Did you know about the clone method in jQuery? By the word clone, it is clear that we are trying to make a copy of something. You will be amazed to know that we can make a copy or you might say a clone of any JavaScript logic of a particular element and use the same in some other element. In this article let’s delve into the very useful jQuery method i.e. the clone() method and learn its functions, working and use with a real-life fun project so without any further due, let’s get started!
Introduction to jQuery Library
Don’t you find the JavaScript DOM (Document Object Model) a little tedious and complex? If you do then you would love jQuery. It is a kind of substitute for DOM in JavaScript which is loved by developers all around the world and highly preferred over DOM due to the ease of its use. It is a JavaScript library that is concise, quick and rich with more features. It highly simplifies the use of event handling, Ajax, animation, document traversal and manipulation. It provides a readymade API that is feasible across a multitude of browsers.
Key Features of jQuery Library
Some of the key features of jQuery library are listed below:
- jQuery makes it easy to interact with JavaScript DOM and work with the HTML elements as it provides a readymade API.
- The Event handling events such as clicks, keypresses, and other user interactions are easily optimized by jQuery because it provides simple methods to attach event handlers to elements.
- Asynchronous JavaScript and XML or Ajax use is simplified as jQuery includes methods for making asynchronous HTTP requests. It makes it easy to load data from a server without refreshing the entire page.
- Animations such as fading in/out, sliding up/down, and more are created with ease, also various effects are applied to elements on a web page.
- jQuery makes your API browser versatile and pushes away many of the browser-specific complexities. In short, it provides a consistent API that works across all kinds of different web browsers.
- jQuery comes with a bulk collection of plugins which helps it to add new features and improves functionality.
- One of the features that makes jQuery distinctive is the chaining. You can code more than one function or action to an element in a single line.
To use jQuery in your file, you must first include its library in your HTML code. Add the following script tag to your HTML file:
<script src="https://code.jquery.com/jquery-3.6.4.min.js"></script>
Syntax:
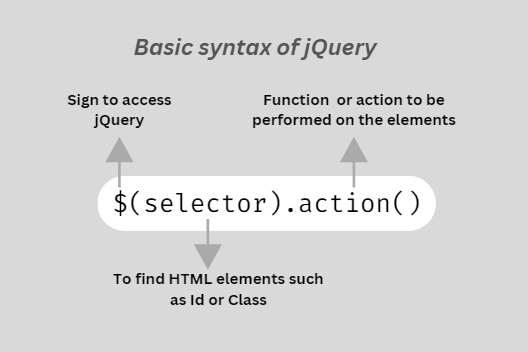
Here is a basic example of selecting and working on an element using jQuery:
$(".pokemon").hide()
This hides all elements with class=”pokemon”.
Methods of jQuery Library
Some very basic and most used methods of jQuery are listed below:
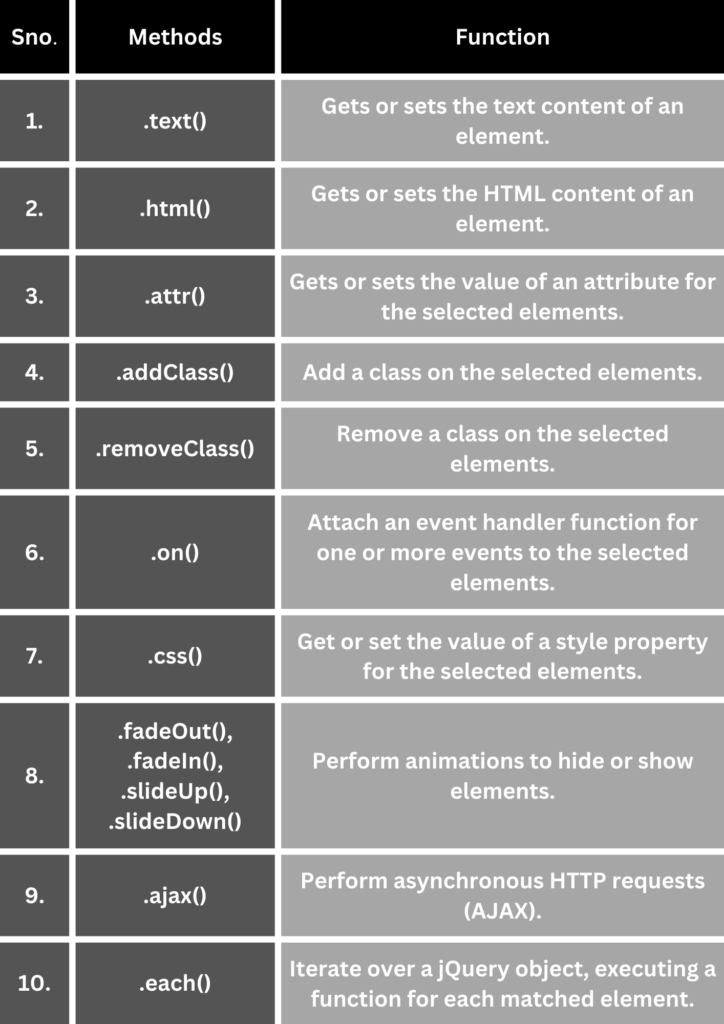
Got fascinated with jQuery, we have a whole lot of separate articles on it so do check out:
One such useful method is the .clone( ) method, let’s discuss about it.
