Did you know that the Node package manager has a very cool library to work with Date and Time? If not, no worries! In this article, we will be exploring the ‘date-and-time’ library of Node.js and seeing how we can experiment with different formats for presenting the Date and Time in Node.js projects. So, let’s begin.
Node.js date-and-time Module
The ‘date-and-time‘ module helps with dates and times in JavaScript. It’s simple, small, and easy to understand. Nowadays, many JavaScript tools are big and complicated, relying on lots of other tools. Keeping things simple and small is crucial, especially for basic tools like handling dates and times.
Key Features
This library proves to be very effective in our projects and some of the best reasons why it is so popular are given below:
- This library has a Minimalist Size that is approximately 2KB when minified and gzipped.
- Extensible with Plugins as it supports easy customization through a plugin system.
- The library offers Multi-language support.
- The library is designed to work across different platforms, providing Universal Compatibility.
- It provides support for TypeScript as well.
- Functions on older browsers, including IE6, for wider applicability.
Installation Syntax
Node Package Manager or NPM makes it easy for JavaScript developers to install, manage, and share code libraries, simplifying the workflow and handling project dependencies in Node.js environments. To use the given ‘date-and-time’ library, we need to first install it in our environment using the npm.
The command to install the ‘date-and-time’ library is given below:
npm i date-and-time
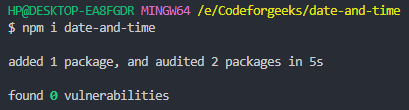
Importing Syntax
Now to use the given library in our projects, we need to import the module. The syntax to do so is given below.
CommonJS Modules:
const date = require('date-and-time');
ES6 Modules:
import date from 'date-and-time';
If you want to use ES Modules directly in Node.js, you can do so by specifying “type”: “module” in your package.json file. Alternatively, you can change the file extensions of your JavaScript files from .js to .mjs.
This configuration allows Node.js to recognize and handle ES Modules natively or you might run into errors like ‘SyntaxError: Cannot use import statement outside a module’, do check out our full explanatory article on the same – Resolving ‘SyntaxError: Cannot use import statement outside a module’
Node.js date-and-time Module API Methods
Now that we are known this library, let’s learn some of its most useful API methods and their real-life implications. We will discuss them with relevant examples to test and learn their work.
1. parse(dateString, arg[, utc])
In the date-and-time library in Node.js, the parse(dateString, arg[, utc]) function parses a date string. It’s important to note that this method is unique to the date-and-time library and differs from the built-in JavaScript Date.parse() method.
If parsing fails, the function returns an Invalid Date as a Date object (not NaN or null), with a default date of January 1, 1970, and a time of 00:00:00.000. Missing values are automatically filled with these defaults. The maximum parsable date is December 31, 9999, and the minimum is January 1, 0001.
Syntax:
date.parse(dateString, format)
Parameters: dateString is a string for date and time, and format is a string defining the expected dateString format. If the dateString does not include a time zone offset, this function assumes it represents a local date and time. You can set the utc option to true (the third parameter) if the dateString is a UTC date and time.
Example 1:
const date = require('date-and-time');
const dateString = '2024-02-12 12:30:00';
const parsedInfo = date.parse(dateString, 'YYYY-MM-DD HH:mm:ss');
console.log(parsedInfo);
This script uses the ‘date-and-time’ library to parse the date string ‘2024-02-12 12:30:00’ according to the specified format ‘YYYY-MM-DD HH:mm:ss’, and then logs the parsed information (a Date object) to the console.
Output:

Example 2:
const date = require('date-and-time');
const dateString = '2025-02-29';
const parsedInfo = date.parse(dateString, 'YYYY-M-DD');
console.log(parsedInfo);
This script attempts to parse the date string ‘2025-02-29’ according to the specified format ‘YYYY-M-DD’, and then logs the parsed information (a Date object) to the console. It will show ‘Invalid’ because February 29, 2025, does not exist (not a leap year).
Output:

2. format(dateObj, arg[, utc])
The format(dateObj, arg[, utc]) method is employed to structure the date based on a specific pattern.
Syntax:
date.format(dateObj, formatString[, utc])
Parameters: This function accepts two parameters, dateObj, which is the date object, and formatString, representing the new string format for displaying the date.
Strings in parentheses within the formatString are treated as comments and ignored. By default, this function outputs a local date and time string. Set the utc option (3rd parameter) to true if you want a UTC date and time string.
Example:
const date = require('date-and-time');
const currentDate = new Date();
const dateFormat = 'dddd, MMMM D YYYY HH:mm:ss';
const formattedDate = date.format(currentDate, dateFormat);
console.log('The Current date and time in the given format:', formattedDate);
This script generates the current date and time as a Date object, formats it into a string representation using the specified format ‘dddd, MMMM D YYYY HH:mm:ss’, and finally, logs the formatted date to the console.
Output:

3. compile(formatString)
The compile(formatString) function is used to assemble a format string for the parser, saving the pattern and eliminating the need to add the format repeatedly.
Syntax:
date.compile(formatString)
Parameters: This function accepts the formatString as a parameter.
Example:
const date = require('date-and-time');
const formatString = 'dddd, MMMM D YYYY HH:mm:ss';
const mould = date.compile(formatString);
const parsedDate = date.parse('6 April 2009', 'D MMMM YYYY');
const formattedDate = date.format(parsedDate,mould);
console.log('Formatted Date:', formattedDate);
This script compiles a format string ‘dddd, MMMM D YYYY HH:mm:ss’ into a function, parses the date string ‘6 April 2009’ using the format ‘D MMMM YYYY’, and then formats the parsed date using the compiled format function, finally logging the result to the console.
Output:

