JavaScript uses objects everywhere, there are only a few primitive data types in JavaScript, and all the remaining data types are considered objects, these objects are of multiple types such as Boolean, Number, String, Array, Data, and Object.
Also Read: JavaScript Object
Understanding Objects in JavaScript
Below is the code that contains different types of objects.
const obj1 = {name: 'aditya'}; //'object'
const obj2 = ['aditya', 'gupta']; //'object'
const obj3 = new Date(); //'object'
const obj4 = Array(); //'object'
const obj5 = new Boolean(); //'object'
const obj6 = new Number(); //'object'
const obj7 = new String(); //'object'
const obj8 = new Object; //'object'
Using typeof() to Check for Data Types
The typeof() is a method that is preferred to check for the data types, let’s try it on different data types.
const var1 = 1; //'number'
const var2 = 'aditya'; //'string'
const var3 = true; //'boolean'
const var4 = null; //'object'
const var5 = undefined; //'undefined'
console.log('Type of var1: ' + typeof(var1));
console.log('Type of var2: ' + typeof(var2));
console.log('Type of var3: ' + typeof(var3));
console.log('Type of var4: ' + typeof(var4));
console.log('Type of var5: ' + typeof(var5));
Output:
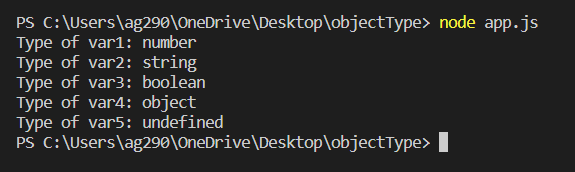
Here we are able to know the type, let’s try this method on different types of objects to know their type.
const obj1 = {name: 'aditya'}; //'object'
const obj2 = ['aditya', 'gupta']; //'object'
const obj3 = new Date(); //'object'
const obj4 = Array(); //'object'
const obj5 = new Boolean(); //'object'
const obj6 = new Number(); //'object'
const obj7 = new String(); //'object'
const obj8 = new Object; //'object'
console.log('Type of obj1: ' + typeof(obj1));
console.log('Type of obj2: ' + typeof(obj2));
console.log('Type of obj3: ' + typeof(obj3));
console.log('Type of obj4: ' + typeof(obj4));
console.log('Type of obj5: ' + typeof(obj5));
console.log('Type of obj6: ' + typeof(obj6));
console.log('Type of obj7: ' + typeof(obj7));
console.log('Type of obj8: ' + typeof(obj8));
Output:
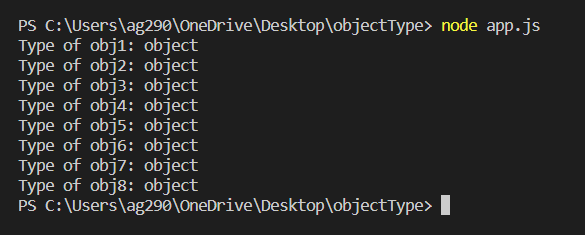
Here you have noticed that we are not able to get the type of object, the typeof() method just returns ‘object’ not its type.
It is difficult to understand which objects are of which type, this makes it complex to debug a large application that has multiple objects of different types.
Getting the Type of NodeJS Object
Whenever an object is created, it is created from a class that specifies its type, the class has a constructor which is called when an object is created from that class, and since the name of the constructor is the same as the class name, so if somehow manage to get the constructor name of the class from which the object is created, we get the information about the object type as it is same as the class name.
We can use dot notation with the keyword ‘constructor’ to select an object’s constructor and then use the dot notation with the ‘name’ keyword to get the constructor’s name.
Syntax:
object.construtor.name
where the object can be any JavaScript object.
Example:
const obj1 = {name: 'aditya'}; //'object'
const obj2 = ['aditya', 'gupta']; //'object'
const obj3 = new Date(); //'object'
const obj4 = Array(); //'object'
const obj5 = new Boolean(); //'object'
const obj6 = new Number(); //'object'
const obj7 = new String(); //'object'
const obj8 = new Object; //'object'
console.log('Type of obj1: ' + obj1.constructor.name);
console.log('Type of obj2: ' + obj2.constructor.name);
console.log('Type of obj3: ' + obj3.constructor.name);
console.log('Type of obj4: ' + obj4.constructor.name);
console.log('Type of obj5: ' + obj5.constructor.name);
console.log('Type of obj6: ' + obj6.constructor.name);
console.log('Type of obj7: ' + obj7.constructor.name);
console.log('Type of obj8: ' + obj8.constructor.name);
Output:
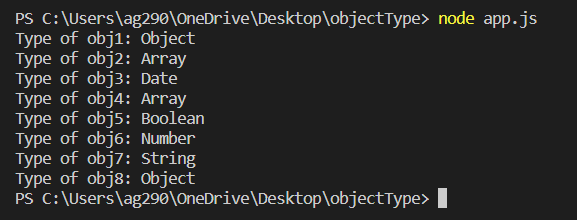
In the above output, we get the type of different objects.
Frequently Asked Questions (FAQs)
How to check type of value in JavaScript?
In JavaScript, we can check the type of a value using various operators and functions such as typeof operator to check a variable or object type, instanceof operator to check if an object is an instance of a particular class, Object.prototype.toString.call() for more in-depth information of an object type, Array.isArray() to check if a value is an array type and finally isNaN() to check if a value is NaN.
What is object type in JS?
Object type is the most used data type in JavaScript which represents a variable containing data in the form of key-value pairs inside curly braces. We can check whether a variable is of object type or not by using the JavaScript typeof operator.
How to check if type is object in JavaScript?
You can check if type is an object in JavaScript by using the instanceof operator with the keyword Object after the variable name you want to check for. Syntax – “variableName instanceof Object”.
How to get value from object type in JavaScript?
For getting the value from object type in JavaScript you have to use the object name then use a dot notation and type the name of the object properties you want to get the value of.
What is the use of typeof() function?
Just like typeof operator, the typeof() function is used to check the type of the operand, variable or object in JavaScript. They both are actually the same, when you use parentheses with typeof it becomes a function and without it, it is considered as an operator.
Summary
Objects are a fundamental part of JavaScript and are used everywhere in the language. There are few primitive data types, and all the remaining data types are considered objects. These objects include boolean, number, string, array, data, and object.
We can use the typeof() method to check for primitive data types, but this method won’t tell us the type of object. To get the type of an object, we can use the object constructor’s name to get the class name from which the object was created. In this article, we discussed the different types of objects in JavaScript, how to use the typeof() method, and how to get the type of an object.
Read More: JavaScript Date Objects (with a Real-Time Clock Application)
Reference
https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Operators/typeof
https://stackoverflow.com/questions/332422/get-the-name-of-an-objects-type