JavaScript object notation or JSON is a simple data exchange format that is simple for computers to understand and produce as well as for people to read and write. It is a text-based format that was inspired by the syntax of JavaScript object notation because of its simplicity and readability, represented as key-value pairs with curly brackets around them and also frequently used for configuration files data storage and data transmission between a server and a web application and is represented as key-value pairs with curly brackets around them.
With the help of beneficial tips and examples, this article will show you how to read and write JSON files with Node.js, giving you the knowledge you need to handle JSON files efficiently in your Node.js apps.
Reading JSON Files in Node.js
The fs modules and the built-in require() function in the Node runtime environment may be used to load and read JSON files.
1. Using Require Method
The main purpose of the Node.js require() function is to load modules. Regarding JSON files, it allows you to import and read JSON files natively.
Example:
const Data = require('./your-json-file.json');
console.log(Data);
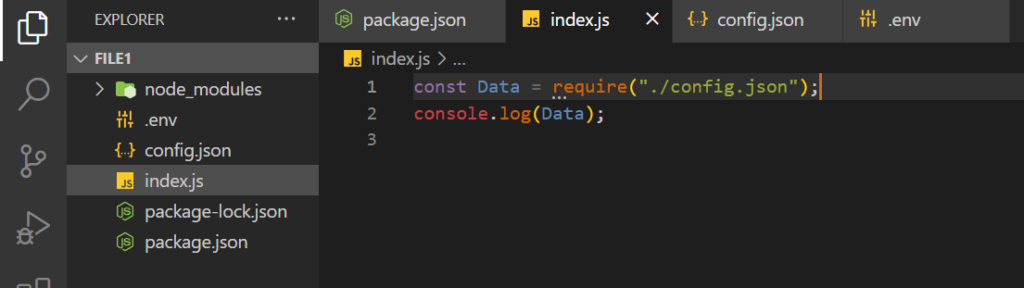
When loading a JSON file with Node.js require() function, the file loads synchronously and its content is cached. This implies that instead of reading the file again, subsequent calls to need the same file will obtain the data from the cache.
Output:
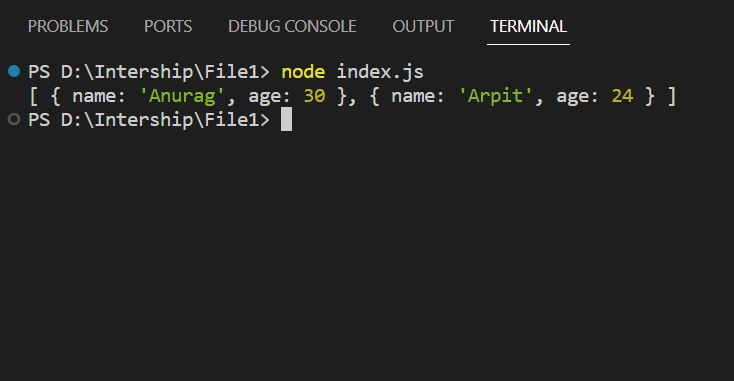
If you are working with dynamic data or in cases where the JSON file is expected to change while the program is running, this caching behaviour should be known by developers who can consider other approaches like asynchronous file reading and more ways to deal with dynamic data when real-time updates are required.
2. Using File System Module
The fs module provides functionality for working with the file system. We can read a JSON file using the fs module in Node.js can be done either synchronously or asynchronously.
Using “fs.readFile” to Read a JSON File Asynchronously
To read JSON files, use the readFile method. It reads the complete contents of the file asynchronously into memory. The readFile method takes three arguments
Syntax:
fs.readFile(path, options, callback);
Parameters:
- path – The path to the file you wish to read is indicated by this argument.
- options – You can specify extra settings for reading the file using this optional argument. It might be an object with many parameters, such as encoding.
- callback – This parameter is a function that will be called once the file has been completely read or an error occurs during reading.