jQuery clone() Method
As the name suggests, this method is used to “clone” or make a deep copy of the selected elements. It helps to duplicate elements efficiently. We select a jQuery object which represents the element we want to clone. The .clone() method creates a copy of this element and all its children.
Syntax:
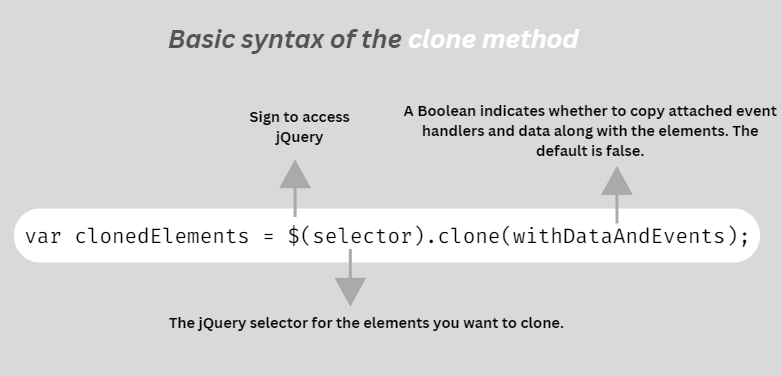
Creating Clones of Objects Using the clone() Method
There are mainly two ways to clone, both are briefed below:
Normal Cloning:
It is the general way of using the clone method. Basically using the given method without any specific parameters and functions. We first select the given element using jQuery and then just add “.clone( )” to it.
var adibas = $('.adidas').clone();
The above line of code just creates a simple deep copy of the selected element along with its child nodes and stores it in the given variable.
Cloning with Events and Data:
Normally, while cloning, any event handlers attached to the original element are not copied to the clone. This is because the default value for this method is false. Yes, you got it right! It is a Boolean type. In simple words, if you wish to copy the event handlers and data of the selected element along with the clone of the selected element, we use withDataAndEvents. Which means you need to assign “true” or “false” in the braces.
var pooma = $('.puma').clone(true);
The above line of code creates a clone along with the event handlers attached to the original. You can also enhance the given code using deepWithDataAndEvents which would additionally copy the events and data for all children of the cloned element.
Still not clear with the clone method? No worries, let’s make it clearer using a real-life example.
How to Use the clone() Method?
Have you ever seen Pokemon? Did you know about a Pokemon called Ditto? It had a special power to clone itself into any Pokemon. So let’s try to build a website that has a Pokemon and a Ditto button which would clone that particular Pokemon as many times as the button is clicked.
Consider the following HTML code:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<link rel="stylesheet" href="style.css">
<title>Ditto workspace</title>
<script src="https://code.jquery.com/jquery-3.6.4.min.js"></script>
</head>
<body>
<h2>Hello and welcome to Ditto's workspace</h2>
<h3>Click on the Ditto button to clone your Pokemon</h3>
<div class="pokemon-container">
<img src="pikachu.jpg" alt="Pikachu">
<div class="clone-container"></div>
</div>
<button id="cloneButton">Ditto</button>
<script src="script.js"></script>
</body>
</html>
This HTML document sets up a page with text, an image of Pikachu, and a button, and intends to use jQuery for cloning functionality. We also import the jQuery library and add external CSS and Script files.
This code results in a page like this:
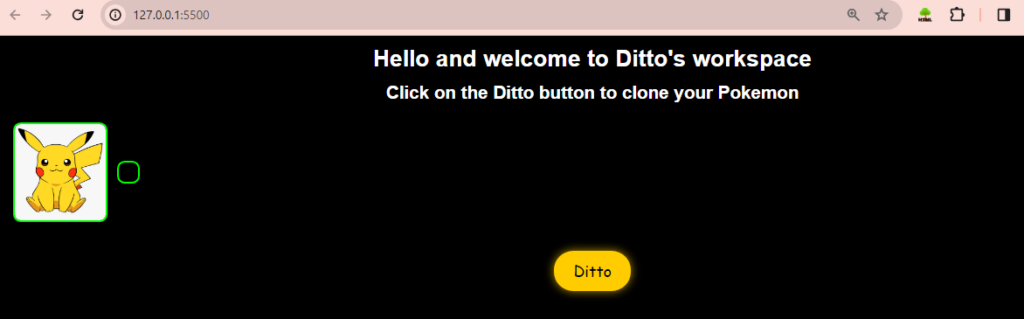
Now to make the “Ditto” button work, we need to add some JavaScript logic, something like this:
$(document).ready(function() {
$('#cloneButton').on('click', function() {
var clonedPikachu = $('.pokemon-container img').clone();
$('.clone-container').empty().append(clonedPikachu);
});
});
The above is the code snippet from the script.js. Here is a brief explanation of it:
- $(document).ready(function() { – When the document is fully loaded and ready, execute the following code.
