What if you do not fancy the for loop, but would like to run iterations on an input? Is there a way around doing that in Python? Seems there is! Enter the map( ) function. This function effectively loops over all the items listed in the input and transforms them into the desired results. This article sets out to explore the nuances of the map( ) function in Python alongside throwing a handful of demonstrations of its use cases.
Python map( ) function
As the name indicates, this function maps every item given in the input for passing through the given function so as to return the desired results.
Syntax
Below is the syntax of the map( ) function detailing its basic constructs:
map(function, iterable 1, iterable 2,….., iterable n)
where,
- function – the function for transforming the given iterable into the entities of the desired result
- iterable – the item or a list of items that are to be transformed to deduce the desired result
Following are the different methods through which the map( ) function can be deployed in Python.
- Using the map( ) function over strings
- Using the map( ) function over numbers
Using the map( ) function over strings
Let us consider the following set of strings as the input that is to be transformed through the map( ) function.
ip = ['Madras', 'Kolkata', 'Madrid', 'Cairo']
Now we shall try to convert the aforementioned strings into the upper case using the map( ) function.
op = map(str.upper, ip)
Once the above code is run, it is time to view the results using the print( ) function.
print(op)

One might be in for a surprise when trying to view the results through the print( ) function. This is because printing the output using the conventional technique returns the address at which the mapping takes place rather than returning the actual results. So, how to get over this you ask?
Enter the list( ) function!
When this function is nested within the print( ) function then the results are returned as expected.
print(list(op))
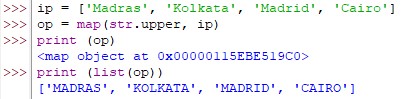
This is just a glimpse of what could be done to a set of strings using the map( ) function. One can very well extend its capabilities far beyond applications such as removing blank spaces or dots or punctuation marks by passing the iterable through the strip( ) function declared within the map( ) function.
Using the map( ) function over numbers
Rather than using the built-in functions in Python, one can also create customised functions and use them within the map( ) function as given below for finding half of the 10th power of the given iterable. We shall get started using the def function.
def p10(x):
return (x**10)/2
ip = [10, 1, 9, 2]
Now let us run them through the map( ) function.
map(p10, ip)
list(map(p10, ip))
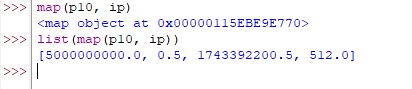
Let us try something different with the numbers this time.
def kelvinator(x):
return x+273.15
The above function is to convert the values in degrees Celsius to Kelvin. So, let us run it through an input using the map( ) function as shown below.
temp = [20, 250, -60]
list(map(kelvinator, temp))
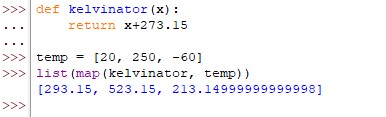
One can also deploy other transformational functions such as factorial( ), and sqrt( ) or create customised functions for the conversion of units such as metres to millimetres or litres to gallons and the likes within the map( ) function.
Conclusion
Now that we have reached the end of this article, hope it has elaborated on the different ways of using the map() function in Python. Here’s another article that can be our definitive guide to the fpdf module in Python. There are numerous other enjoyable and equally informative articles in CodeforGeek that might be of great help for you to gain valuable insights. Until then, ciao!