In programming, conditional statements are used for making decisions based on different conditions. Given certain conditions, different courses of action are taken based on those determinants. A programmer defines those conditions and if their boolean value evaluates to True, then some pre-defined action is taken, else, the code defined for the false value is computed. Some examples of conditional statements in javascript are if…else statements and switch cases.
In this tutorial, you will learn about the if…else statement.
Also Read: JavaScript Error Handling: Try, Catch, and Finally
Table of Contents
The if…else Statement
The if…else statement controls the course of a program based on conditions. If a condition is true then one block of code is executed, else, another block of code is executed. There can be more than one condition specific to different blocks of code, in that case, we shall use an else…if condition.
Parameters of “if…else” Statement
Condition: Required to evaluate expressions to be true or false.
The first statement of an if…else condition must contain an “if” statement followed by the required condition as its parameter. If there are more than two conditions then the “if” condition is followed by “else-if” conditions with respective choices for each of those statements and at the end, we have the “else” condition. The “else” condition does not require any condition to be passed as a parameter because it is like the default statement which will be executed if no other condition is true.
Syntax of the JavaScript if…else Statement
The control flow of the program would be as given in the flowchart below:
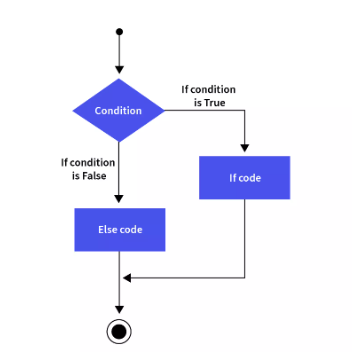
The syntax of the if…else condition is:
if (1stcondition==True)
{
// block of code to be executed
} else if (2ndcondition==False)
{
// block of code to be executed if 1st condition is false but 2nd condition is true
} else
{
// block of code to be executed if both conditions are false
}
Examples of if…else Statement
1st Example: Check if a number is even or odd
The code given below determines whether a number given by a user as input is either even or odd using the “if…else” statement.
var x = prompt("Enter a Value", "0");
var num1 = parseInt(x);
if (num1%2 ==0)
{
document.write("number is even");
}
else
{
document.write("number is odd");
}
After running the above code, the user will be prompted to enter a number that would look something like this:
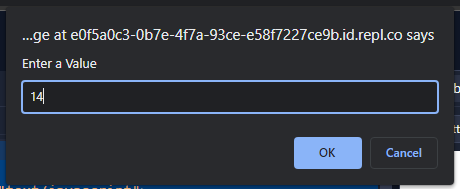
In this case, I have entered the number 14 to check the “if” condition. The output is shown below:
number is even
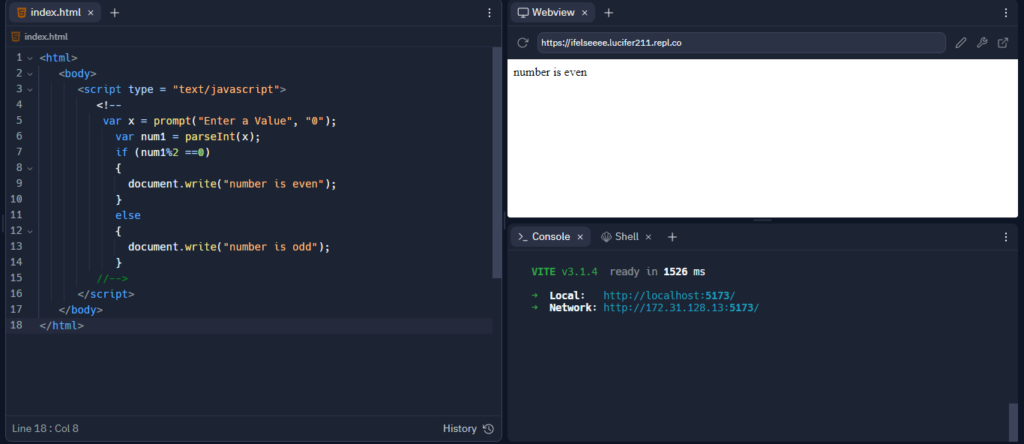
Note: An odd number can also be given as input to check if the “else” condition is working as well.