Example:
const fs = require("fs");
fs.readFile("./config.json", "utf8", (error, data) => {
if (error) {
console.log(error);
return;
}
console.log(JSON.parse(data));
});
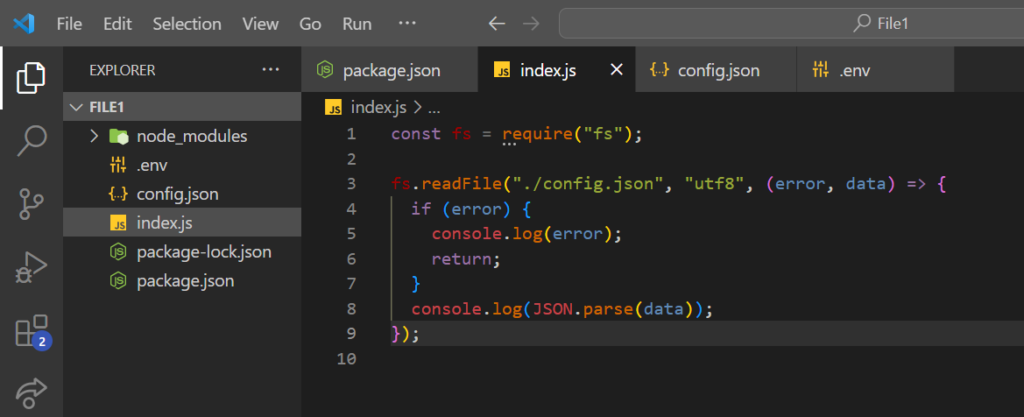
The “readFile” method is used to asynchronously read the contents of the specified file. Firstly we will import the built-in Node.js module “fs” (file system) then the “readFile” function is called with three arguments: the first argument takes the path of the file, the second argument “utf8” specifies the encoding of the file and the third argument is a callback function that will be executed once the file reading operation is complete. It takes two parameters: error and data. If an error occurs during the reading process, it will be stored in the error parameter. If the reading is successful, the contents of the file will be stored in the data parameter.
Output:
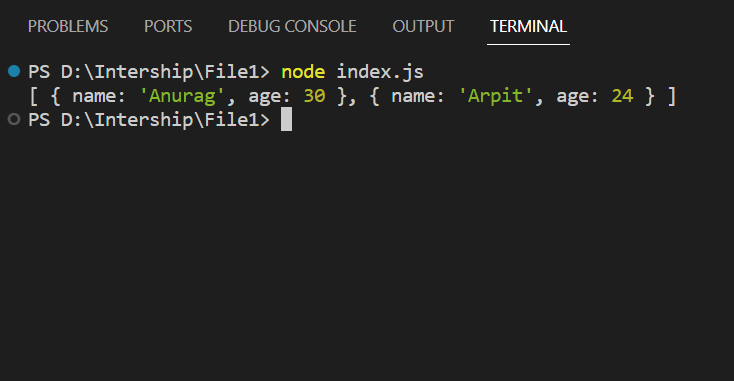
Using “fs.readFileSync” to Read a JSON File Synchronously
Similar to “readFile”, “readFileSync” is another built-in Node function for reading files. The way the two differ is that “readFileSync” reads the file synchronously, it stops the event loop and also the execution of the remaining code.
Syntax:
fs.readFileSync(path, options);
Parameters:
- path – The path to the file you wish to read is indicated by this argument. The file path may be contained in a buffer or a string.
- options – You can specify extra settings for reading the file using this optional argument. It might be an item with several options, like encoding.
Example:
const { readFileSync } = require('fs');
const data = readFileSync('./config.json','utf-8');
console.log(JSON.parse(data));
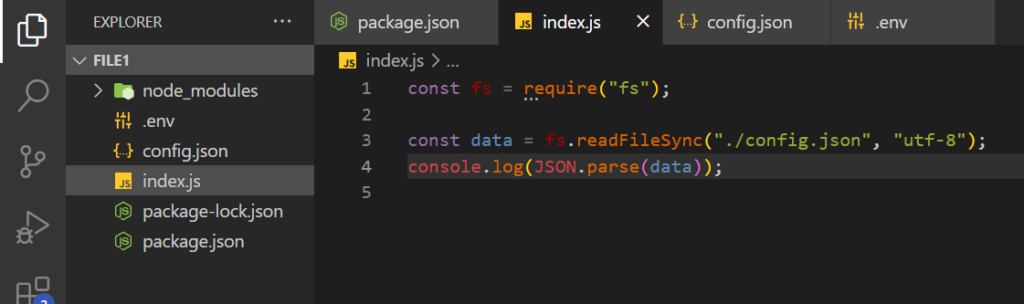
The above line of code illustrates how to use the “readFileSync” method from the Node.js “fs” module to synchronously read the contents of a JSON file called “config.json”. The code first imports the “readFileSync” function from the Node.js fs module. This function is used to synchronously read the contents of a file. The “readFileSync” function is called to read the contents of the “config.json” file. The code assumes that the content of the “config.json” file is a valid JSON string. It then uses the JSON.parse function to parse this JSON string into a JavaScript object.
