jQuery is one of the most popular JavaScript libraries of its time, it becomes so much more popular because of the extended functionality that it provided to web developers. jQuery is a JavaScript library that makes it easy to handle events, asynchronous JavaScript requests, Document Object Module manipulation, and animation. So in this post, we are going to discuss some of the most common and most important jQuery methods or you can say functions that are available in jQuery and provide some magical functionality to our code.
Steps to Install jQuery
To install jQuery in your project just add the following line of code inside your HTML page head tag.
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.5.1/jquery.min.js"></script>
If we go to the given link in the above HTML tag then we can easily get the complete source code of jQuery. Hence in simple words, importing that large set of code in our file with a single line.
jQuery load() Method
This function comes into action when you want to load data from an external source or you can say any file and show that data in any object that is available in the DOM tree. This definition is quite complex to understand but let’s make it simple. Suppose you have made a skeleton of the to-do list on your HTML page. Then you want to search for data from any file where your to-do list is stored. Then we can use the load function from jQuery to patch that data and fit it into our HTML code dynamically.
Example:
<!DOCTYPE html>
<html>
<head>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.5.1/jquery.min.js"></script>
<script>
$(document).ready(function () {
$("button").click(function () {
$("#changeText").load("demo.txt", function (responseTxt, statusTxt, err) {
if (statusTxt == "success") alert("The content was successfully loaded!");
if (statusTxt == "error") alert("Error: " + err.status + ": " + err.statusText);
});
});
});
</script>
</head>
<body>
<!-- Body Starts Here -->
<div id="changeText">Text to be changed.</div>
<button>Click me!</button>
</body>
</html>
Explanation:
- We have created a div tag that has an ID of “changeText” and something is written inside that div tag.
- Then we are initializing the complete document Global object with the ready function that is available in jQuery.
- After that, we picked up the button and added are click event to it, and passed another function inside it.
- We are adding functions inside functions because this is how jQuery works.
- Then in the last step, we picked up the “changeText” ID using # and used the load method on it.
- The first argument that we passed is the file is the location from where we want to load data and then a call back function which has three perimeters response, status, and error.
- Callback function is a function passed as an argument to another function and does its work just like regular functions but is called on behalf of another function. In our case, the callback function is used to get response text, status text, or any errors if they are encountered.
- You just need to handle errors because everything else will be done by jQuery.
Output:
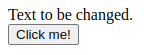
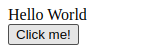
jQuery get() Method
Till now we were only dealing with local files that were present in our system. Now in case we want to get some data from an external server that is hosted on the internet then we can use the get() method. Below we have shown the complete implementation of the get method with explanation and output.
Example:
$(document).ready(function(){
$("button").click(function(){
$.get("https://jsonplaceholder.typicode.com/todos/1", function(data, status){
alert("Data: " + data + "\nStatus: " + status);
});
});
});
<!-- Body Starts Here -->
<button id="getRequest">Get Request Demo</button>
Explanation:
- In this case, everything is the same as of load method but the only difference that we see is in the argument that we passed.
- The first argument that we passed is the URL from where we want to get data.
- The second argument is a function that contains the received data and status.
- Then we simply used the pre-build alert function to check whether data is coming or not. We got an object because our API is returning us an object.
Output:
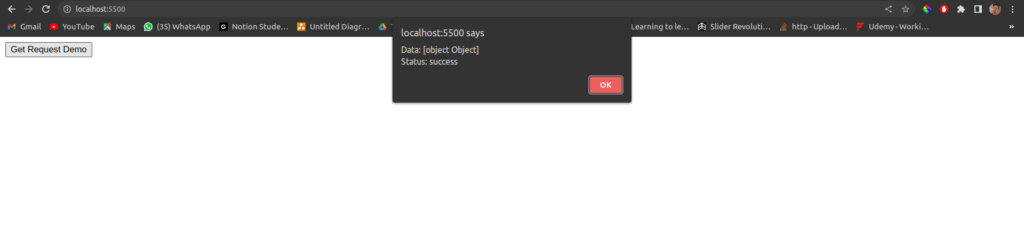
jQuery post() Method
We had already learned how we can get data from the server using jQuery. Now it’s time to learn how we can send data to the server using the post method.
Example:
$(document).ready(function(){
$("button").click(function(){
$.post("https://jsonplaceholder.typicode.com/posts",
{ title: "Donald Duck" },
function(data,status){
alert("Data: " + data.title + "\nStatus: " + status);
});
});
});
<!-- Body Starts Here -->
<button id="postRequest">Post Request Demo</button>
Explanation:
- In the above code, we used the post method and the first thing that we passed is the API URL on which we want to post our data.
- The next argument is of parameters that your API will accept. In our case, the API is for posting a new post in a database and there is a parameter of title in that data so we are adding the title as “Donald Duck”.
- You can go to the API URL from your browser to get a more brief knowledge of what we are posting and why we are writing the title.
- Else everything will be the same and in the alert function, we are printing the title of data that we get back from this request.
Output:
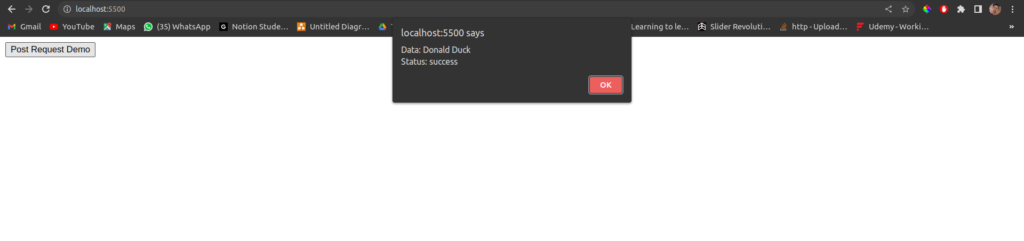
Also Read: Online Rich Text editor using jQuery
Summary
The three methods that we learned in the tutorial were the most important and mostly used methods when you are working with jQuery. We hope that this post was helpful and that now you can use these methods comfortably.