Node.js has the console object, a widely used global object that provides a simple debugging terminal with a message. One of its methods is the console.log(). The console.log() can print any sort of variable that has previously been declared, as well as any message that needs to be shown to the user. By using this method developers can debug their Node.js application
Syntax:
console.log()
Parameter: It accepts a parameter, which may be an object, an array, or any message.
Return: It prints the value of the given parameter but does not return any value.
We recommend exploring additional resources for an in-depth understanding of various console methods.
Examples of Using console.log() Method
Let’s look at an example to understand how many ways the console.log() can be used to print in Node.js.
Example 1: Passing a Number as an Argument
One useful approach in Node.js for printing numbers to the console is the console.log() function. When this method gets a variable holding number as an argument, it makes sure that the provided number is displayed.
const variable = 5;
console.log(variable);
console.log("the value of variable is: ", variable);
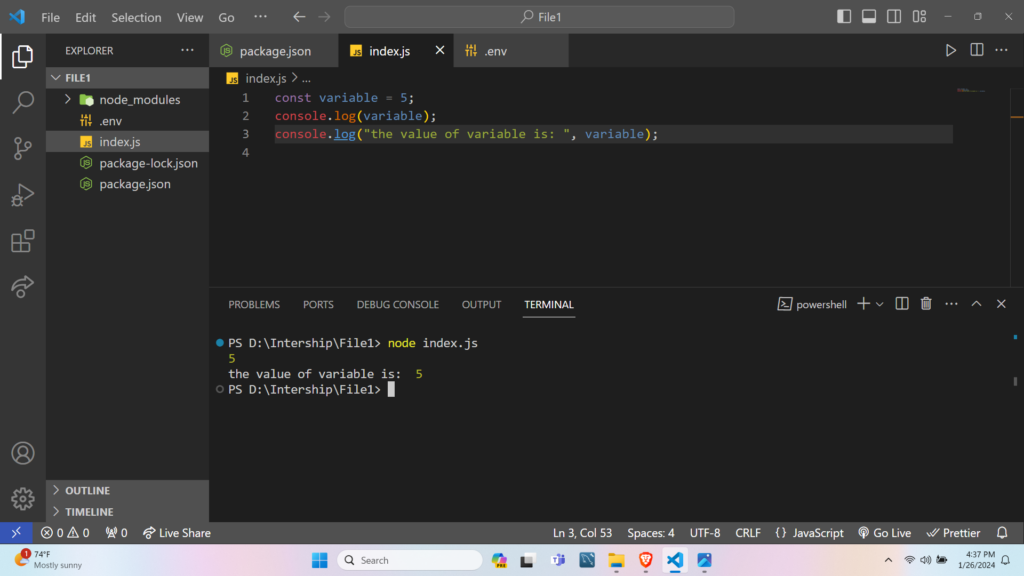
We have declared a variable assigning a value of 5. The first console.log method will simply log the value of the variable and in the second console.log method, it will log a message with the variable.
Example 2: Passing a String as an Argument
The console.log() method can display strings passed as an argument. This feature makes it an essential tool for watching and generating text information in the terminal.
const name = "Anurag Pandey";
console.log(name);
console.log("My name is " + name);
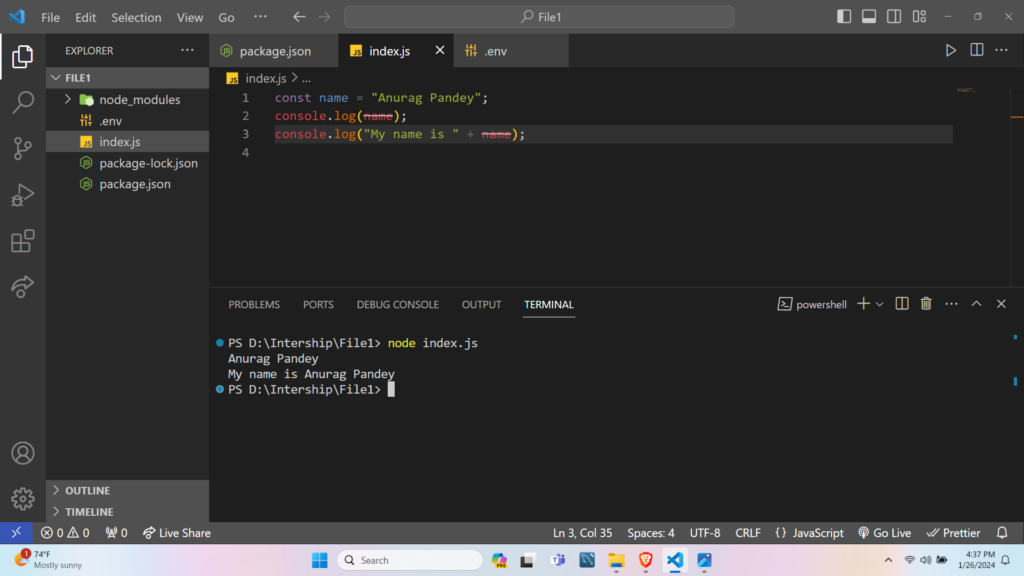
We have declared a variable called “name” in which we have assigned a value of “Anurag Pandey”. The first console.log method will simply log the value of the variable and in the second console.log method we have used “+” to concatenate a message string with the variable so that it will log a message with the “name”.