Remember, for consistent formatting of multiple dates using the same format string, it’s more efficient to use date.compile(). However, if you’re formatting a single date, use date.format() directly is simpler and adequate.
4. locale([locale])
The locale([locale]) method does two things. First, it helps you find out what language setting is currently being used. Second, it lets you change from one language setting to another. This is handy because it gives you the flexibility to show dates and times in different languages, making sure your app works well for users around the world.
Syntax:
date.locale([locale])
Parameters: This function can be used without a parameter to get information about the current locale. Alternatively, you can provide a locale code as a parameter to set a specific locale.
Example:
const date = require('date-and-time')
const myplace = date.locale();
console.log("The language code of the current locale :- " + myplace)
This script retrieves the current locale and then logs the current locale to the console in the form of language code.
Output:

5. isSameDay(date1, date2)
isSameDay(date1, date2) is a function used to determine if two dates represent the same day, ignoring the time component.
Syntax:
date.isSameDay(dateA, dateB)
Parameters: This function takes two parameters, dateA and dateB, representing Date objects.
Example:
const date = require('date-and-time');
const date1 = new Date(2024, 0, 25, 0);
const date2 = new Date(2024, 0, 25, 23, 59);
const date3 = new Date(2024, 0, 16, 23, 59);
const isSameDay1and2 = date.isSameDay(date1, date2);
const isSameDay1and3 = date.isSameDay(date1, date3);
console.log('Are date1 and date2 the same day?', isSameDay1and2);
console.log('Are date1 and date3 the same day?', isSameDay1and3);
This program, utilizing the ‘date-and-time’ package, checks if two dates share the same day (ignoring the time). The results are then logged to the console.
Output:
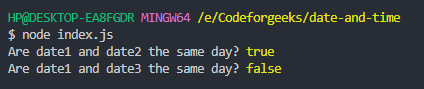
6. add()
The add() function adds additional days, months, hours, years, seconds, and minutes to the current date and time.
Below is a list of all date.add() functions along with their syntax, parameters, and purposes:
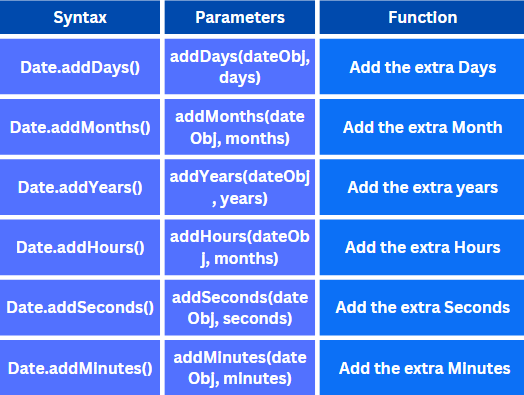
Example:
const date = require('date-and-time')
const now = new Date();
console.log("Original Date and time :- " + now)
const UpdateDAy = date.addDays(now,10);
console.log("Date and time with updated Days :- " + UpdateDAy)
const UpdateMon = date.addMonths(now,6);
console.log("Date and time with updated Months :- " + UpdateMon)
const UpdateYr = date.addYears(now,2);
console.log("Date and time with updated Years :- " + UpdateYr)
const UpdateHr = date.addHours(now,5);
console.log("Date and time with updated Hours :- " + UpdateHr)
const UpdateSec = date.addSeconds(now,80);
console.log("Date and time with updated Seconds :- " + UpdateSec)
const UpdateMin = date.addMinutes(now,30);
console.log("Date and time with updated minutes :- " + UpdateMin)
The provided code creates a current date and time (now), then demonstrates how to add and update days, months, years, hours, seconds, and minutes to the original date and time, printing each result to the console.
Output:
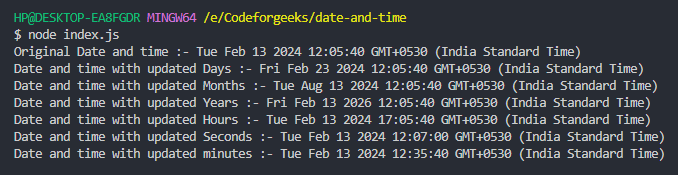
7. subtract(date1, date2)
The subtract(date1, date2) function is employed to find the difference between two date objects, making it valuable for calculating the time span between two dates.
Syntax:
date.subtract(dateA, dateB)
Parameters: This function takes two parameters, dateA (the first date object) and dateB (the second date object), for subtraction.
Example:
const date = require('date-and-time')
const ExamStart = new Date(2024,2,17);
const ExamEnd = new Date(2024,2,27);
const gap = date.subtract(ExamEnd,ExamStart);
// Display the result
console.log("The duration of Exams is "
+ gap.toDays()+" Days")
The provided code calculates the duration between two exam dates (ExamStart and ExamEnd) in days using the date.subtract() method. The result is then displayed in the console as the duration of exams in days.
Output:

Conclusion
To sum it up, the ‘date-and-time’ library in Node.js is a great tool for managing dates and times in JavaScript projects. It’s not too big, but it can do a lot! You can add extra features through plugins, and use it in different languages, and it works smoothly with TypeScript.
For developers, exploring its features, installing it, and understanding how to use it with examples can liberate its full potential. Whether you are figuring out the time difference, displaying dates in a specific way, or comparing them, ‘date-and-time’ is a useful library for developers to make working with dates and times much easier.
Want to know more stuff about Date and Time, we have got more, consider reading – Get the Current Timestamp in NodeJS – Multiple Easy Methods and JavaScript Date Objects (with a Real-Time Clock Application)
Reference
https://www.npmjs.com/package/date-and-time