Removing node_modules from a project file when not needed is like clearing your mind. Even when we are doing many things at once, our brain needs to get rid of unnecessary thoughts to help us focus better. Similarly, in the case of Node.js, the ‘node_modules’ folders are large. They take up a lot of space on our computers, and the problem is that we often forget to delete them after we finish our work. So, we end up with many of these folders on our computer, and it uses up a bunch of our storage. If we keep them when we don’t need to, it can impact the efficiency of the program. In this article, let us explore how we cut down these hefty modules.
Node.js node_modules Folder
In Node.js projects, “node_modules” is like a special folder. It keeps all the things your project needs to work. Node.js uses something called a package manager, like npm or Yarn, to handle these things. When you start a Node.js project or add new stuff to it, the package manager grabs and saves those things in the “node_modules” folder.
Here’s a simple example of what the “node_modules” directory structure might look like:
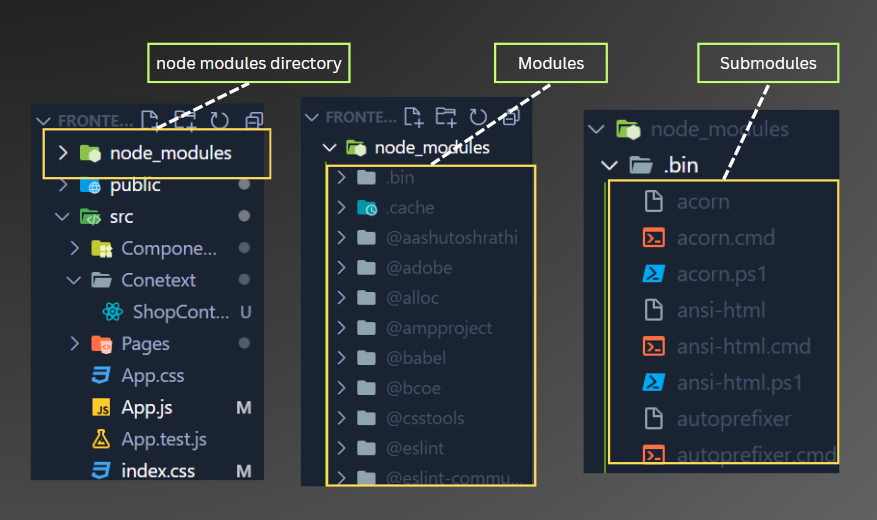
The external modules that are specified in the project’s “package.json” file, package manager installs them in the “node_modules” directory.
Modules in node_modules Folder
A module is a bunch of code encapsulated into a unit that has a specific functionality. Node.js has a module system that allows you to create, import, and use modules in your applications.
You may create your own module by defining functions, variables, or objects in a separate file. To use the created module in another file, you can use the require function in Node.js.
const myMod = require('./createdModule');
Node.js also provides a set of built-in core modules that can be used without installation. These are also imported using the require function without specifying a path, as Node.js knows where to find them.
const fs = require('fs');
Also, you may use some third-party modules by installing them via npm. These are also downloaded into the node_modules directory.
const axios = require('axios');
Submodules in node_modules Folder
The essential information inside a module is further divided into sub-files having data stored about the given module classified according to their type of data. A simple example is about the storage of dependencies and version details.
Why We Need to Remove node_modules Folder
Removing the “node_modules” folder in your project is just like cleaning up extra stuff in your project. It is necessary to do so as it helps us to omit all the unnecessary dependencies. It reduces the project size, so it’s easier to share or put on the internet. Clearing these heavy files can increase the loading and downloading speeds. We may remove such modules that have large dependencies as they tend to fill hard disk space on our filesystem. We usually do this only when we want to share or publish your project, not every day while we’re working on it.
Now, that you know the need to remove node_modules, let’s explore some ways to do so.
Different Ways to Remove node_modules in Node.js
1. Using Command Line
To delete the node_modules using some prompts in the command line, you need to first open your terminal and bash, then just run the below command. Make sure you specify the directory in which the node_modules file you wish to delete is present.
rm -rf "node_modules"
rm stands for remove and rf means without any recursion, so the above command collectively means that it would remove the given file or, we must say remove node_modules without any recursive confirmation.
