Do you know that _dirname in Node.js is responsible for representing the current directory of the script? Knowing the current directory is essential in Node.js applications for handling files, establishing reliable module paths, and maintaining a consistent file structure. So in this article, let’s try to get an in-depth knowledge of this variable and explore its significance and use in Node.js applications.
Overview of __dirname
In Node.js, __dirname is a special variable that gives the absolute path of the directory where the current script or module is running. It’s in the global scope and is commonly used to create dependable and platform-independent file paths.
The job of __dirname in Node.js is to help with handling files in your application. When combined with other tools for dealing with paths, __dirname lets developers make sure that the code finds and uses files dependably. This ensures that everything works well, no matter where the application is run or what operating system it’s on.
It gives the full path to the current module’s directory. This is important when dealing with modules, making sure they are found and used correctly. When it comes to tasks like reading or manipulating files, __dirname is a useful starting point. It helps keep a structured file setup in a Node.js app, ensuring consistent file management. In short, __dirname is crucial for working with files and modules, making Node.js applications strong and adaptable.
Using __dirname in Node.js
Now that we know what is _dirname, let’s see what are its use cases and how we can implement it into our Node.js applications.
1. Basic Usage
The provided code prints the absolute path of the directory containing the currently executing script using the console.log function.
console.log(__dirname);
When this script is executed, it will output the absolute path of the directory in which the “basic.js” file is located.
Output:

2. Constructing File Paths
The given code uses path.join to combine the current module’s directory (__dirname) with a relative path (‘../Hello.txt’). The variable filePath now contains the full path to the “Hello.txt” file, found by going up one level from the script’s directory.
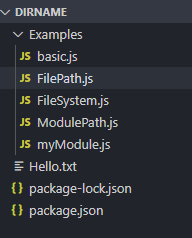
const path = require('path');
const filePath = path.join(__dirname, '../Hello.txt');
console.log(filePath);
When this script is executed, it prints the constructed file path to the console.
Output:

3. Resolving Module Paths
The given code imports a module named “myModule” and logs a greeting message obtained by calling its getMessage function. The “myModule” module exports a function that returns a specific greeting message.
//ModulePath.js
const path = require('path');
const myModule = require(path.join(__dirname, 'myModule'));
console.log(myModule.getMessage());
//myModule.js
module.exports.getMessage = function () {
return 'Greetings from myModule!,Welcome to CodeForGeek!';
};
When this script is executed, it prints the greeting message.
Output:

4. File System Operations
The use of __dirname is helpful for file system operations such as reading and writing. Let’s take a look at how.
Reading a File
The given code reads the content of a file named “Hello.txt” using the Node.js fs (File System) module. It constructs the file path using path.join, then uses fs.readFile to asynchronously read the file’s content in UTF-8 encoding. If successful, it logs the content to the console, otherwise, it logs any encountered error.
const fs = require('fs');
const path = require('path');
const filePath = path.join(__dirname, '../Hello.txt');
fs.readFile(filePath, 'utf8', (err, data) => {
if (err) {
console.error(err);
return;
}
console.log(data);
});
When this script is executed, it prints the greeting message from Hello.txt.
Output:

Writing to a File
The given code uses the fs (File System) module to write specified content to a file named “Print.txt”. It constructs the file path using path.join with __dirname, and then uses fs.writeFile to asynchronously write the content. If successful, it logs a success message, otherwise, it logs any encountered error.
const fs = require('fs');
const path = require('path');
const contentToWrite = 'We will learn to write content to a file using _dirname.';
const filePath = path.join(__dirname, 'Print.txt');
fs.writeFile(filePath, contentToWrite, 'utf8', (err) => {
if (err) {
console.error('Error writing file:', err);
return;
}
console.log('File written successfully:', filePath);
});
When you run the given Script you’ll see a new file added to the file structure.
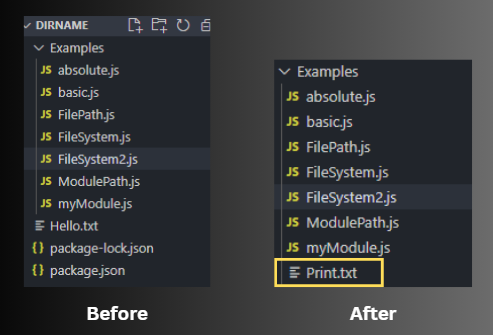
Output:

5. Constructing Absolute Paths
An absolute path is the full and exact location of a file or directory in a file system. The given code constructs the absolute path to a file named “Hello.txt” using the path.join method with __dirname.
const path = require('path');
const filePath = path.join(__dirname, 'Hello.txt');
console.log('Absolute Path:', filePath);
When this script is executed, it logs the resulting absolute path to the console.
Output:

6. Resolving Relative Paths
A relative path specifies the location of a file or directory about the current working directory. The given code resolves a relative file path (‘./files/File.txt’) to its absolute path using the path.resolve with __dirname. It then logs the resolved absolute path to the console.
const path = require('path');
const relativeFilePath = './files/File.txt';
const absolutePath = path.resolve(__dirname, relativeFilePath);
console.log('Resolved Absolute Path:', absolutePath);
Here the Relative.js file and the files directory are in the same directory and the File.txt file lies inside the files directory. When this script is executed, it logs the resolved absolute path to the console.
Output:

Difference Between process.cwd() and __dirname in Node.js
__dirname is favoured over process.cwd() for module-specific file operations because it supplies the constant directory path of the current module. This ensures reliability and independence from alterations in the process’s working directory, offering a consistent reference point for file operations within the module. Let’s see how __dirname differs from process.cwd().
_dirname | process.cwd() |
__dirname is like a fixed address for the current module, giving the absolute path to the directory where the current script is running. | process.cwd() shows where the Node.js process is currently working, indicating the directory from which it was launched. |
It is Module-Dependent. | It is Process-Dependent. |
It’s tied to the module it’s in and is helpful for creating paths based on the current module’s location. | It’s not module-specific, it shows the overall working directory of the entire Node.js process which is helpful for tasks dealing with files at the process level. |
It is Stable and Predictable. | It is Dynamic and Changeable. |
Example Use Case: Building paths to other files in the same module. | Example Use Case: Handling files and directories, even outside the current module, especially when the application changes directories while running. |
Conclusion
To sum up, the __dirname variable in Node.js acts like a reliable guide, giving the exact path of the current module’s folder. Even though it seems simple, it plays a big role in tasks like handling files and finding modules. For developers, using __dirname helps create more adaptable and strong applications. Let this little variable help you navigate directories, making your Node.js projects clear and easy to move around.
Continue Reading –
Reference
https://nodejs.org/docs/latest/api/modules.html#__dirname