UUIDs (Universally Unique Identifiers) play a vital role in modern software development. Creating Unique IDs is a standard need for many Node.js applications, from session management to database operations and for tracking inventory goods, upholding user accounts, and facilitating secure transactions are often included in software applications.
The goal of this article is to give a thorough understanding of UUIDs, their importance in Node.js development, and the several methods and tools available for safely and effectively creating them.
Introduction to UUIDs
UUIDs (Universally Unique Identifiers) are 128-bit numbers used to uniquely identify information in a distributed computing environment. They are designed to be unique across both space and time, meaning that even when generated by different systems at different points in time, the probability of two UUIDs being the same is extremely low.
Dynamically Generated UUID Structure:
xxxxxxxx-xxxx-Mxxx-Nxxx-xxxxxxxxxxxx
Here:
- x represents hexadecimal digits (0-9, A-F). These hexadecimal characters add to the UUID’s uniqueness and unpredictability.
- M represents the version of the UUIDs, which range from 1 to 5
- N specifies the variant of the UUIDs. Variants are specific bit patterns that define the UUID’s internal structure and layout.
Because of its standardized style, UUIDs created with Node.js are guaranteed to follow accepted practices and be readily understood by a variety of applications and systems.
Important of UUIDs in Node.js:
- Uniqueness – It offers a way to generate identifiers that are very unlikely to conflict with those created in other systems.
- Scalability – It enables these applications to scale seamlessly without risking collisions in identifiers.
- Globally Accessibility – It is standardized and widely recognized across different programming languages and generally accepted across many platforms.
- Security – It offers a way to generate unique IDs, which makes them good for applications that are sensitive to security, such as session tokens.
Methods for Generating UUIDs in Node.js
Below are several methods to generate UUID in Node.js.
1. crypto Package
This method was introduced in Node.js version 15.5.0. The built-in crypto package provides a randomUUID function for creating UUIDs. Here’s how you can use it.
Step 1: Import Package – Import the crypto package. We don’t need to install it.
const crypto = require('crypto');
Step 2: Call the randomUUID method to generate a unique id.
const uuid = crypto.randomUUID();
console.log(uuid);
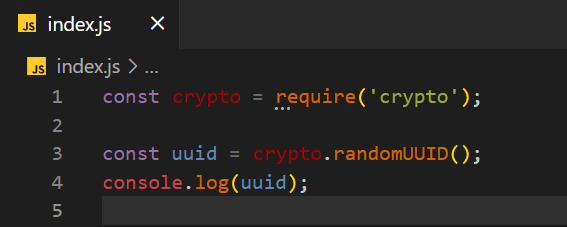
Step 3: To run the script we will run the following command.
node index.js
Output:
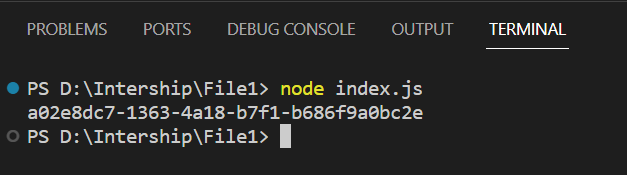
2. short-uuid Package
RFC4122 v4-compliant UUIDs are the starting point for NPM short-uuid, which converts them into other, often shorter forms. Additionally, it offers translators for converting UUIDs that comply with RFCs back and forth to shorter forms.
To install and set up the NPM short-uuid package in your Node.js project, follow these steps:
Step 1: Install the package – You can install the short-uuid package via NPM by running the following command in your terminal.
npm install short-uuid
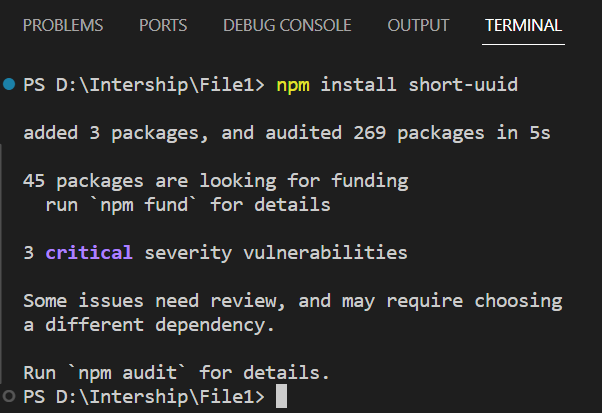
Step 2: Import the package – Once installed, you can start by importing the short-uuid module in your Node.js code using require function.
const short = require('short-uuid');
Step 3: Here’s a basic example of how to generate short UUIDs using the NPM short-uuid package.
const short = require('short-uuid');
const translator = short();
const shortUUID = translator.new();
console.log(shortUUID);
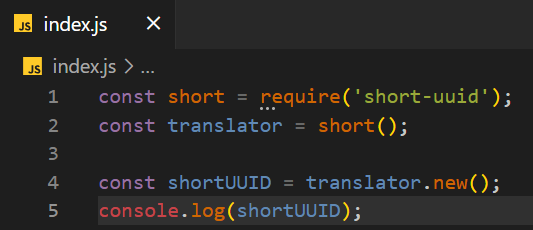
Here, we import the npm short-uuid module using the require function. This allows us to use the functionality provided by the short-uuid package in our code. We create a new instance of the short-uuid module by calling the function short(). Then we generate a short UUID by calling the new() method on the translator instance. This method generates a new short UUID using the default configuration (length and alphabet). The generated short UUID is assigned to the variable shortUUID. Finally, we log the generated short UUID to the console, allowing us to see the result of the UUID generation.
