Hello coders! Welcome to a new chapter of Python guides. Today, we will see how to calculate absolute values in Python using NumPy. We’ll explore the NumPy fabs() function, understand its syntax, and how it works, and see examples, demonstrations, and its drawbacks. We will also see how to address the given disadvantages. So, stay tuned and follow along!
What Is Absolute Value?
The absolute value of a number is like its distance from zero on a number line. It shows how far the number is from zero, no matter if it’s positive or negative. For example, the absolute value of 6 is 6, and the absolute value of -6 is also 6. We write it with two straight lines around the number, like |x|.
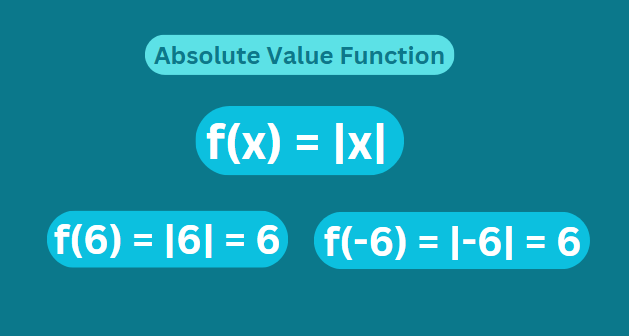
When a number is positive, its absolute value stays the same. But when a number is negative, you just take away the minus sign to get its absolute value. And when the number is zero, its absolute value is also zero because it’s right on top of zero on the number line.
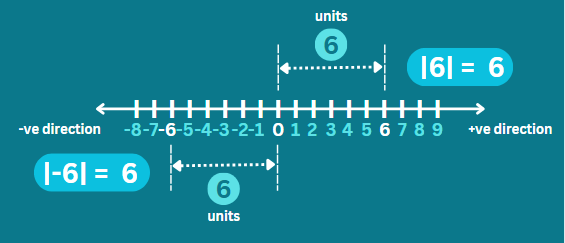
Why Do We Need Absolute Value?
Here’s why:
- It helps us know how big a number is, ignoring whether it’s positive or negative. This is helpful with things like distances or sizes, where the direction doesn’t matter.
- It is crucial in solving equations and inequalities.
- In geometry and physics, we use absolute value to find distances between points or other things we’re studying in math.
- In computer programming, absolute value functions are used a lot for different reasons, like dealing with errors, organizing data, and doing math calculations.
numpy.fabs() in Python
Numpy offers us a fabs() function, short for “absolute value“, it helps find the absolute value of numbers, whether it’s one number or a bunch of them stored in a list.
Syntax:
The syntax for using fabs() is simple. You just need to call it as a function from the numpy module and give it the number or list of numbers you want to find the absolute values for. It looks like this:
numpy.fabs(x)
Here, x can represent various types of inputs:
- A scalar (single numeric value)
- A one-dimensional array (numpy array, list, tuple, etc.)
- A multidimensional array
How Does It Work?
If you give the function just one number, it finds the absolute value of that number and gives it back to you. If you give it a bunch of numbers in an array, it goes through each number one by one, finding the absolute value for each. If it’s a simple list of numbers, it finds the absolute value of each number individually. But if it’s a more complicated array with multiple layers, it still does the same thing, going through each number and finding its absolute value.
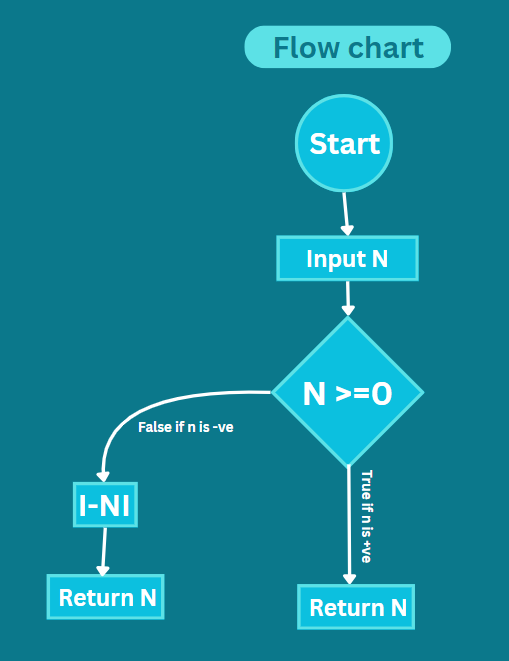
The fabs() function works well with whole numbers, but it struggles with decimals and complex numbers. If you use fabs() with arrays containing decimals, complex numbers or NaN values, it might give wrong results or cause errors. To handle decimals and complex numbers correctly, you should use np.abs() instead.
Finding Absolute Values Using numpy.fabs() in Python
Now that we know a bit about this function, let’s try to understand it more using code examples.
Example 1: Firstly we will test with scalar input.
import numpy as np
number = int(input("Enter the Number : "))
print("The absolute value of the given value is: ", np.fabs(number))
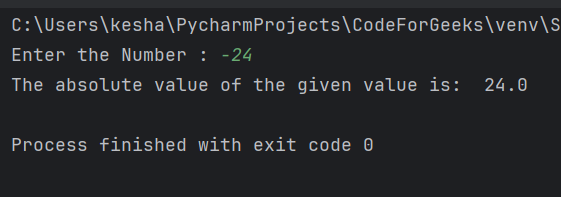
Example 2: Now let’s provide an array input.
import numpy as np
arr = np.array([0, -5, 10, 15, -20])
print("The array containing the absolute values of all elements is: ", np.fabs(arr))