- $(‘#cloneButton’).on(‘click’, function() { – When the element with ID ‘cloneButton’ is clicked, do the following.
- var clonedPikachu = $(‘.pokemon-container img’).clone(); – It says to create a variable called ‘clonedPikachu’ and store a copy of the image within the element with the class ‘pokemon-container’.
- $(‘.clone-container’).empty().append(clonedPikachu); – Does two things. First, it removes any content inside the element with the class ‘clone-container’. Second, it adds the cloned Pikachu image to the ‘clone-container’.
Now that you know how the code would work, let’s test it out. We will click on the “Ditto” button again and again to see if our Pikachu has cloned or not.
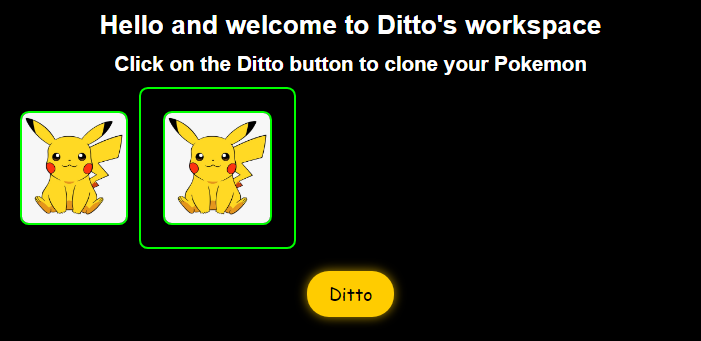
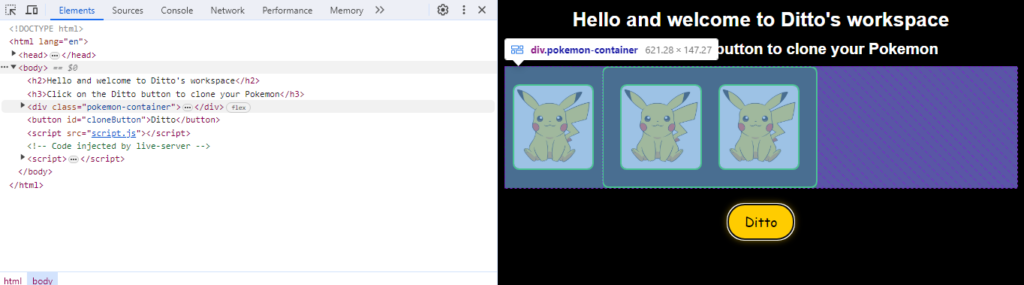
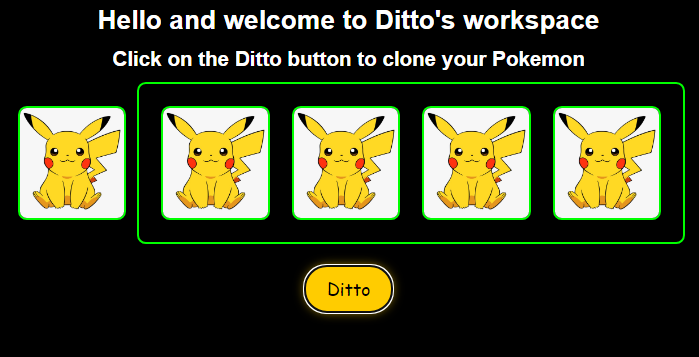
And yay! You have just made a site that portrays the power of the Ditto Pokemon using the clone method.
Things to keep in mind:
- You can modify cloned elements before inserting them with the clone() method.
- It is always suggested to use the class selectors while using the clone method as the ID selectors are supposed to be unique.
Conclusion
In this article, we have tried to provide a detailed explanation of the syntax, the cloning process, and the optional parameters for cloning events and data. We hope that this helped you to build a strong understanding of the clone() method in jQuery. We hope that you enjoyed reading how to use the clone method using the real-life example involving Pokémon Ditto. And we heartily apologize if you are not a Pokemon fan.