JavaScript setInterval() method is used to continually run a function at every given time interval. This is best for cases where we have to repeat the execution of a function at some interval, like refreshing the server every 5 seconds or fetching data every second.
Unlike the setTimeout() method which only calls the specified function one time after the specified time, setInterval() executes a function repeatedly at a given time interval until the window is closed or the clearInterval() method is called.
Syntax & Parameters of setInterval()
The setInterval() is a global function so it can be called directly anywhere in a program using the below syntax:
setInterval(function, milliseconds, params);
Parameters:
- function – a function containing a block of code
- milliseconds – the time interval between the execution of the function
- params – optional parameters to pass to the function
Return:
This method returns the ID of the timer which is a numeric value and can be used later to cancel the timer by passing it as a parameter to the clearInterval() method.
How setInterval() Function Works in JavaScript
Since JS is single-threaded, its code is executed by a single thread and for activities like managing timing events, we have methods like setTimeout() and setInterval(). When you invoke these functions, the browser timer system typically operates independently from the main JavaScript execution thread.
Upon timer expiration, the associated callback function is not immediately placed on the execution stack. Instead, it’s added to a queue known as the “task queue” or “callback queue”. The JavaScript engine operates an event loop that continuously checks whether the execution stack is empty. If it is, the event loop moves tasks from the task queue to the execution stack, executing them one by one. Therefore, when a timer expires, the callback function is placed in the task queue and waits for the execution stack to empty before being processed by the event loop.
Example 1: Displaying a Text Message Every Second
Let’s see how we can display a text message every second using this method.
function greet() {
console.log('Hello world');
}
setInterval(greet, 1000);
In this example, the greet() function is set to invoke every 1000 milliseconds (1 second). As a result, the string ‘Hello world’ is logged to the console each second until the program ends or the window is closed.
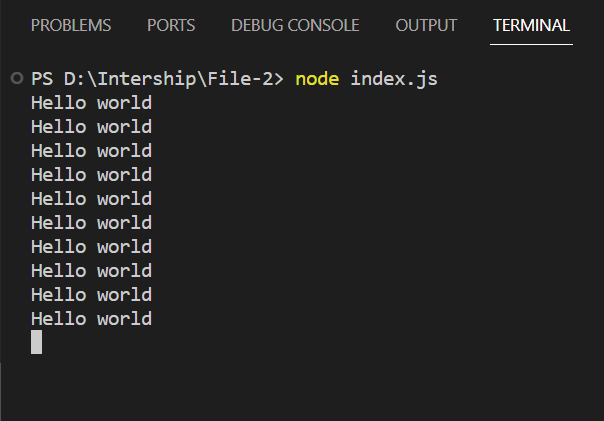
Example 2: Creating a Live Counter
Updating a live counter is a typical situation in web development where the visible value in the counter changes continuously with no input from the user.
Using setInterval() function is good for this objective. We can create a dynamic user experience by scheduling the periodic execution of code to update the counter value at set intervals.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Live Counter</title>
</head>
<body>
<div id="counter">0</div>
<script>
let count = 0;
const counterElement = document.getElementById('counter');
function updateCounter() {
count++;
counterElement.textContent = count;
}
// Update the counter every second
const intervalId = setInterval(updateCounter, 1000);
</script>
</body>
</html>
The updateCounter() method is used to update the text content of the HTML element having the id “counter” and display the current count by incrementing a counter variable (count). The setInterval() function makes this updating process happen in the delay of one second, creating a live counter that increments in value automatically.
