The word “Java” in “JavaScript” proves no direct connection between these two languages, they do have similar names but are very different if seen purpose and syntax wise.
Java is an object-orientated programming language used to create software, programs and applications. On the other hand, JavaScript is a scripting language primarily used for building web applications.
How is JavaScript Different from Java?
Many people think that switching from Java to JavaScript is like going from playing the piano to the guitar — they expect it to be similar since both are musical instruments. But that’s not true. While both are used to make music, the skills and way of playing are very different. Similarly, Java and JavaScript are very different. Let’s start with some history.
1. History of Java and JavaScript
Java:
Java was initiated by James Gosling in 1991 and completed in 1995 at Sun Microsystems (later under Oracle). It was first named Greentalk, then Oak, and finally Java.
Java was first created to run on devices like TVs and set-top boxes. But it became popular when people started using it for programming on the internet. This led to Java being used widely all over the world. It has had many updates over time, adding new features and improvements, making it a top programming language.
JavaScript:
JavaScript was invented by Brendan Eich in 1995 and standardised as ECMA-262 in 1997.
Netscape transferred JavaScript to ECMA. That led to the creation of a committee—TC39—which would oversee further development.
Internet Explorer 4 was the first browser with support for JavaScript as a standard—that is, ES1.
A disagreement over ECMAScript 4 resulted in renaming it ES5, with plans for some time soon for a major future release to be ES6. Both ES5 (2009) and ES6 (2015) enjoyed a wide reach, with full browser support in 2013 and 2017, respectively. It was first named Mocha, then LiveScript, and finally Javascript.
2. Key Features of Java and JavaScript
Java:
- This language stands on four pillars of the OOPS concept.
- We use classes and objects in Java, which helps us write reusable and meaningful code.
- Java binds data with objects and helps with encapsulation.
- A property of one class can be inherited by another class to minimise code redundancy.
- Classes are also confined to access modifiers, which help us keep our data abstract and safe.
- It supports multithreading, which makes it more efficient in handling huge functions.
- Java is simple to write and easy to compile and debug, which makes it stand out from other languages.
- Among all these glittering features of Java, one of its drawbacks is that its performance is slow and it uses a huge amount of storage.
JavaScript:
- JavaScript has the power to convert a static website into a live, functioning application.
- It is a scripting language used for making animated websites using DOM.
- In JavaScript, functions behave like objects and have their own properties and methods. You can pass functions as arguments to other functions.
- It introduces interactions on webpages, like fetching data from a database, actions performed on click, and animations.
- This language not only shows up on the frontend but can also be integrated with the backend of the webpage.
- It runs on a browser; hence, it is a popular choice for making applications that run on a browser.
- JavaScript stands for its versatility, which means if you want to learn both the frontend and the backend, then you need to learn the syntax only once.
3. Execution Differences Between Java and JavaScript
Java:
Java works on a virtual machine (JVM). Programs are compiled only once and can run on any platform, which is why it is known as a platform-independent language. Let me make it more clear.
The source code gets compiled only once and then converted to byte code. Now, this byte code can be executed on any platform by the Java virtual machine.
JavaScript:
JavaScript is not a compiled but an interpreted language, it runs live on the browser. It executes with the help of a browser engine like the V8 engine for Chrome.
JavaScript can behave differently across browsers because each uses a different engine to interpret the code. It does not make full programs on its own, instead, it’s a part of making a web page and cannot function without HTML.
JavaScript on its own can be used for server-side programming with environments like Node.js.
4. Syntax Comparison Between Java and JavaScript
Java:
Java has a structured and complex syntax. It is very specific about spelling and also case-sensitive. But due to its organised signature, it’s easy to understand.
Here is how we display a Hello greeting in Java:
public class HelloWorld {
public static void main(String[] args) {
System.out.println("Hello and welcome to Codeforgeek!");
}
}