Step 4: To run the script run the below command.
node index.js
Output:
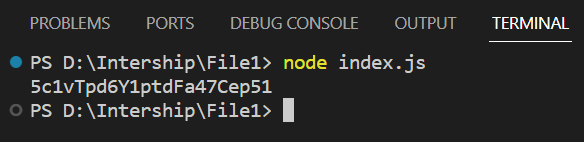
3. nanoid Package
“nanoid” is another NPM package that can be used to create a UUID. When it comes to JavaScript, it is a more user-friendly, dependable, and secure string ID generator than other smaller packages.
Step 1: Install the package – You can install the nanoid package using NPM.
npm i nanoid
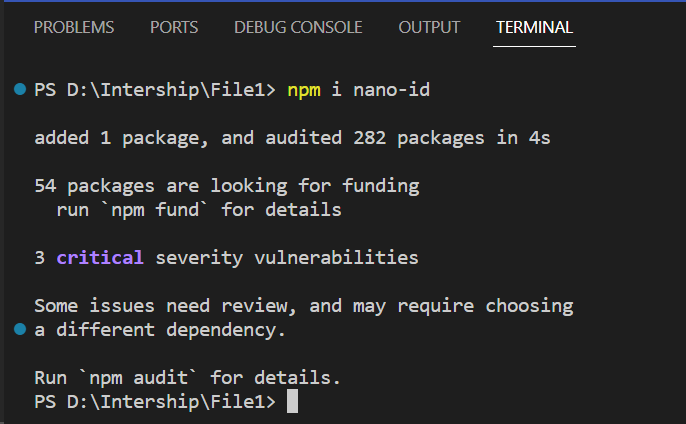
Step 2: Import the Package – You can start by importing the nanoid module in your Node.js code.
const { nanoid } = require('nanoid');
Step 3: Here’s a basic example of how to generate UUIDs using npm nanoid package.
const { nanoid } = require('nanoid');
// Generate a unique ID
const id = nanoid();
console.log(id);
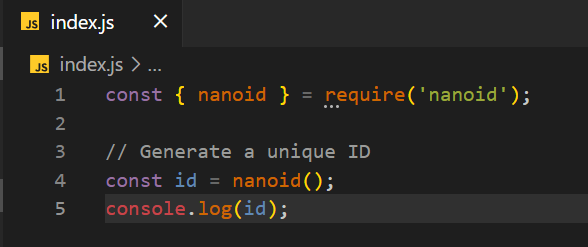
The nanoid() function generates a unique ID string of a default length of 21 characters. This ID is composed of URL-safe characters (A-Za-z0-9_-) and is designed to be collision-resistant.
First, we import the nanoid function from the nanoid package using destructuring. Then, we call the nanoid() function, which generates a random unique ID. The generated ID is stored in the id variable.
Finally, we log the generated ID to the console.
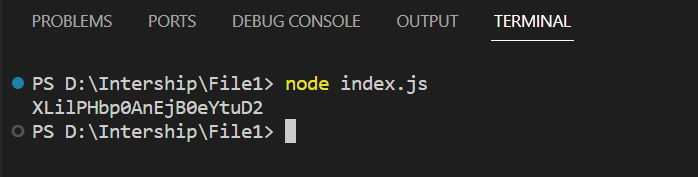
We can also customize the length of the generated ID by passing a number as an argument to the nanoid() function:
const customLengthId = nanoid(10); // Generate a unique ID of length 10
console.log(customLengthId);
This will generate a unique ID with a length of 10 characters. The length argument must be between 1 and 36, inclusive.
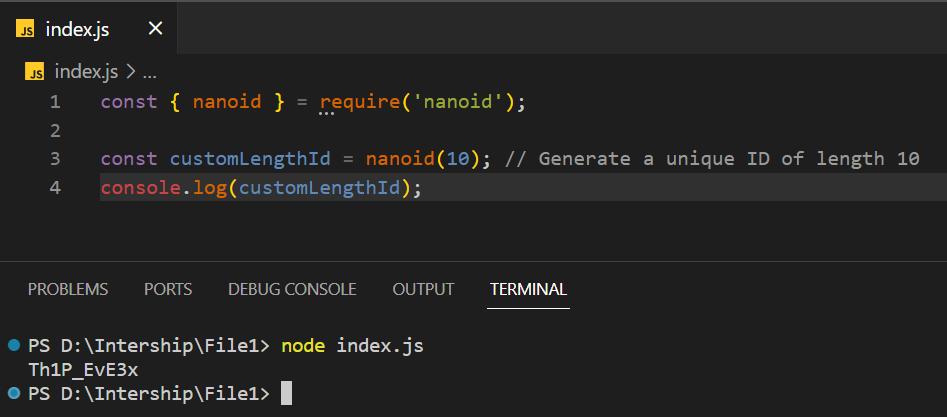
Conclusion
In conclusion, we can create UUIDs using Node.js by utilizing a variety of techniques and libraries, including the nanoid package, short-uuid via NPM and built-in crypto module. Node.js provides a number of solutions through built-in modules and NPM packages to produce unique identifiers quickly and effectively. Developers may choose the approach or package that best suits their needs and intended use.
Continue Reading:
- JavaScript setInterval() Method: Live Counter & Digital Clock Examples
- Reading and Writing JSON Files in Node.js
Reference
https://stackoverflow.com/questions/23327010/how-to-generate-unique-id-with-node-js