Example 3: Passing a Message as an Argument
The console.log() method is also used to display the specified message directly.
console.log("Anurag Pandey");
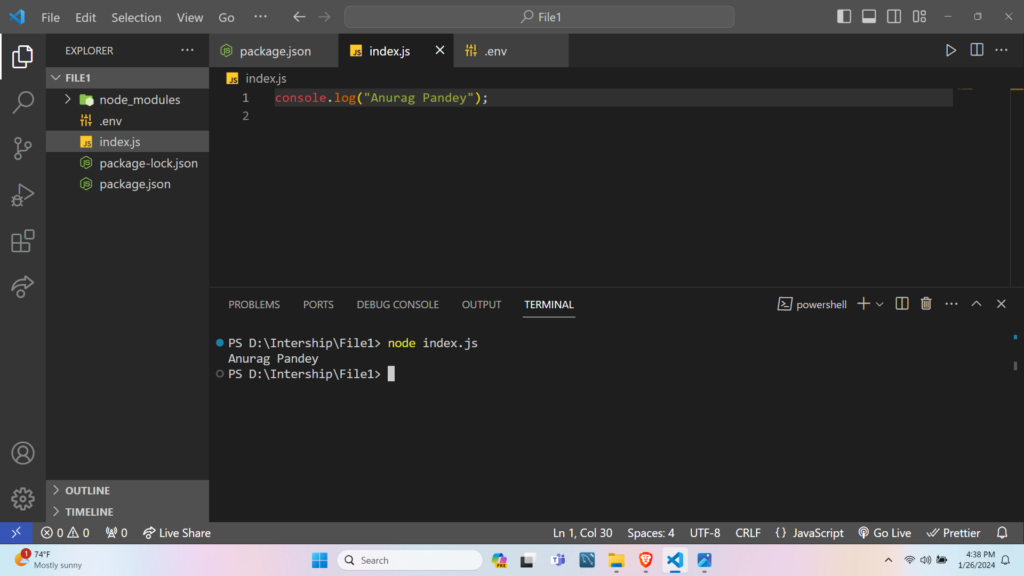
We have simply logged the message string “Anurag Pandey” in the console.
Example 4: Passing a Function as an Argument
The console.log() method, when provided with a function as an argument, will display the function return value.
function func() {
return (10*10);
}
console.log(func());
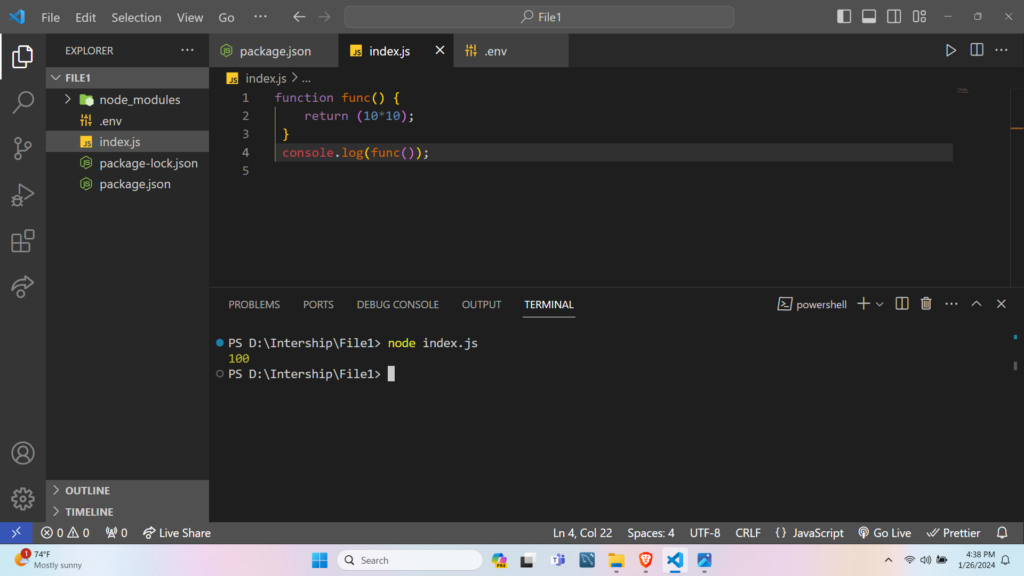
We have declared the “func()” function in which we have returned an expression (10*10) then it is called inside the console.log method which will print 100.
