Did you know that we can make different types of HTTP requests to a server in Node.js? The request can be a GET, POST, PULL or DELETE request. Managing these requests manually is quite complex, especially handling responses and errors. So here comes the ultimate ‘Axios’ library.
In this tutorial, we will learn about the Axios library and its syntax for making different types of HTTP requests. We will also create a random recipe generator using Axios and Node.js to demonstrate its usage in a real-life scenario.
Introduction to Axios
Axios in Node.js is a thirty module that is used to make HTTP request directly from our code. Axios makes it super straightforward for Node.js developers to make HTTP requests like GET, POST, PUT, DELETE, etc and handle responses efficiently. We can write both synchronous and asynchronous code using it.
Key Features of Axios:
- Axios handles HTTP requests using promises, making it perfect to use async/await to write cleaner and easier-to-read asynchronous code.
- Axios convert JSON to JavaScript objects, eliminating the need to do manual conversion using JSON.parse() methods.
- Using it we can intercept HTTP responses to log or handle errors before actually receiving them in the application.
- It allows us to set custom header and query parameters.
- Axios also support old browsers, making it best for a wider audience.
- Using it we can cancel HTTP requests, even if sent, if they are no longer needed or are taking too long to respond.
Installing Axios:
Since Axios is not a built-in module in Node.js, we have to install it manually using NPM or Yarn.
npm install axios
Or
yarn add axios
Syntax for Making HTTP Requests With Axios
The codes that we can use to make different types of HTTP requests using Axios are given below.
For GET Request:
GET request is used to get data from a server or an external source.
const axios = require('axios');
axios.get('url')
.then(response => {
console.log(response.data);
})
.catch(error => {
console.error(error);
});
For POST Request:
A POST request is used to send data to a server.
const axios = require('axios');
axios.post('url', {
key1: 'value1',
// ...
keyN: 'valueN'
})
.then(response => {
console.log(response.data);
})
.catch(error => {
console.error(error);
});
For PUT Request:
A PUT request is used to update existing data.
const axios = require('axios');
axios.put('url', {
key1: 'value1',
// ...
keyN: 'valueN'
})
.then(response => {
console.log(response.data);
})
.catch(error => {
console.error(error);
});
For DELETE Request:
A DELETE request is used to delete data.
const axios = require('axios');
axios.delete('url/dataToDelete')
.then(response => {
console.log(response.data);
})
.catch(error => {
console.error(error);
});
Using Axios in Node.js
Using Axios with Promises:
Axiox uses promise to make HTML requests so we can use .then() and .catch() methods to handle the response and error.
const axios = require('axios');
axios.get('url')
.then(response => {
console.log(response.data);
})
.catch(error => {
console.error(error);
});
Using Axios with Async/Await:
We can also handle response and error using async/await. It is easier to use and readable than handling promises with .then() and .catch().
const axios = require('axios');
async function fetchData() {
try {
const response = await axios.get('url');
console.log(response.data);
} catch (error) {
console.error(error);
}
}
fetchData();
Creating a Random Recipe Generator Using Axios and Node.js
The best way to learn any concept is to build an application around it. Let’s try to create a random recipe generator using Axios in Node.js. Let’s see if this is helpful in providing a straightforward way to consume the API and get the required response.
const axios = require('axios');
async function getRandomRecipe() {
try {
const response = await axios.get('https://www.themealdb.com/api/json/v1/1/random.php');
const randomRecipe = response.data.meals[0];
console.log('Random Recipe:');
console.log('Name:', randomRecipe.strMeal);
console.log('Category:', randomRecipe.strCategory);
console.log('Instructions:', randomRecipe.strInstructions);
console.log('Ingredients:');
for (let i = 1; i <= 20; i++) {
const ingredient = randomRecipe['strIngredient' + i];
const measure = randomRecipe['strMeasure' + i];
if (ingredient && measure) {
console.log(`${measure} ${ingredient}`);
}
}
} catch (error) {
console.error(error);
}
}
getRandomRecipe();
Here we started by importing the ‘axios’ module. Make sure you have already installed it using the commands mentioned in the above section. Then we created a function getRandomRecipe() which uses the axios.get() method with async/await to make a request to the MealDB API to get a random recipe. The rest of the code after this separates the response object into different valuable sections and logs them to the console. In addition, we have also implemented the catch() method to catch if any error occurs while retrieving the data.
Output:
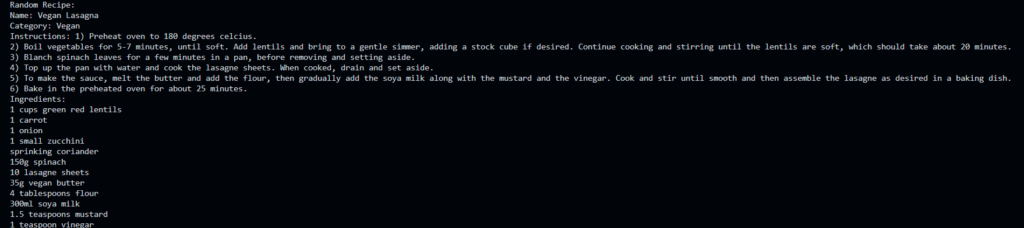
Conclusion
In conclusion, Axios is the easiest way to handle HTTP requests in Node.js. No matter which approach you prefer, whether using Promises or async/await, it can support both. It is faster than other methods and provides a way to write cleaner and more efficient code. We hope you enjoyed reading this article. To learn about other ways to make HTTP requests, click here and to create your own RESTful API in Node.js, click here.
Reference
https://www.npmjs.com/package/axios