Ever wanted to create simple Word documents all right from your favourite web browser? With the power of JavaScript – you can! In this article we’ll walk you through it step by step, explaining it in the easiest possible terms, and with code examples for both basic and styled document creation.
Setting the Stage
Before diving into the code, let’s explore the tools we’ll be using:
- JavaScript: The language which make it possible for us to create interactive elements in web pages.
- docx.js: JavaScript library for generating Word documents (DOCX).
- FileSaver.js: The other JavaScript library that is used to save the generated document as a file in a downloadable file format.
To install docx and file-saver in our project, we can use npm like this:

Creating a Simple Word Document
Our first code example demonstrates creating a basic document with the text “Hello, World!”:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Generate Word Doc</title>
<script src="https://unpkg.com/docx"></script>
<script src="https://cdnjs.cloudflare.com/ajax/libs/FileSaver.js/2.0.5/FileSaver.min.js"></script>
</head>
<body>
<button id="generate">Generate Word Document</button>
<script>
document.getElementById("generate").addEventListener("click", function () {
const {
Document,
Packer,
Paragraph
} = docx;
try {
const doc = new Document({
sections: [{
children: [new Paragraph("Hello, World!")],
}, ],
});
Packer.toBlob(doc).then((blob) => {
saveAs(blob, "document.docx");
}).catch((error) => {
console.error("Error generating the document:", error);
});
} catch (error) {
console.error("Error initializing the document:", error);
}
});
</script>
</body>
</html>
Explanation:
- HTML Structure: It creates a basic webpage with a button.
- Libraries: We use CDN links for docx.js and FileSave.js.
- Button Click Event: This will run the JavaScript code when the button is clicked.
- Importing Components: We import Document, Packer and Paragraph from docx.js.
- Creating the Document: It creates a new Document with a single section with a Paragraph.
