NumPy is a famous and often-used Python library that provides various mathematical functions when it comes to performing operations on arrays. These functions make computations involving array elements easier and more efficient. In this article, we’ll look at one such function, numpy.cbrt() which is used for calculating cube roots in Python. We’ll understand the implementation of this function in depth along with some examples.
Also Read: An Introduction to Python Comments
Introducing numpy.cbrt() Function
When it comes to performing operations like finding the cube root on array elements, we would be required to loop through each of those array elements and perform the cube root operation at each iteration. As easy as it may be, NumPy provides an even easier method of finding cube roots using the numpy.cbrt() method.
Syntax:
numpy.cbrt(x, /, out=None, *, where=True, casting='same_kind', order='K', dtype=None)
- x: Input values that we need to find the cube root of, can be an array, matrices or single values,
- out: Name of the output array. If set to None or not given a value, a new array will be created to store the resultant array containing cube roots,
- where: Used to specify the range of elements whose cube roots have to be found. This parameter has a default of True, meaning the cube roots of all the elements in the array should be computed,
- casting: Used to specify whether data type casting is allowed in the resultant array. Here, same_kind indicates that casting can be done between the same kind of data types (eg: casting can be done between float and integers as they are both numeric data types),
- order: Used to specify the memory layout of the output array. Here, K indicates that the original order of the input array should be preserved when laying out the memory for the output array,
- dtype: Used to specify the data type of the output array. Here, None indicates that the data type of the output array must be inferred according to that of the input array.
Now let us understand numpy.cbrt() in detail with some examples.
Calculating Cube Root of Each Element in NumPy Array
Here, we’ll look at some examples to understand how we can use numpy.cbrt() to find cube roots of elements, whether they are single or in an array. We will also see how changing the parameters of the function can change the output array and how we can extend this function to 2D arrays (matrices).
Calculating Cube Root of Single Values (Scalars)
We’ll start off with a simple example, where we will use numpy.cbrt() to find the cube root of a single value.
import numpy as np
x = 8
cube_root = np.cbrt(x)
print("Cube root of", x, "is", cube_root)
Output:
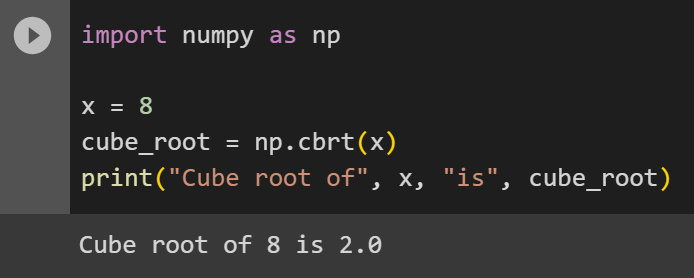
This function can also be used to handle negative scalar values.
Calculating Cube Roots of All Elements in an Array
Now we’ll apply numpy.cbrt() to an array (arr) of perfect cubes and calculate the cube roots of the elements present in that array.
import numpy as np
arr = [1, 8, 27, 64]
cube_roots = np.cbrt(arr)
print("Cube Roots:", cube_roots)
Output:
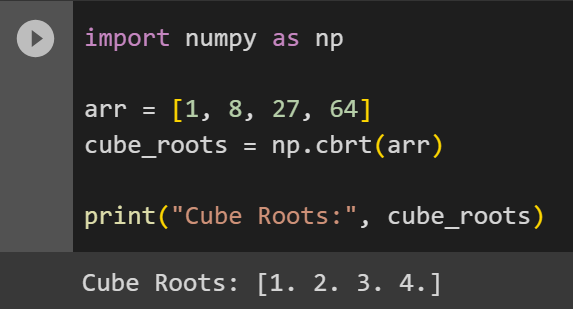
Using the where Parameter to Calculating Cube Root of Specific Elements
As mentioned earlier, the where parameter is used to find cube roots of only specific elements in an array. Here, we’ll find the cube roots of only the positive numbers in the input array.
import numpy as np
arr = np.array([1, -8, -27, 64])
cube_roots = np.cbrt(arr, where=(arr>0))
print("Cube Roots:", cube_roots)
Output:
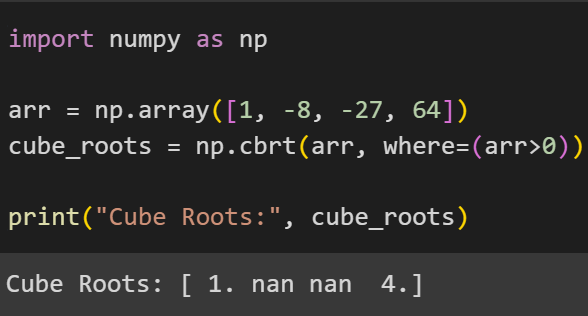
Here only the cube roots of positive elements are present in the output array, the negative values are represented by nan which is used to represent undefined values in an array.
Calculating Cube Roots of Elements in a Matrix
The usage of numpy.cbrt() can be extended from 1D array to 2D array or matrices as well. Here, we’ll look at how we can find the cube roots of all the elements in a 2×3 matrix.
import numpy as np
matrix = [[8, 27, 64],
[125, 216, 343]]
cube_roots = np.cbrt(matrix)
print("Cube Roots:")
print(cube_roots)
Output:
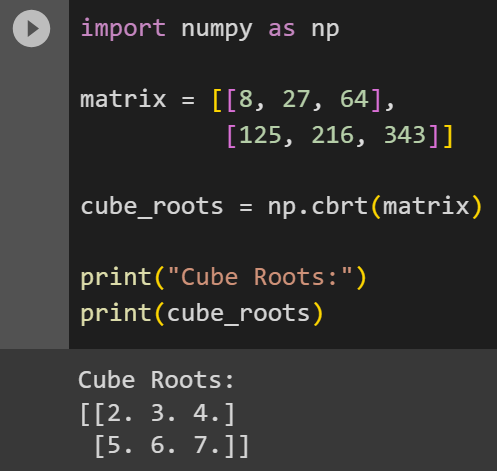
Conclusion
As we have seen in this article, NumPy provides us with the numpy.cbrt() function which makes computation the cube roots of single elements (scalars), array elements or matrix elements easier and more efficient. We looked at all of these implementations with supporting examples and also how we can find the cube roots of only a specific range of elements in an array. By knowing how to effectively use numpy.cbrt() we can perform calculations on arrays easier than before.
Reference
https://numpy.org/doc/stable/reference/generated/numpy.cbrt.html