You have to write long code for short logic because we create classes and constructors in Java.
For example, if we had to make an object named Singer with the properties firstname, lastname, and song, we would write it like this:
class Singer {
String firstName;
String lastName;
String song;
public Singer(String firstName, String lastName, String song) {
this.firstName = firstName;
this.lastName = lastName;
this.song = song;
}
public String getFirstName() {
return firstName;
}
public String getLastName() {
return lastName;
}
public String getSong() {
return song;
}
}
public class Main {
public static void main(String[] args) {
Singer singer = new Singer("Charlie", "Puth", "Attention");
System.out.println("First Name: " + singer.getFirstName());
System.out.println("Last Name: " + singer.getLastName());
System.out.println("Song: " + singer.getSong());
}
}

JavaScript:
On the other hand, JavaScript has a simple and relaxed format for writing code. It provides us with ease and flexibility in writing syntax.
A Hello greeting in JavaScript will look like this:
console.log("Hello, World!");

Pretty simple, right? Now, if we want to rewrite the singer object code (which we previously wrote in Java) in JavaScript, we will do so in the following way:
let singer = {
firstName: "Charlie",
lastName: "Puth",
song: "Attention"
};
console.log(`First Name: ` + singer.firstName);
console.log(`Last Name: ` + singer.lastName);
console.log(`Song Name: ` + singer.song);
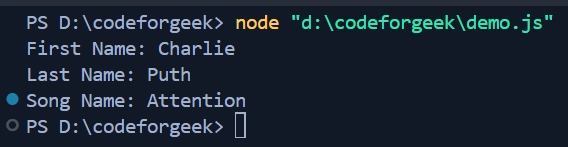
The code comparison will show you how much easier the syntax of Javascript is than Java.
5. Applications of Java and JavaScript
Java:
- It is used for many desktop applications, mobile applications, and games.
- It is also used for the backend building of a website, for example, Wikipedia, Google, and Netflix. Click here to learn more about which tech is best for the backend!
- Due to its security and multitasking, it’s used to create IOT applications and AI programs.
JavaScript:
- JavaScript empowers the developer to make the webpage dynamic and interactive. It allows for features like animations, form validation, and changing content on the fly without reloading the page.
- JavaScript can also be used for server-side programming with environments like Node.js that help in developing scalable and efficient web servers and backend services.
- Finally, using frameworks like React Native, and JavaScript enables cross-platform mobile applications that run on iOS and Android using the same codebase.
Java vs. JavaScript: Comparison Table
S.No. | Java | JavaScript |
1. | It is a stand-alone language that works on its own. | It is implemented with HTML. |
2. | This is very peculiar about syntax and has strict writing rules. | This has a relaxed writing style. |
3. | It is compiled before execution. | It is interpreted before execution. |
4. | A huge amount of memory is required. | A small memory is enough. |
5. | We use the .java extension to save the code file. | We use the .js extension to save the code file. |
6. | Static in nature. | Dynamic in nature. |
7. | Runs on any platform or browser using the Java virtual machine. | It runs not only in the browser but also on the server using Node.js. |
8. | It supports multithreading. | It is a single-threaded language and does not support multithreading. |
9. | Mainly used for backend | Used for frontend and backend both. |
10. | JDK (Java Development Kit) is required to run the Java code. | Any text editor or browser console can be used to run the code. |
Conclusion
Java vs. JavaScrpit fits perfectly in the category of “same but different”. Many tech rookies get confused at first when they discover these two due to the similarity in their names, but as we have seen, these two are far from being the same.
Java and Javascript are similar like Car and Carpet are similar but they are different in purpose, working, syntax and applications. But one good thing is that both of them are great boons for development. These two play a significant role in building applications that we use daily, hence, both are somewhat equally popular.
If you want to read more comparison guides like this, here’s the list:
- Node.js vs Python
- Node.js vs PHP
- Node.js vs GoLang
- Node.js vs .NET
- Node.js vs Vanilla JavaScript
- NodeJS vs AngularJS
- NodeJS vs Spring
Reference
https://stackoverflow.com/questions/245062/whats-the-difference-between-javascript-and-java