Creating files is a fundamental task in Node.js development, allowing developers to generate new files dynamically for various purposes such as storing data, logging information, or serving as configuration files.
The built-in fs module in Node.js provides functions for file operations, including creating, reading, and writing files. In this article, we will focus on creating files using it. We will look at many approaches, including synchronous and asynchronous strategies.
Creating a File Synchronously Using openSync() Method
For creating files in Node.js, we can use the synchronous method fs.openSync() function. This method is specifically designed for creating files and opens to write when used with the ‘wx’ flag. When executed, the operation will fail if a file with the same name already exists, preventing unintentional overwrites. However, if the supplied file does not already exist, it will be created.
Syntax:
fs.openSync(path, flags)
Parameters:
- path – It holds the name of the file or the entire path if stored at another location.
- flags – Specifies the behaviour of the operation. i.e. r (read), w (write), r+ (read & write).
Example:
const fs = require('fs');
try {
const fd = fs.openSync('newfile.txt', 'wx');
console.log('File created successfully!');
} catch (err) {
console.error('Error:', err);
}
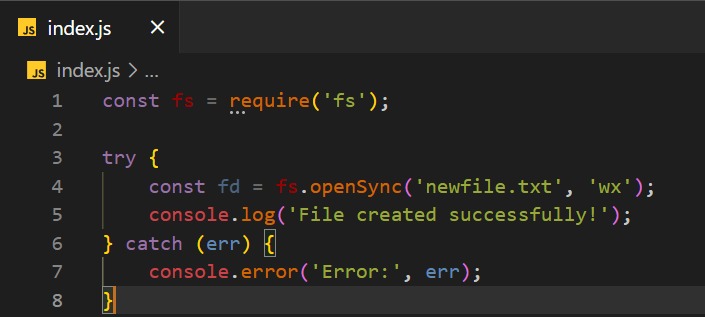
The fs.openSync() here is used to create and open a file named ‘newfile.txt’ with the ‘wx’ flag. This flag indicates that the file will be created for writing (‘w’), but if the file already exists, the operation will fail (‘x’). If the file is successfully created, the method will log “File created successfully” to the console. However, if an error occurs, such as the file already existing, it is caught by the catch block, and the error message is logged to the console.
Output:
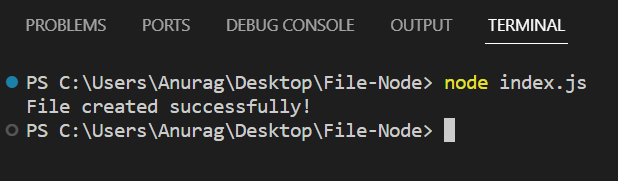
Creating a File Asynchronously Using fs.open() Method
Using the asynchronous method fs.open() with the ‘wx’ flag allows you to create a file and open it to write in Node.js while ensuring that the operation fails if the file already exists. As we already specified, this flag is very useful when you want to create a new file but prevent accidental overwriting of existing files.
Syntax:
fs.open(path, flags, callback)
Parameters:
- path: It holds the path of the file.
- flags: Specifies the behaviour of the operation. i.e. r (read), w (write), r+ (read & write)
- callback: It is a callback function that is called after reading a file.
