The strict mode in JavaScript helps us to write secure code by detecting accidental errors and bugs in the code. It produced the visible mistakes hidden in JavaScript, by default JavaScript just failed silently without showing the errors.
In this tutorial, you will learn about the strict mode in JavaSript, the process of activating strict mode for a program or an individual function in JavaScript, and some typical actions that are forbidden by strict mode in JavaScript.
Activating JavaScript Strict mode
For activating Strict mode, you can add ‘use strict’; or “use strict”; at the beginning of the script.
Syntax:
‘use strict’;
// or
"use strict";
This statement should be the first statement in the script excluding comments as JavaScript just ignore them.
If there is any other statement before ‘use strict’; or “use strict”; then string mode will not activate.
Adding ‘use strict’; or “use strict”; at the beginning of the script has a global scope which makes all the code in that script executed in string mode.
Example:
"use strict";
name = "John";
console.log(name);
Output:
name = "John";
^
ReferenceError: name is not defined
at Object.<anonymous> (C:\Users\ag290\OneDrive\Desktop\journalDev-2023\january\strict mode\app.js:2:6)
at Module._compile (internal/modules/cjs/loader.js:759:30)
at Object.Module._extensions..js (internal/modules/cjs/loader.js:770:10)
at Module.load (internal/modules/cjs/loader.js:628:32)
at Function.Module._load (internal/modules/cjs/loader.js:555:12)
at Function.Module.runMain (internal/modules/cjs/loader.js:826:10)
at internal/main/run_main_module.js:17:11
Here, we have got an error as Strict Mode doesn’t allow using an undeclared variable.
Without Strict Mode:
name = "John";
console.log(name);
Output:
John
Activating JavaScript Strict Mode in Individual Functions
You can also enable string mode for a particular function by adding ‘use strict’; or “use strict”; at the beginning of the function.
Syntax:
function functionName() {
‘use strict’;
// function body
}
// or
function functionName() {
"use strict";
// function body
}
This statement should be the first statement of the function excluding comments.
Adding ‘use strict’; or “use strict”; at the beginning of a function has a local scope which means all the code in that script is executed usually and the code inside the function will only execute in strict mode.
Example:
function Name() { // Function without strict mode
name = "Rack"
console.log("Name is: " + name) // No error here
}
function Age() { // Function with strict mode
"use strict";
age = "22"
console.log("Age is: " + age) // Error here
}
Name()
Age()
Output:
Name is: Rack
C:\Users\ag290\OneDrive\Desktop\journalDev-2023\january\strict mode\app.js:8
age = "22"
^
ReferenceError: age is not defined
at Age (C:\Users\ag290\OneDrive\Desktop\journalDev-2023\january\strict mode\app.js:8:9)
at Object.<anonymous> (C:\Users\ag290\OneDrive\Desktop\journalDev-2023\january\strict mode\app.js:13:1)
at Module._compile (internal/modules/cjs/loader.js:759:30)
at Object.Module._extensions..js (internal/modules/cjs/loader.js:770:10)
at Module.load (internal/modules/cjs/loader.js:628:32)
at Function.Module._load (internal/modules/cjs/loader.js:555:12)
at Function.Module.runMain (internal/modules/cjs/loader.js:826:10)
at internal/main/run_main_module.js:17:11
PS C:\Users\ag290\OneDrive\Desktop\journalDev-2023\january\strict mode>
Here, the first function doesn’t cause any error but the second function throws an error, since it executes the code in strict mode, and as we know in strict mode, a variable is not used if it is not declared.
Actions Not Allowed in Strict Mode
Some typical actions that are forbidden in strict mode are given below:
1. Undeclared objects or variables.
We have already seen that using a variable or an object without declaring it is not allowed in strict mode.
Example:
"use strict";
user = {
name: 'John',
age: 30,
gender: 'male',
}
console.log(user.name) // John
Output:
