The ability to display Word documents within an application running in a browser improves the user experience greatly by allowing content to be viewed without the need for the user to download anything. There are many efficient ways of doing just this in JavaScript, from the use of libraries to tapping into external services like Google Docs Viewer. This is a guide that will cover different ways of showing Word documents using JavaScript, coding samples for use.
Why Display Word Documents in HTML?
Displaying Word documents in the browser is easier and more accessible. This is particularly useful for applications such as:
- Educational Platforms: To share notes and material for study.
- Document Management Systems: For quick previewing of files.
- Collaboration Tools: To simplify workflows without the need of additional software.
Now let’s look at ways of displaying a Word document.
Want to know how to create a Word document using JavaScript? Then click here!
1. Using File Upload and Mammoth.js
Mammoth.js libraries can convert .docx files into clean HTML which is relatively easy to display in a web app. Mammoth.js is all about extraction of useful content from Word documents, eliminating stupid styles.
How It Works:
- A .docx file is uploaded by the user.
- The FileReader API reads the file so the browser can read this file.
- The file is processed by Mammoth.js and is then converted to HTML.
- A <div> is used to display the HTML content.
Example Code:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Display Word Document</title>
<script src="https://cdnjs.cloudflare.com/ajax/libs/mammoth/1.4.2/mammoth.browser.min.js"></script>
</head>
<body>
<h1>Upload and View Word Document</h1>
<input type="file" id="upload" accept=".docx" />
<div id="output" style="border: 1px solid #ccc; padding: 10px; margin-top: 10px;"></div>
<script>
document.getElementById('upload').addEventListener('change', function (event) {
let reader = new FileReader();
reader.onload = function (event) {
mammoth.convertToHtml({ arrayBuffer: event.target.result })
.then(function (result) {
document.getElementById('output').innerHTML = result.value;
})
.catch(function (err) {
console.error("Error: ", err);
});
};
reader.readAsArrayBuffer(event.target.files[0]);
});
</script>
</body>
</html>
Output:
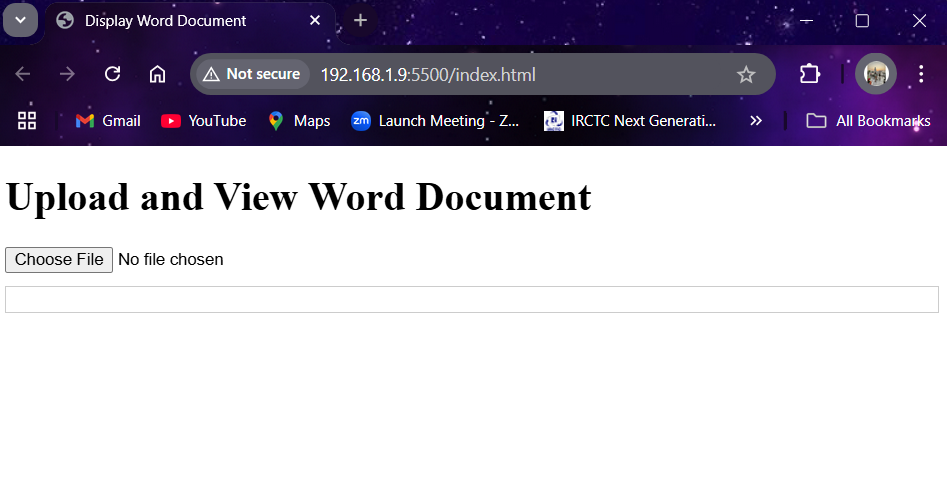
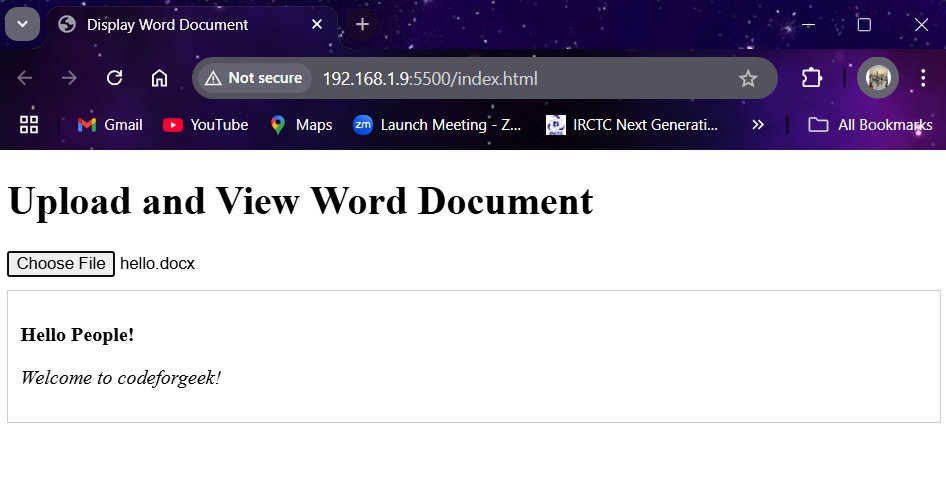
2. Using Google Docs Viewer
With Google Docs Viewer you can embed a viewer in your website to display documents. This approach is easy and only works for the URL of the document.