Example:
const fs = require('fs');
const fileName = 'newfile.txt';
fs.open(fileName, 'wx', (err, fd) => {
if (err) {
if (err.code === 'EEXIST') {
console.error(`File '${fileName}' already exists.`);
} else {
console.error('Error:', err);
}
return;
}
console.log(`File '${fileName}' created successfully!`);
// It's good practice to close the file after you're done with it
fs.close(fd, (err) => {
if (err) {
console.error('Error closing file:', err);
}
});
});
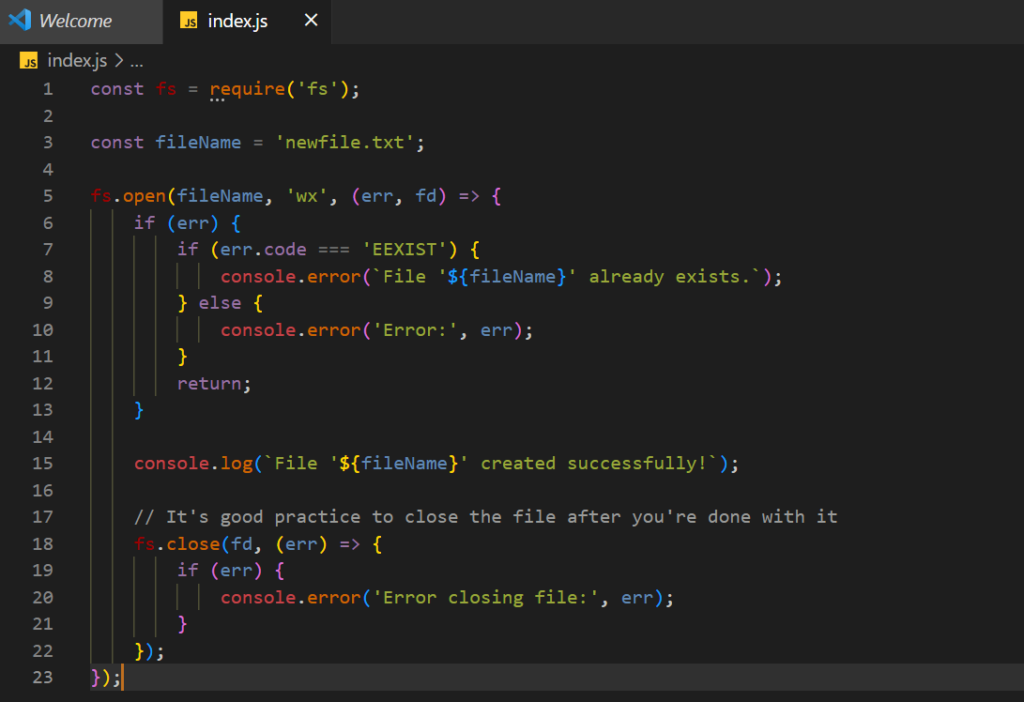
Here we have used fs.open() for write-only mode (‘w’) while ensuring it fails if the file already exists (‘x’). If the file exists, the err object code property will be ‘EEXIST’, indicating that the file already exists. If the file does not exist, it will be created, and the file descriptor (fd) will be passed to the callback function and log a success message.
Finally, it is a good practice to close the file after you are done with it to free up system resources. We use fs.close() for this purpose.
Output:
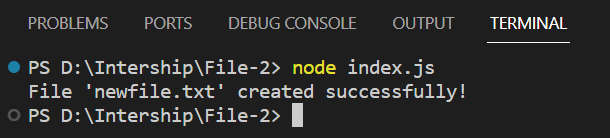
Creating a File Using the Third-Party fs-extra Module
For creating files in Node.js we can also use the fs-extra package, you just need to follow some simple steps to set it up.
Step 1: Install the Package – We can install fs-extra using NPM. Open your terminal or command prompt and run the below command.
npm install fs-extra
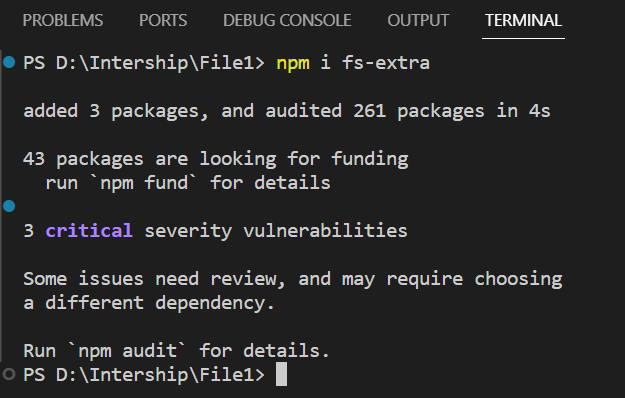
Step 2: Import the Package – You need to import the fs-extra module.
const fs = require('fs-extra');
Step 3: Using Package to Create an Empty File – Now we can use all the functions provided by the fs-extra package.
