Syntax error ‘cannot use import statement outside a module’ is one of the very common errors occurring when you work with Node.js. Did you know that JavaScript uses two types of modules: one is the ES or ES6 module, and the other is the CommonJS module? The above-mentioned error occurs when we try to use the ES6 syntax of import and export in a CommonJS environment. In this article, let’s discuss the two modules of JavaScript, their syntax of import or export, and possible solutions for the given error.
JavaScript Module Types
To better understand the error, let’s first discuss modules in JavaScript. A module is a self-contained unit of code that can be imported and utilized in various parts of the application. Modules help developers organize their code into smaller, reusable components, making it easier to manage and maintain the codebase.
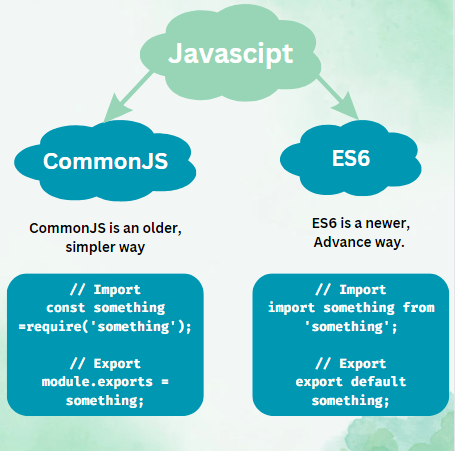
1. ES6 Module
ES6 Modules, also known as ECMAScript 2015, introduced a new way to organize and structure JavaScript code. This was done by providing a native module system. If you are using Node.js, you can use ES6 modules in two ways. First, you can use the .mjs extension for module files. Alternatively, you can set “type”: “module” in your package.json file.
Let’s make it clearer with an example where we create a project to find the cube of a number. This way, we can see the syntax of both import and export. We will create the logic of the function in another file, export it, and then use it in another file after importing.
Firstly, we will create the cube.mjs file to store the logic of the cube function:
// cube.mjs
export const calculateCube = (number) => {
return number * number * number;
};
Then, we will create the app.mjs file to use the logic and print the answer:
// app.mjs
import { calculateCube } from './cube.mjs';
const result = calculateCube(3);
console.log(`The Cube of 3 is: ${result}`);
If you are using a Node.js environment that supports ES6 modules, you can run the ES6 example using the “–experimental-modules” flag.
node --experimental-modules app.mjs

2. CommonJS Module
CommonJS is a module system used in JavaScript that was at first designed for server-side development using Node.js. It follows a synchronous module format and uses the require() function to import modules and the module.exports to export values from a module.
Just like we have created an example to understand the ES6 Module, similarly we will do for the CommonJS module.
Let’s create the cube.js file with the following code:
// cube.js
const calculateCube = (number) => {
return number * number * number;
};
module.exports = calculateCube;
And then the app.js file with the following code:
// app.js
const calculateCube = require('./cube');
const result = calculateCube(3);
console.log(`The Cube of 3 is: ${result}`);
We will run the given code using:
node app.js

