A template engine in Nodejs refers to a system that injects data into the HTML template of your app from the client side.
It allows us to embed JavaScript code directly into the HTML pages so that we can use JS methods, conditional statements, and loops. We can use conditional statements to determine which element of HTML to show and which to hide such as hiding the login button and showing the logout if the user is already logged in, this is just a use case, there are many.
Some of the best templating engine libraries available to use in Node.js are Pug, Handlebars, Jade, Mustache, Dust.js, etc but out of them, the best is EJS.
Now that we understand what are templating engines in Nodejs, let us now take a look at some benefits.
The benefits of using Templating Engines are as follow:
- Enhances the productivity of a developer.
- Improves the readability, usability and maintainability of your application.
- Fastens the performance of your application.
- Maximizes client-side data processing.
- Makes it easy to reuse templates on multiple pages.
- Enables accessibility of templates from CDNs also known as Content Delivery Networks.
This EJS tutorial will teach us to use the EJS template engine in Node.js. Throughout this guide, we will be using Express.js as our server framework so we will recommend you read our tutorial if you don’t have the basic knowledge of Express, click here to read.
EJS Template Engine in Node.js
EJS is the most popular template engine that allows you to generate HTML markup with pure JavaScript. It allows embedded JavaScript templating making it perfect for Node.js applications. It can be used for client-side as well as server-side rendering.
Simply put, the EJS template engine helps easily embed JavaScript into your HTML template.
Using EJS Template Engine in Node.js Project
To use the EJS template engine in your Nodejs project, we use Express which is best compatible with EJS.
1. Creating & Configuring Nodejs Project
Create a project folder and locate it in the terminal and type the below command to initiate npm.
npm init
NPM stands for Node Packages Manager, by which we can install any Node.js module.
Your package.json file should now be created and should look like this:
{
"name": "6_EJS_NODEJS_APP",
"version": "1.0.0",
"description": "",
"main": "index.js",
"scripts": {
"test": "echo "Error: no test specified" && exit 1"
},
"keywords": [],
"author": "",
"license": "ISC"
}
Create an index page where we will write Node.js code. You can create it manually or execute the below command in the terminal to create it directly.
touch index.js
Install the express and ejs as dependencies using npm which we have initiated previously.
npm install express
npm install ejs
Now that we have our packages installed, let us get started with setting up our Express server and integrating the EJS template engine with our front end.
const express = require('express');
const app = express();
const path = require('path');
// Setting EJS as templating engine
app.set('views', path.join(__dirname, 'views'));
app.set('view engine', 'ejs');
app.listen(3000, () => {
console.log("APP IS LISTENING ON PORT 3000!")
})
EJS template engine looks for a ‘views’ folder to render our HTML templates. These lines make sure that the ‘views’ folder is accessible globally:
const path = require('path');
app.set('views', path.join(__dirname, 'views'));
If you don’t get what the path module does, click here to read about it!
Output:
Up to this point, the index.js and file structure of your application must look like this.
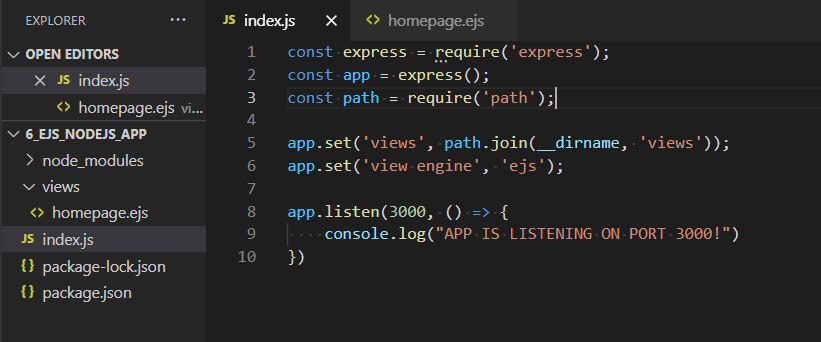
2. Creating EJS Templates
Create a ‘views’ folder in our project’s main directory if you haven’t. Inside it, create the homepage’s ejs file and name it homepage.ejs. Make sure your template file extension names consist of ‘.ejs’ and not ‘.html’ or ‘.htm’ otherwise your templates will fail to render.
