Node Package Manager (NPM) is a package manager for Node.js. It provides many commands to manage our dependencies or packages inside a Node.js project.
Packages and dependencies are external modules that make our coding life easy by letting us implement additional functionalities. We can install a package using npm and import it inside a project using the require() statement.
NPM with all its functionalities allows us to uninstall a package as well. Removing a package or dependency is helpful in various scenarios such as when you no longer need it or you want to reinstall it due to some errors.
In this article, we will learn how we can uninstall npm packages or dependencies from our Node.js project.
Also Read: Why does “npm install” rewrite package-lock.json?
NPM Uninstall Local Dependency
Local packages are the npm modules that are installed in a directory when you run npm install <package_name>, and are put in the node_modules folder under this directory. The name of such packages is also referenced as keys in different dependencies sections in the package.json file of the repository.
To uninstall a local package we can use the uninstall command from npm. Doing so will remove everything npm installed on its behalf, the package from node_modules, and its reference in any dependencies, devDependencies, optionalDependencies, and peerDependencies objects in package.json.
Syntax:
npm uninstall <package_name>
If your package has a scope you may also add the scope of the package before package_name.
npm uninstall <@scope/package_name>
Example:
To explain the npm uninstall command, I have taken an existing Node.js project where you can see nodemon is installed as a dev dependency. For reference, a dev dependency is a package that is only used during development.
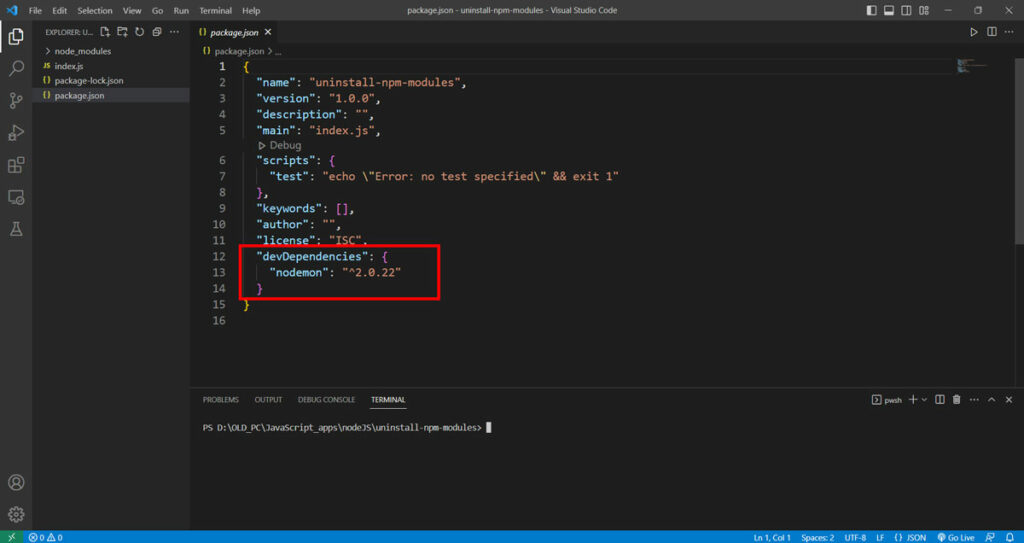
Run the following commands in your terminal to uninstall and remove nodemon from the project:
npm uninstall nodemon
Output:
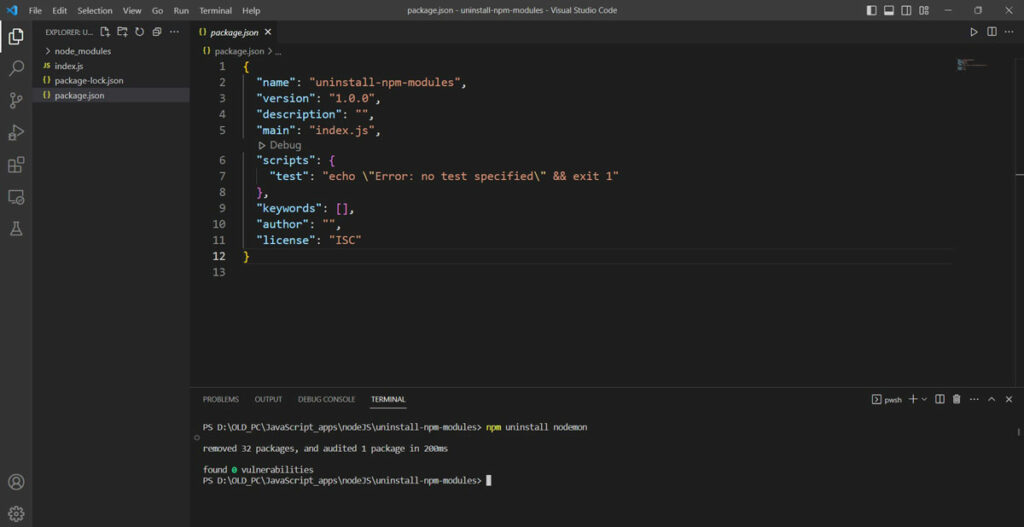
You can find nodemon has been completely removed from the local project. Secondly, the devDependencies section has also been removed because there are no devDependencies in the project.