Why ‘Cannot use import statement outside a module’ Error Occurs?
Now that we know the two different modules under JavaScript, it would be easy for us to understand why the ‘Cannot use import statement outside a module’ error occurs.
Some of the reasons why this error occurs are listed below:
- When building a web application with ES6 modules, it’s crucial to ensure that the server is configured to provide the correct MIME type for the module files. Failure to do so can cause the browser to be unable to recognize the file as a module, which will result in errors.
- Using ES6 import in a CommonJS environment like Node.js can cause an error because the system doesn’t recognize it.
- One possible cause of the error is using an incorrect file extension for ES6 modules. ES6 modules should have a .mjs extension or the .js extension with the “type”: “module” setting in the package.json file.
Steps to Fix This Syntax Error
To resolve the ‘Cannot use import statement outside a module’ error, we will use three ways but first, try to understand it using a real-life example.
We will create a Node.js project with an index.js file and a package.json file using:
npm init
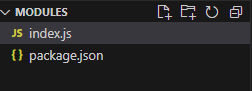
And add the following code to the index.js file:
import open from 'open';
open('https://codeforgeek.com/')
.then(() => {
console.log('File opened successfully!');
})
.catch((error) => {
console.error('Error opening file:', error.message);
});
This code uses the ‘open‘ library to open a website, and if the site is opened, it logs a success message to the console.
To use ‘open,’ you need to first install it:
npm install open
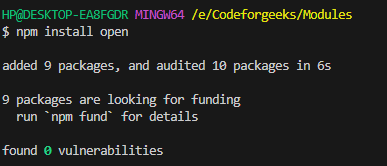
Then we will try to run the given project:
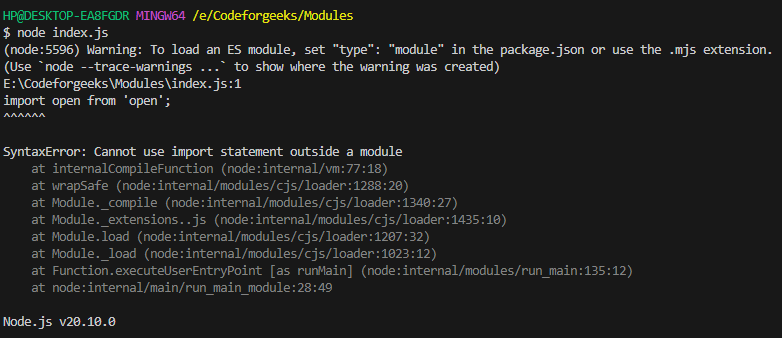
The error has been displayed because we tried to run a project with ES6 modules. This is because Node.js uses CommonJS modules by default, so any JavaScript files you write are treated as CommonJS files by default. If you carefully look at the code inside the index.js file, you’ll see that we used the ‘import’ keyword, which is not used in the CommonJS module. That is the reason it throws the error ‘SyntaxError: Cannot use import statement outside a module’. Let’s try to fix it using the following three ways.
1. Change the File Extension
If we change the file name from ‘index.js‘ to ‘index.mjs,’ your system will treat it as an ES6 module and will not throw any error.
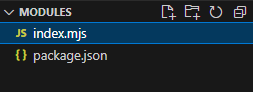

2. Change the “type” Section in the package.json
If you look under the “type” section in the package.json:

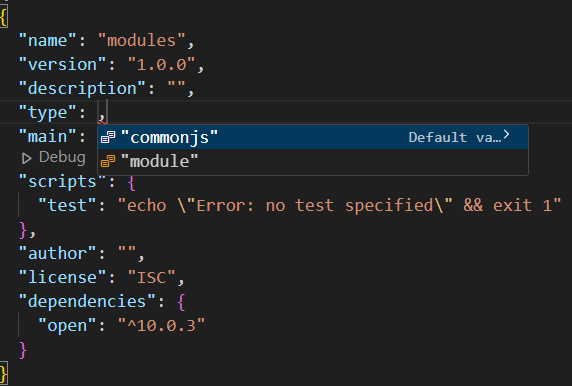
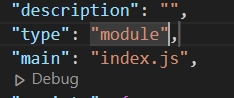
We have changed the type section to “type”: “module”.
Now your project will be treated as ES6 and no errors will be thrown:

3. Convert into CommonJS Syntax
Another solution to this problem can be to change the syntax from the ES6 to CommonJS.
Here we have changed the import syntax with require() method:
const opn = require('opn');
opn('https://codeforgeek.com/')
.then(() => {
console.log('File opened successfully!');
})
.catch((error) => {
console.error('Error opening file:', error.message);
});
Also, it is very important to know that the library you are using is compatible with the CommonJS module. Here, we have used ‘opn‘ instead of ‘open‘ because ‘open‘ does not work well with CommonJS. ‘opn‘ functions similarly to ‘open‘ and opens the given website.
If you change the syntax to CommonJS, it is necessary to make the corresponding changes in the ‘package.json’ file by selecting ‘commonjs’ for the ‘type’ section.
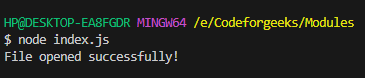
Conclusion
In this article, we explored a common error in Node.js — ‘SyntaxError: Cannot use import statement outside a module.’ We learned that JavaScript has two types of modules: ES6 and CommonJS. The error occurs when trying to use ES6 syntax in a CommonJS environment. We identified several reasons behind the error, which included incorrect MIME types, using ES6 import in a CommonJS environment, and incorrect file extensions.
To fix the error, we considered three approaches: changing the file extension to “.mjs,” modifying the “type” section in “package.json” to “module,” or converting the syntax to CommonJS.
If you enjoyed reading this one, check out more of our error-fixing articles –
- Resolving Cannot find module ‘node:fs’ Error: An Easy Guide
- Resolving ‘error /node_modules/node-sass: Command failed’ Error in Node.js