How It Works:
- The URL of a publicly accessible Word document is given by user.
- Google Docs Viewer link embeds the URL in an <iframe>.
- It is displayed as a document inside the browser.
Example Code:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Google Docs Viewer</title>
</head>
<body>
<h1>View Word Document</h1>
<input type="text" id="urlInput" placeholder="Enter URL of .docx file" style="width: 300px;" />
<button id="loadDoc">Load Document</button>
<iframe id="docViewer" style="width:100%; height:600px; margin-top: 10px;" src=""></iframe>
<script>
document.getElementById('loadDoc').addEventListener('click', function () {
let url = document.getElementById('urlInput').value;
let viewerUrl = `https://docs.google.com/gview?url=${encodeURIComponent(url)}&embedded=true`;
document.getElementById('docViewer').src = viewerUrl;
});
</script>
</body>
</html>
Output:
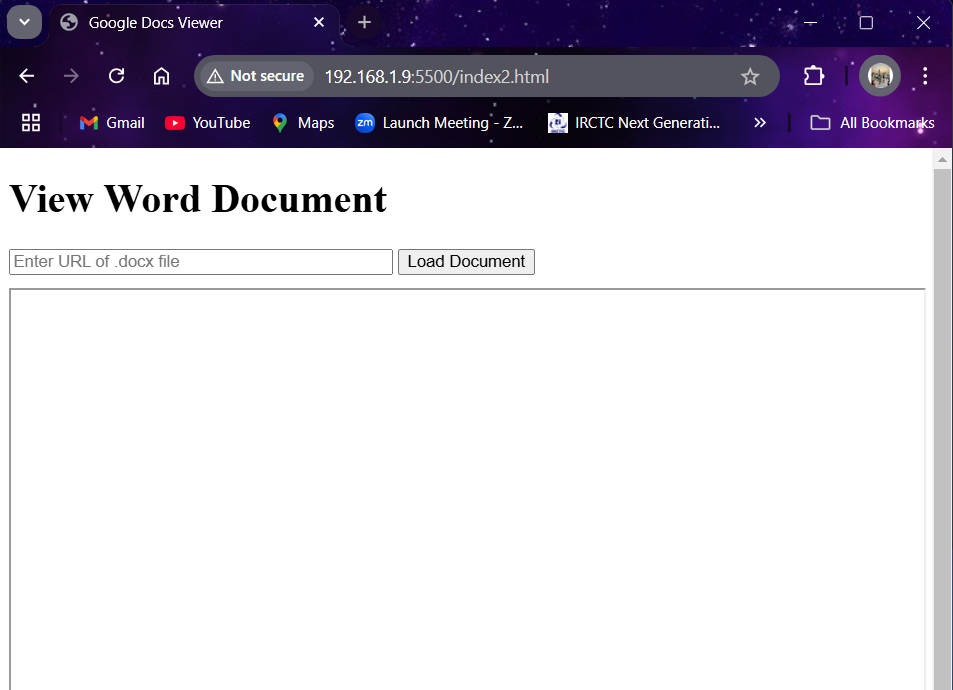
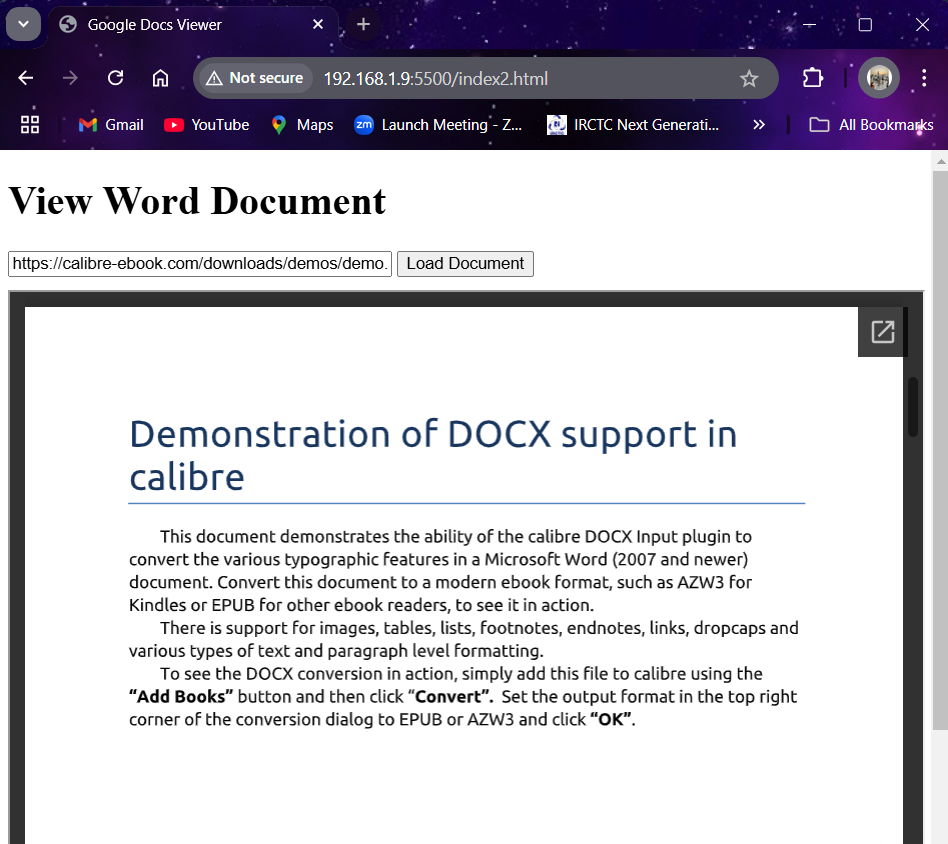
3. Converting Word Documents to PDF