2nd Example: Check the current time and report on it
This program obtains the present time from the website and determines what time of day it is:
var Time_today = new Date().getHours();
if (Time_today<10)
{
document.write("It is morning time.");
}
else if (Time_today<20) {
document.write("It is day time.");
}
else
{
document.write("It is evening/night time.");
}
The output of the above code would be:
It is morning time
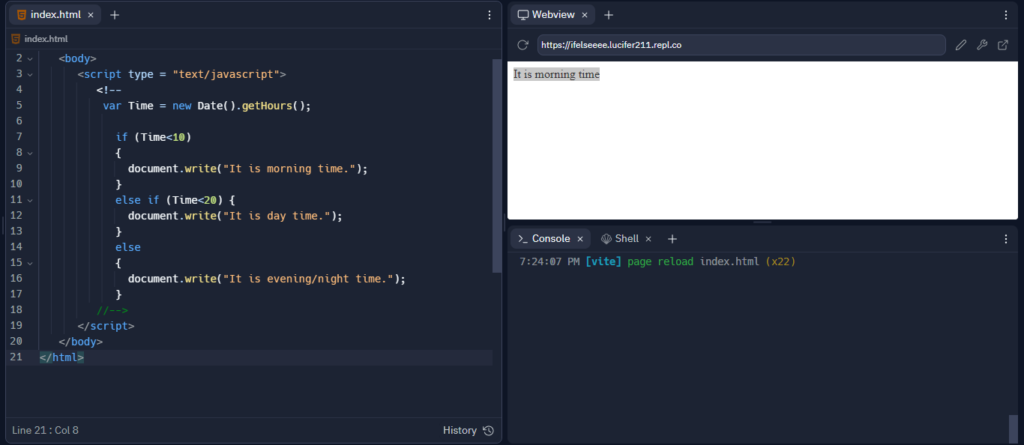
3rd Example: To check the sides of a triangle and determine its type
In this example, we will take the sides of a triangle as user input from the user and determine whether the triangle is equilateral(all sides equal), isosceles (any two sides) or scalene (none of the sides are equal). The following is the code for the same:
var firstside=prompt("Enter 1st side of triangle");
var secondside=prompt("Enter 2nd side of triangle");
var thirdside=prompt("Enter 3rd side of triangle");
var num1 = parseInt(firstside);
var num2 = parseInt(secondside);
var num3 = parseInt(thirdside);
if ((num1 == num2) && (num1==num3))
{
document.write("It is an equilateral triangle.");
}
else if ((num1 == num2 ) || (num2==num3) || (num3==num1))
{
document.write("It is an isosceles triangle");
}
else
{
document.write("It is a scalene triangle.");
}
Now, the user would be prompted to enter the three sides of a triangle. I entered the three sides as 5, 6, and 7. The output is shown below:
It is a scalene triangle.
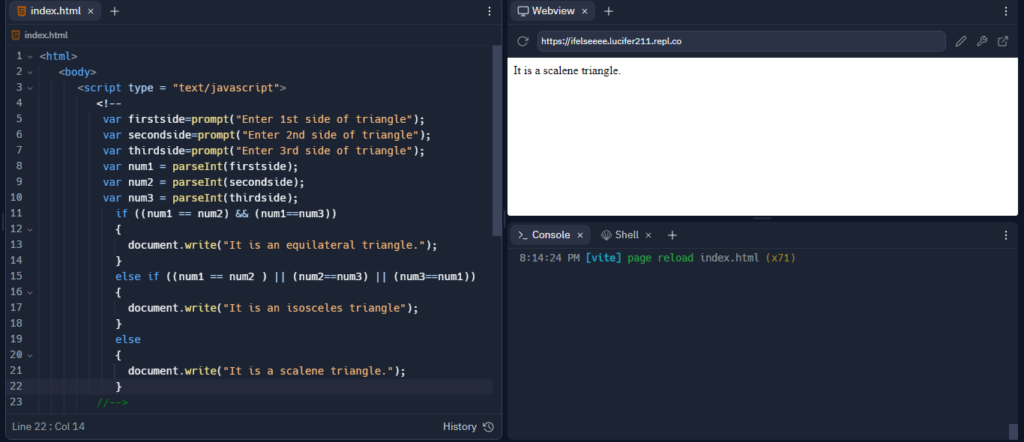
Summary
This article discusses the basics of if…else statements in JavaScript and their proper syntax. It describes the parameters which decide the course of a program. Illustrated via three different examples we can observe how we can use this particular control statement for various tasks.
Reference
https://developer.mozilla.org/en-US/docs/Learn/JavaScript/Building_blocks/conditionals