Example 5: Passing an Object as an Argument
The console.log() method, when provided with an object as an argument, will display the object itself. Developers have to use bracket notation or dot notation to access and log the object inside the console.
let obj = {name: "John", age: 30};
console.log(obj);
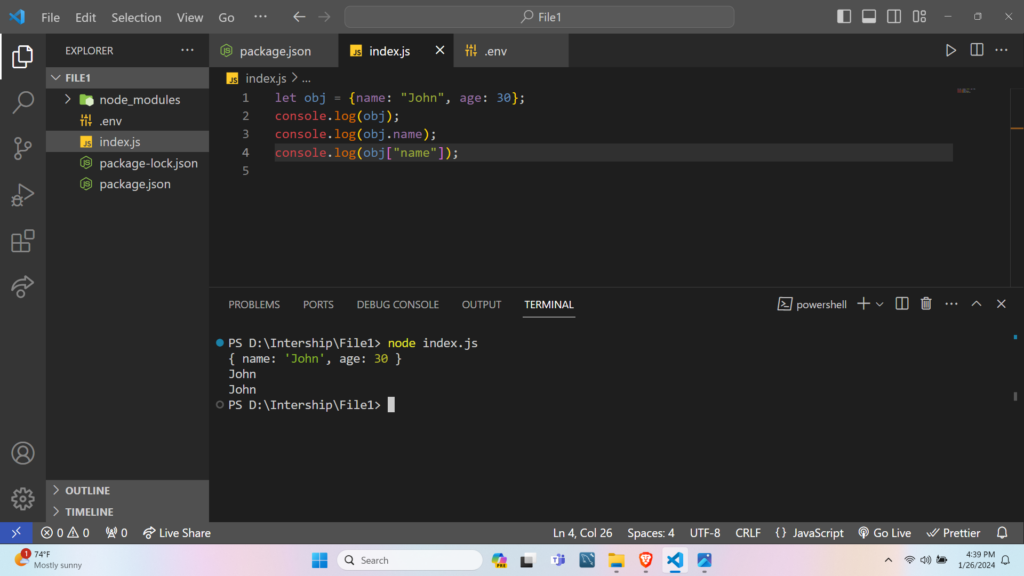
To access an object property inside an object, you can use dot notation or bracket notation.
console.log(obj.name);
console.log(obj["name"]);
Example 6: Passing an Array as an Argument
If you use the console.log() function with an array as an argument, the console will show the entire array. Inside the console, the array will always print as it is represented.
const myArray = ['apple', 'orange', 'banana', 'kiwi'];
console.log(myArray);
console.log("All the fruits are " + myArray);
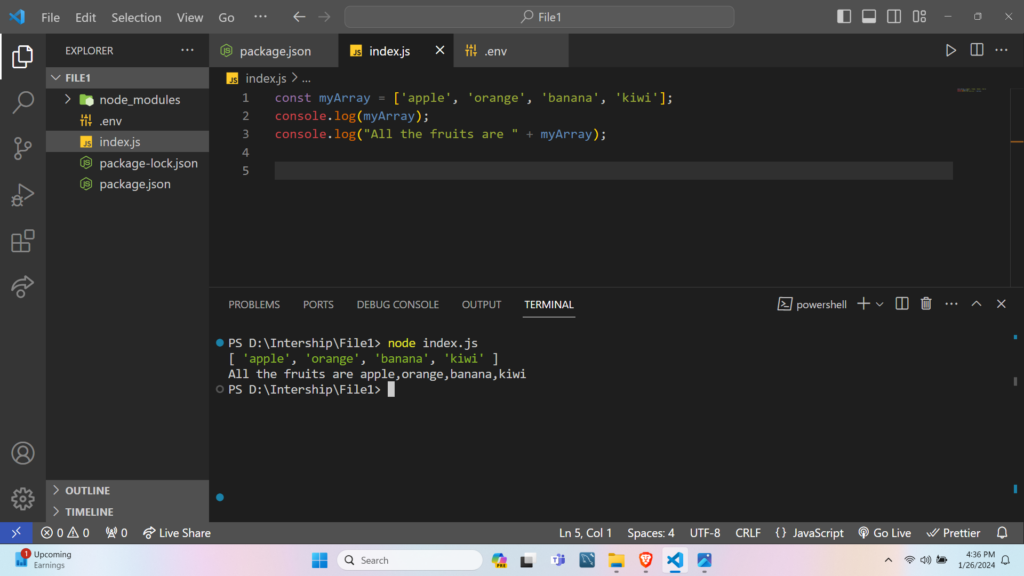
When you use “+” to concatenate a string and an array (as in “All the fruits are “+ myArray), the array is internally transformed into a string using the toString() function, which turns each array component into a string and places commas between them.
Also Read: JavaScript Operators (with Examples)
Conclusion
The console.log() function is an essential and vital tool in web development. Its ease of use and adaptability make it a priceless tool for developers, supporting debugging, and tracking code execution.
In this article, we have provided you with a brief overview of how to use console.log for different kinds of data, which will help you to better debug, track, and understand our code.
We sincerely hope you find this tutorial helpful and that it will enable you to become an even more proficient debugger!
Reference
https://nodejs.org/api/console.html#consolelogdata-args