Programming with arrays can be difficult at times but JavaScript provides us with some handy functions for working with arrays, map() is one of them. It is commonly used to iterate over an array and transform each element in it. However, the power of map() can be extended to iterate over objects as well. In this article, we’ll look at some methods for mapping through objects with examples.
JavaScript map() Method
The map() method makes transforming elements in an array easier by calling a function on each of them and creating a new array with the values returned by the function. It takes a callback function as an argument which is executed for each element. Let us look at an example of how we can use map() on arrays.
Example:
Here, we have an array of marks containing some marks. We want a new array that contains +5 added to each element in the marks array.
const marks = [90, 85, 76, 88, 95]
// Using map() to increase each element in marks by 5
const newMarks = marks.map((mark) => mark+5)
console.log(newMarks);
Output:
[95, 90, 81, 93, 100]
We call map() on our original array marks and pass in each element (referred to as mark, in this case) and return the new value mark+5, this gives the new array newMarks containing the new values, while marks remain the same.
Mapping Over Objects in JavaScript
Objects in JavaScript are non-iterable, however, there exist methods that can loop over each key and their values. Let’s see them one by one.
Methods to Mapping Over Objects in JavaScript:
- Mapping Object Keys
- Mapping Object Values
- Mapping Object Properties
- Mapping using Custom Functions
1. Mapping Object Keys
JavaScript provides Object.keys() to transform the keys of an Object into an array while keeping its corresponding values unchanged.
Example:
Here we’ll look at an example where the keys of the given object book are changed to uppercase.
const book = {
title: 'animal farm',
author: 'George Orwell',
pages: 140
};
const mappedBook = Object.keys(book).map(key => key.toUpperCase());
console.log(mappedBook);
//This code gives the output: ['TITLE', 'AUTHOR', 'PAGES']
Output:
['TITLE', 'AUTHOR', 'PAGES']
To iterate through the keys of the object book we must first convert it into an array, this is done using Object.keys(), which returns an array of keys. We can now apply the map() function to this newly returned array and call the in-built function toUpperCase() on each element (referred to as key, in this case) and convert it to uppercase.
The new array containing the uppercase keys is then stored in a constant mappedBook.
2. Mapping Object Values
Just like Object.keys(), JavaScript also provides a method Object.values() which is used to transform the values of an object into an array while keeping the keys intact.
Example:
Here let’s take an example of an object animalCount, which contains the number of each animal. However, we want to increment the original count of each animal by 3.
const animalCount = {
cats: 6,
dogs: 3,
birds: 8
};
const mappedAnimalCount = Object.values(animalCount).map(value => value+3);
console.log(mappedAnimalCount);
Output:
[9, 6, 11]
In this code, Object.values() returns the values of the object as an array. On the array, map() is called to iterate over each element (referred to as value in this case) and then incremented by 3. The transformed array is stored in the constant mappedAnimalCount.
3. Mapping Object Properties
Now that we know how to iterate through keys and values separately, we can move on to iterating through a key-value pair. This can be done using Object.entries() which returns each key-value pair as a separate array. So our final output is basically an array of arrays!
Example:
Let’s understand this with an example of an object temperature which contains the name of cities and their corresponding temperatures.
We need to iterate through each key-value pair in the object and return a new array of objects containing the city name and weather based on if the temperature is hot or cold. The logic of checking temperature can be implemented using a simple conditional statement.
const temperatures = {
paris: 19,
tokyo: 26,
mumbai: 30
};
const mappedTemperature = Object.entries(temperatures).map(([city, temperature]) => ({
city,
weather: temperature >= 20 ? 'Hot' : 'Cold'
}));
console.log(mappedTemperature);
First, let’s see the output when we use Object.entries() on the object temperature.
Output:
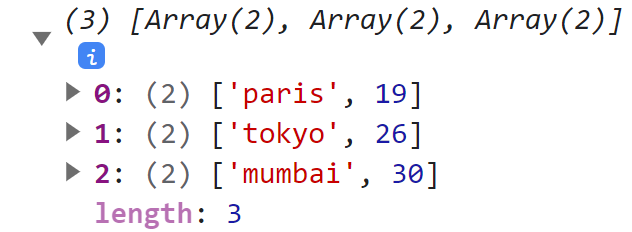
We obtain a 2-dimensional array which we can now map over.
The output after mapping through the 2D array and transforming each key-value pair:
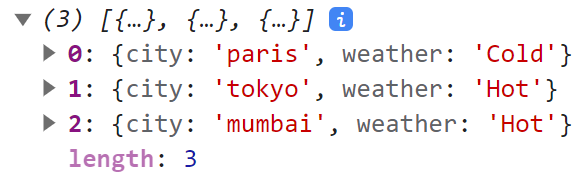
In this code, we iterate over each property (a key-value pair) using Object.entries() which returns a 2D array. The map() is called on each key (referred to as city in this case) and its corresponding value (temperature, in this case).
This returns new objects containing a city and weather, where the weather is checked using a conditional statement on the temperature to see if its value is greater than or equal to 20. The array of objects is stored in a constant called mappedTemperature.
4. Mapping using Custom Functions
As seen above, JavaScript provides us with various functions that help us iterate through objects but we can also go the extra mile and write our own custom functions to map through objects.
Example:
Here’s an example of a custom function tripleValue() which iterates through an object and returns the keys as it is but triples the value corresponding to it.
//Custom function for tripling the values in an object
function tripleValue(object) {
const newObject = { };
for (let key in object) {
if (object.hasOwnProperty(key)) {
// Obtaining the values corresponding to each key
const value = object[key];
// Tripling the value and assigning it to the new object
newObject[key] = value * 3;
}
}
return newObject;
}
const oldObject = { 'a': 2, 'b': 3, 'c': 5 };
const tripledObject = tripleValue(oldObject);
console.log(tripledObject);
Output:
{ 'a': 6, 'b': 9, 'c': 15 }
In the code above, the function tripleValue() takes an object as a parameter.
We declare an empty object newObject which will contain the keys and its new tripled values. Then we run a ‘for…in’ through the object and check if a certain key exists using the in-built hasOwnProperty() function.
Then we store the values corresponding to each key in a constant value and then we triple the value and store it with its corresponding key in newObject. The function finally returns newObject. Now we can use this custom function on our object oldObject containing key-value pairs of alphabets and numbers and store the new object in a constant tripleObject.
Conclusion
Here we see that by using map() to its full potential we can transform not just arrays but objects as well. We have explored the usage of various object transformation functions such as Object.keys(), Object.entries(), Object.values() and also looked at how we can write our own custom functions to iterate through objects if required. Being able to map through objects can be a key achievement in your JavaScript journey.
Reference
https://stackoverflow.com/questions/14810506/map-function-for-objects-instead-of-arrays