To create a file in Node.js we will use the createfile() function given by the fs-extra. The createFile() function is used to create an empty file if it doesn’t exist. If the file already exists, it will not modify it.
Example:
const fs = require('fs-extra');
async function createEmptyFile(fileName) {
try {
await fs.createFile(fileName);
console.log(`File '${fileName}' created successfully using npm fs-extra`);
} catch (err) {
console.error('Error:', err);
}
}
// Call the function to create the empty file
createEmptyFile('newfile.txt');
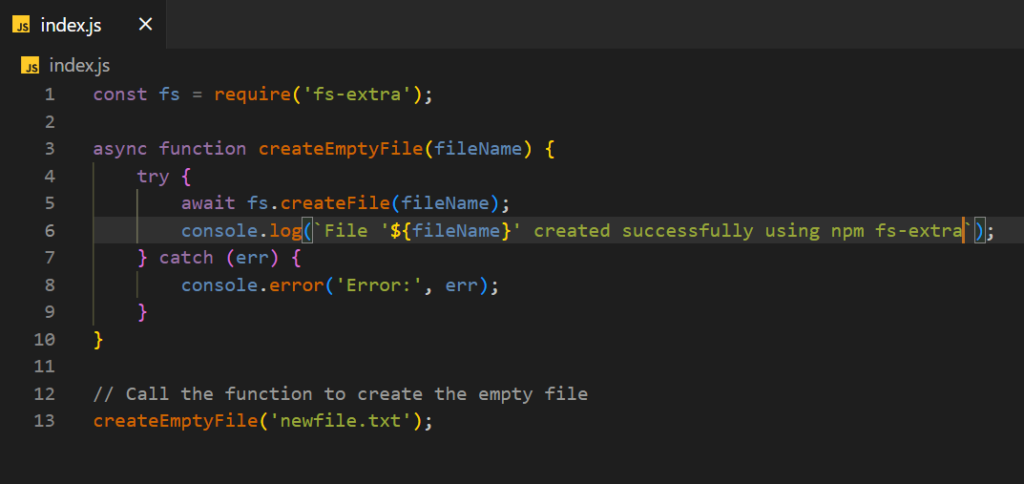
Here we have defined an asynchronous function createEmptyFile. This function takes a fileName parameter representing the name of the file to be created.
Inside the function, the fs.createFile method is awaited to create the file specified by the fileName. Upon successful creation of the file, a success message is logged to the console indicating that the file was created successfully. In case of an error during file creation, an error message is logged to the console.
Output:
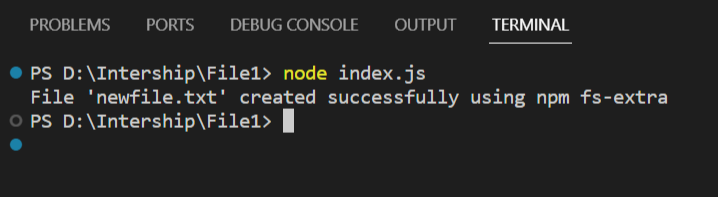
Conclusion
We explored three different methods for creating files in Node.js, each with unique benefits and applications. We started by exploring the built-in module fs, which offers both synchronous and asynchronous methods. Finally, we presented the createFile() method from the NPM fs-extra package, which offers a simple way to easily create empty files. Every approach accommodates different styles and project needs.
Continue Reading –
- Node.js console.log() Method: Introduction, Syntax & Examples
- How to fix [nodemon] app crashed – waiting for file changes before starting…
Reference
https://nodejs.org/api/fs.html