Example 3: Let’s see what happens with a 2-D array.
import numpy as np
arr = [[0, -5, 10], [2, 3, -6], [7, -9, 13]]
print("The array containing the absolute values of all elements is: ", np.fabs(arr))
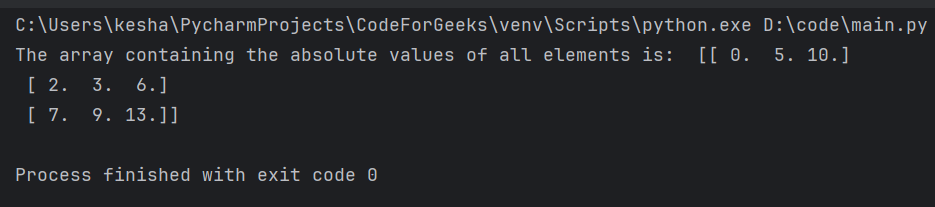
Example 4: If we input complex, decimal, or NaN values, the function will throw an error as it does not support this type of input.
import numpy as np
array_2d = np.array([
[1.5, 2.3, np.nan, 4.7 + 3.2j],
[5.6, 6.8, 8.1, 9.4],
[10.2, np.nan, 12.5 + 1.8j, 13.9]
])
print("The array containing the absolute values of all elements is: ", np.fabs(array_2d))

Finding Absolute Values Using numpy.abs() in Python
There are some drawbacks to the fabs() function. Python’s abs() functions work as a powerful alternative to this problem. This function finds how far a number is from zero, no matter if it’s positive or negative. For example , abs(-2) gives 2, and abs(2) also gives 2. It works with different types of numbers like integers, decimals, and complex numbers.
Syntax:
The root syntax for this function is:
abs(a)
Where “a” is the number or array you want to find the absolute value of.
Example:
Let’s understand using the previous example of fabs() where we got stuck.
import numpy as np
array_2d = np.array([
[1.5, 2.3, np.nan, 4.7 + 3.2j],
[5.6, 6.8, 8.1, 9.4],
[10.2, np.nan, 12.5 + 1.8j, 13.9]
])
print("The array containing the absolute values of all elements is: ", np.abs(array_2d))
In this result, we clearly see that the abs() function sorts out our problem! It prints the absolute value of complex, decimal, and NaN values.

Conclusion
And that’s it for this guide! We’ve covered every important detail of the fabs() and abs() functions. We’ve gained a great understanding of what absolute value is and its importance. We’ve learned how to implement it in Python with some good examples. I hope you’ve followed along well and enjoyed it.
You may also learn how to calculate differences easily and also how to round numbers in Python.
Reference
https://numpy.org/doc/stable/reference/generated/numpy.fabs.html