In this tutorial, we will learn about the JavaScript trim() method along with its four different variants, which are trimStart(), trimEnd(), trimLeft() and trimRight().
JavaScript trim methods are used to remove the whitespace from a string. By whitespace, I mean spaces, tabs, non-breaking spaces, line feed characters, and carriage return characters. These characters are important for formatting, but they are often unnecessary when we are processing strings. All these characters are considered whitespace and they can be eliminated using the trim methods. Let’s learn about this in detail!
Introduction to JavaScript trim() Method
Assume that you are the owner of a bookstore and you are maintaining a list of books in your database. You may want to organize them such that there are no extra spaces at the end or start of each book title.
This is where the JavaScript trim() function comes into the picture. This method is used to remove whitespace characters from the start and end of a string. The trim() function returns a new string where the spaces from the beginning and end of the string are removed, without even changing the original string.
Syntax:
string.trim()
The trim() method does not accept any parameters.
Example:
Let’s say we have a book title that contains whitespace in the beginning and end. To add its details to the database, we need to remove the space. To do so:
let text = " The Silent Patient ";
let trimmedText = text.trim();
console.log(trimmedText);
Output:

As we can see from the above output, the trim() method is called on the text string, which contains whitespace at the beginning and its end. This method removed the whitespace and the trimmed text is stored within the variable trimmedText.
Other Trim Methods
There can be a certain scenario where you want to remove whitespace from the beginning or end of a string. JavaScript provides custom methods for this:
- trimStart()
- trimEnd()
- trimLeft()
- trimRight()
Let’s understand these methods in detail!
JavaScript String trimStart() and trimLeft() Method
Assume a situation where you have a list of usernames, but some of them contain unnecessary whitespace characters at the beginning. So what to do now?
The trimStart() and trimLeft() can turn out as perfect for this situation. These methods in JavaScript are used to remove whitespace from the beginning of a string.
Syntax:
string.trimStart()
string.trimLeft()
Example:
Let’s clean up the usernames by removing the leading whitespace using the trimStart() and trimLeft() methods:
// List of usernames with leading whitespace
let usernames = [
" jai_kapoor",
" nitin_singh",
" priya_pandey",
"shubham_mishra"
];
function cleanUsernames(usernames) {
// Using trimStart()
let cleanedUsernamesStart = usernames.map(username => username.trimStart());
// Using trimLeft() for comparison
let cleanedUsernamesLeft = usernames.map(username => username.trimLeft());
return { cleanedUsernamesStart, cleanedUsernamesLeft };
}
// Clean up the usernames
let { cleanedUsernamesStart, cleanedUsernamesLeft } = cleanUsernames(usernames);
console.log("Cleaned Usernames using trimStart():");
cleanedUsernamesStart.forEach(username => console.log(username));
console.log("\nCleaned Usernames using trimLeft():");
cleanedUsernamesLeft.forEach(username => console.log(username));
In the above code, we have defined a function named cleanUsernames() that takes an array of usernames as input and uses both trimStart() and trimLeft() methods to remove the leading whitespace from each username.
Output:
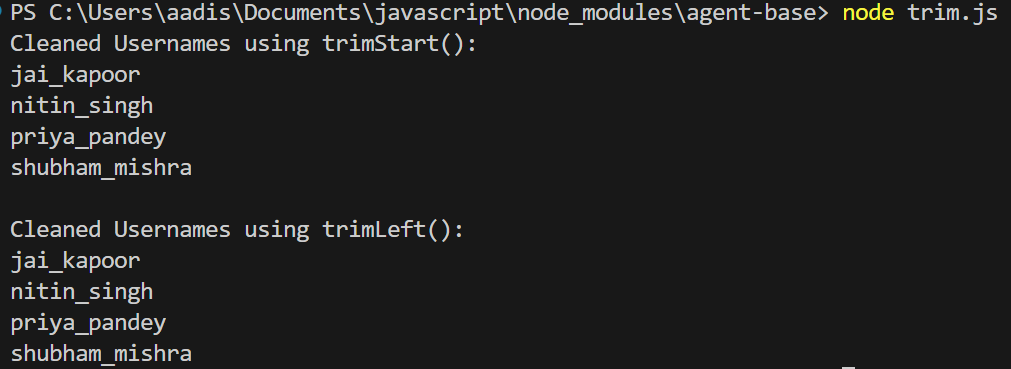
As you can see, both trimStart() and trimLeft() methods return the same results, by removing leading whitespace from each username in the array. This shows that they have similar functionality.
