JavaScript has a Date object that provides information regarding the date and time. This information can be used for different purposes such as getting the current time, comparing dates, formatting dates, reminders, etc.
JavaScript Date objects represent a specific point in time and do not depend on the platform they are being used on. The date and time returns from this object can be stored to maintain a record such as when a user posts an article, when a message is seen, etc.
This tutorial will provide a comprehensive guide to understanding JavaScript Date Objects.
Also Read: JavaScript null and undefined
Creating Date Object in JavaScript
The date object in JavaScript can be created using four ways.
- new Date()
- new Date(milliseconds)
- new Date(Date string)
- new Date(year, month, day, hours, minutes, seconds, milliseconds)
Let’s see them one by one.
new Date()
A date object created using the new Date() constructor will contain the current date and time.
Example:
let date = new Date();
console.log(date);
Output:
2023-01-26T06:00:19.567Z
new Date(milliseconds)
A date object created using this method contains the date and time specifying the number of milliseconds that have passed since the zero time, i.e., January 1, 1970, 00:00:00 UTC.
Example:
let date = new Date(100000000000);
console.log(date);
Output:
1973-03-03T09:46:40.000Z
new Date(date string)
A date object created using this method contains the date and time according to the date string passed as an argument.
Example:
let date = new Date("2023-01-26");
console.log(date);
Output:
2023-01-26T00:00:00.000Z
new Date(year, month, day, hours, minutes, seconds, milliseconds)
This will create an object from the specific date and time.
Example:
let date = new Date(2023, 1, 27, 4, 12, 11, 0);
console.log(date);
Output:
2023-02-26T22:42:11.000Z
JavaScript Date Methods
There are multiple methods that can be used with the Date object to provide different functionalities, let’s see some of them which are frequently used.
getHours() Method
This method returns the hour in the range of 0 to 23, based on the current time.
date = new Date();
date = date.getHours(); // returns the hour (from 0-23)
getMinutes() Method
This method returns the minutes in the range of 0 to 59, based on the current time.
date = new Date();
date = date.getMinutes(); // returns the minutes (from 0-59)
getSecond() Method
This method returns the seconds, based on the current time.
date = new Date();
date = date.getSeconds(); // returns the seconds (from 0-59)
getDay() Method
This method returns the day of the week in the range of 0 to 6. “0” denote the first day of the week and “6” represent the last day.
date = new Date();
date = date.getDay(); // returns the day of the week (from 0-6)
getDate() Method
This method returns the current date.
date = new Date();
date = date.getDate(); // returns the day of the month (from 1-31)
getMonth() Method
This method returns the current month.
date = new Date();
date = date.getMonth(); // returns the month (from 0-11)
getFullYear() Method
Gets the year according to local time
date = new Date();
date = date.getFullYear(); // returns the year
Real-Time Clock Application in JavaScript
Let’s create a real-time clock application in JavaScript to demonstrate the use of the Date object.
Project Structure: The project structure is simple, we have to create two files, index.html for writing the HTML and index.js for writing JavaScript code.
index.html
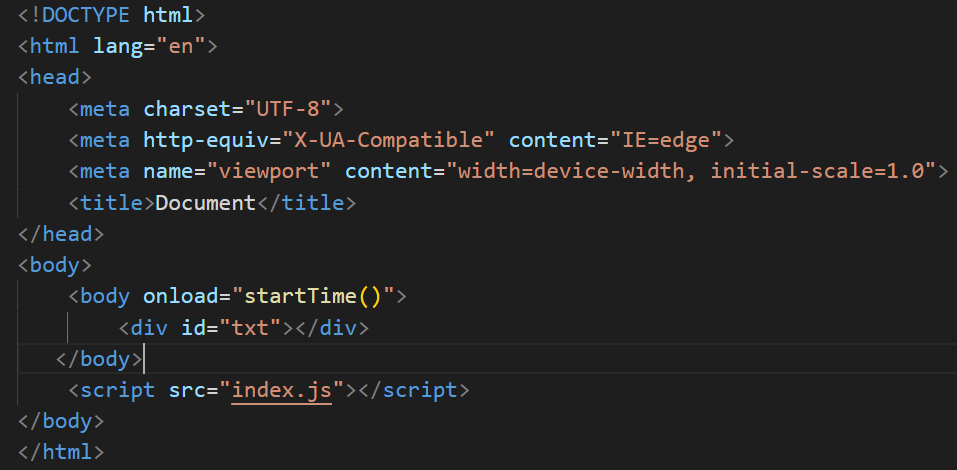
Here we have created a simple HTML file, that contains the “onload” attribute in the body tag, and contain a method “startTime()” which we will create using javascript to show the time, this method triggers after the website gets completed loaded in the browser. Then inside the body tag, we have created another div having an Id ”txt” which will use to display the time. Let’s now see the JavaScript code for creating the “startTime()” function.
index.js
function startTime() {
var today = new Date();
var h = today.getHours();
var m = today.getMinutes();
var s = today.getSeconds();
document.getElementById('txt').innerHTML =
h + ":" + m + ":" + s;
setTimeout(startTime, 500);
}
Here we first started with creating an object using “new date()” which will return the current date and time, then we reformatted it to get hours, minutes and seconds separately, then combined them and display them to the div having Id ‘txt’ which we have created in the previous step, and then use “setTimeout()” function to reload the function again and again, so that it will shows every second property.
We have already covered the “setTimeout()” function in another tutorial Node.js Timers Module: setTimeout, setInterval, setImmediate, if you want to read it.
Output:
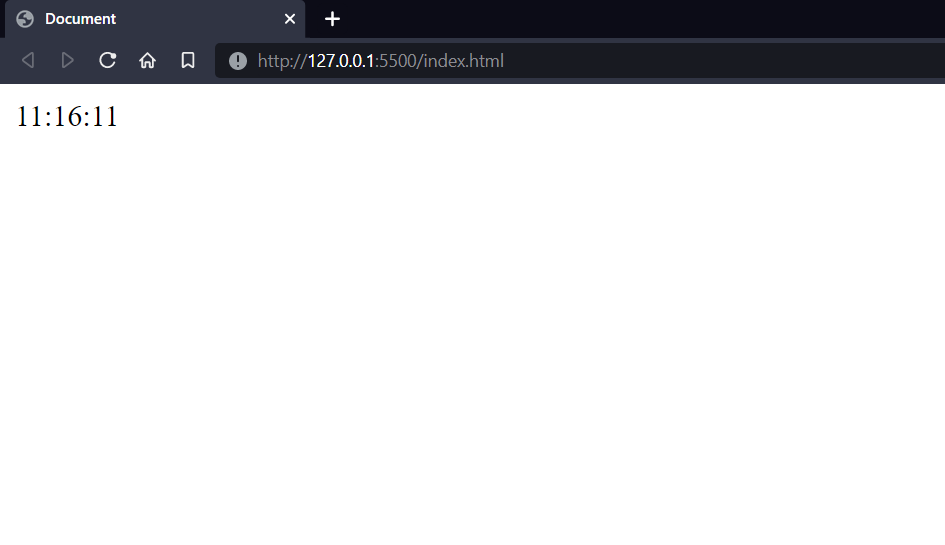
Summary
JavaScript has a Date object used to get the date and time, getting the date and time is the most essential part of developing any application as it is required to store time in very steps, such as for a social media website, when a message is sent, when receive, when user post sometime, which somebody comments, etc all of this records are super important. Hope this tutorial helps you to understand the Date object in JavaScript.
Reference
https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Date
https://stackoverflow.com/questions/50438363/show-real-time-clock-on-html-using-javascript