Output:
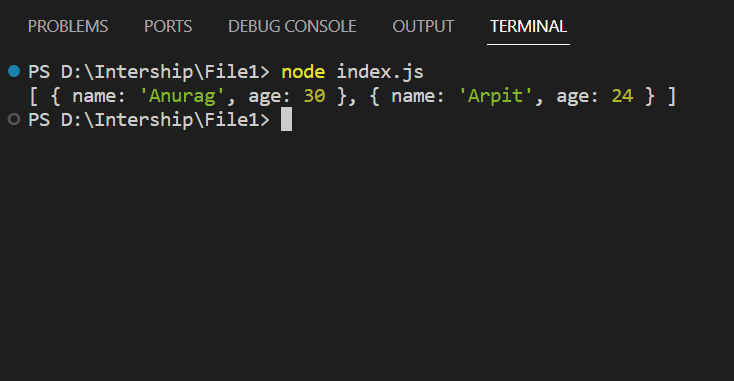
Writing JSON Files in Node.js
The fs module has built-in methods for writing to JSON files, just like it does for reading JSON files. The fs module “writeFile” and “writeFileSync” functions are usable. “writeFile” is asynchronous, but “writeFileSync” is synchronous.
Using “fs.writeFile” to Write a JSON File Asynchronously
The “fs.writeFile()” function in Node.js is used to asynchronously write data to a file.
Syntax:
fs.writeFile(file, data, options, callback)
Parameters:
- file – The name or descriptor of the file on which the data will be stored.
- data – The data to write to the file.
- options – A string or object that indicates the flag, mode, and/or encoding.
- callback – The function that will be used if an error occurs or the file has been written.
Example:
const fs = require('fs');
const users = require("./config.json");
const path = './config.json';
const user = {"name": "Abhishek", "age": 25};
users.push(user);
fs.writeFile(path, JSON.stringify(users, null, 2), (error) => {
if (error) {
console.log('An error has occurred ', error);
return;
}
console.log('Data written successfully to disk');
});
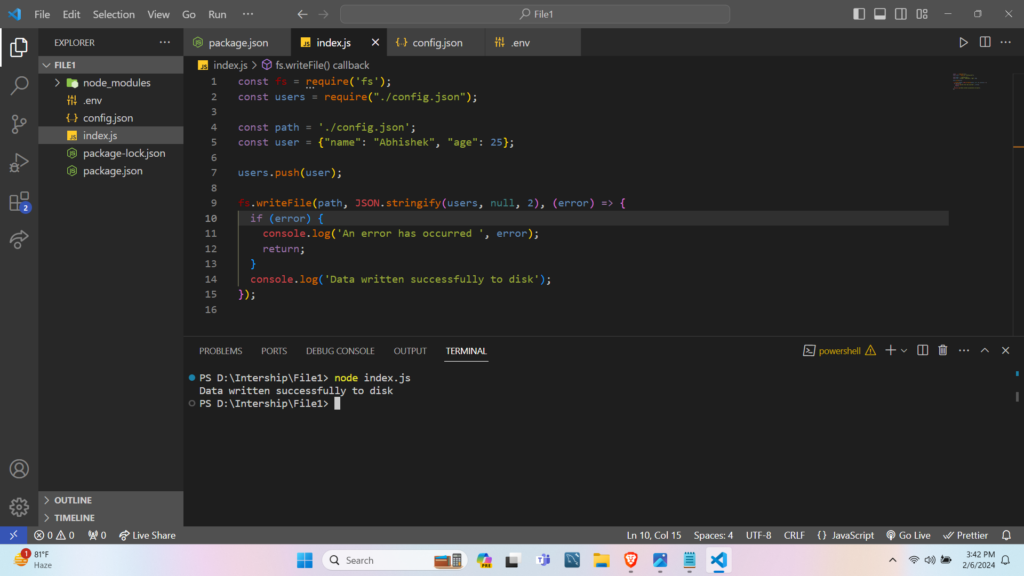
The code begins by importing the “fs” module and also importing “config.json” file using require() function, the file contains an array of objects that is stored in users variable then a new user object named user is created containing properties such as “name” and “age”. This object will be added to the users array by using push() method.
