NumPy is one of the most commonly used Python libraries when it comes to performing mathematical operations on arrays. This library provides various functions that can make performing these operations on arrays or matrices easier and more efficient. In this article, we’ll understand the numpy.square() function provided by NumPy which helps in calculating squares in Python. We will also look at some examples to understand the implementation of this function.
Also Read: Numpy Broadcasting (With Examples)
Introducing numpy.square() Function
numpy.square() is a mathematical function provided by the NumPy library which takes in an array of integers or decimal values and returns an array containing the squares of each of the elements in the input array. Let’s look at the syntax of this function and understand its parameters.
Syntax:
numpy.square(arr, /, out=None, *, where=True, casting='same_kind', order='K', dtype=None)
- arr: Input array containing the elements to be squared.
- out: Name of the output array. If set to None or not given a value, a new array will be created to store the resultant array containing squares.
- where: A boolean array used to specify which elements from the input array should be squared. This parameter has a default of True, meaning all elements from the input array must be squared.
- casting: Used to specify whether data type casting is allowed in the resultant array. Here, same_kind indicates that casting can be done between the same kind of data types (eg: casting can be done between float and integers as they are both numeric data types).
- order: Used to specify the memory layout of the output array. Here, K indicates that the original order of the input array should be preserved when laying out the memory for the output array.
- dtype: Used to specify the data type of the output array. Here, None indicates that the data type of the output array must be inferred according to that of the input array.
Now let’s understand the implementation of this function with some examples.
Calculating Squares of Each Element in NumPy Array
In this section, we’ll understand how to use numpy.square() by looking at some examples and also see how the output array varies when certain parameters are changed while using this function. We will also explore how the application of numpy.square() can be extended to 2D arrays (matrices) as well.
Calculating Squares of All Elements in an Array
Let’s see a basic example, with no additional parameters specified other than the input array. We take a simple array arr containing some integers. We then use numpy.square() on arr to calculate the squares of each of the elements in the array. The output array is stored in squared_arr.
import numpy as np
arr = [1, -3, 5, -7, 9]
squared_arr = np.square(arr)
print(squared_arr)
Output:
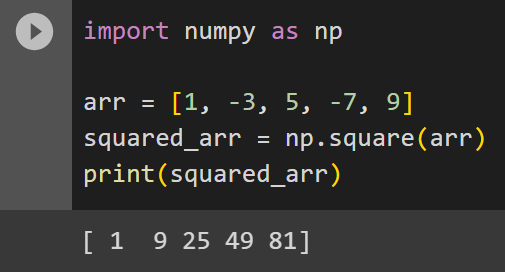
Using dtype to Change the Data Type of Output Array
In this example, we’ll specify the data type of the resultant array using the dtype parameter, while squaring the input array arr from the previous example.
import numpy as np
arr = [1, -3, 5, -7, 9]
squared_arr = np.square(arr, dtype=float)
print(squared_arr)
Even if the input array contains integers, the output array containing squares will have float values.
Output:
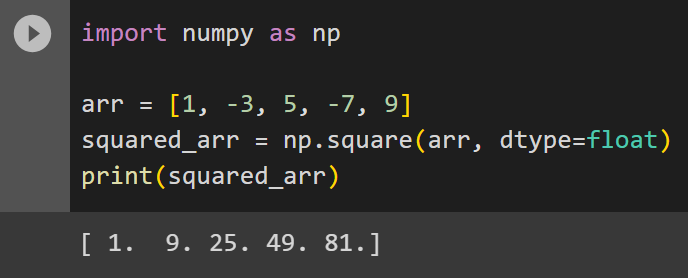
Using the where Parameter to Square Specific Elements
In this example, we’ll see how we can use the where parameter which will help us conditionally square only certain elements in an array. Here, we’ll square only the positive numbers (1,5 and 9).
import numpy as np
arr = np.array([1, -3, 5, -7, 9])
squared_arr = np.square(arr, where=arr > 0)
print(squared_arr)
Output:
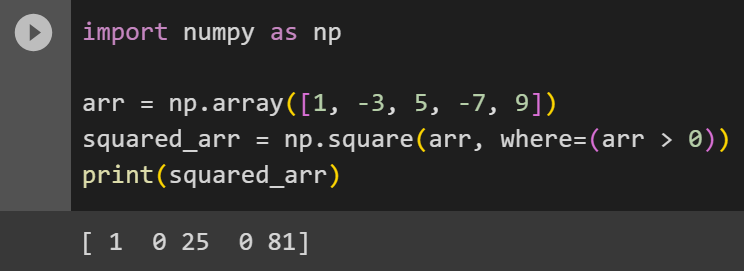
Squaring Elements in a Matrix
In this example, we’ll look at how we can use numpy.square() to square elements in a matrix. This works similarly to how it does in arrays. Here we’ll take a 3×3 matrix and square all its elements.
import numpy as np
matrix = [[1, -3, 5],
[-7, 9, -11]]
squared_matrix = np.square(matrix)
print(squared_matrix)
Output:
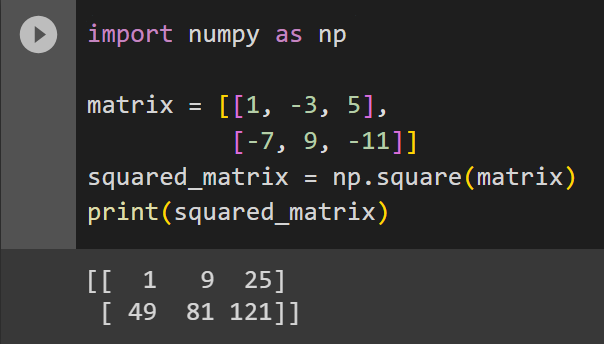
Conclusion
NumPy provides us with various handy functions to perform mathematical computations on arrays. In this article, we have explored the numpy.square() function which provides us with an easy way to square multiple elements in an array. We have understood the working of numpy.square() with some supporting examples and also looked at how we can change the output array according to our preference using various parameters like dtype and where. Whether we need to square elements of an array or matrix, using numpy.square() is the simplest way to go.
Reference
https://stackoverflow.com/questions/34598632/python-numpy-square-values-issue