Have you seen the “copy to clipboard” button on the web? This is done for sample code, API keys, connection strings, URLs, etc to give the facility to the users to copy the data directly into your system clipboard without custom selecting the text and then using Ctrl + C (Windows) or ⌘ + C (macOS). This may look like a complicated feature but it can be implemented in JavaScript only by using a few lines of code.
In this tutorial, let’s write some code for creating a simple single-page website with the option to copy the text to the clipboard only using HTML and JavaScript. I will provide you with step-by-step instructions to make sure that the tutorial is totally beginner-friendly.
You might also like: String Interpolation in JavaScript
How to Copy Text to the Clipboard
We have two popular ways to add the copy to the clipboard feature. The first one is by using the document.execCommand(command) function which is an older and more widely used method for this. The second is by using the navigator.clipboard.writeText() function. But this way is relativity new and uses the asynchronous approach which might be complicated for beginners. Also as it is new, it is not supported in all browsers. So I will be teaching you copying using the execCommand function.
JavaScript document.execCommand Function
The execCommand function is used with the document object, it can be used to create copy-to-clipboard functionality. This function is supported in most browsers including Chrome, Firefox and Safari.
Syntax:
document.execCommand(command);
where command can be any command such as copy, cut, paste, bold, italic, etc., that is to be executed.
Creating Copy Text to Clipboard Option
Let’s create a website that has this copy-to-clipboard option.
Step 1: Basic Setup
The project setup is very simple. You just have to create a folder, open the folder inside any code editor, and create an index.html file for writing the code for making a button to copy which we will link to a JavaScript function for creating copy-to-clipboard functionality. For the JavaScript fucntion, we will write code on a separate file index.js so create that file too inside the same folder. You can also write JavaScript code in the HTML file using the script tag without making a separate file.
Step 2: Writing HTML Code
Now, let’s write the code in the index.html file.
index.html
<!DOCTYPE html>
<html>
<head>
<title>Copy Text to Clipboard</title>
</head>
<body>
<!-- Input field to enter the text -->
<input type="text" id="input" value="Text to be copied" />
<!-- Button to trigger the copy function -->
<button onclick="copyText()">Copy</button>
</body>
</html>
In the above code, we have a boilerplate code that has an input element with an id of “input” (you can use textarea for paragraph or long text to copy), we also have a function “copyText()” attached to the button which will be executed when this button is clicked. This function handles copy-to-clipboard functionalities.
Step 3: Handling Copy to Clipboard Button Using JavaScript
Now let’s write JS code for the copyText() function for creating the copy-to-clipboard functionality.
index.js
function copyText() {
var copyText = document.getElementById("input");
copyText.select();
document.execCommand("copy");
alert("Copied the text: " + copyText.value);
}
Here we first selected the element with an ID “input” and assigned it to the “copyText” variable. Then we extracted the text using “copyText.select()” and executed the “execCommand()” fucntion with the “copy” command to copy the extracted text into the clipboard and then alert it.
Step 4: Linking JavaScript to HTML
It is super important to link the JavaScript file (index.js) with HTML (index.html). If you haven’t then the function will not trigger and functionality will not work.
index.html
<!DOCTYPE html>
<html>
<head>
<title>Copy Text to Clipboard</title>
<script src="index.js"></script>
</head>
<body>
<!-- Input field to enter the text -->
<input type="text" id="input" value="Text to be copied" />
<!-- Button to trigger the copy function -->
<button onclick="copyText()">Copy</button>
</body>
</html>
Our index.js file and index.html are at the same level so we did not specify any directory, we just passed the file name inside the “src” attribute.
Step 5: Opening Website in the Browser
To open the website you can either manually open the HTML file on any browser or use the extension “Live Server” on VS Code to directly open the website on the default browser.
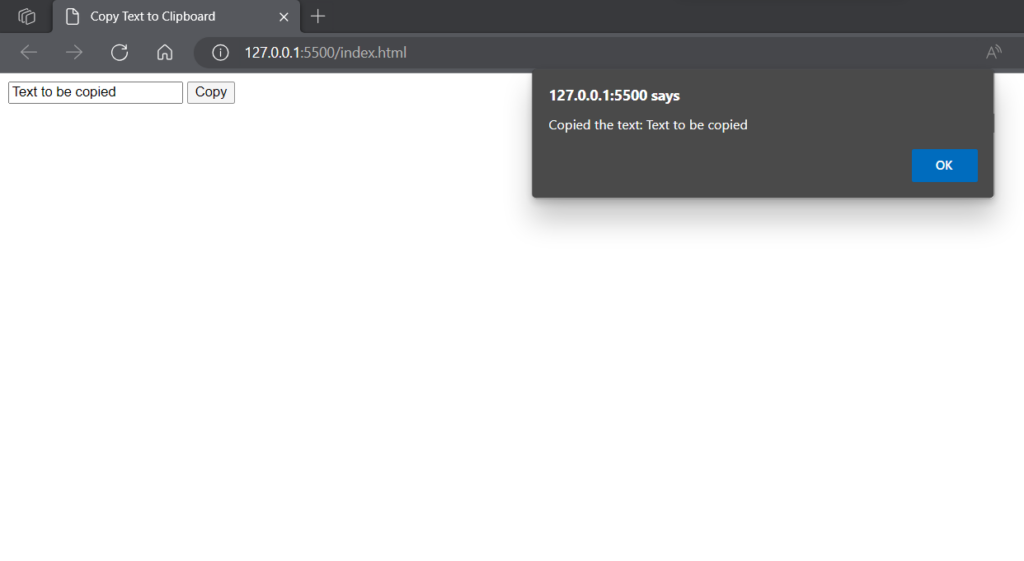
You can now check the clipboard, it will contain the text you copied. The application doesn’t look beautiful as we haven’t written CSS code.
Summary
In this tutorial, we have learned to copy text to the system clipboard with JavaScript. We have implemented the JavaScript function execCommand() for creating this “copytoclipboard” functionality. You can also use the writeText() method of the clipboard API navigator.clipboard, which takes the text to be copied to the clipboard as an argument. This method is mainly recommended for production & asynchronous operation but requires additional steps such as checking for browser permissions, etc. Hope you have enjoyed reading the content.
If you are new to JavsScript:
- Javascript Random Number Generator
- Scope In JavaScript – Block, Function, Local, and Global
- How to Check If a Date Is Today in JavaScript
Reference
https://stackoverflow.com/questions/400212/how-do-i-copy-to-the-clipboard-in-javascript