Splitting a string may not seem like a very useful feature but it is super necessary, especially in JavaScript-based languages that heavily rely on string manipulation. Splitting a string can be used in many places such as when you have to extract the username and password from the connection string, parse data from an API, get user request data, etc.
These are just a few examples, once you start progressing in Node.js and start building applications, you will realise that splitting strings is required very frequently so it is better to learn today than to worry later. In this tutorial, I will teach you everything about splitting a string in a very simple way. Let’s start with the function that let this operation happen.
An Introduction to JavaScript split() Method
The split() method of JavaScript divides a string value (plain text value) into substrings, stores them in an array, and returns that array. The string is divided based on a pattern which is passed in the first argument when calling the method.
Syntax:
split(separator, [limit])
Parameters:
- separator – accepts a character or a regular expression. If nothing passes, it will return the entire string inside an array.
- [limit] – a limit that you may specify as an integer. It is optional.
The split() method will not change the original string in any way which means you can access it over and over again.
If we pass a blank separator to this method, it will perform a character split, meaning every character inside the string is separated.
When we pass a space character to the separator, it will perform a word split.
Splitting and Modifying Strings in Node.js
Let’s see how to use this method. Just keep in mind that this method can be only used on strings, not on any other datatype but it can be applied on strings stored in different containers like variables, arrays, and objects.
Split a String Stored in a Variable
Following is the sample code enclosing a variable containing a string.
const studentName = 'Sarah Lee';
Let us split every character in the string that is stored in a studentName variable using the split() method.
studentName.split(''); // ["S", "a", "r", "a", "h", " ", "L", "e", "e"]
Next, let’s pass a string with a space character.
studentName.split(' '); // ["Sarah", "Lee"]
Now, let us pass a limit to split our characters.
studentName.split('', 4); // ["S", "a", "r", "a"]
Let’s add more words and test out words split.
var sarahZodiac = 'Sarah is a Gemini'
sarahZodiac.split(' ', 2) // ["Sarah", "is"]
Split a String Stored in an Array
Let’s say we have an array of names with us, then how do split them?
const nameList = ['Tarry Wood', 'Aneesha Shaikh', 'Bamidele Onibalusi'];
To select a name, we will first select the array and then one of the strings using the index match.
nameList[2].split(''); // ["B", "a", "m", "i", "d", "e", "l", "e", " ", "O", "n", "i", "b", "a", "l", "u", "s", "i"]
You might also like: How to Remove a Particular Element from an Array in JavaScript.
Split a String Stored in an Object
Let’s now try to perform a split operation on a JS object containing strings as the key’s values.
const productList = { name: 'Nike Air Force 1', price: '$109.5', category: 'Women'}
productList.name.split(' ', 3) // ["Nike", "Air", "Force"]
Passing Characters to the Separator Parameter
We haven’t yet tried passing any characters to the separator parameter. Let us take a look at that.
const nikeShoes = 'Nike Air Force 1';
nikeShoes.split('Nik'); // ["", "e Air Force 1"]
The output we have here indicates the characters we specified in the separator argument have been chopped off of the string.
In all the above examples, I haven’t logged the value to the console for simplicity, but if you want you can pass the function into a variable and then use console.log to log the returned array.
var nikeShoes = 'Nike Air Force 1';
nikeShoes = nikeShoes.split('Nik');
console.log(nikeShoes);
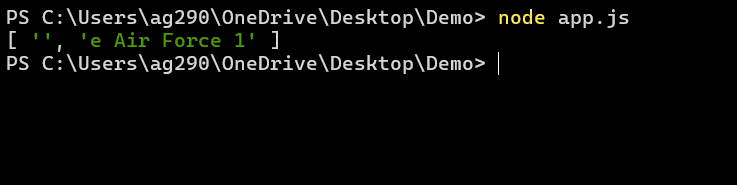
Error Handling While Splitting Strings
If you arrived at this section after reading the whole article, I hope you better have an idea about what the split() function is and how it is used with variables, arrays and objects to slit the string contained in them.
Let’s end this tutorial with some error-handling techniques so you can save yourself from unexpected program behaviour when trying to implement the above concept.
1. Make sure that the string on which you are applying the split operation is not empty. For this, you can use an if/else statement.
if (!string) {
console.log("String is empty")
}
2. When splitting a string with manual arguments, make sure that it exists in the string, if not, the split will not work.
if (string.indexOf('Nik') === -1) {
console.log("Separator not exist in the string")
}
3. Make sure the unexpected characters in the string are cut off first for successful operation.
string = string.replace(/[^a-zA-Z0-9\s]/g, '')
4. You can use the trim() method on the string before performing the split operation to remove unwanted whitespace characters to get the correct result.
string = string.trim()
If you don’t know how to implement the if/else statement, you can read “JavaScript if…else Conditional Statement” and click here to read about the trim() method.
Conclusion
The split() method of JavaScript is useful for developers to split a string, be it stored in a variable, in an array, or an object. This string splitting has prominent use cases in NodeJS like authentication of clients, extracting data from strings, etc. Not limited to Node only, but can also be a very useful feature in programming languages like Python, Java, etc. Thank you for reading until the end.
Reference
https://stackoverflow.com/questions/15134199/how-to-split-and-modify-a-string-in-nodejs