File System module in Node.js provides a variety of functionalities, one of which is copying files from one location to another. In this tutorial, we will see both asynchronous and synchronous ways of doing this.
Copying a File in Node.js Using File System Module
Did you know Node.js is popular for writing asynchronous JavaScript code? This is what we are going to use. We will use its fs module’s asynchronous as well as traditional synchronous methods to copy files and folder contents.
- In an asynchronous method, the last argument is a completion callback which returns an error/exception so that if the operation fails, we can find and resolve the problem.
- In the synchronous method, any errors are thrown immediately which we can handle using a try/catch block.
1. Nodejs fs.copyFile() Function
The copyFile() method is used to copy files from one directory to another directory asynchronously. As default behaviour, if the file already exists at the specified destination, it overwrites it instead of appending new content to it.
Syntax:
fs.copyFile(src, dest[, mode], callback)
Parameters:
- src (String, Buffer, or URL) – Represents source file name to copy,
- dest (String, Buffer, or URL) – Represents destination file name to create,
- mode (Optional Integer) – Represents modifiers of the copy operation (by default it is 0),
- callback (Function) – A callback function to check for an error.
To use this method in the script, we first need to import the fs module which we can do using the require() module:
const fs = require('fs');
Example:
const fs = require('fs');
// Printing the current file name before copying
console.log("File Contents of sample_file:",
fs.readFileSync("sample_file.txt", "utf8"));
// Copying sample_file.txt to a different name
fs.copyFile("sample_file.txt", "async_copied_file.txt", (err) => {
if (err) {
console.log("Oops! An Error Occured:", err);
} else {
// Printing the current file name after executing the function
console.log("File Contents of async_copied_file:",
fs.readFileSync("async_copied_file.txt", "utf8"));
}
});
Here we first imported the file system module, then used readFileSync() to print the content of our file, then used copyFile() to copy the content of the file to a new file path. The callback prints the error if any or prints the newly created file content.
sample_file.txt:
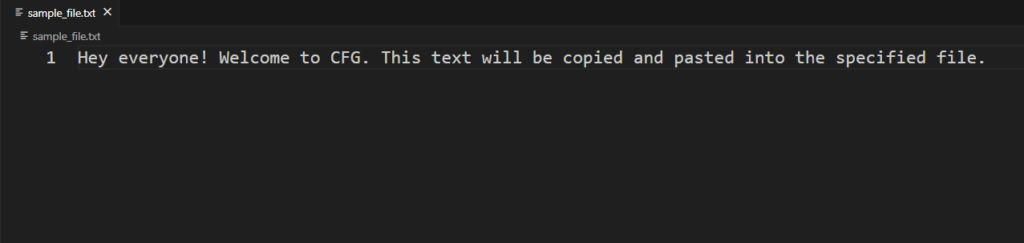
Output:
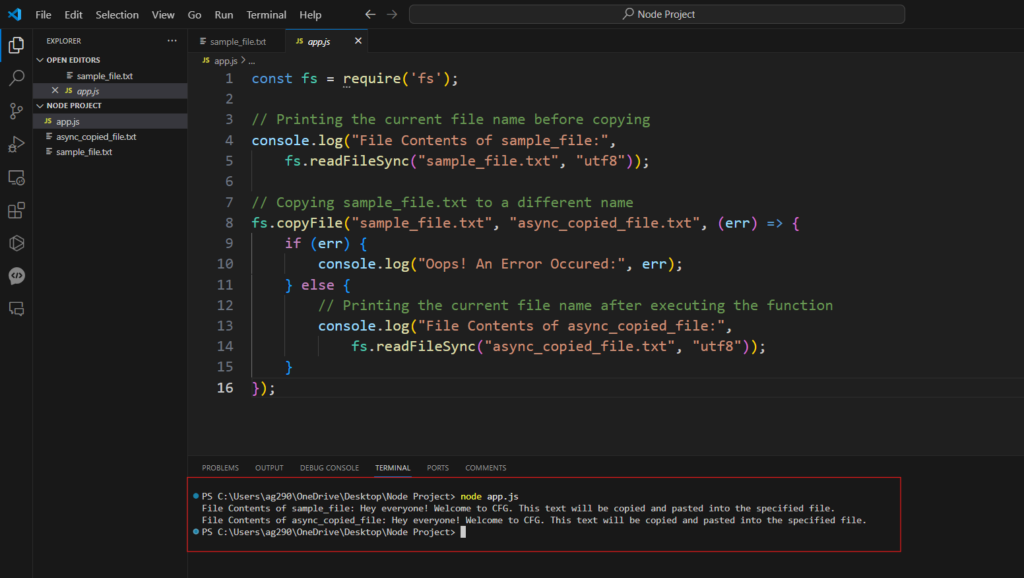
In the above output, you can see that the content of the newly created file is the same as the content of the previous file, so this async method copied the file successfully.
