CPU usage with Node.js? Is it possible? In this article, we will discuss four such ways in which you can get to know the status of CPU usage very easily. It is very vital to understand how your application utilizes the CPU and how much memory it consumes. We do so to know what to do to optimize its performance. By the end of this guide, you will have a clear understanding of how to implement these straightforward methods to monitor CPU usage in your Node.js applications.
Understanding CPU Usage
As we know the CPU is an important component of a computer, which has the role of performing calculations and carrying out instructions. Thus it is also important that we perform an inspection or monitor its usage which is essential for optimizing the overall performance. In Node.js, CPU usage refers to the CPU resources consumed by an application during execution.
The CPU usage is usually expressed as a percentage, which shows how much time the CPU is actively processing compared to the total time. The calculation is based on the amount of time spent in various states, such as user, system, and idle, over a specific period of time.
Monitoring CPU usage in Node.js involves tracking the percentage of CPU resources that your application uses over time. This data helps find performance issues, improve code efficiency, and optimize your application’s use of system resources.
Developers need to have a good understanding of CPU usage as it helps them identify which parts of their code are causing high computational load or inefficiencies. This metric is crucial for optimizing performance and ensuring that the application runs smoothly without putting unnecessary strain on the system.
The easiest four ways to know about your CPU usage are discussed below.
Getting CPU Usage Using Node.js OS Module
The os module in Node.js contains a set of operating system-related utility methods. It allows you to access information about the operating system, such as CPU architecture, available memory, and platform-specific newline characters.
If you wish to know more about this module and its methods do follow – NodeJS OS Module: A Complete Guide
To know about the usage of the CPU, first create a JavaScript file and add the following code to it.
Example:
const os = require('os');
// Get CPU information
const cpus = os.cpus();
console.log('CPU Information:', cpus);
// Get average CPU usage over all cores
const avgCPUUsage = os.loadavg();
console.log('Average CPU Usage (1 min):', avgCPUUsage[0]);
When you save and run the given file with Node.js, you should get the following result:
node cpu.js
Output:
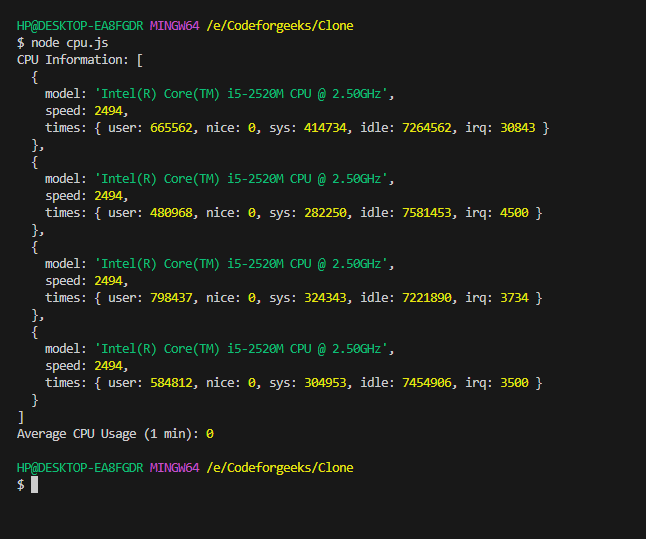
Explanation of the code:
- The first line imports the built-in OS module so that we can use it.
- The os.cpus() function returns an array of objects containing information about the CPU cores. This includes their model, speed, and usage times for different activities such as user, system, idle, and irq. This information is then stored in the cpus variable and printed into the console.
- The method os.loadavg() provides an array that shows the system load average over the last 1, 5, and 15 minutes. The following example demonstrates the average CPU usage over the last 1 minute.
Getting CPU Usage Using Node.js process.cpuUsage() Method
The process object in Node.js provides information about the current Node.js process and allows you to interact with it. When we add .cpuUsage() with it, it provides us with information related to the CPU usage. It helps us to obtain an object representing the user and system CPU usage of the current Node.js process.
Example:
const cpuUsage = process.cpuUsage();
console.log('CPU Usage:', cpuUsage);
Run the file with Node.js.
Output:
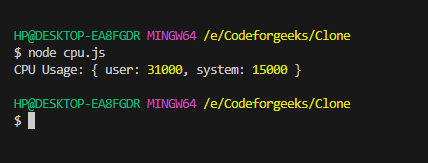
Explanation of the code:
- To get CPU usage information, you can use the process.cpuUsage() method. It returns an object with two properties: “user” and “system“. “user” tells you the CPU time spent in user mode (in microseconds), while “system” tells you the CPU time spent in system mode (in microseconds).