Using npm uninstall to Remove Local Packages from node_modules Without Updating package.json
Additionally, we can also remove everything that belongs to the local package but still keep its reference in the project’s package.json file using a –no-
Syntax:
npm uninstall --no-save <package_name>
Example:
In this example, you can see we have express installed in our Node.js project and our node_modules folder has an express folder along with other dependencies of the express package.
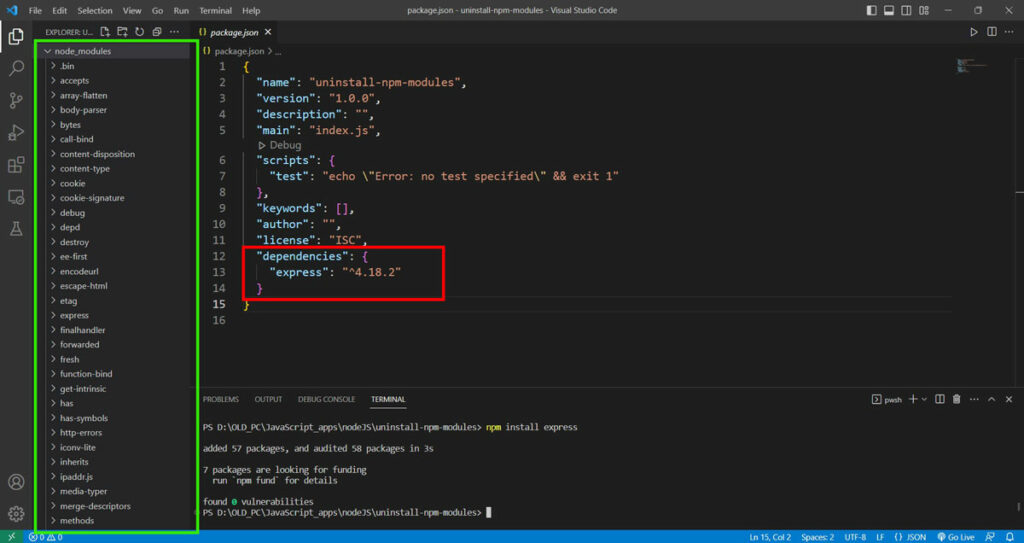
Now let’s try uninstalling the package with the –no-save flag
npm uninstall --no-save express
Output:
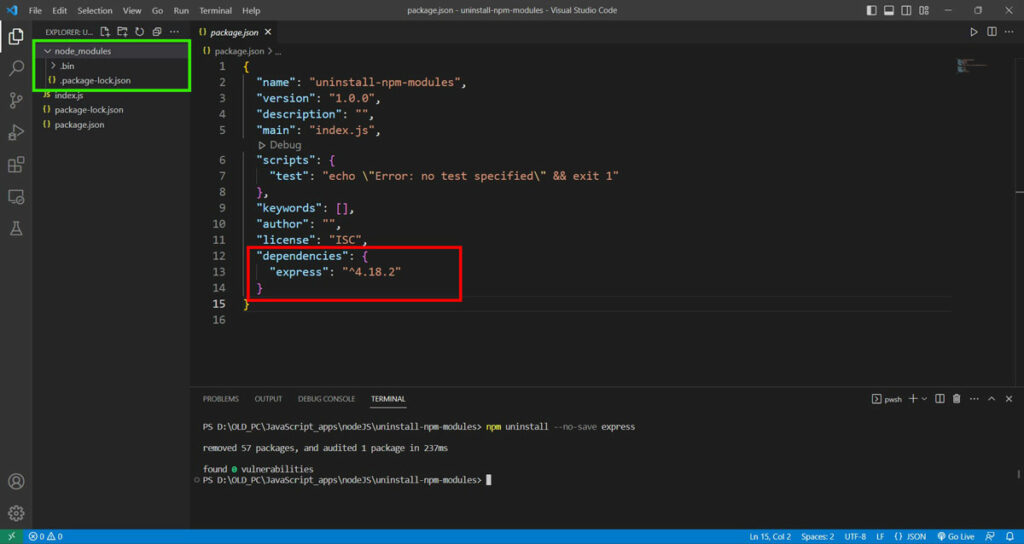
We can see that everything that was installed with express including express has been removed from our node_modules however, a reference to express has not been lost in our package.json.
