If you have been coding in JavaScript for longer, you have already covered the methods dealing with numbers and strings, which are frequently used. There are other special methods in JavaScript that can turn out to be important while creating web pages. The alert(), prompt() and confirm() method displays multiple kinds of dialog boxes. In this tutorial, we will learn about these methods with the help of practical examples.
JavaScript alert() Method
The alert() method in JavaScript is used to show a virtual alert box that provides a message or warning to the user. The alert() requires the user to close the alert box before moving to the other sections of the website. It is frequently used for displaying informational messages, notifications, cautions and error alerts.
Syntax
alert(message);
- ‘message’: The text you want to display in the alert dialog.
Example
Below is an example of the alert method to help you understand how it works:
<!DOCTYPE html>
<html lang="en">
<head>
<title>Alert method</title>
</head>
<body>
<p>Click to see the alert </p>
<form>
<input type = "button" value = "Alert" onclick = "myFunc();" />
</form>
<script>
function myFunc() {
alert("Here is the alert!");
}
</script>
</body>
</html>
Output
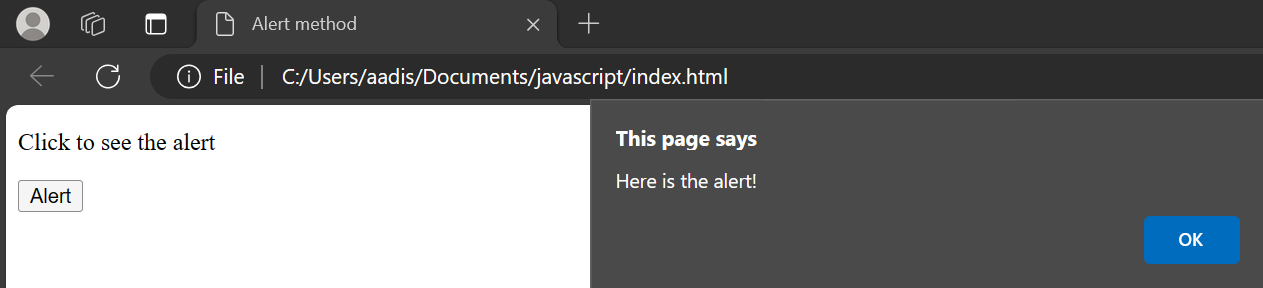
As you can see, the alert() method returns “Here is the alert!’ when you click the button “Alert”.
When you click the “OK” button, the alert will be dismissed.
JavaScript prompt() Method
The prompt() method in JavaScript is used to display a dialogue box that prompts users to fill in the input. This method provides the user to fill in the data which can then be used by the JavaScript program. It is frequently used to gather information from users or to request some input from users.
Syntax
prompt(message, defaultText);
- ‘message’: The text you want to display in the dialog.
- ‘defaultText’: The default value which will be displayed in the input field of the dialog box. (optional)
Example
Below is an example of the prompt method to help you understand how it works:
<!DOCTYPE html>
<html lang="en">
<head>
<title>Prompt method</title>
</head>
<body>
<p>Click to see the prompt </p>
<form>
<input type="button" value="Prompt" onclick="myFunc();" />
</form>
<script>
function myFunc() {
let userInput = prompt("Please enter your name:", "User");
if (userInput !== null) {
alert("Hello, " + userInput + "! Welcome to our website.");
} else {
alert("You have canceled the prompt.");
}
}
</script>
</body>
</html>
Output
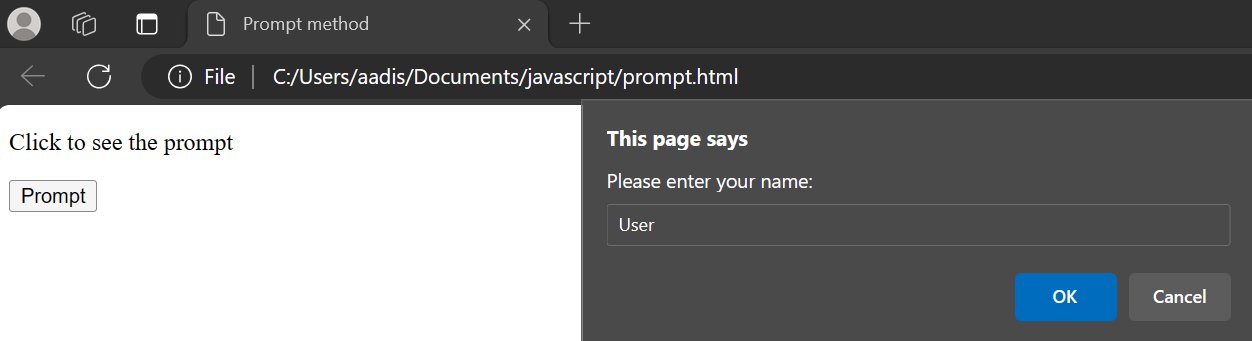
As you can see, the prompt() method returns a dialog box that prompts you to fill in the input when you click the button named “Prompt”.