Let us now set up our home page route in index.js and pass a name value in the form of an object (key-value pair):
app.get('/', (req, res) => {
res.render('homepage', { name: 'Monroe' })
})
Go ahead and configure a simple HTML template and try to render that name value on our home route via the EJS template engine from the client side. For the sake of simplicity, we have embedded CSS code into my HTML template.
homepage.ejs
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>
<%=name%>'s Sweets & Candy
</title>
<style>
body {
background-color: pink;
}
h1 {
color: darkslategrey;
text-align: center;
font-family: 'Gill Sans', 'Gill Sans MT', 'Calibri', 'Trebuchet MS', sans-serif;
}
p {
margin: 5%;
font-family: Verdana, Geneva, Tahoma, sans-serif;
border: deeppink 12px dashed;
padding: 2%;
}
</style>
</head>
<body>
<h1>Welcome to <%=name%>'s Sweets & Candy!</h1>
<p>Lorem ipsum dolor sit amet consectetur adipisicing elit. Neque officia sint explicabo non labore sit eaque quasi
deleniti ullam fugiat obcaecati blanditiis molestiae, praesentium dicta tempore laborum, similique, soluta
aliquam.
Dolores alias molestias quidem, culpa minima, repellendus sapiente suscipit, vero inventore error magnam quas
provident debitis? Tenetur repellat blanditiis perferendis laborum. Hic a qui eum aperiam, voluptatibus sint!
Dicta, totam?
Laudantium explicabo natus dolore aspernatur laboriosam perspiciatis provident hic facilis accusamus delectus
praesentium voluptates nobis quam, a, corrupti aliquid officiis. Eaque, corrupti nostrum repellat dolorum fuga
enim voluptas molestias obcaecati!
Beatae tempore quod esse distinctio ipsa commodi aperiam iure architecto, numquam illum delectus. Maxime cumque
reprehenderit, nam ut eligendi voluptate a assumenda similique ea quae quibusdam reiciendis explicabo sequi in?
Animi fuga aliquid enim repudiandae ratione optio inventore aspernatur eaque consectetur totam. Aut officia at
accusamus, commodi iste nostrum molestias sed. Harum quis libero iusto ea quae nostrum fuga nobis!</p>
</body>
</html>
Notice the title element and the H1 element consisting of the EJS template engine code, accepting the ‘name’ value. You can try your hands on and change the name value to see the changes.
Output:
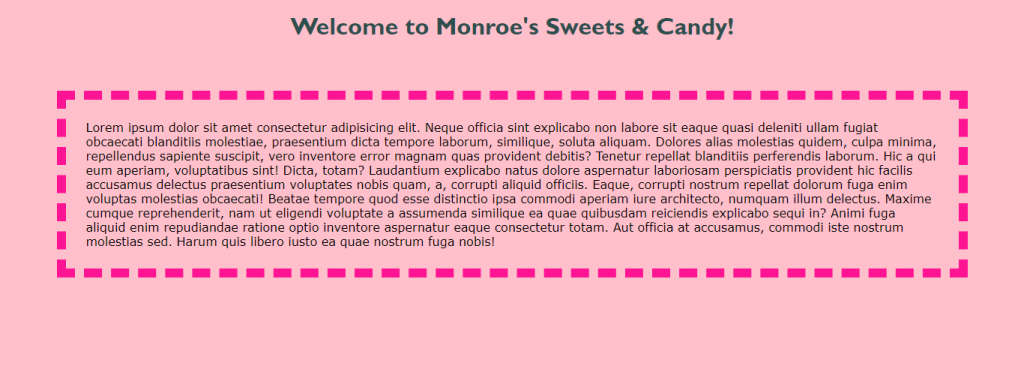
Conclusion
This tutorial explained the templating engines, and some of their benefits and briefed you about the EJS template engine in Node.js. EJS is the most popular, fast, reliable, and usable with other frameworks, so don’t forget to try it for your next Node.js project after reading this tutorial.
Reference
EJS Template Engine Official Website