- Generating and Saving: It turns the document into a blob and then saves it to the client.
Output:
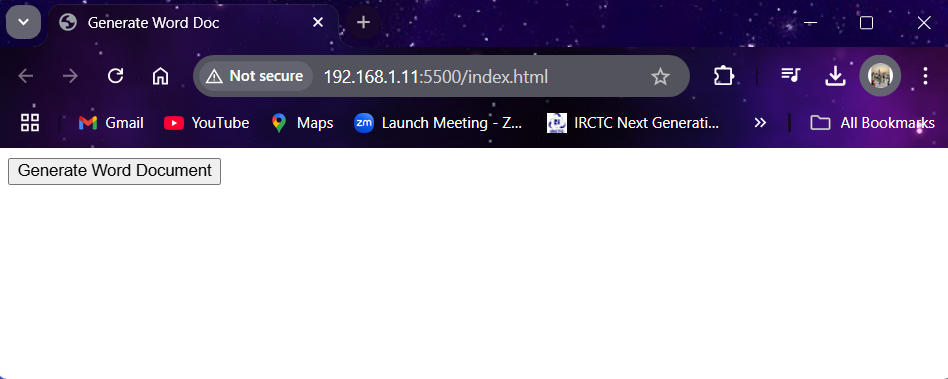
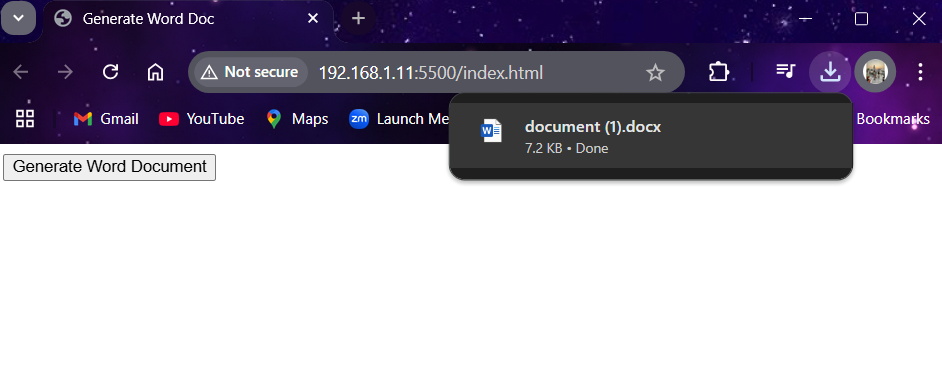
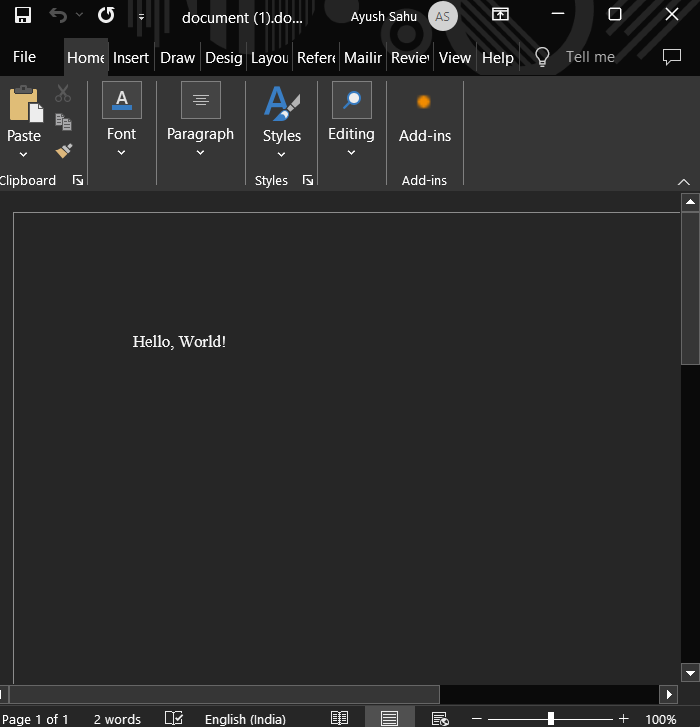
Formatting and Styling the Document
This example shows how to create a styled document with a title, font size changes, and bold text:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Generate Word Doc</title>
<script src="https://unpkg.com/docx"></script>
<script src="https://cdnjs.cloudflare.com/ajax/libs/FileSaver.js/2.0.5/FileSaver.min.js"></script>
</head>
<body>
<button id="generate">Generate Word Document</button>
<script>
document.getElementById("generate").addEventListener("click", function () {
const {
Document,
Paragraph,
Packer,
TextRun
} = docx;
function generateWordDocument() {
const {
Document,
Packer,
Paragraph,
TextRun
} = docx;
// Initialize the document with sections
const doc = new Document({
sections: [{
children: [
new Paragraph({
children: [
new TextRun({
text: "Styled Title",
bold: true,
size: 48,
}),
],
}),
new Paragraph({
children: [
new TextRun({
text: "This is a paragraph with some styled text. ",
}),
new TextRun({
text: "This text is bold.",
bold: true,
}),
],
}),
],
}, ],
});
// Generate and save the document
Packer.toBlob(doc)
.then((blob) => {
saveAs(blob, "StyledDocument.docx");
})
.catch((error) => {
console.error("Error generating the document:", error);
});
}
// Call the function to generate the document
generateWordDocument();
});
</script>
</body>
</html>
Key improvements in this example:
- TextRun: Now we have TextRun to apply styles to parts of text in a paragraph.
- Styling Options: We show size (font size), bold, italic and font properties.
- Multiple Paragraphs: Showing how to add more than one paragraph and one with basic styling.
- Function for Structure: By encapsulating the document creation logic inside a function (generateWordDocument), we make the code more organized and reusable.