JavaScript String trimEnd() and trimRight() Method
Now let’s say you have a list of file extensions that may contain trailing whitespace and to save these files you need to clean these files’ extensions. How can we do this?
The trimEnd() and trimRight() methods in JavaScript have similar functionality, which means that they remove the trailing whitespace characters from the end of a string.
Syntax:
string.trimEnd()
string.trimRight()
Example:
Let’s clean up the file extensions by removing the trailing whitespace. To do this:
// List of file extensions with trailing whitespace
let fileExtensions = [
"jpg ",
"png ",
" gif",
"svg"
];
function cleanFileExtensions(fileExtensions) {
// Using trimEnd()
let cleanedExtensionsEnd = fileExtensions.map(ext => ext.trimEnd());
// Using trimRight() for comparison
let cleanedExtensionsRight = fileExtensions.map(ext => ext.trimRight());
return { cleanedExtensionsEnd, cleanedExtensionsRight };
}
// Clean up the file extensions
let { cleanedExtensionsEnd, cleanedExtensionsRight } = cleanFileExtensions(fileExtensions);
console.log("Cleaned File Extensions using trimEnd():");
cleanedExtensionsEnd.forEach(ext => console.log(ext));
console.log("\nCleaned File Extensions using trimRight():");
cleanedExtensionsRight.forEach(ext => console.log(ext));
In this example, we have defined a function named cleanFileExtensions() that takes an array of file extensions as input and uses both trimEnd() and trimRight() methods to remove the trailing whitespace from each extension.
Output:
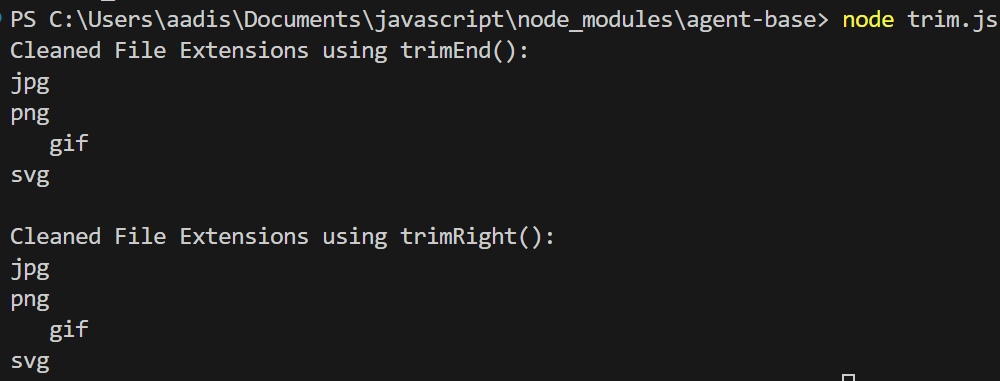
As you can see, both trimEnd() and trimRight() methods return similar results by removing trailing whitespace from the end of each file extension. This shows their equivalence in functionality.
Conclusion
In this tutorial, we have learned about the JavaScript trim() method with trimStart(), trimEnd(), trimLeft(), and trimRight() for removing whitespace from strings. These methods provide practical answers to string manipulation problems and make our code effective. It is better to make these methods inhabit so that you can utilize them and maintain clean and readable code.
Also Read:
- How to Split a String in Node.js Using JavaScript split() Function
- JavaScript setInterval() Method: Live Counter & Digital Clock Examples
Reference
https://stackoverflow.com/questions/498970/trim-string-in-javascript