While building web applications that run in the browser, memory management is one of the important steps to consider. It often occurs when garbage collection fails. In this tutorial, we will learn about memory leaks in JavaScript, exploring the causes of them with the help of practical implementation and highlighting common mistakes to avoid.
Introduction to Memory Leaks
A memory leak in JavaScript occurs when objects are retained in memory unintentionally even when they are no longer needed which then leads to an increase in memory consumption and performance issues. It prevents the JavaScript engine’s garbage collector from freeing up that memory space.
Why Memory Leaks Matter
Memory leaks in JavaScript matter for many reasons:
- Performance: Memory leaks make your application less efficient and responsive by slowing it down.
- Resource Utilization: Memory leaks can increase the utilization of system resources by your application which can impact the performance of other processes that are running on the user’s device.
- Stability: Extended memory leaks have the potential to result in application crashes and can make your application unresponsive.
Causes of Memory Leaks
Memory leaks can occur in various situations:
1. Unintentional Variable Retention
In JavaScript, closures can accidentally capture variables from their surroundings and later prevent those variables from being garbage collected even when they are no longer needed.
let globalObject = { data: "some data" };
function doSomething() {
let localVar = globalObject;
console.log("Inside doSomething(): localVar assigned with globalObject:", localVar);
}
doSomething();

In this function, ‘localVar’ is declared and assigned the value of ‘globalObject’. When the ‘doSomething’ function is executed, the ‘localVar’ variable holds a reference to ‘globalObject’ which prevents it from being garbage collected.
Prevention
To avoid this, it is important to ensure that reference objects are released when they are no longer needed.
In this function, setting ‘localVar’ to ‘null’ would prevent the memory leak.
let globalObject = { data: "some data" };
function doSomething() {
let localVar = globalObject;
console.log("Inside doSomething(): localVar assigned with globalObject:", localVar);
localVar = null; // Release the reference to globalObject
console.log("Inside doSomething(): localVar set to null to release the reference to globalObject");
}
doSomething();

In the above code the ‘localVar’ is set to ‘null’. This helps prevent a memory leak by allowing the JavaScript engine’s garbage collector to reclaim memory previously occupied by the object.
2. Event Handlers
If you fail to remove event listeners it can lead to memory leaks because the DOM elements preserve references to event handlers even after they are no longer required.
const button = document.getElementById('myButton');
function onClickHandler() {
console.log('Button clicked');
}
button.addEventListener('click', onClickHandler);
console.log("Event listener added for 'click' event on button");

In this function, if the button is removed from the DOM or if the listener is no longer needed. If it is not removed it can lead to a memory leak.
Prevention
To avoid this, it is important to provide a way to remove event listeners. You can also use the ‘removeEventListerens’ function.
const button = document.getElementById('myButton');
function onClickHandler() {
console.log('Button clicked');
}
button.addEventListener('click', onClickHandler);
button.removeEventListener('click', onClickHandler);
console.log("Event listener removed for 'click' event on button");

3. Timers and Intervals
If you fail to clear timeouts and intervals when it is no longer needed, it can lead to memory leaks because of the continuous execution of the timer or interval function.
function startInterval() {
setInterval(() => {
console.log('Interval running...');
}, 1000);
}
startInterval();
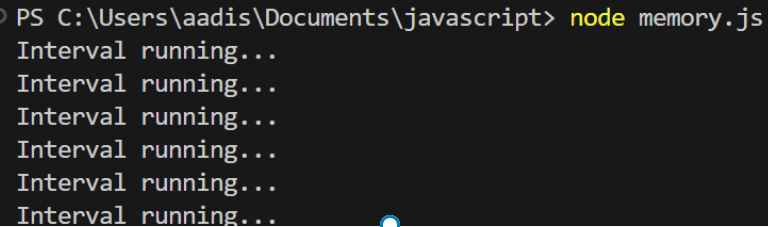
In this function, the interval is not cleared. It will continue to run indefinitely which can cause memory leaks.
Prevention
To avoid this, always clear intervals when it is no longer required.
In this function, you can use the ‘clearInterval’ function to stop the interval when no longer required.
let intervalId; // Declare intervalId globally
function startInterval() {
intervalId = setInterval(() => {
console.log('Interval running...');
}, 1000);
}
// Call this function when the interval is no longer needed
function stopInterval() {
clearInterval(intervalId);
console.log('Interval stopped');
}
// Start the interval
startInterval();
// Stop the interval after 5 seconds (for demonstration purposes)
setTimeout(stopInterval, 5000);
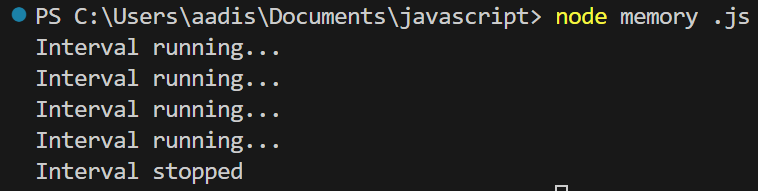
This code correctly declares a global variable ‘intervalId’ to store the interval ID and stops the interval using ‘setTimeout’ after 5 seconds, the interval will be stopped using ‘clearInterval’, and “Interval stopped” will be logged to the console. This ensures proper cleanup of intervals to prevent memory leaks.
Conclusion
It can be a challenging task to figure out the cause of memory leaks and also find out ways to resolve these issues but if you have a better understanding of their reasons and additionally best practices to prevent such problems, you are all set to build efficient web applications. In this tutorial, we have covered the causes and ways to resolve memory leaks. We hope you enjoyed it.
Also Read:
- JavaScript Error Handling: Try, Catch, and Finally
- JavaScript Strict Mode: What it Does and Doesn’t Allow
Reference
https://stackoverflow.com/questions/16256487/how-do-i-create-a-memory-leak-in-javascript