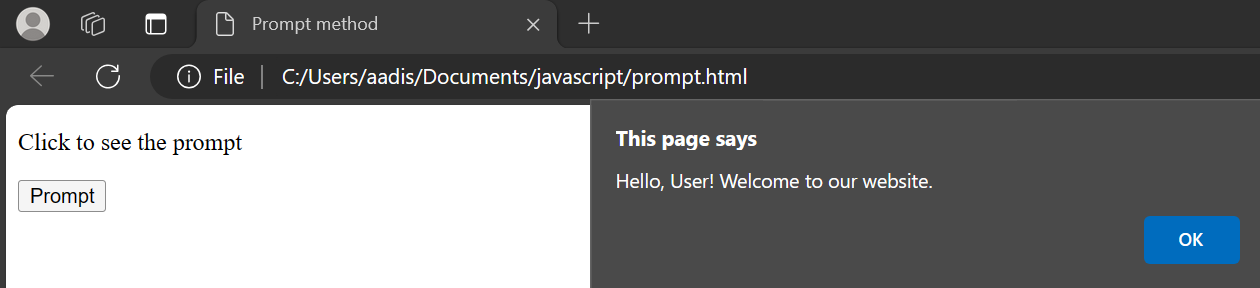
After you provide the input and click “OK”, a message is displayed welcoming you with the input which you have entered.
If you cancel the prompt by clicking “Cancel”, a message is displayed which will indicate the cancellation.
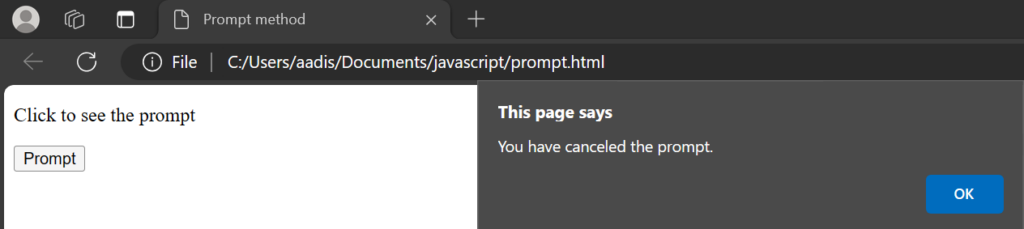
JavaScript confirm() Method
The confirm() method in JavaScript is used to display a dialog box with a message and the OK and Cancel buttons. The confirm() method allows users to confirm or cancel an action. It is frequently used to ask users to confirm a decision or action.
Syntax
confirm(message);
- ‘message’: The text you want to display in the dialog.
Example
Below is an example of the confirm method to help you understand how it works:
<!DOCTYPE html>
<html lang="en">
<head>
<title>Confirm method</title>
</head>
<body>
<p>Click to see the confirmation dialog</p>
<form>
<input type="button" value="Confirm" onclick="myFunc();" />
</form>
<script>
function myFunc() {
let result = confirm("Do you want to proceed?");
if (result === true) {
alert("User confirmed.");
} else {
alert("User canceled.");
}
}
</script>
</body>
</html>
Output
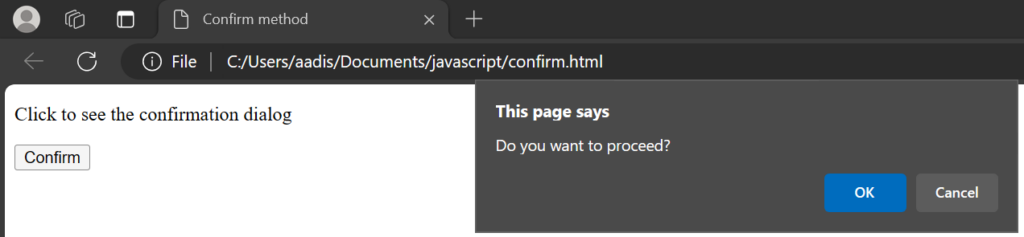
As you can see, the confirm() method displays a dialog box with a message and “OK” and “Cancel” buttons when you click the button named “Confirm”.
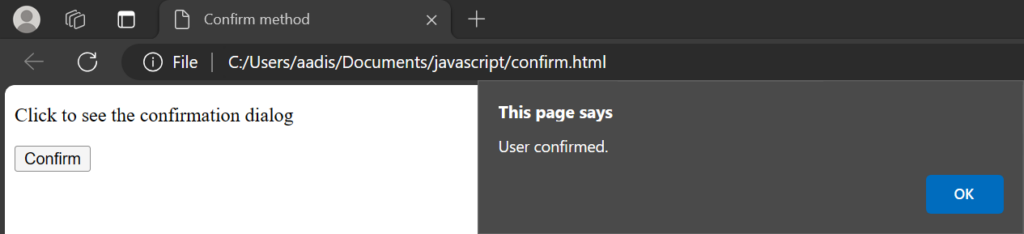
You will see the confirmation dialog.
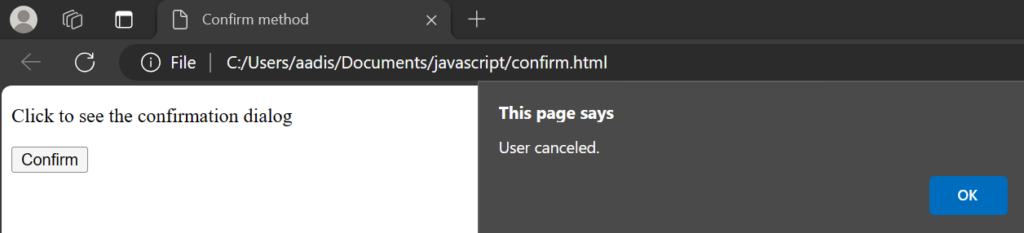
If you cancel the prompt by clicking “Cancel”, you will see a message which will indicate the cancellation.
Conclusion
In JavaScript, the alert(), prompt() and confirm() methods can be turned out as valuable tools to interact with users. By combining these methods into your website, you can communicate with users and extract information from them. It is important to understand when and how to use these methods. We hope you enjoyed it.
Also Read:
- JavaScript setInterval() Method – Live Counter & Digital Clock Examples
- Pause JavaScript Code Execution – Top 3 Methods With Examples