2. Nodejs fs.copyFileSync() Function
The copyFileSync() method is the fastest way to copy files synchronously in Node.js.
Syntax:
fs.copyFileSync(src, dest[, mode])
Parameters:
- src (String, Buffer, or URL) – Represents source file name to copy,
- dest (String, Buffer, or URL) – Represents destination file name to create,
- mode (Optional Integer) – Represents modifiers of the copy operation (by default it is 0),
Example:
Let’s see an easy example of this method as well.
const fs = require('fs');
// Printing the current file name before copying
console.log("File Contents of sample_file:",
fs.readFileSync("sample_file.txt", "utf8"));
// Copying sample_file.txt to a different name
fs.copyFileSync("sample_file.txt", "sync_copied_file.txt");
// Printing the current file name after executing the function
console.log("File Contents of sync_copied_file:",
fs.readFileSync("sync_copied_file.txt", "utf8"));
Here we first imported the file system module, then used readFileSync() to print the contents of the file, then used copyFileSync() to copy the content to a new file, and finally printed the newly created file.
Output:
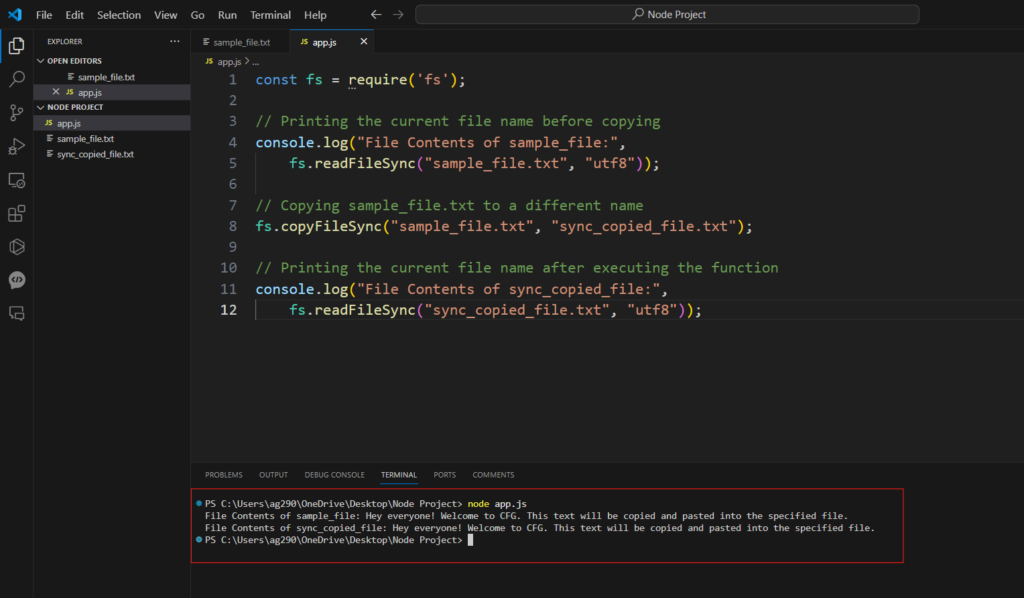
This way we have successfully copied a file from a source to a destination synchronously.
Conclusion
Just like we copy data, copying a file from one location to another is an essential feature and in Node.js we can use both asynchronous (using fs.copyFile) and synchronous (using fs.copyFileSync) ways of doing this. We can access these functions by using the file system module.
If you are new to Node.js:
- What is Node.js?
- Asynchronous Programming in Node.js – Callback, Promises & Async/Await
- How to send an HTML File in Node.js?
References
- https://nodejs.org/api/fs.html#fscopyfilesrc-dest-mode-callback
- https://nodejs.org/api/fs.html#fscopyfilesyncsrc-dest-mode