We can easily add text to images within our web app using JavaScript. To do this, we have to create a canvas and place both the image and text. This allows us to add a text description to the image, position the text anywhere we want, and customise it as needed. Let’s see how.
Also Read: JavaScript Popup Boxes – Alert(), Prompt(), & Confirm() Methods
6 Steps to Add Text to Image Using JavaScript
Imagine we have an image of a scene, and we want to add a ‘Good Morning’ greeting to it. Here’s how we can do that:
Step 1: Create an Image Object
Firstly, we will add an image to our website. To do this, we create an image object and display it using the document.body.appendChild() method and set the source for our image.
// Create an image object
var image = new Image();
document.body.appendChild(image);
// Set the source of the image
image.src = "scene.jpg";
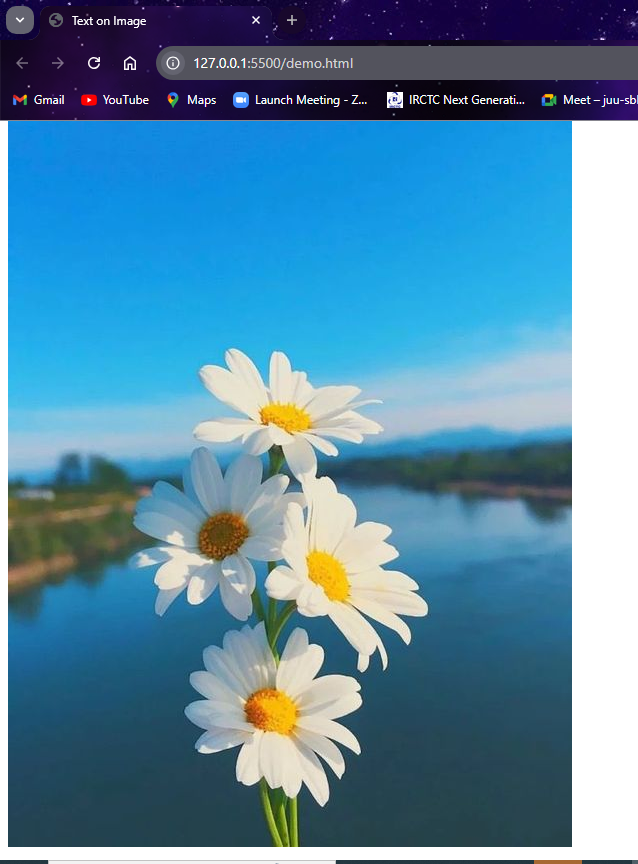
Step 2: Create a Canvas Element
Now it’s not directly possible to write on the image, so what we do is we create a canvas and put our image and text into it.
A canvas acts like a drawing paper where we first stick our image and then write on it. We will add the canvas only when the image is loaded.
var image = new Image();
// When the image is fully loaded, do this
image.onload = function() {
// Create a canvas element (a place to draw)
var canvas = document.createElement("canvas");
var context = canvas.getContext("2d");
// You can now use context to draw on the canvas
};
document.body.appendChild(image);
image.src = "scene.jpg";
Step 3: Draw the Image on the Canvas
Now we will match the size of the image with the size of the canvas and draw the image on the canvas.
var image = new Image();
image.onload = function() {
var canvas = document.createElement("canvas");
var context = canvas.getContext("2d");
// Set canvas size to match the image
canvas.width = image.width;
canvas.height = image.height;
// Draw the image on the canvas
context.drawImage(image, 0, 0);
};
document.body.appendChild(image);
image.src = "scene.jpg";
Step 4: Write Text on the Canvas
When we write the text on the canvas, we eventually write text on the image because the image is set on the canvas. You can set the font, colour, and content according to your requirements.
var image = new Image();
image.onload = function() {
var canvas = document.createElement("canvas");
var context = canvas.getContext("2d");
// Set canvas size to match the image
canvas.width = image.width;
canvas.height = image.height;
// Draw the image on the canvas
context.drawImage(image, 0, 0);
// Add text on top of the image
context.font = "40px Arial"; // Font size and style
context.fillStyle = "white"; // Text color
context.fillText("Good Morning!", 50, 100); // Text and position
document.body.appendChild(canvas); // Display the canvas
};
image.src = "scene.jpg";
Step 5: Display the Canvas
Next, we will display the canvas. Till now, we are appending the image object, but the text is written on the canvas, so we will display the canvas using the document.body.appendChild() method.
