this complete guide, we will delve into the world of OpenCV and its effective image-loading function, imread. OpenCV (Open Source Computer Vision Library) is an exceptionally acclaimed open-source computer vision and machine learning software program library, significantly utilized in numerous industries, together with robotics, automatic vehicles, and image processing. The cv2.imread function is a crucial tool within the OpenCV library, permitting us to load images, that’s a essential step in several computer vision programs.
Understanding OpenCV and Its Importance
OpenCV plays a vital function in the region of computer vision and image processing. It is a very versatile and reliable library and is frequently preferred by many developers and researchers for many assignments. With OpenCV, we are able to carry out an array of tasks, such as image and video assessment, item detection, facial recognition, and extra.
The imread function is a cornerstone of any image processing project. It enables us to read picture documents from our local storage or web servers, allowing us to work with real-world visual data. By learning this function, we open up a world of possibilities in the field of computer vision.
Loading an Image with cv2.imread() Method
Before diving into the technical details of the cv2.imread function, let’s first understand how to use it to load an image. Suppose we have an image stored in our local directories named CodeForGeeks.png and we want to load this image using imread function.
We have to first install the opencv-python package in our Python environment.
Execute the following command in the terminal:
pip install opencv-python
This command will install the package opencv-python with all its functions including imread.
Now we will look in the code for loading the CodeForGeeks.png image using opencv imread function:
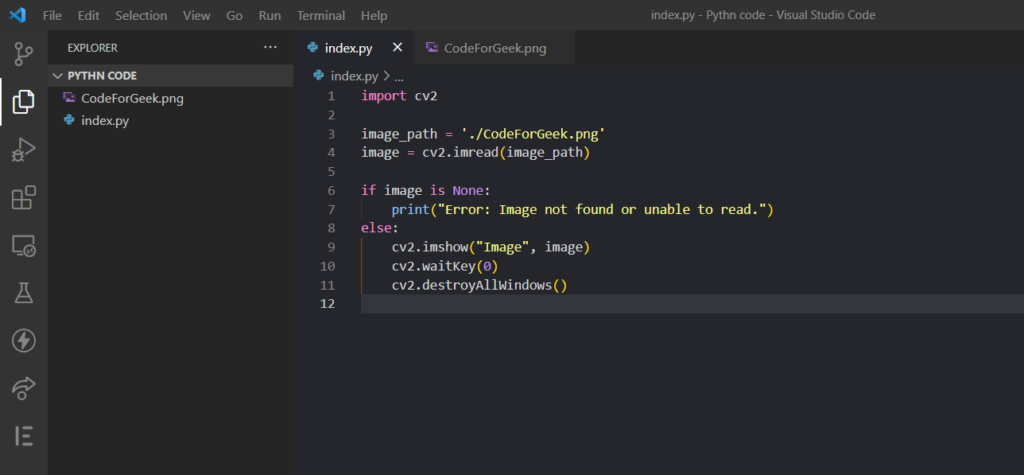
After running the above code imread function will look for CodeForGeek.png file in our local directory and creates a window named “Image” and displays the image in it.
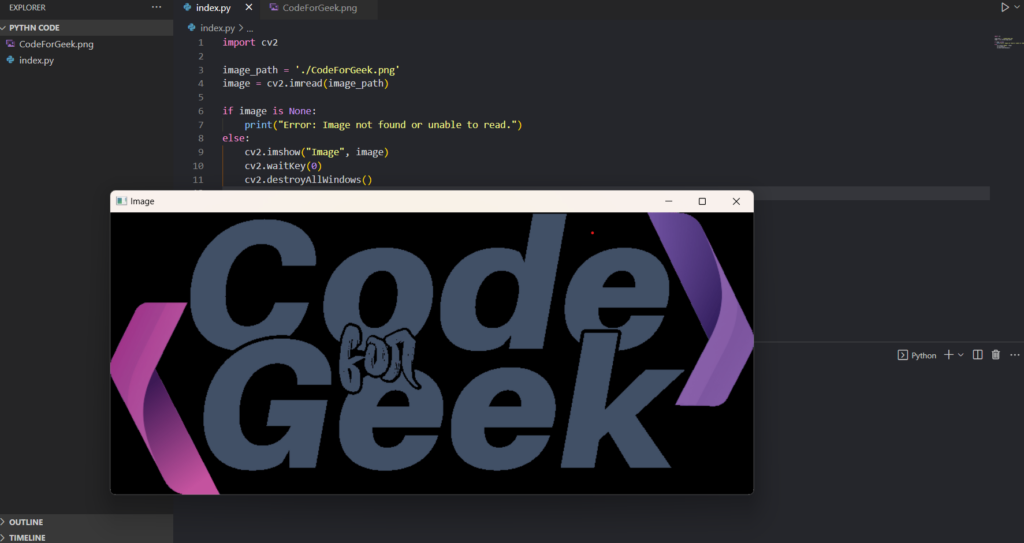
Parameters of cv2.imread() Method
The imread function in OpenCV is used to read an image from a file and load it into memory as a NumPy array. It has the following parameters:
- filename: This parameter specifies the file path of the image to be studied. It ought to be a string containing the path to the document on the file system. This may be an absolute or relative path.
