Are you tired of finding a way to auto-refresh your Node.js application? Well, you are not alone, I also searched a lot on the internet, even using new-age AI, but couldn’t find the solution. This auto-refresh issue is not new to Node.js and websites that use it already suffer from it, but you know there is a hack to make it work.
After reading this tutorial, you will be able to make your Node.js applications fully dynamic. Either you want to auto-refresh when new data comes in or make a real-time connection to the database or other things, then you can use my method to implement real-time auto-refresh without actually refreshing the entire page. Let’s see.
Creating a Simple Node.js Application
Let’s first create a simple Node.js and Express application.
Step 1: Create a project folder, and open it inside a code editor.
Step 2: Open a terminal and execute the below command to install the Express and EJS modules.
npm i express ejs
If EJS Is New to You – Using the EJS Template Engine in Node.js
Step 3: Create an ‘app.js’ file and write the below code inside it.
const express = require('express');
const path = require('path');
const app = express();
app.set('view engine', 'ejs');
app.set('views', path.join(__dirname, 'views'));
app.get('/', (req, res) => {
const currentTime = new Date().toLocaleString();
res.render('index', { currentTime: currentTime });
});
app.listen(3000, () => {
console.log('Server is running on http://localhost:3000');
});
This is very basic Express code which first imports the ‘express’, ‘path’ and ‘ejs’ modules, then creates an instance of ‘express’ to get its methods, then sets EJS as a view engine, then use the get() method to create a router for the home route “/” which render the ‘index.ejs’ file with the current time. Finally, it uses the listen() method to specify that the server runs on port 3000.
Step 4: Create an ‘index.ejs’ file inside the ‘views’ directory which shows our frontend.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>A Node.js Application</title>
</head>
<body>
<h1 id="currentTime"><%= currentTime %></h1>
</body>
</html>
Output:
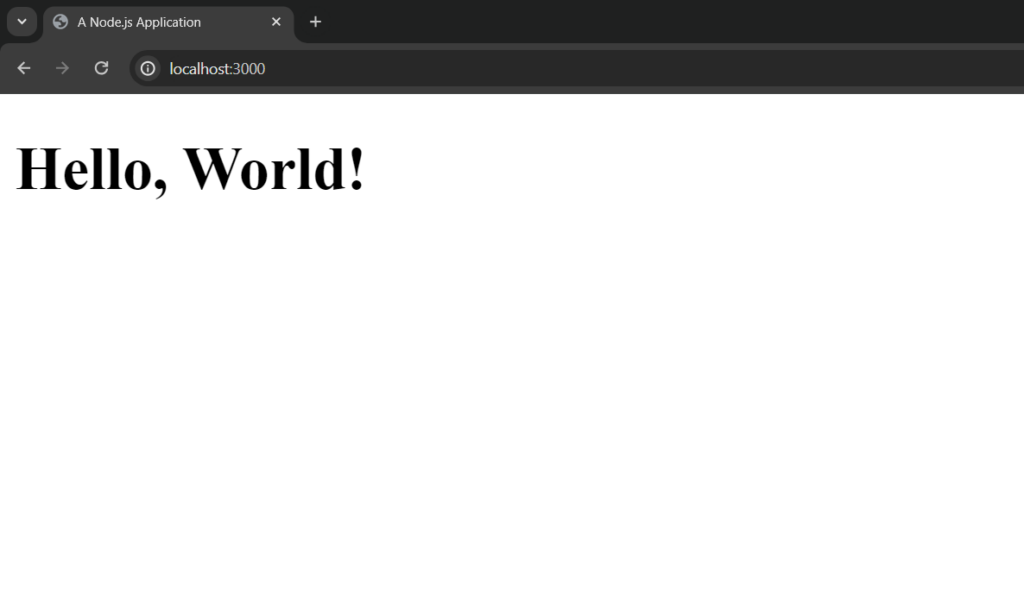
Now the question is how we can automatically load the new upcoming data from the server, for instance, our Node.js code sends the current date and time, and we want to fetch it continuously, how can we do that?
Well, we can use the setInterval() method but then we have to load the page again and again.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>A Node.js Application</title>
</head>
<body>
<h1 id="currentTime"><%= currentTime %></h1>
<script>
setInterval(function() {
location.reload();
}, 5000);
</script>
</body>
</html>
Output:
Updating Page Values Without Refresh
Above is just an example, there are a lot of methods on the internet to solve this auto refresh problem but none of them are right. You can’t use setInterval() directly to get the content without refreshing the page, but here’s what you can do.
You can create a new route that sends real-time data from the server to the client in JSON. Then on the client side, you can make an AJAX request to the server to get that data and you can make this AJAX request every second using setInterval() and this starts getting real-time data from the server without refreshing the page.
If jQuery Is New to You – Introduction to jQuery & Creating Clones of Objects
Let’s implement this using jQuery:
Step 1: Add a new route to send current data and time in JSON.
app.get('/getData', (req, res) => {
const currentTime = new Date().toLocaleString();
res.json(currentTime);
});
Step 2: Update the HTML code to use jQuery().
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>A Node.js Application</title>
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
</head>
<body>
<h1 id="currentTime"><%= currentTime %></h1>
<script>
function refreshContent() {
$.get('/getData', function(data) {
$('#currentTime').empty().append(data);
});
}
refreshContent();
setInterval(refreshContent, 1000);
</script>
</body>
</html>
Here we are first using jQuery CDN, then using <script> tag we are writing jQuery code, the code creates a function that makes an AJAX request and sends a GET request to the ‘/getData’ route which we have created in the previous step, which send the current date and time. Then it appends the fetch data to our h1 tag using append() and removes any previous value using empty(). Then we call the function.
Note that we are using the setInterval() method so that the fucntion can be called every 1 minute as we want to update time in real-time.
Output:
See we are fetching real-time data from the server even without page refresh.
Conclusion
In short, the best way to make the auto-refresh functionality is to create a new route on the server side that sends the real-time data you want to get, then on the client side make an AJAX request to the server per second to the same route you created and append the data to where you want.
Reference
https://stackoverflow.com/questions/32445295/updating-values-on-page-without-refreshing-in-node-js