The fs.writeFile function is then invoked to write the modified users array back to the “config.json” file. It takes three arguments: the file path (path), the data to be written (the modified users array serialized to JSON format using JSON.stringify), and a callback function to handle any errors that may occur during the writing process.
Inside the callback function, an error parameter is checked. If an error occurs, an error message is logged to the console. If no error occurs, a success message indicating that the data has been successfully written to the disk is logged to the console.
Output:
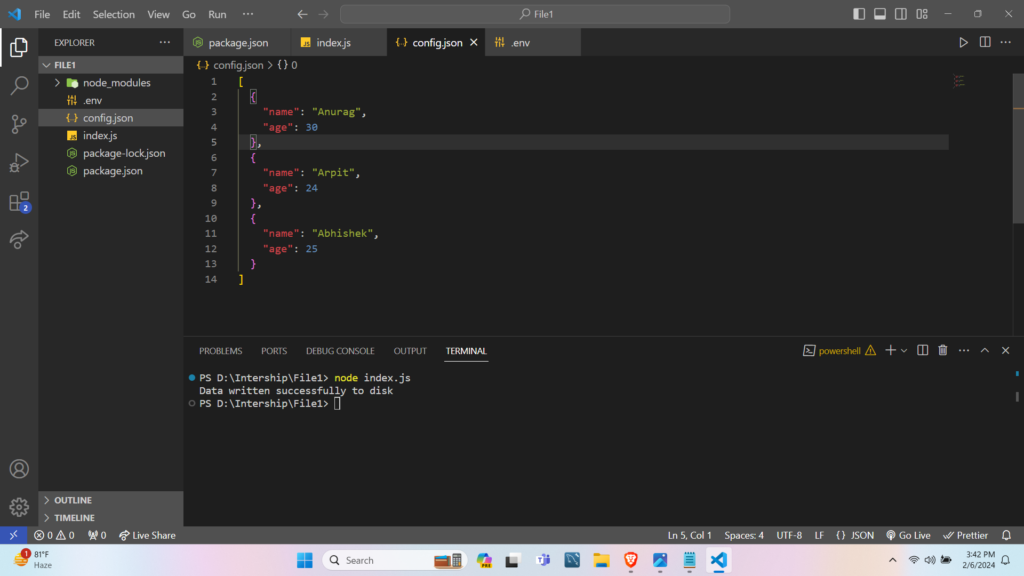
Using “fs.writeFileSync” to Write a JSON File Synchronously
The “writeFileSync()” method synchronously writes to a file. If the “writeFileSync()” method is used, the event loop and the remaining code will not run until the operation is completed or an error occurs.
Syntax:
fs.writeFileSync(file, data, options)
Parameters:
- file – The filename or descriptor that will be used to store the data.
- data – The information to be written to the file.
- options – A string or object that indicates the flag, mode, and/or encoding.