NPM Uninstall Global Packages
Unlike local packages which can be found in the node_modules of our project, global packages are installed at some place in our system depending on our Node installation and operating system irrespective of where we run npm install-g <package_name>.
To uninstall a global package from the system we need to use the -g flag with the uninstall command.
Syntax:
npm uninstall -g <package_name>
Example:
Open any terminal/PowerShell and run the below command.
npm list -g
This will give you the list of globally installed npm modules. In my system, I have the following packages installed.
+-- [email protected]
+-- [email protected]
+-- [email protected]
+-- [email protected]
+-- [email protected]
+-- [email protected]
+-- [email protected]
+-- [email protected]
+-- [email protected]
+-- [email protected]
+-- [email protected]
`-- [email protected]
To follow along you may install any package such as React globally using the following command:
npm install -g react
Now, we will uninstall the globally installed react package with the following command:
npm uninstall -g react
Output:
Once the uninstallation is over, again run npm list -g to see the new list of globally installed packages and as expected we won’t find our React package in the list.
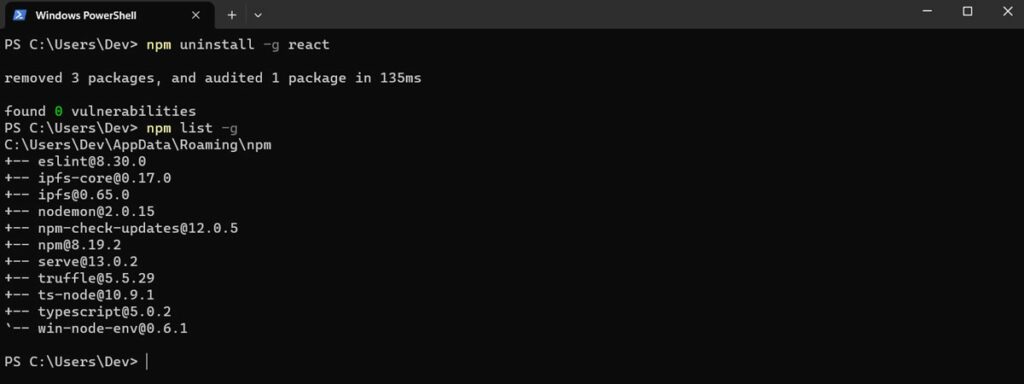
Frequently Asked Questions (FAQs)
How to install npm dependencies?
We can install npm dependencies using the command ‘npm install package_name’. This will install specified package and their dependencies in Node.js.
How to check npm packages installed?
NPM provides us with commands like ‘npm ls‘ or ‘npm list’ to show all the packages installed. These commands list all the packages from the project’s root directory.
How to delete package version from npm?
You can use the npm uninstall command and specify a package name with the version you want to uninstall. Open the terminal and execute the ‘npm uninstall package_name@version’ command.
How to remove and reinstall npm?
First, it is required to clear all the cache using the command ‘npm cache clean -f’ then run ‘npm install -g npm’ to reinstall the latest version of npm globally.
How do I uninstall all npm packages globally?
There is no such particular command to uninstall all npm packages globally but we can combine some commands to do the operation. Run ‘npm ls -q | xargs npm -g rm’ command, this lists all packages installed and uninstalls them globally.
How to update npm packages?
You can run the ‘npm update’ command to update all packages in a project to their latest version.
Conclusion
NPM is not just the default package manager for Node.js but is extensively feature-rich when it comes to managing packages. In this article, we covered some basics of different installations of npm modules and then discussed how we can uninstall them from our local Node project’s node-modules directory and also globally from the system.
Wondering what to read next? Learn about the –save option for npm install.
References
- https://docs.npmjs.com/uninstalling-packages-and-dependencies
- https://stackoverflow.com/questions/13066532/how-can-i-uninstall-npm-modules-in-node-js