- It is then stored in the cpuUsage and logged into the console.
Getting CPU Usage Using NPM node-os-utils Library
node-os-utils is a Node.js library that simplifies obtaining system metrics like CPU, memory, and disk usage. It helps developers monitor and optimize their applications for better performance. It has various features which let you see the status of your CPU easily.
Before using the ‘node-os-utils’ library, you need to install it first. To install the given library run the following command:
npm install node-os-utils
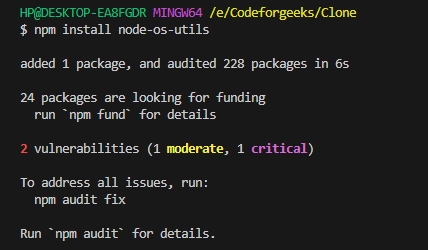
Once the library is installed, we will create our Node.js file.
Example:
const osu = require('node-os-utils');
async function getCPUUsage() {
try {
const info = await osu.cpu.usage();
console.log('CPU Usage:', info);
} catch (error) {
console.error('Error getting CPU usage:', error);
}
}
getCPUUsage();
Save and run the file with Node.js.
Output:

Explanation of the code:
- The first line imports the node-os-utils library, to use it further.
- An asynchronous function named getCPUUsage is declared.
- We use a try block to handle errors and declare a constant variable ‘info’ to store CPU usage data. Then we asynchronously call the ‘osu.cpu.usage()‘ method to get CPU usage information.
- The code `console.log(‘CPU Usage:’, info)` logs CPU usage info to the console.
- Any errors during execution are caught by `catch (error)`, and `console.error(‘Error getting CPU usage:’, error)` logs the error to the console.
Getting CPU Usage Using NPM pidusage Library
Node.js developers can use the ‘pidusage‘ library to get information about how much CPU and memory a specific process is using. The library offers simple functions to monitor individual processes, which can help with analyzing performance and optimizing Node.js applications. The “cpu” property in the “stats” object returned by pidusage represents the percentage of CPU usage by the process.
To use the given library, we need to install it first. Run the following command to install the “pidusage” library.
npm install pidusage
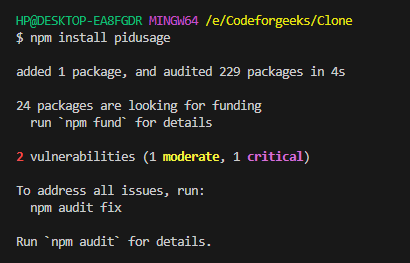
Now that the library is installed, let’s create our Node.js file.
Example:
const pidusage = require('pidusage');
const pid = process.pid;
pidusage(pid, (err, stats) => {
if (err) {
console.error('Error getting CPU usage:', err);
} else {
console.log('CPU Usage:', stats.cpu);
}
});
Now save and run this file with Node.js.
Output:
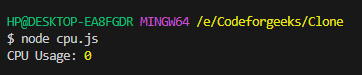
Explanation of the code:
- The first line imports the ‘pidusage’ library, making it ready for use.
- The process ID (PID) of the current Node.js is retrieved using process.pid.
- The pidusage function is called, and the PID along with a callback function is passed as arguments.
- The CPU and memory usage information for the specified process is requested through pidusage(pid, callback).
- The callback function is executed when the CPU usage information is available.
- If an error occurs during the process, it is logged to the console.
- If successful, CPU usage information is logged to the console under stats.cpu.
Summary
In this article, we have discussed four simple ways to monitor CPU usage in Node.js, which can help improve application optimization. It is essential to understand CPU usage to identify performance issues and improve the efficiency of your code.
We have explored methods using the ‘os’ module, the ‘process’ object, the ‘node-os-utils’ library, and the ‘pidusage’ library. Each technique provides insights into CPU usage, allowing developers to enhance their application’s performance. By the end of this article, you will have a clear understanding of how to implement these straightforward techniques to keep your Node.js applications running smoothly.
If you like this one then you must definitely like – How to Remove node_modules in Node.js: 3 Easy Ways
Reference
https://stackoverflow.com/questions/36816181/get-view-memory-cpu-usage-via-nodejs