The reduce() method of an array in JavaScript is a built-in method that helps to reduce the elements of an array into a single value. By applying a function to each array element, it creates one result by combining them. It’s useful for tasks like adding up numbers, calculating averages, or merging data into one object or string. Conceptually, it facilitates operations that require the processing of all elements in an array to reach a final result.
Understanding the reduce() Method
The reduce() method reduces all the elements of an array into a single value, and that single value can be a number, string, object, etc. It does not execute the function for empty array elements. Also, it does not modify the original array.
Syntax:
The reduce() method is called on an array. Here is the syntax:
array.reduce(callbackFunction, initialValue)
Parameters:
This method primarily takes two parameters:
- callbackFunction: This function itself has two parameters: 1. accumulator and 2. current element to calculate the results. The current element is used to select every element in the array, one by one, for the operation. The accumulator is used to store the result after an operation on every element.
- initialValue: It is completely optional to provide this parameter. It is used to give an initial value to the accumulator. If this is not provided, then the first element in the array will be considered the initial value for the accumulator by default.
Return:
The reduce() method returns the last value stored in the accumulator which is a single value.
Purpose and Working of the reduce() Method
In this section, we will understand the purpose and working of the reduce() method.
Consider an array with 4 elements. If you are required to find the sum of each element, then you would probably write it this way:
const numbers = [5,-5,10,15]
let sum = 0;
for(let n of numbers)
sum += n;
console.log(sum);

You use a loop to iterate through each element and add it to the sum.
But what if I tell you that there is a more professional way of doing this? Yes, we use the reduce() method here:
const numbers = [5,-5,10,15]
const sum = numbers.reduce(total);
function total(acc,ce){
return acc+ce;
}
console.log(sum);
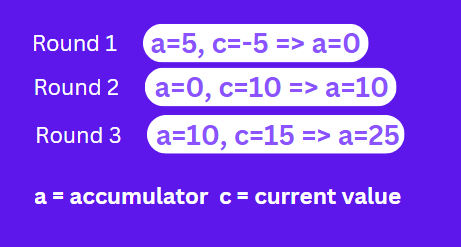
Now, values in the array will pass into the total function with the help of the reduce method.
Inside the total method, the value in the accumulator is not initialised externally so it takes the value of the first element, i.e., 5, as the initial value of the accumulator. The current element value is the value next to the accumulator, i.e., -5.
The callback function will execute multiple times, and each time the current value is set to one element in the array. For the first round of operation, the accumulator value results in 5+(-5)=0. Likewise, for the next round, the accumulator results in 0 + 10 = 10. And finally, in the last round, the accumulator value is returned as the output to the reduce method, which here is 25.

Now, observe the given code:
const numbers = [5,-5,10,15]
const sum = numbers.reduce(total,0);
function total(acc,ce){
return acc+ce;
}
console.log(sum);
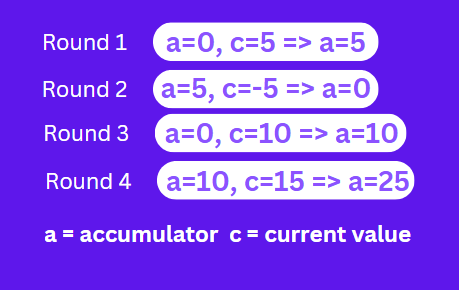
If you look closely, you will see that we have passed an initial value along with the callback function to the reduce method. This initial value sets the accumulator to 0 at the start, and the first element of the array (5 in this case) becomes the current value for the first round of calculations. The process then continues like this for the rest of the elements.
The output remains the same:

So, essentially, with the reduce() method, we start with an initial value, then loop through the array and convert all the elements into a single value, which is the accumulator.
Use Cases of the reduce() Method
This method can be used in any case where we expect the result to be a single value. Let us discuss some important use cases where we need a single output from an array.
1. Finding the Maximum Value
Reduce can be used to find the element with the largest value in a given array.
const numbers = [5,-5,10,15]
const max = numbers.reduce(findMax);
function findMax(acc,ce){
return Math.max(acc,ce);
}
console.log(max);
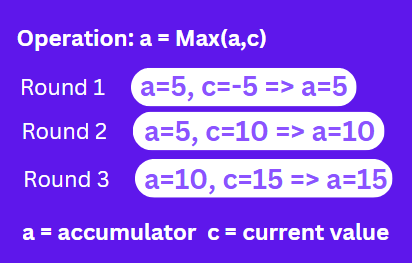

2. Finding the Minimum Value
Reduce can be used to find the element with the smallest value in a given array.
const numbers = [5,-5,10,15]
const min = numbers.reduce(findMin);
function findMin(acc,ce){
return Math.min(acc,ce);
}
console.log(min);
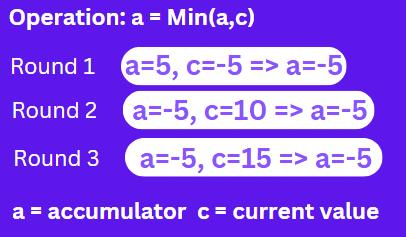

3. Finding the Sum of Rounded Values
We can round all the elements and find their sum using the reduce() method. In this case, we need to initialise the accumulator and set it to 0, or else the first value will not be rounded and the result will be inaccurate.
const numbers = [0.5,10.4,3.75,2.45]
const sum = numbers.reduce(total,0);
function total(acc,ce){
return acc + Math.round(ce);
}
console.log(sum);
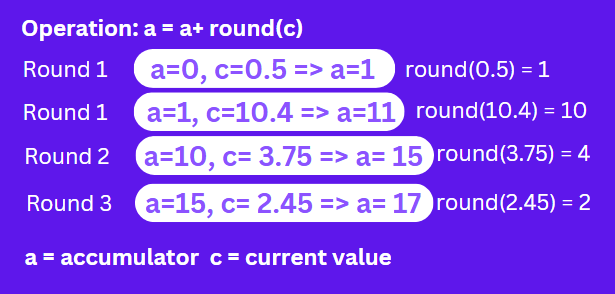

Summary
The reduce() method is a looping method that processes each element in an array from start to finish. It applies a “reducer” function to each element, combining them into a single value. The result from each step is passed into the next step as the accumulator. The final value of this accumulator, after the last element is processed, becomes the output of the reduce() method.
If you want to dive into more advanced array methods, check out these:
Reference
https://stackoverflow.com/questions/33392307/what-does-the-array-method-reduce-do