One other way to achieve this is to convert Word documents to PDFs, which can then be shown using PDF.js libraries (which helps again with consistent formatting and it is easier to render PDFs with different browsers).
How It Works:
- Offline or server side convert Word document to PDF.
- Upload the PDF.
- To display the PDF, simply use PDF.js and put the PDF in a <canvas> element.
Example Code:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>PDF Viewer</title>
<script src="https://cdnjs.cloudflare.com/ajax/libs/pdf.js/2.6.347/pdf.min.js"></script>
</head>
<body>
<h1>Upload and View PDF</h1>
<input type="file" id="upload" accept=".pdf" />
<canvas id="pdfViewer" style="border: 1px solid #ccc; margin-top: 10px;"></canvas>
<script>
document.getElementById('upload').addEventListener('change', function (event) {
let file = event.target.files[0];
let fileReader = new FileReader();
fileReader.onload = function () {
let typedArray = new Uint8Array(this.result);
pdfjsLib.getDocument(typedArray).promise.then(function (pdf) {
pdf.getPage(1).then(function (page) {
let viewport = page.getViewport({ scale: 1 });
let canvas = document.getElementById('pdfViewer');
let context = canvas.getContext('2d');
canvas.height = viewport.height;
canvas.width = viewport.width;
let renderContext = {
canvasContext: context,
viewport: viewport
};
page.render(renderContext);
});
});
};
fileReader.readAsArrayBuffer(file);
});
</script>
</body>
</html>
Output:
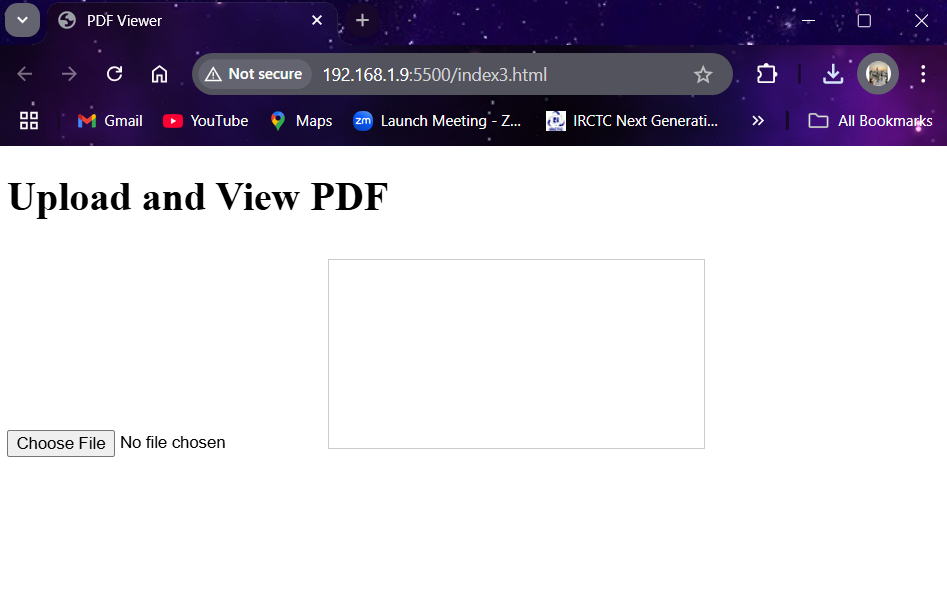
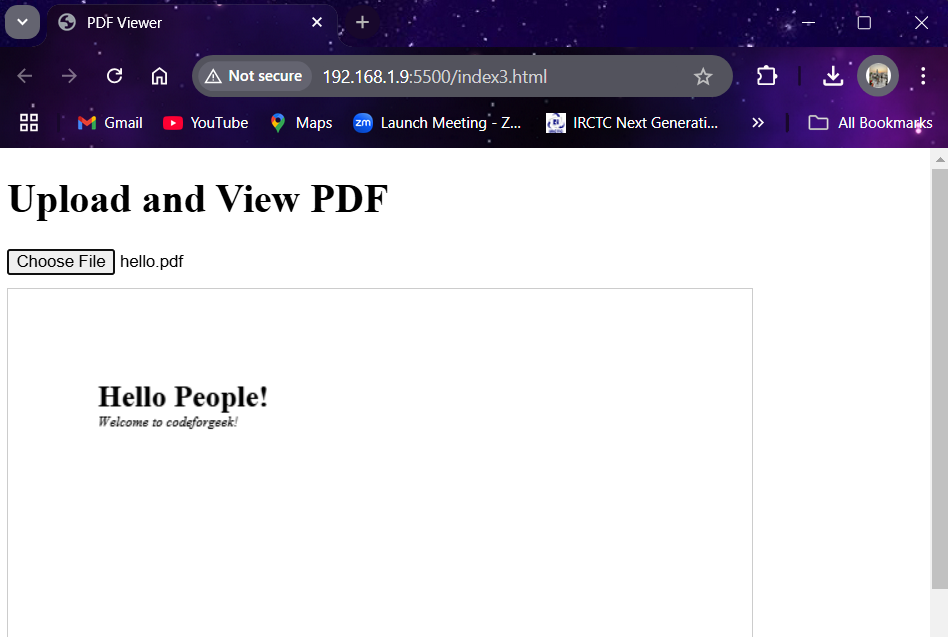
Conclusion
Each method discussed above caters to different requirements:
- Mammoth.js is for clean and fast HTML rendering of Word documents.
- Using Google Docs Viewer is an easy option for embedding.
- Use PDF.js if you need the document to be formatted.
If you choose the right method, you’ll be offering better document accessibility and a better user experience on your web application.
If you are new to JavaScript:
- JavaScript Variables
- JavaScript if…else Conditional Statement
- JavaScript Array
- JavaScript Object
- Scope In JavaScript
- JavaScript Error Handling