Do you know how developers create applications to check the weather, get current city temperature, track bitcoin value, etc. even if they do not have such data? Well, welcome to the world of APIs.
Millions of websites and servers on the Internet provide APIs that we can use to get different types of data. For example, to create an application that shows the weather based on the user’s location, we can use OpenWeatherMap API, make a GET request for the weather details of the given location, and then show it to the user the way we want.
What is an API?
API stands for application programming interface, which one application can provide to another to access its resources. It can be created for internal use or made public so the world can use it. It is like a restaurant menu containing a list of dishes you can request for.
What is a RESTful API?
A REST (also called RESTFul) API stands for Representational State Transfer Application Programming Interface that applications can use to communicate over the internet using a set of rules. REST APIs use simple HTTP methods such as GET (to get data), POST (to send data), PUT (to update data), and DELETE (to remove data), making it secure and easy to handle for both server and client.
Want to create your own RESTful API using Node and MongoDB? Click here.
Consuming RESTful API in Node.js
There is only one way to fetch data from a RESTful API, that is by making a valid HTTP request (such as GET, POST, PUT, or DELETE) to interact with the API endpoints and in Node.js we have many ways to do so.
Different method returns different types of responses containing different properties and methods for an API so it is essential to learn multiple methods so that you can choose them according to the project requirement. Let’s understand some of the top ones.
Making HTTP Requests in Node.js
Let’s now get started with learning the different methods to make an API call using Node.js.
fetch()
The best way to make an HTTP request is using the Fetch API in Node.js. In browsers, the Fetch API is built-in and is used to make HTTP requests to the server, get data, and handle responses.
In the Node.js version below v17.5, using Fetch API is not possible natively so we have to use a third-party library named node-fetch to get it. But this method is so not advantageous when there is a better option, which is to upgrade your Node.js application to version v18 and above where this Fetch API comes built-in. This eliminates the need to rely on a third-party library and increases scalability and reliability.
Use the below command in the terminal to check for the Node.js version installed on your system:
node -v
You can download the setup file of Node.js latest version from the Node.js Official Website.
To make HTTP requests in node.js with fetch API, you have to use it by using the –experimental-fetch parameter as is an experimental feature:
node fileName –experimental-fetch
where the fileName is the file you want to execute
Syntax to use the fetch Method:
fetch(url)
where the url is the address of an API
Node.js Fetch API returns a promise that resolves to a response object containing the following methods and properties:
- text() – returns the response as a string
- json() – parser the response into a JSON string
- status – return the status code
- statusText – return the status message
- ok – return true if the request is successfully completed
- url – the value of the final URL
- header – contains response header
Example:
const url = 'https://api.adviceslip.com/advice';
fetch(url)
.then((response) => response.json())
.then((data) => {
console.log(data);
});
Output:
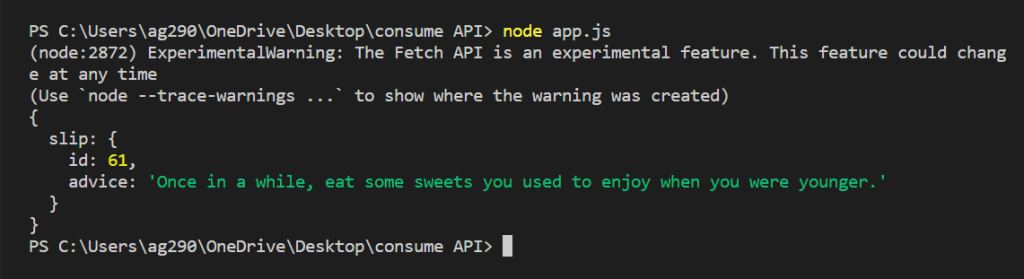
axios()
Another easy-to-use method to consume data from an API is using Axios. It provides a simple interface for making HTTP requests. Axios convert JSON data to JavaScript objects, eliminating the need to do manual conversion using JSON.parse() methods.
To use this method, we have to use the Axios module. Axios module can be installed by npm using the below command:
npm i axios
This method takes a URL as an argument in order to send the get request.
Syntax:
axios(url)
where the url is the address of an API
Axios returns a response object containing the following properties:
- data – the actual data
- status – return the status code
- statusText – return the status message
- header – contains response header
- config – the request configuration
- request – the request object
Example:
const axios = require('axios');
const url = 'https://api.adviceslip.com/advice';
(async () => {
const response = await axios(url);
console.log(response.data);
})();
Output:
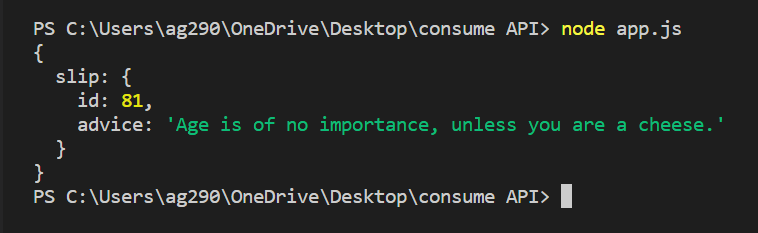
https.request()
This method can be used to send the HTTPS request to the API in order to fetch the data. For using this method it is required to import the HTTPS module. The HTTPS is a built-in module used to send an HTTPS request to a secure web server.
This method takes a URL as an argument and a callback which makes it asynchronous in nature.
Syntax:
https.request(url, callback)
where the url is the address of an API
This method returns the data stream containing data in chunks which can be finalized using the stream’s event.
We have a separate tutorial on Node.js Events if you want to learn about them.
Example:
const https = require('https');
const url = 'https://api.adviceslip.com/advice';
const request = https.request(url, (response) => {
let data = '';
response.on('data', (chunk) => {
data = data + chunk.toString();
});
response.on('end', () => {
const body = JSON.parse(data);
console.log(body);
});
})
request.on('error', (error) => {
console.log('An error', error);
});
request.end()
Output:
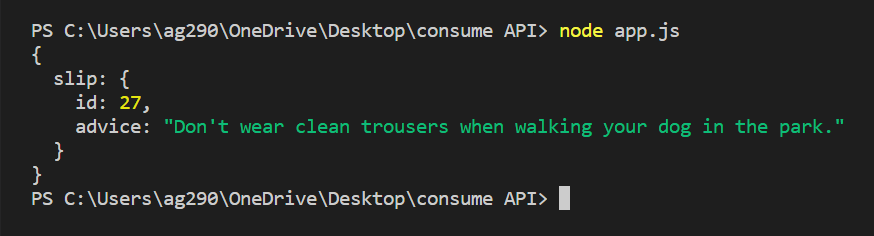
Summary
Whether you are doing web development or app development, the APIs are the best way to use the methods and data of the other websites and servers in your project in order to get different types of information such as weather reports, sports news, movie releases dates, etc. For consuming RESTful API we can use many different methods such as fetch(), axios(), request(), etc.
If you are using Node.js version v18 and above, the best option might be to use the fetch() methods, but if you do not want to upgrade, you can use axios(), which is easy to use, straightforward and gives data in the best suitable format. Hope this tutorial helps you to learn the methods of consuming the RESTful API in NodeJS.
References
- https://nodejs.org/api/https.html
- https://www.npmjs.com/package/axios
- https://nodejs.org/api/https.html#httpsrequestoptions-callback