Output:
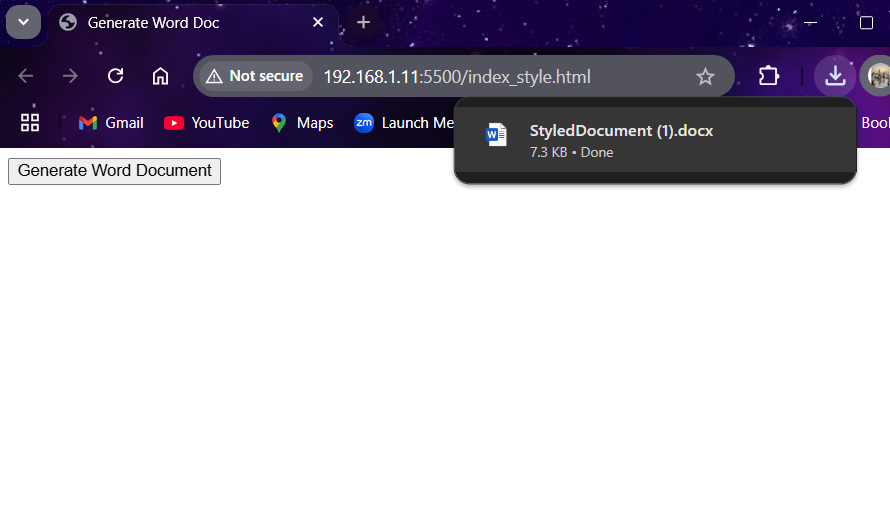
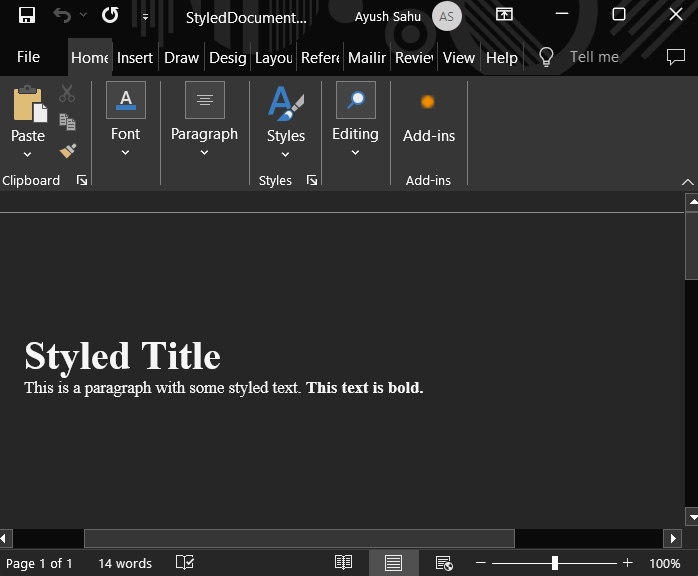
More Features
The docx.js library is much more than just basic text and styling. You can add:
- Tables: Layouts of structured data.
- Images: Inserting images in your documents.
- Lists: Make lists, ordered and unordered lists.
- Page Breaks: Control page layout.
- Headers and Footers: Add some consistent information to every page.
The full suite of available features can be explored in the docx.js documentation.
Conclusion
In this article I’ve shown how we can create a Word document in a browser right there, using JavaScript, docx.js, and FileSaver.js. We began with simple text and moved on to styling with varying font sizes, bold, italics, and fonts.
Creating Word documents with JavaScript is particularly important for tasks such as report generation (e.g. sales reports or data analysis summaries), invoice generation (e.g. e-commerce order confirmations) or custom document templates (e.g. contracts or proposals). With this client-side approach you reduce server load, improve user experience and allow offline access to documents, providing a practical solution to document-centric web approaches.
If you are new to JavaScript:
- JavaScript Variables
- JavaScript if…else Conditional Statement
- JavaScript Array
- JavaScript Object
- Scope In JavaScript
- JavaScript Error Handling
Reference
https://stackoverflow.com/questions/15899883/generate-a-word-document-in-javascript-with-docx-js