Example:
const fs = require("fs");
const users = require("./config.json");
const path = "./config.json";
const user = { name: "Ayush", age: 26 };
users.push(user);
try {
fs.writeFileSync(path, JSON.stringify(users, null, 2), "utf8");
console.log("Data successfully saved");
} catch (error) {
console.log("An error has occurred ", error);
}
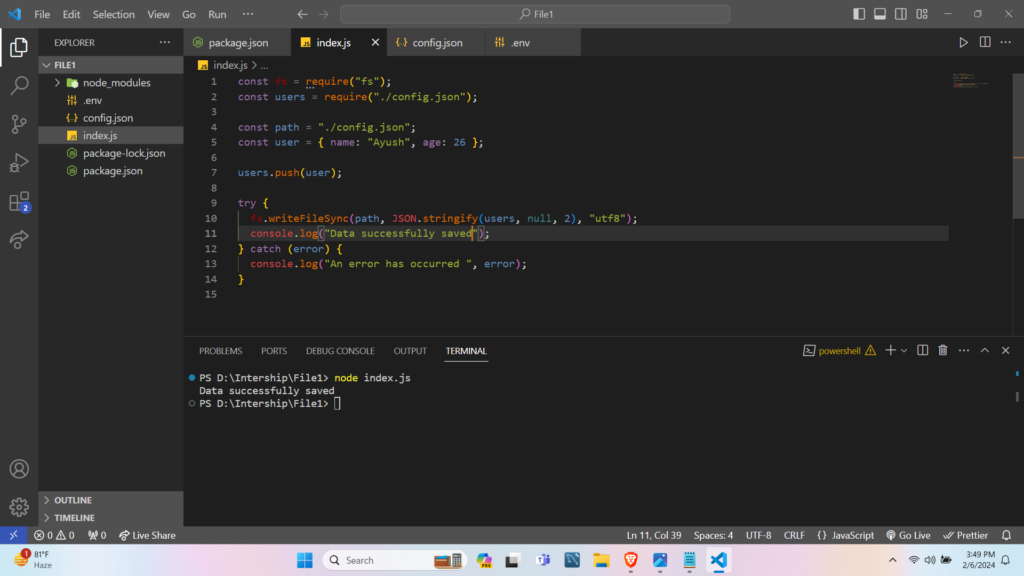
The provided code starts by importing the “fs” module, which provides functions for interacting with the file system in Node.js. It also imports the content of the “config.json” file into the user’s variable using the require function then a new user object named user is created, containing properties such as “name” and “age” and added to the user’s array using the push method. Inside a try-catch block the fs.writeSync function is used, this method is synchronous which means the execution of the code will block until the file write operation is complete.
Output:
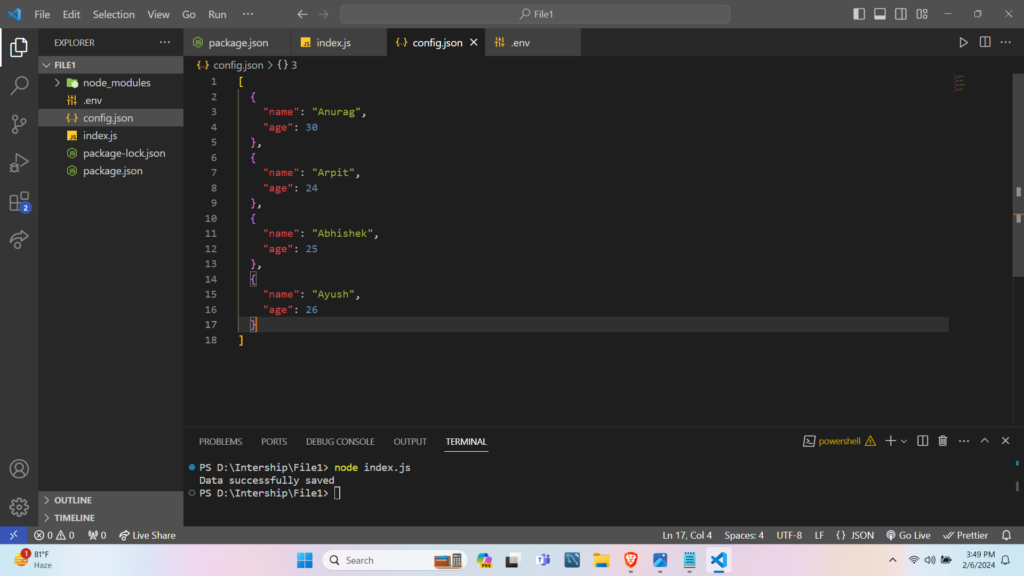
Conclusion
As a summary, we looked at two approaches to read and write JSON files in Node.js. The first one was using the require() function through which we can import JSON files as JavaScript objects directly. The second option employed the fs module that has functions for interacting with the file system. The fs module allows us to use both synchronous and asynchronous calls when reading and writing JSON files.
Each of these approaches is beneficial because it is applicable in specific situations. But whether you decide on one or the other will depend on many factors including things like file size, complexity and if you want your operations to be synchronously or asynchronously carried out.
Continue Reading:
- JSON.parse(): Convert JSON String To Object Using NodeJS
- Send a JSON response using Express Framework