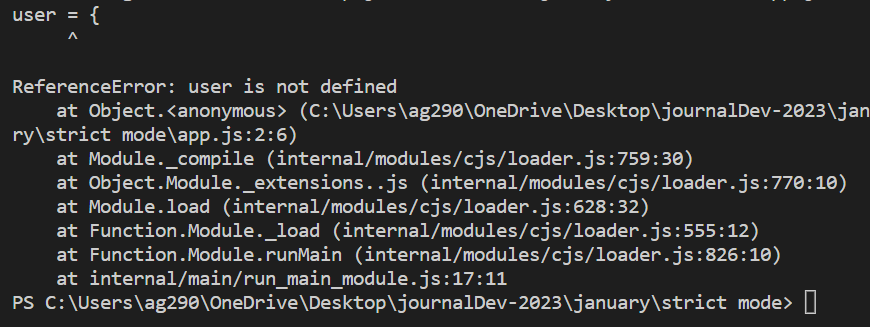
2. Deleting an object, variable, or function.
Example:
"use strict";
let user = {
name: 'John',
age: 30,
gender: 'male',
}
delete user; // Error here
Output:
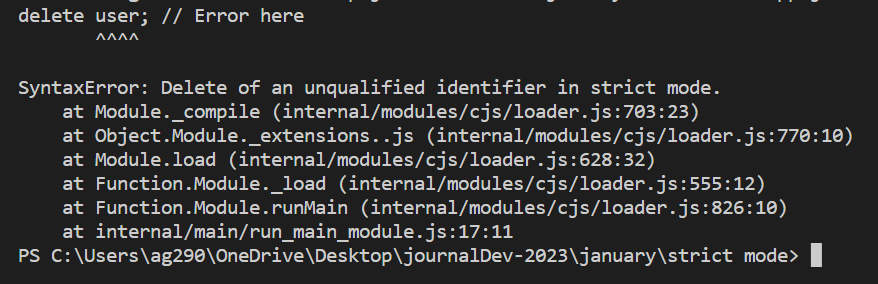
3. Duplicating a parameter name.
Example:
"use strict";
function hello(value, value){
console.log(value + " " + value);
}
Output:
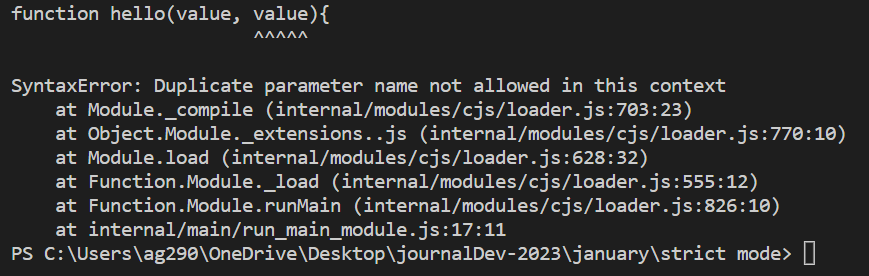
4. Writing to a read-only property, get-only property & undeletable property is not allowed.
Example:
"use strict";
const user = {};
Object.defineProperty(user, "name", {value:0, writable:false});
user.name = "John";
Output:
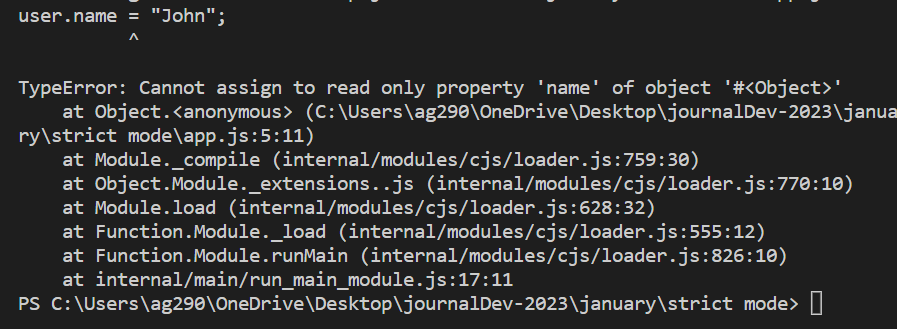
4. Octal numeric literals & Octal escape characters are not allowed.
Example:
"use strict";
let num = "\010"; // Error here
Output:
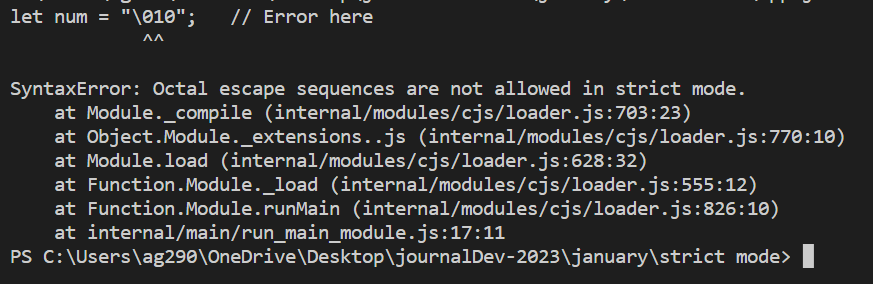
5. ‘eval’ cannot be used as a variable.