var image = new Image();
image.onload = function() {
var canvas = document.createElement("canvas");
var context = canvas.getContext("2d");
// Set canvas size to match the image
canvas.width = image.width;
canvas.height = image.height;
// Draw the image on the canvas
context.drawImage(image, 0, 0);
// Add text on top of the image
context.font = "40px Arial"; // Font size and style
context.fillStyle = "white"; // Text color
context.fillText("Good Morning!", 50, 100); // Text and position
// Display the canvas on the webpage
document.body.appendChild(canvas);
};
// Set the source of the image
image.src = "scene.jpg";
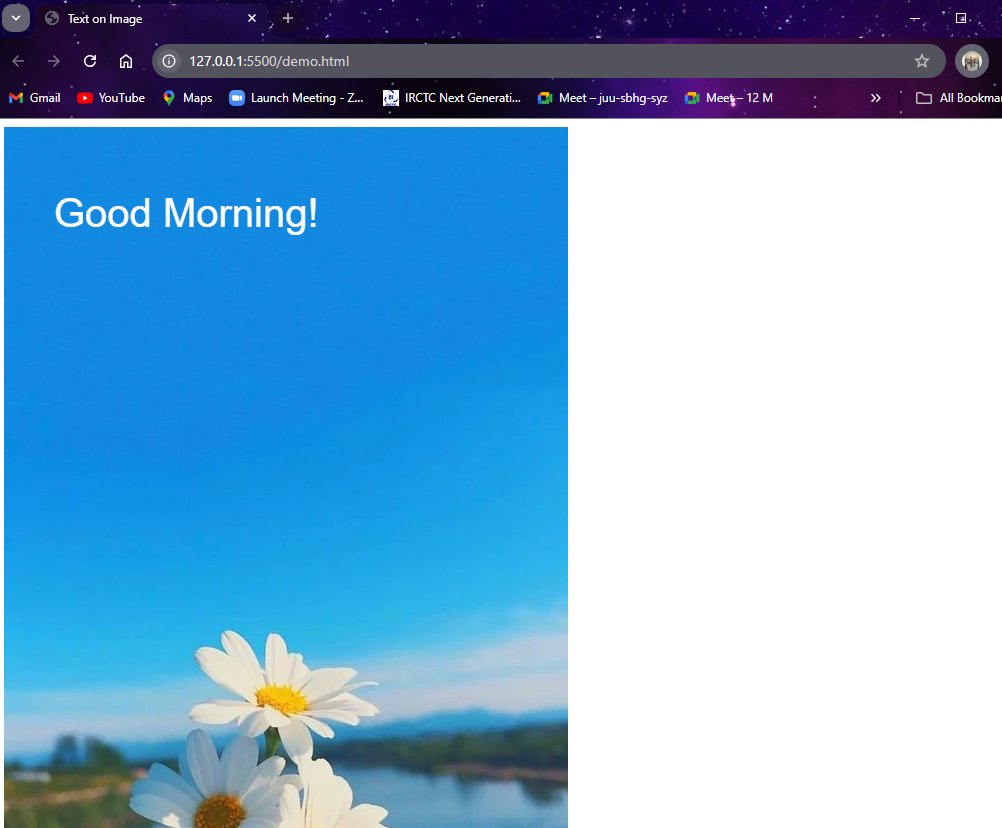
Step 6: Add Stroke and Outline Effects (Optional)
To add stroke and outline effects to our text, we can use the ‘strokeText()` method. We can also specify the stroke colour and width like this:
var image = new Image();
image.onload = function() {
var canvas = document.createElement("canvas");
var context = canvas.getContext("2d");
// Set canvas size to match the image
canvas.width = image.width;
canvas.height = image.height;
// Draw the image on the canvas
context.drawImage(image, 0, 0);
// Text customization
context.font = "40px Arial"; // Font size and style
context.strokeStyle = "black"; // Outline color
context.lineWidth = 4; // Outline thickness
context.fillStyle = "white"; // Text color
// Draw text with outline and fill
context.strokeText("Good Morning!", 50, 100); // Draw the outline of the text
context.fillText("Good Morning!", 50, 100); // Fill the text
// Display the canvas on the webpage
document.body.appendChild(canvas);
};
// Set the source of the image
image.src = "scene.jpg";
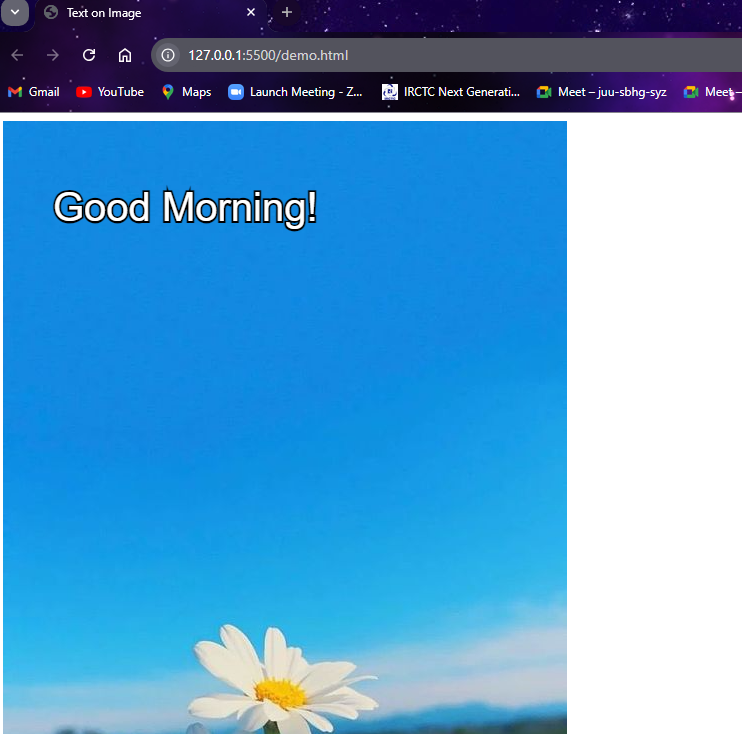
Summary
By following these simple steps, you will come to realize that with the use of JavaScript, you will be able to add text to an image. Adding text to an image will make it more meaningful and attractive, thus, adding value to your webpage. Many tools are available with most image editors for this purpose, but coding will be an effective one when flexibility and making changes are the main issues.
Learn the differences between Java and JavaScript by clicking here!
Reference
https://stackoverflow.com/questions/22942746/add-text-on-image-that-was-loaded-by-javascript