- dst (elective): The dst parameter is every other non-compulsory argument that permits you to offer a pre-allotted NumPy array in which the image statistics will be stored. This can be useful if you need to reuse a current array to save memory or perform particular operations. If no longer provided, the feature will create a new NumPy array to keep the image records.
- Flags: The flags parameter is an optional argument that controls how the image is read. It specifies the way the image should be dealt with in phrases of colour channels and image properties. It can take several special values or an aggregate of them, which can be represented as integer constants defined in OpenCV.
Some commonly used flags consist of:
- cv2.IMREAD_COLOR (or simply 1): This is the default flag used if no flags parameter is distinct. It masses the image in the BGR colour format (Blue-Green-Red) with 3 colour channels.
- cv2.IMREAD_GRAYSCALE (or simply 0): This flag hundreds the picture in grayscale mode, which means that it’ll have the most effective one-colour channel representing the intensity of the pixels.
- cv2.IMREAD_UNCHANGED (or simply -1): This flag loads the image as it’s far, along with the alpha channel (if present). It preserves all of the channels and no longer performs any colour or channel conversions.
The imread function returns a NumPy array representing the image if it’s far more efficiently read. If there’s an error in reading the image (e.g. file not found or unsupported), it returns None.
Best Practices for Image Loading with OpenCV
To maximize the efficiency and reliability of image loading using cv2.imread, consider the following best practices:
1. Use Absolute File Paths
Using absolute file paths in your code ensures that the images can be consistently positioned throughout unique environments, for that reason enhancing the reproducibility of your tasks.
Relative paths, then again, are depending on the contemporary working directory, which can vary among distinctive systems or execution contexts. This can cause discrepancies, as the code may also fail to find the image files in certain conditions.
By the use of absolute paths, you provide an express and fixed location for the files, eliminating the reliance on the current working directory. This is especially crucial whilst sharing code with others or walking it on unique machines
2. Check for Image Load Success
After loading the image using cv2.imread(), check if the returned image is None. This indicates that there was an issue with reading the image, such as a file not found or an unsupported format. Handling this gracefully will prevent unexpected errors in your code.
image = cv2.imread(image_path)
if image is None:
print("Error: Image not found or unable to read.")
else:
# Continue with further processing
3. Leverage Grayscale Mode
If colour information is not essential for your computer vision task, consider loading the image in grayscale mode (cv2.IMREAD_GRAYSCALE). Grayscale images occupy less memory and require less processing power, making them ideal for certain applications.
image = cv2.imread(image_path, cv2.IMREAD_GRAYSCALE)
4. Maintain Image Aspect Ratio
Preserving the aspect ratio when displaying or processing images in OpenCV is crucial to avoid distortion and ensure accurate representation. When resizing, maintain the aspect ratio by specifying either the width or height while keeping the other dimension proportional. For cropping, select regions that preserve the original aspect ratio to avoid loss of important information.
Conclusion
In conclusion, understanding how to load images using OpenCV’s cv2.imread function is a fundamental skill for any computer vision enthusiast or professional. The versatility and power of OpenCV make it an indispensable tool in the field of image processing and analysis. By following best practices and optimizing for the image-loading process, we can enhance the performance and reliability of our computer vision applications.
Also Read:
Reference
https://stackoverflow.com/questions/46540831/how-to-read-an-image-in-python-opencv