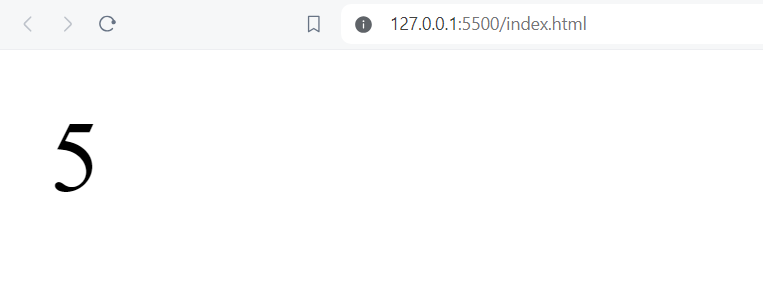
Example 3: Creating a Digital Clock
Digital clocks are crucial in a variety of digital platforms. For creating this, we can use the setInterval() method and update the clock per second.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Digital Clock</title>
</head>
<body>
<div id="clock"></div>
<script>
function updateClock() {
const clockElement = document.getElementById('clock');
const now = new Date();
const hours = now.getHours().toString().padStart(2, '0');
const minutes = now.getMinutes().toString().padStart(2, '0');
const seconds = now.getSeconds().toString().padStart(2, '0');
clockElement.textContent = `${hours}:${minutes}:${seconds}`;
}
updateClock();
const intervalId = setInterval(updateClock, 1000);
</script>
</body>
</html>
The updateClock() function gets the current time and formats it into hours, minutes, and seconds. It is invoked immediately upon page load to update the clock. Additionally, setInterval(updateClock, 1000) ensures that updateClock() is called every 1000 milliseconds (1 second), guaranteeing consistent clock updates. The time is displayed within a <div> element having the id ‘clock’.
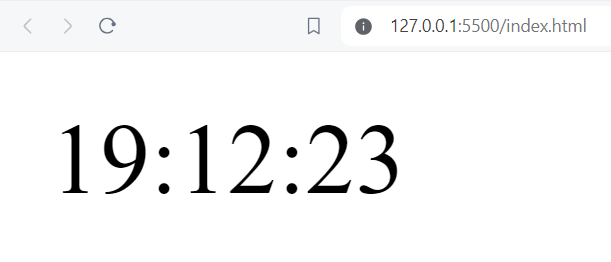
Clearing Intervals with clearInterval() Function
Another built-in JavaScript method called clearInterval() is used to end the interval that was set by setInterval(). It accepts a single parameter, the identifier or ID that the setInterval() method returned when it was first invoked. The function that is given in setInterval() is stopped from being executed again when clearInterval() is called, therefore ending the interval.
let count = 0;
function printCount() {
console.log('Count:', count);
count++;
if (count >= 5) {
clearInterval(intervalId);
console.log('Interval stopped after 5 counts');
}
}
const intervalId = setInterval(printCount, 1000);
Here the printCount() function logs the current value of the count to the console and increments it. Utilizing setInterval(), printCount() is called every 1000 milliseconds (1 second). Within printCount(), a check is performed to see if the count has reached 5. If this condition is met, clearInterval() is invoked to stop the interval, followed by logging a message.
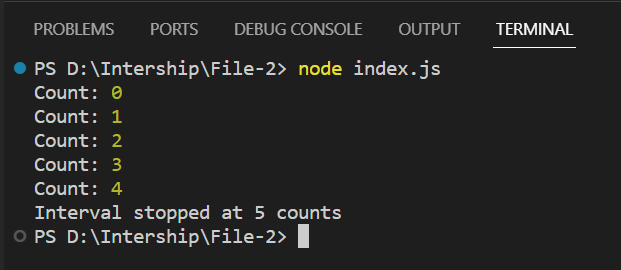
Application of setInterval() Method
- Animations: Using the setInterval() method, an element’s location, size, or colour may be changed regularly on a webpage.
- Real-time data updates: The setInterval() method may be used to routinely get data from a server or API and refresh the UI.
- Timer countdowns: A timer countdown that is shown on a webpage at regular intervals can be made smaller until it approaches 0 by using the setInterval() function.
- Games: You may move objects, check for collisions, and continuously update the game state by using the setInterval() method.
- Automatic slide shows: setInterval() may be used to generate automatic slide shows on a webpage by recurrently altering the displayed picture after a predefined interval.
Related Tutorials:
- Node.js Timers Module: setTimeout, clearTimeout, setInterval, clearInterval, setImmediate, clearImmediate
- Pause JavaScript Code Execution: Top 3 Methods With Examples
Conclusion
Using JavaScript setInterval() we can continually run a function or code block at predetermined intervals. It’s essential for jobs like updating user interface components, retrieving data from servers or making animations that call for recurring updates or operations.
However, using it too frequently can have a bad effect on performance. So to maintain maximum application performance, it must be used sparingly, taking into account performance consequences and making sure that thorough cleanup with clearInterval() is carried out when intervals are no longer needed.
See Also: JavaScript Popup Boxes: Alert(), Prompt(), & Confirm() Methods
Reference
https://developer.mozilla.org/en-US/docs/Web/API/setInterval