Example:
"use strict";
let eval = "Hello World";
console.log(eval);
Output:
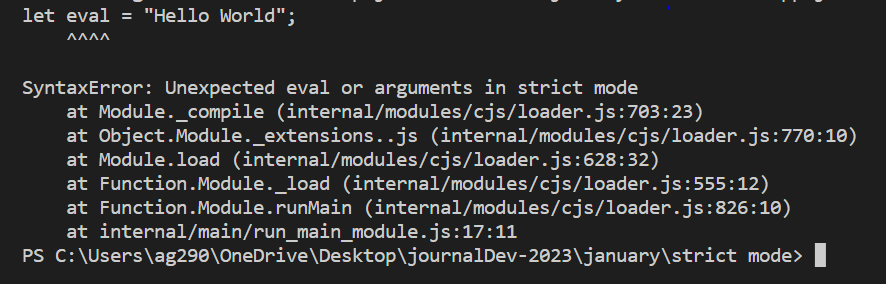
6. ‘arguments’ cannot be used as a variable.
Example:
"use strict";
let arguments = "Hello World";
console.log(arguments);
Output:
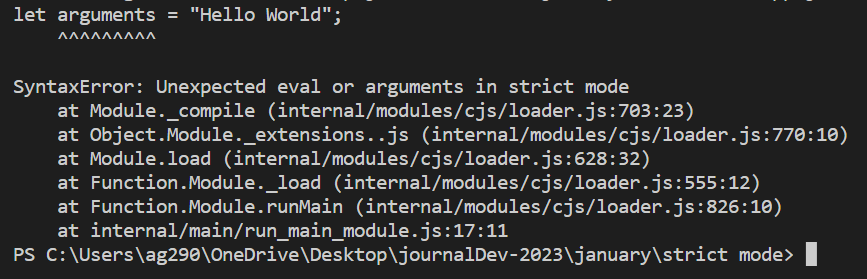
7. ‘with’ is not allowed.
Example:
"use strict";
with ([1, 2, 3]) {
console.log(toString());
}
Output:
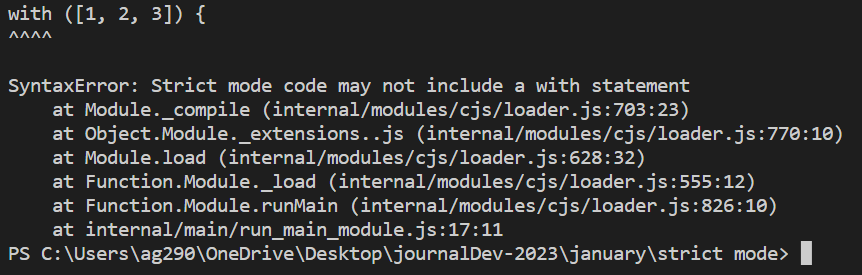
8. Keywords such as implements, interface, static, package, private, protected, public, let and yield are prohibited in strict mode.
Example:
"use strict";
let public = "public";
Output:
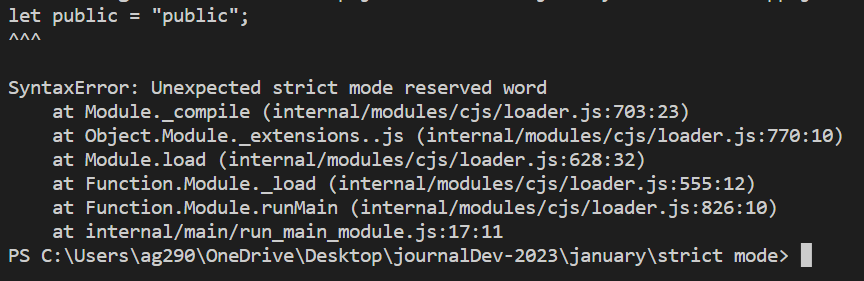
Summary
In this tutorial we have learned that JavaScript doesn’t throw errors in various places and failed slightly, this might waste the programmer’s time to figure out what goes wrong, the solution is to add ‘use strict’; or “use strict”; at the beginning of the script, this makes the script execute in strict mode and produces the visible errors that a programmer easily sees.
We have also covered the different actions that are not allowed in the strict mode so that when you give it a try, avoid these mistakes like using a variable without declaring it, which we usually do in JavaScript.
Hope this tutorial helped you to understand the concept of strict mode in JavaScript.
Reference
https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Strict_mode