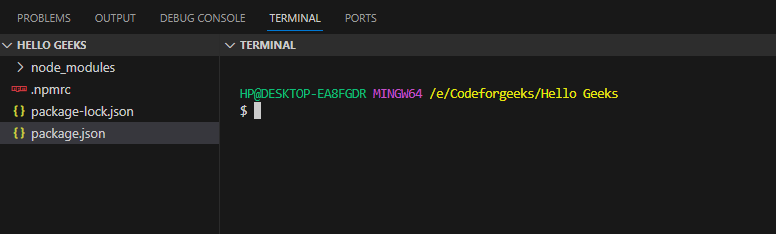
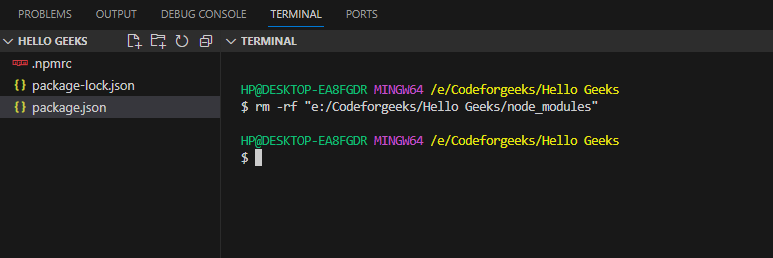
2. Using NPX
NPX stands for Node Package Execute helps you to use npm commands without actually installing the same. It is one of the fastest and fun ways to remove node_modules. Just run the following commands:
npx npkill
Once you run the above, you will get to see a cool display like this:
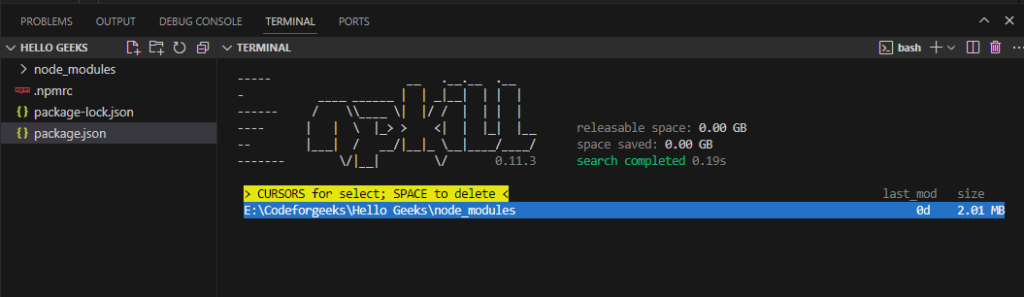
As you see the highlighted yellow portion, it clearly says to select the file using the cursor and press the space key to delete the same.
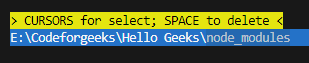
Now as soon as you hit the space key, the “node_modules” vanishes.
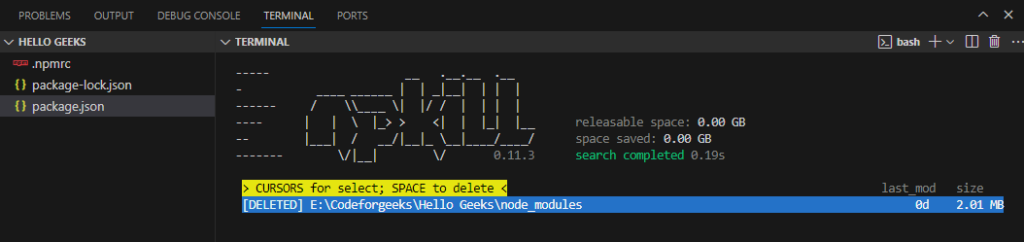
3. Using NPM
The “rimraf” in npm helps to remove files and in this case, it helps to remove node_modules. To use the “rimraf” package of npm, you need to first install it globally.
npm install -g rimraf
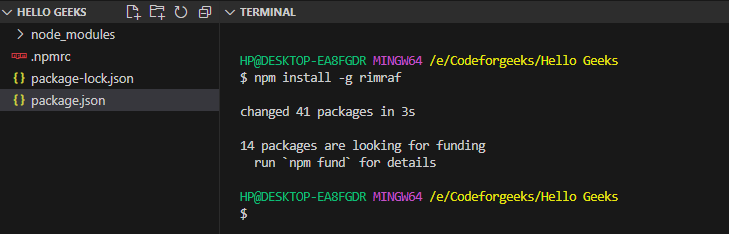
Now that we have it installed, let’s use it to remove “node_modules”.
rimraf node_modules
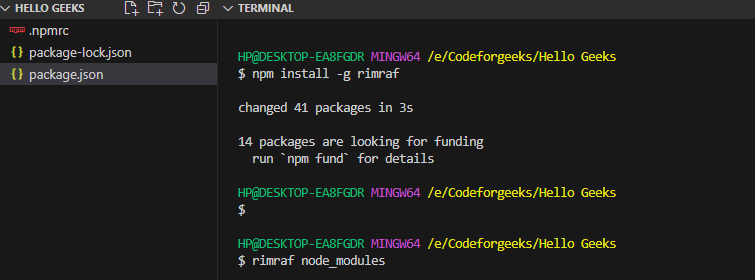
Conclusion
Okay, so that’s the end of this article. We hope this information has helped you build a clear understanding of why we should remove node modules and what the ways are to do so. The fastest way is to use npx. Additionally, if you don’t prefer bash or commands, you can directly remove the ‘node_modules’ folder using mouse clicks. However, that’s not considered professional or developer-friendly. Anyway, it’s your choice how to proceed.
Continue Reading: